node connector for us
Embed:
(wiki syntax)
Show/hide line numbers
CushionNodeConnector.h
00001 #ifndef CUSHION_NODE_CONNECTOR_H 00002 #define CUSHION_NODE_CONNECTOR_H 00003 00004 #include "mbed.h" 00005 #include "SocketIO.h" 00006 #include "picojson.h" 00007 00008 00009 class CushionNodeConnector 00010 { 00011 public: 00012 int MESS_STOP; 00013 int MESS_START; 00014 int MESS_SIT; 00015 int MESS_FRUST; 00016 00017 /* 00018 Constructor 00019 @param hostAndport format : "hostname:port" 00020 */ 00021 CushionNodeConnector(char * hostAndPort); 00022 /* 00023 Constructor 00024 if you didn't use any paramators, connect to chikura-server. 00025 */ 00026 CushionNodeConnector(); 00027 00028 00029 /* 00030 connect to server 00031 @return true if the connection is established, false otherwise 00032 */ 00033 bool connect(); 00034 00035 /* 00036 send message to server 00037 @return the number of bytes sent 00038 @param type Message type(prease show and choose from line 14 to 17 in souece code) 00039 @param msg message payload 00040 */ 00041 int mess_send(int type, char * msg); 00042 00043 /* 00044 Recieve message from server 00045 @return if recieved succsessful, Message type(prease show and choose from line 14 to 17 in souece code), otherwise returns -1. 00046 @param msg pointer to the message to be read. 00047 */ 00048 int mess_recv(char * msg); 00049 00050 /* 00051 To see if there is a SocketIO connection active 00052 @return true if there is a connection active 00053 */ 00054 bool is_connected(); 00055 00056 bool close(); 00057 00058 private: 00059 SocketIO *socketio; 00060 std::map<int,string> type_name; 00061 std::map<string,int> name_type; 00062 }; 00063 00064 #endif
Generated on Tue Jul 12 2022 18:27:01 by
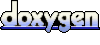