Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #ifndef MBED_EXTENDED_TESTS 00002 #error [NOT_SUPPORTED] Parallel tests are not supported by default 00003 #endif 00004 00005 #include "mbed.h" 00006 #include "ESP8266Interface.h" 00007 #include "TCPSocket.h" 00008 #include "greentea-client/test_env.h" 00009 #include "unity/unity.h" 00010 #include "utest.h" 00011 00012 using namespace utest::v1; 00013 00014 00015 #ifndef MBED_CFG_TCP_CLIENT_ECHO_BUFFER_SIZE 00016 #define MBED_CFG_TCP_CLIENT_ECHO_BUFFER_SIZE 64 00017 #endif 00018 00019 #ifndef MBED_CFG_TCP_CLIENT_ECHO_THREADS 00020 #define MBED_CFG_TCP_CLIENT_ECHO_THREADS 3 00021 #endif 00022 00023 #ifndef MBED_CFG_ESP8266_TX 00024 #define MBED_CFG_ESP8266_TX D1 00025 #endif 00026 00027 #ifndef MBED_CFG_ESP8266_RX 00028 #define MBED_CFG_ESP8266_RX D0 00029 #endif 00030 00031 #ifndef MBED_CFG_ESP8266_DEBUG 00032 #define MBED_CFG_ESP8266_DEBUG false 00033 #endif 00034 00035 #define STRINGIZE(x) STRINGIZE2(x) 00036 #define STRINGIZE2(x) #x 00037 00038 00039 ESP8266Interface net(MBED_CFG_ESP8266_TX, MBED_CFG_ESP8266_RX, MBED_CFG_ESP8266_DEBUG); 00040 SocketAddress tcp_addr; 00041 Mutex iomutex; 00042 00043 void prep_buffer(char *tx_buffer, size_t tx_size) { 00044 for (size_t i=0; i<tx_size; ++i) { 00045 tx_buffer[i] = (rand() % 10) + '0'; 00046 } 00047 } 00048 00049 00050 // Each echo class is in charge of one parallel transaction 00051 class Echo { 00052 private: 00053 char tx_buffer[MBED_CFG_TCP_CLIENT_ECHO_BUFFER_SIZE]; 00054 char rx_buffer[MBED_CFG_TCP_CLIENT_ECHO_BUFFER_SIZE]; 00055 00056 TCPSocket sock; 00057 Thread thread; 00058 00059 public: 00060 // Limiting stack size to 1k 00061 Echo(): thread(osPriorityNormal, 1024) { 00062 } 00063 00064 void start() { 00065 osStatus status = thread.start(callback(this, &Echo::echo)); 00066 TEST_ASSERT_EQUAL(osOK, status); 00067 } 00068 00069 void join() { 00070 osStatus status = thread.join(); 00071 TEST_ASSERT_EQUAL(osOK, status); 00072 } 00073 00074 void echo() { 00075 int err = sock.open(&net); 00076 TEST_ASSERT_EQUAL(0, err); 00077 00078 err = sock.connect(tcp_addr); 00079 TEST_ASSERT_EQUAL(0, err); 00080 00081 iomutex.lock(); 00082 printf("HTTP: Connected to %s:%d\r\n", 00083 tcp_addr.get_ip_address(), tcp_addr.get_port()); 00084 printf("tx_buffer buffer size: %u\r\n", sizeof(tx_buffer)); 00085 printf("rx_buffer buffer size: %u\r\n", sizeof(rx_buffer)); 00086 iomutex.unlock(); 00087 00088 prep_buffer(tx_buffer, sizeof(tx_buffer)); 00089 sock.send(tx_buffer, sizeof(tx_buffer)); 00090 00091 // Server will respond with HTTP GET's success code 00092 const int ret = sock.recv(rx_buffer, sizeof(rx_buffer)); 00093 bool result = !memcmp(tx_buffer, rx_buffer, sizeof(tx_buffer)); 00094 TEST_ASSERT_EQUAL(ret, sizeof(rx_buffer)); 00095 TEST_ASSERT_EQUAL(true, result); 00096 00097 err = sock.close(); 00098 TEST_ASSERT_EQUAL(0, err); 00099 } 00100 }; 00101 00102 Echo *echoers[MBED_CFG_TCP_CLIENT_ECHO_THREADS]; 00103 00104 00105 void test_tcp_echo_parallel() { 00106 int err = net.connect(STRINGIZE(MBED_CFG_ESP8266_SSID), STRINGIZE(MBED_CFG_ESP8266_PASS)); 00107 TEST_ASSERT_EQUAL(0, err); 00108 00109 printf("MBED: TCPClient IP address is '%s'\n", net.get_ip_address()); 00110 printf("MBED: TCPClient waiting for server IP and port...\n"); 00111 00112 greentea_send_kv("target_ip", net.get_ip_address()); 00113 00114 char recv_key[] = "host_port"; 00115 char ipbuf[60] = {0}; 00116 char portbuf[16] = {0}; 00117 unsigned int port = 0; 00118 00119 greentea_send_kv("host_ip", " "); 00120 greentea_parse_kv(recv_key, ipbuf, sizeof(recv_key), sizeof(ipbuf)); 00121 00122 greentea_send_kv("host_port", " "); 00123 greentea_parse_kv(recv_key, portbuf, sizeof(recv_key), sizeof(ipbuf)); 00124 sscanf(portbuf, "%u", &port); 00125 00126 printf("MBED: Server IP address received: %s:%d \n", ipbuf, port); 00127 tcp_addr.set_ip_address(ipbuf); 00128 tcp_addr.set_port(port); 00129 00130 // Startup echo threads in parallel 00131 for (int i = 0; i < MBED_CFG_TCP_CLIENT_ECHO_THREADS; i++) { 00132 echoers[i] = new Echo; 00133 echoers[i]->start(); 00134 } 00135 00136 for (int i = 0; i < MBED_CFG_TCP_CLIENT_ECHO_THREADS; i++) { 00137 echoers[i]->join(); 00138 delete echoers[i]; 00139 } 00140 00141 net.disconnect(); 00142 } 00143 00144 // Test setup 00145 utest::v1::status_t test_setup(const size_t number_of_cases) { 00146 GREENTEA_SETUP(120, "tcp_echo"); 00147 return verbose_test_setup_handler(number_of_cases); 00148 } 00149 00150 Case cases[] = { 00151 Case("TCP echo parallel", test_tcp_echo_parallel), 00152 }; 00153 00154 Specification specification(test_setup, cases); 00155 00156 int main() { 00157 return !Harness::run(specification); 00158 } 00159
Generated on Tue Jul 12 2022 15:14:29 by
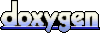