Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
at24mac.h
00001 /* 00002 * Copyright (c) 2014-2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef AT24MAC_H 00017 #define AT24MAC_H 00018 00019 #include "PinNames.h" 00020 #include "I2C.h" 00021 #include "drivers/DigitalInOut.h" 00022 #include "platform/mbed_wait_api.h" 00023 00024 /* 00025 * AT24MAC drivers. 00026 * 00027 * This is a EEPROM chip designed to contain factory programmed read-only EUI-64 or EUI-48, 00028 * a 128bit serial number and some user programmable EEPROM. 00029 * 00030 * AT24MAC602 contains EUI-64, use read_eui64() 00031 * AT24MAC402 contains EUI-64, use read_eui48() 00032 * 00033 * NOTE: You cannot use both EUI-64 and EUI-48. Chip contains only one of those. 00034 */ 00035 00036 class AT24Mac { 00037 public: 00038 AT24Mac(PinName sda, PinName scl); 00039 00040 /** 00041 * Read unique serial number from chip. 00042 * \param buf pointer to write serial number to. Must have space for 16 bytes. 00043 * \return zero on success, negative number on failure 00044 */ 00045 int read_serial(void *buf); 00046 00047 /** 00048 * Read EUI-64 from chip. 00049 * \param buf pointer to write EUI-64 to. Must have space for 8 bytes. 00050 * \return zero on success, negative number on failure 00051 */ 00052 int read_eui64(void *buf); 00053 00054 /** 00055 * Read EUI-48 from chip. 00056 * \param buf pointer to write EUI-48 to. Must have space for 6 bytes. 00057 * \return zero on success, negative number on failure 00058 */ 00059 int read_eui48(void *buf); 00060 00061 private: 00062 /* 00063 * Dummy class to allow us to reset I2C before the I2C constructor is called in 00064 * the initializer list of AT24Mac's constructor 00065 */ 00066 class I2CReset { 00067 public: 00068 I2CReset(PinName sda, PinName scl); 00069 }; 00070 I2CReset i2c_reset; 00071 mbed::I2C _i2c; 00072 }; 00073 00074 #endif /* AT24MAC_H */
Generated on Tue Jul 12 2022 15:14:29 by
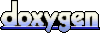