
Example program to demonstrate the use of the UbloxATCellularInterfaceExt class, providing FTP, HTTP, and CellLocate support. It may be used on the C027 and C030 (non-N2xx flavour) boards.
Dependencies: gnss ublox-at-cellular-interface-ext ublox-cellular-base ublox-cellular-driver-gen
Fork of example-ublox-at-cellular-interface-ext by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "UbloxATCellularInterfaceExt.h" 00019 #include "gnss.h" 00020 00021 // The credentials of the SIM in the board. If PIN checking is enabled 00022 // for your SIM card you must set this to the required PIN. 00023 #define PIN NULL 00024 00025 // Network credentials. You should set this according to your 00026 // network/SIM card. For C030 boards, leave the parameters as NULL 00027 // otherwise, if you do not know the APN for your network, you may 00028 // either try the fairly common "internet" for the APN (and leave the 00029 // username and password NULL), or you may leave all three as NULL and then 00030 // a lookup will be attempted for a small number of known networks 00031 // (see APN_db.h in mbed-os/features/netsocket/cellular/utils). 00032 #define APN "portalmmm.ml" 00033 #define USERNAME NULL 00034 #define PASSWORD NULL 00035 00036 // LEDs 00037 DigitalOut ledRed(LED1, 1); 00038 DigitalOut ledGreen(LED2, 1); 00039 DigitalOut ledBlue(LED3, 1); 00040 00041 // The user button 00042 volatile bool buttonPressed = false; 00043 00044 static void good() { 00045 ledGreen = 0; 00046 ledBlue = 1; 00047 ledRed = 1; 00048 } 00049 00050 static void bad() { 00051 ledRed = 0; 00052 ledGreen = 1; 00053 ledBlue = 1; 00054 } 00055 00056 static void event() { 00057 ledBlue = 0; 00058 ledRed = 1; 00059 ledGreen = 1; 00060 } 00061 00062 static void pulseEvent() { 00063 event(); 00064 wait_ms(500); 00065 good(); 00066 } 00067 00068 static void ledOff() { 00069 ledBlue = 1; 00070 ledRed = 1; 00071 ledGreen = 1; 00072 } 00073 00074 static void printCellLocateData(UbloxATCellularInterfaceExt::CellLocData *pData) 00075 { 00076 char timeString[25]; 00077 00078 printf("Cell Locate data:\n"); 00079 if (strftime(timeString, sizeof(timeString), "%F %T", (const tm *) &(pData->time)) > 0) { 00080 printf(" time: %s\n", timeString); 00081 } 00082 printf(" longitude: %.6f\n", pData->longitude); 00083 printf(" latitude: %.6f\n", pData->latitude); 00084 printf(" altitude: %d metre(s)\n", pData->altitude); 00085 switch (pData->sensor) { 00086 case UbloxATCellularInterfaceExt::CELL_LAST: 00087 printf(" sensor type: last\n"); 00088 break; 00089 case UbloxATCellularInterfaceExt::CELL_GNSS: 00090 printf(" sensor type: GNSS\n"); 00091 break; 00092 case UbloxATCellularInterfaceExt::CELL_LOCATE: 00093 printf(" sensor type: Cell Locate\n"); 00094 break; 00095 case UbloxATCellularInterfaceExt::CELL_HYBRID: 00096 printf(" sensor type: hybrid\n"); 00097 break; 00098 default: 00099 printf(" sensor type: unknown\n"); 00100 break; 00101 } 00102 printf(" uncertainty: %d metre(s)\n", pData->uncertainty); 00103 printf(" speed: %d metre(s)/second\n", pData->speed); 00104 printf(" direction: %d degree(s)\n", pData->direction); 00105 printf(" vertical accuracy: %d metre(s)/second\n", pData->speed); 00106 printf(" satellite(s) used: %d\n", pData->svUsed); 00107 printf("I am here: " 00108 "https://maps.google.com/?q=%.5f,%.5f\n", pData->latitude, pData->longitude); 00109 } 00110 00111 static void cbButton() 00112 { 00113 buttonPressed = true; 00114 pulseEvent(); 00115 } 00116 00117 /* This example program for the u-blox C030 and C027 boards instantiates 00118 * the UbloxAtCellularInterfaceExt to do FTP, HTTP and CellLocate operations. 