Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
SPIRIT_DirectRF.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file SPIRIT_DirectRF.c 00004 * @author VMA division - AMS 00005 * @version 3.2.2 00006 * @date 08-July-2015 00007 * @brief Configuration and management of SPIRIT direct transmission / receive modes. 00008 * @details 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "SPIRIT_DirectRF.h" 00040 #include "MCU_Interface.h" 00041 00042 00043 00044 /** 00045 * @addtogroup SPIRIT_Libraries 00046 * @{ 00047 */ 00048 00049 00050 /** 00051 * @addtogroup SPIRIT_DirectRf 00052 * @{ 00053 */ 00054 00055 00056 /** 00057 * @defgroup DirectRf_Private_TypesDefinitions Direct RF Private Types Definitions 00058 * @{ 00059 */ 00060 00061 /** 00062 *@} 00063 */ 00064 00065 00066 /** 00067 * @defgroup DirectRf_Private_Defines Direct RF Private Defines 00068 * @{ 00069 */ 00070 00071 /** 00072 *@} 00073 */ 00074 00075 00076 /** 00077 * @defgroup DirectRf_Private_Macros Direct RF Private Macros 00078 * @{ 00079 */ 00080 00081 /** 00082 *@} 00083 */ 00084 00085 00086 /** 00087 * @defgroup DirectRf_Private_Variables Direct RF Private Variables 00088 * @{ 00089 */ 00090 00091 /** 00092 *@} 00093 */ 00094 00095 00096 00097 /** 00098 * @defgroup DirectRf_Private_FunctionPrototypes Direct RF Private Function Prototypes 00099 * @{ 00100 */ 00101 00102 /** 00103 *@} 00104 */ 00105 00106 00107 /** 00108 * @defgroup DirectRf_Private_Functions Direct RF Private Functions 00109 * @{ 00110 */ 00111 00112 /** 00113 * @brief Sets the DirectRF RX mode of SPIRIT. 00114 * @param xDirectRx code of the desired mode. 00115 * This parameter can be any value of @ref DirectRx. 00116 * @retval None. 00117 */ 00118 void SpiritDirectRfSetRxMode(DirectRx xDirectRx) 00119 { 00120 uint8_t tempRegValue; 00121 00122 /* Check the parameters */ 00123 s_assert_param(IS_DIRECT_RX(xDirectRx)); 00124 00125 /* Reads the register value */ 00126 SpiritSpiReadRegisters(PCKTCTRL3_BASE, 1, &tempRegValue); 00127 00128 /* Build the value to be stored */ 00129 tempRegValue &= ~PCKTCTRL3_RX_MODE_MASK; 00130 tempRegValue |= (uint8_t)xDirectRx; 00131 00132 /* Writes value on register */ 00133 g_xStatus = SpiritSpiWriteRegisters(PCKTCTRL3_BASE, 1, &tempRegValue); 00134 00135 } 00136 00137 00138 /** 00139 * @brief Returns the DirectRF RX mode of SPIRIT. 00140 * @param None. 00141 * @retval DirectRx Direct Rx mode. 00142 */ 00143 DirectRx SpiritDirectRfGetRxMode(void) 00144 { 00145 uint8_t tempRegValue; 00146 00147 /* Reads the register value and mask the RX_Mode field */ 00148 g_xStatus = SpiritSpiReadRegisters(PCKTCTRL3_BASE, 1, &tempRegValue); 00149 00150 /* Rebuild and return value */ 00151 return (DirectRx)(tempRegValue & 0x30); 00152 00153 } 00154 00155 00156 /** 00157 * @brief Sets the TX mode of SPIRIT. 00158 * @param xDirectTx code of the desired source. 00159 * This parameter can be any value of @ref DirectTx. 00160 * @retval None. 00161 */ 00162 void SpiritDirectRfSetTxMode(DirectTx xDirectTx) 00163 { 00164 uint8_t tempRegValue; 00165 00166 /* Check the parameters */ 00167 s_assert_param(IS_DIRECT_TX(xDirectTx)); 00168 00169 /* Reads the register value */ 00170 SpiritSpiReadRegisters(PCKTCTRL1_BASE, 1, &tempRegValue); 00171 00172 /* Build the value to be stored */ 00173 tempRegValue &= ~PCKTCTRL1_TX_SOURCE_MASK; 00174 tempRegValue |= (uint8_t)xDirectTx; 00175 00176 /* Writes value on register */ 00177 g_xStatus = SpiritSpiWriteRegisters(PCKTCTRL1_BASE, 1, &tempRegValue); 00178 00179 } 00180 00181 00182 /** 00183 * @brief Returns the DirectRF TX mode of SPIRIT. 00184 * @param None. 00185 * @retval DirectTx Direct Tx mode. 00186 */ 00187 DirectTx SpiritDirectRfGetTxMode(void) 00188 { 00189 uint8_t tempRegValue; 00190 00191 /* Reads the register value and mask the RX_Mode field */ 00192 g_xStatus = SpiritSpiReadRegisters(PCKTCTRL1_BASE, 1, &tempRegValue); 00193 00194 /* Returns value */ 00195 return (DirectTx)(tempRegValue & 0x0C); 00196 00197 } 00198 00199 00200 /** 00201 *@} 00202 */ 00203 00204 /** 00205 *@} 00206 */ 00207 00208 00209 /** 00210 *@} 00211 */ 00212 00213 00214 00215 /******************* (C) COPYRIGHT 2015 STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 15:14:30 by
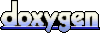