node connector for us
Embed:
(wiki syntax)
Show/hide line numbers
CushionNodeConnector.cpp
00001 #include "CushionNodeConnector.h" 00002 00003 CushionNodeConnector::CushionNodeConnector(char * hostAndPort){ 00004 socketio= new SocketIO(hostAndPort); 00005 MESS_STOP = 0x01; 00006 MESS_START = 0x02; 00007 MESS_SIT = 0x11; 00008 MESS_FRUST = 0x12; 00009 type_name[MESS_STOP]="stop"; 00010 type_name[MESS_START]="start"; 00011 type_name[MESS_SIT]="status_sit"; 00012 type_name[MESS_FRUST]="status_frust"; 00013 name_type["stop"]=MESS_STOP; 00014 name_type["start"]=MESS_START; 00015 name_type["status_sit"]=MESS_SIT; 00016 name_type["status_frust"]=MESS_FRUST; 00017 } 00018 00019 CushionNodeConnector::CushionNodeConnector(){ 00020 CushionNodeConnector("please enter your default hostname"); 00021 } 00022 00023 bool CushionNodeConnector::connect(){ 00024 bool ret=socketio->connect(); 00025 return ret; 00026 } 00027 00028 int CushionNodeConnector::mess_send(int type, char * msg){ 00029 string name=type_name[type]; 00030 char chname[16]; 00031 std::strncpy(chname, name.c_str(), 16); 00032 int ret=socketio->emit(chname,msg); 00033 return ret; 00034 } 00035 00036 int CushionNodeConnector::mess_recv(char * msg){ 00037 picojson::value v; 00038 char recv[256]; 00039 if (socketio->read(recv)) { 00040 int type; 00041 string name; 00042 string sbuf(recv); 00043 const char * crecv=sbuf.c_str(); 00044 string err = picojson::parse(v,crecv,crecv+strlen(crecv)); 00045 if(!err.empty()) 00046 return -1; 00047 name=v.get("name").get<string>(); 00048 strncpy(msg,v.get("args").get<string>().c_str(),256); 00049 type=name_type[name]; 00050 return type; 00051 }else{ 00052 return -1; 00053 } 00054 } 00055 00056 bool CushionNodeConnector::is_connected(){ 00057 return socketio->is_connected(); 00058 } 00059 00060 bool CushionNodeConnector::close(){ 00061 return socketio->close(); 00062 }
Generated on Tue Jul 12 2022 18:27:01 by
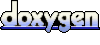