00119 * It uses the site test.rebex.net for FTP testing and the site 00120 * developer.mbed.org for HTTP GET testing. 00121 * Progress may be monitored with a serial terminal running at 9600 baud. 00122 * The LED on the C030 board will turn green when this program is 00123 * operating correctly, pulse blue when an FTP get, HTTP get or CellLocate 00124 * operation is completed and turn red if there is a failure. 00125 */ 00126 00127 int main() 00128 { 00129 UbloxATCellularInterfaceExt *interface = new UbloxATCellularInterfaceExt(); 00130 // If you need to debug the cellular interface, comment out the 00131 // instantiation above and uncomment the one below. 00132 // UbloxATCellularInterfaceExt *interface = new UbloxATCellularInterfaceExt(MDMTXD, MDMRXD, 00133 // MBED_CONF_UBLOX_CELL_BAUD_RATE, 00134 // true); 00135 UbloxATCellularInterfaceExt::Error *err; 00136 UbloxATCellularInterfaceExt::CellLocData data; 00137 char buf[1024]; 00138 int httpProfile; 00139 int numRes; 00140 GnssSerial gnssSerial; // This needed purely to power on the GNSS chip in 00141 // order that Cell Locate on the module can use it 00142 #ifdef TARGET_UBLOX_C027 00143 // No user button on C027 00144 InterruptIn userButton(NC); 00145 #else 00146 InterruptIn userButton(SW0); 00147 #endif 00148 00149 // Attach a function to the user button 00150 userButton.rise(&cbButton); 00151 00152 // Power up GNSS to assist with the Cell Locate bit 00153 gnssSerial.init(); 00154 good(); 00155 printf("Starting up, please wait up to 180 seconds for network registration to complete...\n"); 00156 if (interface->init(PIN)) { 00157 pulseEvent(); 00158 interface->set_credentials(APN, USERNAME, PASSWORD); 00159 printf("Registered, connecting to the packet network...\n"); 00160 for (int x = 0; interface->connect() != 0; x++) { 00161 if (x > 0) { 00162 bad(); 00163 printf("Retrying (have you checked that an antenna is plugged in and your APN is correct?)...\n"); 00164 } 00165 } 00166 pulseEvent(); 00167 00168 // FTP OPERATIONS 00169 // Reset FTP parameters to default then set things up 00170 printf("=== FTP ===\n"); 00171 interface->ftpResetPar(); 00172 interface->ftpSetTimeout(60000); 00173 interface->ftpSetPar(UbloxATCellularInterfaceExt::FTP_SERVER_NAME, "test.rebex.net"); 00174 interface->ftpSetPar(UbloxATCellularInterfaceExt::FTP_USER_NAME, "demo"); 00175 interface->ftpSetPar(UbloxATCellularInterfaceExt::FTP_PASSWORD, "password"); 00176 interface->ftpSetPar(UbloxATCellularInterfaceExt::FTP_MODE, "1"); 00177 00178 // Log into the FTP server 00179 printf("Logging into FTP server \"test.rebex.net\"...