Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
MCR20Drv.h
Go to the documentation of this file.
00001 /*! 00002 * Copyright (c) 2015, Freescale Semiconductor, Inc. 00003 * All rights reserved. 00004 * 00005 * \file MCR20Drv.h 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * o Redistributions of source code must retain the above copyright notice, this list 00011 * of conditions and the following disclaimer. 00012 * 00013 * o Redistributions in binary form must reproduce the above copyright notice, this 00014 * list of conditions and the following disclaimer in the documentation and/or 00015 * other materials provided with the distribution. 00016 * 00017 * o Neither the name of Freescale Semiconductor, Inc. nor the names of its 00018 * contributors may be used to endorse or promote products derived from this 00019 * software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00022 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00023 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00025 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00026 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00027 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00028 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00029 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 */ 00032 00033 #ifndef __MCR20_DRV_H__ 00034 #define __MCR20_DRV_H__ 00035 00036 00037 /***************************************************************************** 00038 * INCLUDED HEADERS * 00039 *---------------------------------------------------------------------------* 00040 * Add to this section all the headers that this module needs to include. * 00041 * Note that it is not a good practice to include header files into header * 00042 * files, so use this section only if there is no other better solution. * 00043 *---------------------------------------------------------------------------* 00044 *****************************************************************************/ 00045 00046 /***************************************************************************** 00047 * PRIVATE MACROS * 00048 *---------------------------------------------------------------------------* 00049 * Add to this section all the access macros, registers mappings, bit access * 00050 * macros, masks, flags etc ... 00051 *---------------------------------------------------------------------------* 00052 *****************************************************************************/ 00053 00054 /* Disable XCVR clock output by default, to reduce power consumption */ 00055 #ifndef gMCR20_ClkOutFreq_d 00056 #define gMCR20_ClkOutFreq_d gCLK_OUT_FREQ_DISABLE 00057 #endif 00058 00059 /***************************************************************************** 00060 * PUBLIC FUNCTIONS * 00061 *---------------------------------------------------------------------------* 00062 * Add to this section all the global functions prototype preceded (as a * 00063 * good practice) by the keyword 'extern' * 00064 *---------------------------------------------------------------------------* 00065 *****************************************************************************/ 00066 00067 /*--------------------------------------------------------------------------- 00068 * Name: MCR20Drv_Init 00069 * Description: - 00070 * Parameters: - 00071 * Return: - 00072 *---------------------------------------------------------------------------*/ 00073 extern void MCR20Drv_Init 00074 ( 00075 void 00076 ); 00077 00078 /*--------------------------------------------------------------------------- 00079 * Name: MCR20Drv_SPI_DMA_Init 00080 * Description: - 00081 * Parameters: - 00082 * Return: - 00083 *---------------------------------------------------------------------------*/ 00084 void MCR20Drv_SPI_DMA_Init 00085 ( 00086 void 00087 ); 00088 00089 /*--------------------------------------------------------------------------- 00090 * Name: MCR20Drv_Start_PB_DMA_SPI_Write 00091 * Description: - 00092 * Parameters: - 00093 * Return: - 00094 *---------------------------------------------------------------------------*/ 00095 void MCR20Drv_Start_PB_DMA_SPI_Write 00096 ( 00097 uint8_t * srcAddress, 00098 uint8_t numOfBytes 00099 ); 00100 00101 /*--------------------------------------------------------------------------- 00102 * Name: MCR20Drv_Start_PB_DMA_SPI_Read 00103 * Description: - 00104 * Parameters: - 00105 * Return: - 00106 *---------------------------------------------------------------------------*/ 00107 void MCR20Drv_Start_PB_DMA_SPI_Read 00108 ( 00109 uint8_t * dstAddress, 00110 uint8_t numOfBytes 00111 ); 00112 00113 /*--------------------------------------------------------------------------- 00114 * Name: MCR20Drv_DirectAccessSPIWrite 00115 * Description: - 00116 * Parameters: - 00117 * Return: - 00118 *---------------------------------------------------------------------------*/ 00119 void MCR20Drv_DirectAccessSPIWrite 00120 ( 00121 uint8_t address, 00122 uint8_t value 00123 ); 00124 00125 /*--------------------------------------------------------------------------- 00126 * Name: MCR20Drv_DirectAccessSPIMultiByteWrite 00127 * Description: - 00128 * Parameters: - 00129 * Return: - 00130 *---------------------------------------------------------------------------*/ 00131 void MCR20Drv_DirectAccessSPIMultiByteWrite 00132 ( 00133 uint8_t startAddress, 00134 uint8_t * byteArray, 00135 uint8_t numOfBytes 00136 ); 00137 00138 /*--------------------------------------------------------------------------- 00139 * Name: MCR20Drv_PB_SPIBurstWrite 00140 * Description: - 00141 * Parameters: - 00142 * Return: - 00143 *---------------------------------------------------------------------------*/ 00144 void MCR20Drv_PB_SPIBurstWrite 00145 ( 00146 uint8_t * byteArray, 00147 uint8_t numOfBytes 00148 ); 00149 00150 /*--------------------------------------------------------------------------- 00151 * Name: MCR20Drv_DirectAccessSPIRead 00152 * Description: - 00153 * Parameters: - 00154 * Return: - 00155 *---------------------------------------------------------------------------*/ 00156 uint8_t MCR20Drv_DirectAccessSPIRead 00157 ( 00158 uint8_t address 00159 ); 00160 00161 /*--------------------------------------------------------------------------- 00162 * Name: MCR20Drv_DirectAccessSPIMultyByteRead 00163 * Description: - 00164 * Parameters: - 00165 * Return: - 00166 *---------------------------------------------------------------------------*/ 00167 00168 uint8_t MCR20Drv_DirectAccessSPIMultiByteRead 00169 ( 00170 uint8_t startAddress, 00171 uint8_t * byteArray, 00172 uint8_t numOfBytes 00173 ); 00174 00175 /*--------------------------------------------------------------------------- 00176 * Name: MCR20Drv_PB_SPIByteWrite 00177 * Description: - 00178 * Parameters: - 00179 * Return: - 00180 *---------------------------------------------------------------------------*/ 00181 void MCR20Drv_PB_SPIByteWrite 00182 ( 00183 uint8_t address, 00184 uint8_t value 00185 ); 00186 00187 /*--------------------------------------------------------------------------- 00188 * Name: MCR20Drv_PB_SPIBurstRead 00189 * Description: - 00190 * Parameters: - 00191 * Return: - 00192 *---------------------------------------------------------------------------*/ 00193 uint8_t MCR20Drv_PB_SPIBurstRead 00194 ( 00195 uint8_t * byteArray, 00196 uint8_t numOfBytes 00197 ); 00198 00199 /*--------------------------------------------------------------------------- 00200 * Name: MCR20Drv_IndirectAccessSPIWrite 00201 * Description: - 00202 * Parameters: - 00203 * Return: - 00204 *---------------------------------------------------------------------------*/ 00205 void MCR20Drv_IndirectAccessSPIWrite 00206 ( 00207 uint8_t address, 00208 uint8_t value 00209 ); 00210 00211 /*--------------------------------------------------------------------------- 00212 * Name: MCR20Drv_IndirectAccessSPIMultiByteWrite 00213 * Description: - 00214 * Parameters: - 00215 * Return: - 00216 *---------------------------------------------------------------------------*/ 00217 void MCR20Drv_IndirectAccessSPIMultiByteWrite 00218 ( 00219 uint8_t startAddress, 00220 uint8_t * byteArray, 00221 uint8_t numOfBytes 00222 ); 00223 00224 /*--------------------------------------------------------------------------- 00225 * Name: MCR20Drv_IndirectAccessSPIRead 00226 * Description: - 00227 * Parameters: - 00228 * Return: - 00229 *---------------------------------------------------------------------------*/ 00230 uint8_t MCR20Drv_IndirectAccessSPIRead 00231 ( 00232 uint8_t address 00233 ); 00234 /*--------------------------------------------------------------------------- 