Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
easy-connect.h
00001 #ifndef __EASY_CONNECT_H__ 00002 #define __EASY_CONNECT_H__ 00003 00004 #include "mbed.h" 00005 00006 #define ETHERNET 1 00007 #define WIFI_ESP8266 2 00008 #define MESH_LOWPAN_ND 3 00009 #define MESH_THREAD 4 00010 #define WIFI_ODIN 5 00011 #define WIFI_REALTEK 6 00012 #define CELLULAR_UBLOX 7 00013 00014 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00015 #include "ESP8266Interface.h" 00016 00017 #ifdef MBED_CONF_APP_ESP8266_DEBUG 00018 ESP8266Interface wifi(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX, MBED_CONF_APP_ESP8266_DEBUG); 00019 #else 00020 ESP8266Interface wifi(MBED_CONF_APP_ESP8266_TX, MBED_CONF_APP_ESP8266_RX); 00021 #endif 00022 00023 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ODIN 00024 #include "OdinWiFiInterface.h" 00025 00026 OdinWiFiInterface wifi; 00027 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_RTW 00028 #include "RTWInterface.h" 00029 RTWInterface wifi; 00030 00031 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_UBLOX 00032 // Note: we use UbloxAtCellularInterfaceExt here but the 00033 // examples work with UbloxATCellularInterface, 00034 // UbloxPPPCellularInterface or UbloxATCellularInterfaceN2xx 00035 // (though noting that the N211 module supports only UDP and not TCP); 00036 // simply uncomment/comment the correct options in the next few lines 00037 #include "UbloxATCellularInterfaceExt.h" 00038 //#include "UbloxPPPCellularInterface.h" 00039 //#include "UbloxATCellularInterface.h" 00040 //#include "UbloxATCellularInterfaceN2xx.h" 00041 00042 // Parameters added to the call here simply to show how debug can be switched on 00043 // For the N2xx interface, change xxx to MBED_CONF_UBLOX_CELL_N2XX_BAUD_RATE. 00044 UbloxATCellularInterfaceExt cellular(MDMTXD, MDMRXD, MBED_CONF_UBLOX_CELL_BAUD_RATE, true); 00045 //UbloxPPPCellularInterface cellular; 00046 //UbloxATCellularInterface cellular; 00047 //UbloxATCellularInterfaceN2xx cellular; 00048 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00049 #include "EthernetInterface.h" 00050 EthernetInterface eth; 00051 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_LOWPAN_ND 00052 #define MESH 00053 #include "NanostackInterface.h" 00054 LoWPANNDInterface mesh; 00055 #elif MBED_CONF_APP_NETWORK_INTERFACE == MESH_THREAD 00056 #define MESH 00057 #include "NanostackInterface.h" 00058 ThreadInterface mesh; 00059 #else 00060 #error "No connectivity method chosen. Please add 'config.network-interfaces.value' to your mbed_app.json (see README.md for more information)." 00061 #endif 00062 00063 #if defined(MESH) 00064 #if MBED_CONF_APP_MESH_RADIO_TYPE == ATMEL 00065 #include "NanostackRfPhyAtmel.h" 00066 NanostackRfPhyAtmel rf_phy(ATMEL_SPI_MOSI, ATMEL_SPI_MISO, ATMEL_SPI_SCLK, ATMEL_SPI_CS, 00067 ATMEL_SPI_RST, ATMEL_SPI_SLP, ATMEL_SPI_IRQ, ATMEL_I2C_SDA, ATMEL_I2C_SCL); 00068 #elif MBED_CONF_APP_MESH_RADIO_TYPE == MCR20 00069 #include "NanostackRfPhyMcr20a.h" 00070 NanostackRfPhyMcr20a rf_phy(MCR20A_SPI_MOSI, MCR20A_SPI_MISO, MCR20A_SPI_SCLK, MCR20A_SPI_CS, MCR20A_SPI_RST, MCR20A_SPI_IRQ); 00071 #elif MBED_CONF_APP_MESH_RADIO_TYPE == SPIRIT1 00072 #include "NanostackRfPhySpirit1.h" 00073 NanostackRfPhySpirit1 rf_phy(SPIRIT1_SPI_MOSI, SPIRIT1_SPI_MISO, SPIRIT1_SPI_SCLK, 00074 SPIRIT1_DEV_IRQ, SPIRIT1_DEV_CS, SPIRIT1_DEV_SDN, SPIRIT1_BRD_LED); 00075 #elif MBED_CONF_APP_MESH_RADIO_TYPE == EFR32 00076 #include "NanostackRfPhyEfr32.h" 00077 NanostackRfPhyEfr32 rf_phy; 00078 #endif //MBED_CONF_APP_RADIO_TYPE 00079 #endif //MESH 00080 00081 #ifndef MESH 00082 // This is address to mbed Device Connector 00083 #define MBED_SERVER_ADDRESS "coap://api.connector.mbed.com:5684" 00084 #else 00085 // This is address to mbed Device Connector 00086 #define MBED_SERVER_ADDRESS "coaps://[2607:f0d0:2601:52::20]:5684" 00087 #endif 00088 00089 #ifdef MBED_CONF_APP_ESP8266_SSID 00090 #define MBED_CONF_APP_WIFI_SSID MBED_CONF_APP_ESP8266_SSID 00091 #endif 00092 00093 #ifdef MBED_CONF_APP_ESP8266_PASSWORD 00094 #define MBED_CONF_APP_WIFI_PASSWORD MBED_CONF_APP_ESP8266_PASSWORD 00095 #endif 00096 00097 /* \brief print_MAC - print_MAC - helper function to print out MAC address 00098 * in: network_interface - pointer to network i/f 00099 * bool log-messages print out logs or not 00100 * MAC address is print, if it can be acquired & log_messages is true. 00101 * 00102 */ 00103 void print_MAC(NetworkInterface* network_interface, bool log_messages) { 00104 #if MBED_CONF_APP_NETWORK_INTERFACE != CELLULAR_UBLOX 00105 const char *mac_addr = network_interface->get_mac_address(); 00106 if (mac_addr == NULL) { 00107 if (log_messages) { 00108 printf("[EasyConnect] ERROR - No MAC address\n"); 00109 } 00110 return; 00111 } 00112 if (log_messages) { 00113 printf("[EasyConnect] MAC address %s\n", mac_addr); 00114 } 00115 #endif 00116 } 00117 00118 00119 /* \brief easy_connect - easy_connect function to connect the pre-defined network bearer, 00120 * config done via mbed_app.json (see README.md for details). 00121 * IN: bool log_messages print out diagnostics or not. 00122 * 00123 */ 00124 NetworkInterface* easy_connect(bool log_messages = false) { 00125 NetworkInterface* network_interface = 0; 00126 int connect_success = -1; 00127 /// This should be removed once mbedOS supports proper dual-stack 00128 #if defined (MESH) || (MBED_CONF_LWIP_IPV6_ENABLED==true) 00129 printf("[EasyConnect] IPv6 mode\n"); 00130 #else 00131 printf("[EasyConnect] IPv4 mode\n"); 00132 #endif 00133 00134 #if MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ESP8266 00135 if (log_messages) { 00136 printf("[EasyConnect] Using WiFi (ESP8266) \n"); 00137 printf("[EasyConnect] Connecting to WiFi %s\n", MBED_CONF_APP_WIFI_SSID); 00138 } 00139 network_interface = &wifi; 00140 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00141 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_ODIN 00142 if (log_messages) { 00143 printf("[EasyConnect] Using WiFi (ODIN) \n"); 00144 printf("[EasyConnect] Connecting to WiFi %s\n", MBED_CONF_APP_WIFI_SSID); 00145 } 00146 network_interface = &wifi; 00147 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00148 #elif MBED_CONF_APP_NETWORK_INTERFACE == WIFI_RTW 00149 if (log_messages) { 00150 printf("[EasyConnect] Using WiFi (RTW)\n"); 00151 printf("[EasyConnect] Connecting to WiFi %s\n", MBED_CONF_APP_WIFI_SSID); 00152 } 00153 network_interface = &wifi; 00154 connect_success = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00155 #elif MBED_CONF_APP_NETWORK_INTERFACE == CELLULAR_UBLOX 00156 if (log_messages) { 00157 printf("[EasyConnect] Using Cellular (u-blox) \n"); 00158 if (strlen(MBED_CONF_APP_APN) > 0) { 00159 printf("[EasyConnect] Connecting to Cellular %s\n", MBED_CONF_APP_APN); 00160 } else { 00161 printf("[EasyConnect] Connecting to Cellular\n"); 00162 } 00163 } 00164 network_interface = &cellular; 00165 connect_success = cellular.connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD); 00166 #elif MBED_CONF_APP_NETWORK_INTERFACE == ETHERNET 00167 if (log_messages) { 00168 printf("[EasyConnect] Using Ethernet\n"); 00169 } 00170 network_interface = ð 00171 connect_success = eth.connect(); 00172 #endif 00173 00174 #ifdef MESH 00175 if (log_messages) { 00176 printf("[EasyConnect] Using Mesh\n"); 00177 printf("[EasyConnect] Connecting to Mesh..\n"); 00178 } 00179 network_interface = &mesh; 00180 mesh.initialize(&rf_phy); 00181 connect_success = mesh.connect(); 00182 #endif 00183 if(connect_success == 0) { 00184 if (log_messages) { 00185 printf("[EasyConnect] Connected to Network successfully\n"); 00186 print_MAC(network_interface, log_messages); 00187 } 00188 } else { 00189 if (log_messages) { 00190 print_MAC(network_interface, log_messages); 00191 printf("[EasyConnect] Connection to Network Failed %d!\n", connect_success); 00192 } 00193 return NULL; 00194 } 00195 const char *ip_addr = network_interface->get_ip_address(); 00196 if (ip_addr == NULL) { 00197 if (log_messages) { 00198 printf("[EasyConnect] ERROR - No IP address\n"); 00199 } 00200 return NULL; 00201 } 00202 if (log_messages) { 00203 printf("[EasyConnect] IP address %s\n", ip_addr); 00204 } 00205 return network_interface; 00206 } 00207 00208 #endif // __EASY_CONNECT_H__
Generated on Tue Jul 12 2022 15:14:29 by
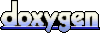