
Example program for the UbloxCellularDriverGen class, providing SMS, USSD and module file system support. This program may be used on the C027 and C030 (non N2xx-flavour) boards.
Dependencies: ublox-cellular-driver-gen ublox-cellular-base
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 #include "UbloxCellularDriverGen.h" 00019 00020 // The credentials of the SIM in the board. If PIN checking is enabled 00021 // for your SIM card you must set this to the required PIN. 00022 #define PIN "0000" 00023 00024 // Network credentials. You should set this according to your 00025 // network/SIM card. For C030 boards, leave the parameters as NULL 00026 // otherwise, if you do not know the APN for your network, you may 00027 // either try the fairly common "internet" for the APN (and leave the 00028 // username and password NULL), or you may leave all three as NULL and then 00029 // a lookup will be attempted for a small number of known networks 00030 // (see APN_db.h in mbed-os/features/netsocket/cellular/utils). 00031 #define APN NULL 00032 #define USERNAME NULL 00033 #define PASSWORD NULL 00034 00035 // If you wish, you may add a mobile phone number here, e.g. 00036 // #define DESTINATION_SMS_NUMBER "+441234567901" 00037 // Note: no spaces are allowed. 00038 // If you do so this program will send a text message to that 00039 // number and then wait for a reply 00040 #define DESTINATION_SMS_NUMBER "" 00041 00042 // LEDs 00043 DigitalOut ledRed(LED1, 1); 00044 DigitalOut ledGreen(LED2, 1); 00045 DigitalOut ledBlue(LED3, 1); 00046 00047 // The user button 00048 volatile bool buttonPressed = false; 00049 00050 static void good() { 00051 ledGreen = 0; 00052 ledBlue = 1; 00053 ledRed = 1; 00054 } 00055 00056 static void bad() { 00057 ledRed = 0; 00058 ledGreen = 1; 00059 ledBlue = 1; 00060 } 00061 00062 static void event() { 00063 ledBlue = 0; 00064 ledRed = 1; 00065 ledGreen = 1; 00066 } 00067 00068 static void pulseEvent() { 00069 event(); 00070 wait_ms(500); 00071 good(); 00072 } 00073 00074 static void ledOff() { 00075 ledBlue = 1; 00076 ledRed = 1; 00077 ledGreen = 1; 00078 } 00079 00080 // See section 12.1 of the u-blox-ATCommands_Manual_(UBX-13002752).pdf 00081 static void printCallForwardingResponse(char * buf) 00082 { 00083 int numValues, a, b; 00084 char number[32]; 00085 00086 numValues = sscanf(buf, "+CCFC: %d,%d,\"%32[^\"][\"]", &a, &b, number); 00087 if (numValues > 0) { 00088 if (a > 0) { 00089 printf("Calling Forwarding is active"); 00090 } else { 00091 printf("Calling Forwarding is not active"); 00092 } 00093 if (numValues > 1) { 00094 if (b > 0) { 00095 if (b & 0x01) { 00096 printf(" for voice"); 00097 } 00098 if (b & 0x02) { 00099 printf(" for data"); 00100 } 00101 if (b & 0x04) { 00102 printf(" for fax"); 00103 } 00104 if (b & 0x08) { 00105 printf(" for SMS"); 00106 } 00107 if (b & 0x10) { 00108 printf(" for data circuit sync"); 00109 } 00110 if (b & 0x20) { 00111 printf(" for data circuit async"); 00112 } 00113 if (b & 0x40) { 00114 printf(" for dedicated packet access"); 00115 } 00116 if (b & 0x80) { 00117 printf(" for dedicated PAD access"); 00118 } 00119 } 00120 } 00121 if (numValues > 2) { 00122 printf(" for %s.\n", number); 00123 } else { 00124 printf(".\n"); 00125 } 00126 } 00127 } 00128 00129 static void cbButton() 00130 { 00131 buttonPressed = true; 00132 pulseEvent(); 00133 } 00134 00135 /* This example program for the u-blox C030 and C027 boards instantiates 00136 * the UbloxCellularDriverGen to do SMS, USSD and module file system operations. 00137 * Progress may be monitored with a serial terminal running at 9600 baud. 00138 * The LED on the C030 board will turn green when this program is 00139 * operating correctly, pulse blue when a USSD action is performed, 00140 * an SMS is sent or received or a file operation is completed, 00141 * and will turn red on a failure condition. 