\n"); 00180 err = interface->ftpCommand(UbloxATCellularInterfaceExt::FTP_LOGIN); 00181 if (err == NULL) { 00182 pulseEvent(); 00183 // Get a directory listing from the server 00184 if (interface->ftpCommand(UbloxATCellularInterfaceExt::FTP_LS, 00185 NULL, NULL, 0, buf, sizeof (buf)) == NULL) { 00186 pulseEvent(); 00187 printf ("Directory listing of FTP server:\n" 00188 "--------------------------------\n%s" 00189 "--------------------------------\n", buf); 00190 } 00191 // FTP GET a file known to be on test.rebex.net into the module file system 00192 if (interface->ftpCommand(UbloxATCellularInterfaceExt::FTP_GET_FILE, "readme.txt") == NULL) { 00193 pulseEvent(); 00194 // Read the file from the module file system into buf 00195 if (interface->readFile("readme.txt", buf, sizeof (buf))) { 00196 printf("FTP GET of file \"readme.txt\" completed. The file contained:\n" 00197 "------------------------------------------------------------\n%s" 00198 "------------------------------------------------------------\n", buf); 00199 } 00200 } 00201 } else { 00202 bad(); 00203 printf ("Unable to log in, error class %d, error code %d.\n", err->eClass, err->eCode); 00204 } 00205 00206 // HTTP OPERATIONS 00207 // Set up HTTP parameters 00208 printf("=== HTTP ===\n"); 00209 printf("Performing HTTP GET on \"developer.mbed.org\"...\n"); 00210 httpProfile = interface->httpAllocProfile(); 00211 interface->httpSetTimeout(httpProfile, 30000); 00212 interface->httpSetPar(httpProfile, UbloxATCellularInterfaceExt::HTTP_SERVER_NAME, "developer.mbed.org"); 00213 00214 // Do the HTTP command 00215 err = interface->httpCommand(httpProfile, UbloxATCellularInterfaceExt::HTTP_GET, 00216 "/media/uploads/mbed_official/hello.txt", 00217 NULL, NULL, 0, NULL, 00218 buf, sizeof (buf)); 00219 if (err == NULL) { 00220 pulseEvent(); 00221 printf("HTTP GET of \"/media/uploads/mbed_official/hello.txt\" completed. The response contained:\n" 00222 "----------------------------------------------------------------------------------------\n%s" 00223 "----------------------------------------------------------------------------------------\n", buf); 00224 } else { 00225 bad(); 00226 printf("Unable to get \"/media/uploads/mbed_official/hello.txt\" from \"developer.mbed.org\", " 00227 "error class %d, error code %d.\n", err->eClass, err->eCode); 00228 } 00229 00230 // CELL LOCATE OPERATIONS (in a loop) 00231 printf("=== Cell Locate ===\n"); 00232 printf("Sending Cell Locate requests in a loop (until the user button is pressed on C030 or forever on C027)...\n"); 00233 while (!buttonPressed) { 00234 interface->cellLocSrvUdp(); 00235 interface->cellLocConfig(1); // Deep scan mode 00236 printf("Sending Cell Locate request...\n"); 00237 if (interface->cellLocRequest(UbloxATCellularInterfaceExt::CELL_HYBRID, 10, 100, 00238 (UbloxATCellularInterfaceExt::CellRespType) 1, 1)) { 00239 // Wait for the response 00240 numRes = 0; 00241 for (int x = 0; (numRes == 0) && (x < 10); x++) { 00242 numRes = interface->cellLocGetRes(); 00243 } 00244 00245 if (numRes > 0) { 00246 interface->cellLocGetData(&data); 00247 if (data.validData) { 00248 pulseEvent(); 00249 printCellLocateData(&data); 00250 } 00251 } else { 00252 bad(); 00253 printf("No response from Cell Locate server.\n"); 00254 } 00255 } 00256 wait_ms(5000); 00257 #ifndef TARGET_UBLOX_C027 00258 printf("[Checking if user button has been pressed]\n"); 00259 #endif 00260 } 00261 00262 pulseEvent(); 00263 printf("User button was pressed, stopping...\n"); 00264 gnssSerial.powerOff(); 00265 interface->disconnect(); 00266 interface->deinit(); 00267 ledOff(); 00268 printf("Stopped.\n"); 00269 } else { 00270 bad(); 00271 printf("Unable to initialise the interface.\n"); 00272 } 00273 } 00274 00275 // End Of File
Generated on Sun Jul 24 2022 17:02:13 by
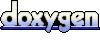