00235 * Name: MCR20Drv_IndirectAccessSPIMultiByteRead 00236 * Description: - 00237 * Parameters: - 00238 * Return: - 00239 *---------------------------------------------------------------------------*/ 00240 void MCR20Drv_IndirectAccessSPIMultiByteRead 00241 ( 00242 uint8_t startAddress, 00243 uint8_t * byteArray, 00244 uint8_t numOfBytes 00245 ); 00246 00247 /*--------------------------------------------------------------------------- 00248 * Name: MCR20Drv_IsIrqPending 00249 * Description: - 00250 * Parameters: - 00251 * Return: - 00252 *---------------------------------------------------------------------------*/ 00253 uint32_t MCR20Drv_IsIrqPending 00254 ( 00255 void 00256 ); 00257 00258 /*--------------------------------------------------------------------------- 00259 * Name: MCR20Drv_IRQ_Disable 00260 * Description: - 00261 * Parameters: - 00262 * Return: - 00263 *---------------------------------------------------------------------------*/ 00264 void MCR20Drv_IRQ_Disable 00265 ( 00266 void 00267 ); 00268 00269 /*--------------------------------------------------------------------------- 00270 * Name: MCR20Drv_IRQ_Enable 00271 * Description: - 00272 * Parameters: - 00273 * Return: - 00274 *---------------------------------------------------------------------------*/ 00275 void MCR20Drv_IRQ_Enable 00276 ( 00277 void 00278 ); 00279 00280 /*--------------------------------------------------------------------------- 00281 * Name: MCR20Drv_RST_PortConfig 00282 * Description: - 00283 * Parameters: - 00284 * Return: - 00285 *---------------------------------------------------------------------------*/ 00286 void MCR20Drv_RST_B_PortConfig 00287 ( 00288 void 00289 ); 00290 00291 /*--------------------------------------------------------------------------- 00292 * Name: MCR20Drv_RST_Assert 00293 * Description: - 00294 * Parameters: - 00295 * Return: - 00296 *---------------------------------------------------------------------------*/ 00297 void MCR20Drv_RST_B_Assert 00298 ( 00299 void 00300 ); 00301 00302 /*--------------------------------------------------------------------------- 00303 * Name: MCR20Drv_RST_Deassert 00304 * Description: - 00305 * Parameters: - 00306 * Return: - 00307 *---------------------------------------------------------------------------*/ 00308 void MCR20Drv_RST_B_Deassert 00309 ( 00310 void 00311 ); 00312 00313 /*--------------------------------------------------------------------------- 00314 * Name: MCR20Drv_SoftRST_Assert 00315 * Description: - 00316 * Parameters: - 00317 * Return: - 00318 *---------------------------------------------------------------------------*/ 00319 void MCR20Drv_SoftRST_Assert 00320 ( 00321 void 00322 ); 00323 00324 /*--------------------------------------------------------------------------- 00325 * Name: MCR20Drv_SoftRST_Deassert 00326 * Description: - 00327 * Parameters: - 00328 * Return: - 00329 *---------------------------------------------------------------------------*/ 00330 void MCR20Drv_SoftRST_Deassert 00331 ( 00332 void 00333 ); 00334 00335 00336 /*--------------------------------------------------------------------------- 00337 * Name: MCR20Drv_RESET 00338 * Description: - 00339 * Parameters: - 00340 * Return: - 00341 *---------------------------------------------------------------------------*/ 00342 void MCR20Drv_RESET 00343 ( 00344 void 00345 ); 00346 00347 /*--------------------------------------------------------------------------- 00348 * Name: MCR20Drv_Soft_RESET 00349 * Description: - 00350 * Parameters: - 00351 * Return: - 00352 *---------------------------------------------------------------------------*/ 00353 void MCR20Drv_Soft_RESET 00354 ( 00355 void 00356 ); 00357 00358 /*--------------------------------------------------------------------------- 00359 * Name: MCR20Drv_Set_CLK_OUT_Freq 00360 * Description: - 00361 * Parameters: - 00362 * Return: - 00363 *---------------------------------------------------------------------------*/ 00364 void MCR20Drv_Set_CLK_OUT_Freq 00365 ( 00366 uint8_t freqDiv 00367 ); 00368 00369 #define ProtectFromMCR20Interrupt() MCR20Drv_IRQ_Disable() 00370 #define UnprotectFromMCR20Interrupt() MCR20Drv_IRQ_Enable() 00371 00372 #endif /* __MCR20_DRV_H__ */
Generated on Tue Jul 12 2022 15:14:29 by
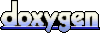