Cellular module AT Serial interface passthrough to Debug COM port. This program can be used on the C030 boards excluding the C030 N2xx version.
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2017 u-blox 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /* The example program for the u-blox C030 boards. It sets up 00018 * - the GNSS module for automatic fix 00019 * - the I2C3 Bus to access bq27441 Battery Charger 00020 * - the bq27441 Battery Charger. 00021 * 00022 */ 00023 00024 #include "mbed.h" 00025 #include "gnss.h" 00026 #include "battery_gauge_bq27441.h" 00027 #include "UbloxCellularDriverGen.h" 00028 #include "onboard_modem_api.h" 00029 00030 // Set the Baudrate for mcu(pc) and modem(dev) 00031 #define BAUDRATE 115200 00032 00033 // Set the minimum input voltage limit for the bq27441 to 3.8 Volt 00034 #define MIN_INPUT_VOLTAGE_LIMIT_MV 3880 00035 00036 00037 // User LEDs 00038 DigitalOut ledRed(LED1, 1); 00039 DigitalOut ledGreen(LED2, 1); 00040 DigitalOut ledBlue(LED3, 1); 00041 00042 // Ethernet socket LED 00043 DigitalOut ledYellow(LED4,1); 00044 00045 // User Button 00046 #ifdef TARGET_UBLOX_C027 00047 // No user button on C027 00048 InterruptIn userButton(NC); 00049 #else 00050 InterruptIn userButton(SW0); 00051 #endif 00052 00053 // GNSS 00054 GnssSerial gnss; 00055 00056 // i2c3 Bus 00057 I2C i2c3(I2C_SDA_B, I2C_SCL_B); 00058 00059 // Battery Charger bq27441 00060 BatteryGaugeBq27441 charger; 00061 00062 RawSerial pc(PD_5, PD_6); 00063 RawSerial dev(PA_9, PA_10); 00064 00065 // Delay between LED changes in second 00066 volatile float delay = 0.5; 00067 00068 // To check if the user pressed the User Button or not 00069 void threadBodyUserButtonCheck(void const *args){ 00070 float delayToggle = delay; 00071 while (1){ 00072 if (userButton.read() == 1 ) { 00073 // User Button is pressed 00074 delay = 0.1; 00075 // Indicate with the Yellow LED on Ethernet socket 00076 ledYellow = 0; 00077 } 00078 else { 00079 // User button is released 00080 delay = 0.5; 00081 // Turn off the Yellow LED on Ethernet socket 00082 ledYellow = 1; 00083 } 00084 } 00085 } 00086 00087 void dev_recv() 00088 { 00089 while(dev.readable()) { 00090 pc.putc(dev.getc()); 00091 } 00092 } 00093 00094 void pc_recv() 00095 { 00096 while(pc.readable()) { 00097 dev.putc(pc.getc()); 00098 } 00099 } 00100 00101 /* 00102 ** Out of the Box Demo for C030 variants 00103 ** 00104 */ 00105 int main() 00106 { 00107 printf("u-blox C030-R410M Bring-up baseline using Out-of-the-Box Demo\n\r"); 00108 00109 // GNSS initialisation 00110 if(gnss.init()) { 00111 printf("GNSS initialized.\n\r"); 00112 } 00113 else { 00114 printf("GNSS initialization failure.\n\r"); 00115 } 00116 00117 // The battery charger initialisation 00118 if(charger.init(&i2c3)) { 00119 printf("Battery charger initialized.\n\r"); 00120 } 00121 else { 00122 printf("Battery charger initialization failure.\n\r"); 00123 } 00124 printf("\n\r"); 00125 00126 // The modem initialisation 00127 onboard_modem_init(); 00128 00129 // Power up the modem 00130 onboard_modem_power_up(); 00131 00132 // Create threadUserButtonCheck thread 00133 Thread threadUserButtonCheck(threadBodyUserButtonCheck); 00134 00135 // Set the LED states 00136 ledRed = 0; 00137 ledGreen = 1; 00138 ledBlue = 1; 00139 00140 pc.baud(BAUDRATE); 00141 dev.baud(BAUDRATE); 00142 00143 pc.attach(&pc_recv, Serial::RxIrq); 00144 dev.attach(&dev_recv, Serial::RxIrq); 00145 00146 // Main loop 00147 while(1) { 00148 wait(delay); 00149 // Shift the LED states 00150 int carry = ledBlue; 00151 ledBlue = ledRed; 00152 ledRed = ledGreen; 00153 ledGreen = carry; 00154 } 00155 } 00156 00157 // End Of File 00158
Generated on Mon Jul 18 2022 05:55:04 by
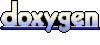