00142 */ 00143 00144 int main() 00145 { 00146 UbloxCellularDriverGen *driver = new UbloxCellularDriverGen(); 00147 // If you need to debug the cellular driver, comment out the 00148 // instantiation above and uncomment the one below. 00149 // UbloxCellularDriverGen *driver = new UbloxCellularDriverGen(MDMTXD, MDMRXD, 00150 // MBED_CONF_UBLOX_CELL_BAUD_RATE, 00151 // true); 00152 char buf[1024]; 00153 int x; 00154 int index[8]; 00155 char number[32]; 00156 #ifdef TARGET_UBLOX_C027 00157 // No user button on C027 00158 InterruptIn userButton(NC); 00159 #else 00160 InterruptIn userButton(SW0); 00161 #endif 00162 00163 // Attach a function to the user button 00164 userButton.rise(&cbButton); 00165 00166 good(); 00167 printf ("Starting up, please wait up to 180 seconds for network registration to complete...\n"); 00168 if (driver->init(PIN)) { 00169 pulseEvent(); 00170 00171 // USSD OPERATIONS 00172 printf("=== USSD ===\n"); 00173 printf("Sending *100#...\n"); 00174 if (driver->ussdCommand("*100#", buf, sizeof (buf))) { 00175 pulseEvent(); 00176 printf("Answer was %s.\n", buf); 00177 } 00178 printf("Getting the status of call forwarding...\n"); 00179 if (driver->ussdCommand("*#21#", buf, sizeof (buf))) { 00180 pulseEvent(); 00181 printf("Answer was \"%s\", which means:\n", buf); 00182 printCallForwardingResponse(buf); 00183 } 00184 00185 // FILE SYSTEM OPERATIONS 00186 printf("=== Module File System ===\n"); 00187 strcpy (buf, "Hello world!"); 00188 x = strlen(buf); 00189 printf("Writing \"%s\" (%d byte(s)) to file \"test.txt\" on the module's file system...\n", buf, x); 00190 x = driver->writeFile("test.txt", buf, strlen(buf)); 00191 printf("%d byte(s) written.\n", x); 00192 if (x == (int) strlen(buf)) { 00193 pulseEvent(); 00194 *buf = 0; 00195 printf("Reading the file back from the module's file system...\n"); 00196 x = driver->readFile("test.txt", buf, sizeof(buf)); 00197 printf("%d byte(s) read.\n", x); 00198 if (x > 0) { 00199 pulseEvent(); 00200 printf("File \"test.txt\" contained \"%s\".\n", buf); 00201 } 00202 printf("Deleting the file...\n"); 00203 if (driver->delFile("test.txt")) { 00204 pulseEvent(); 00205 printf("File deleted.\n"); 00206 } 00207 } 00208 00209 // SMS OPERATIONS 00210 printf("=== SMS ===\n"); 00211 if (strlen(DESTINATION_SMS_NUMBER) > 0) { 00212 printf("Sending a text message to %s...\n", DESTINATION_SMS_NUMBER); 00213 if (driver->smsSend(DESTINATION_SMS_NUMBER, "This is your u-blox mbed board calling.")) { 00214 pulseEvent(); 00215 printf("Text message sent.\n"); 00216 } 00217 } else { 00218 printf("No destination SMS number has been defined (edit DESTINATION_SMS_NUMBER in this file to add one).\n"); 00219 } 00220 00221 printf("Waiting for SMS messages to arrive in a loop (until the user button is pressed on C030 or forever on C027)...\n"); 00222 while (!buttonPressed) { 00223 x = driver->smsList("REC UNREAD", index, sizeof(index)); 00224 for (int y = 0; y < x; y++) { 00225 *number = 0; 00226 if (driver->smsRead(index[y], number, buf, sizeof (buf))) { 00227 pulseEvent(); 00228 printf("Received text message from %s: \"%s\"\n", number, buf); 00229 printf("Replying...\n"); 00230 if (driver->smsSend(number, "mbed board responding: hello there!")) { 00231 pulseEvent(); 00232 printf("Reply sent.\n"); 00233 } 00234 printf("Deleting text message from SIM...\n"); 00235 if (driver->smsDelete(index[y])) { 00236 pulseEvent(); 00237 printf("Message deleted.\n"); 00238 } 00239 } 00240 } 00241 wait_ms(2500); 00242 #ifndef TARGET_UBLOX_C027 00243 printf("[Checking if user button has been pressed]\n"); 00244 #endif 00245 } 00246 00247 pulseEvent(); 00248 printf("User button was pressed, stopping...\n"); 00249 driver->deinit(); 00250 ledOff(); 00251 printf("Stopped.\n"); 00252 } else { 00253 bad(); 00254 printf("Unable to initialise the driver.\n"); 00255 } 00256 } 00257 00258 // End Of File
Generated on Sun Jul 17 2022 05:17:49 by
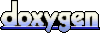