Version of easy-connect with the u-blox cellular platforms C027 and C030 added.
Embed:
(wiki syntax)
Show/hide line numbers
MCR20Drv.c
Go to the documentation of this file.
00001 /*! 00002 * Copyright (c) 2015, Freescale Semiconductor, Inc. 00003 * All rights reserved. 00004 * 00005 * \file MCR20Drv.c 00006 * 00007 * Redistribution and use in source and binary forms, with or without modification, 00008 * are permitted provided that the following conditions are met: 00009 * 00010 * o Redistributions of source code must retain the above copyright notice, this list 00011 * of conditions and the following disclaimer. 00012 * 00013 * o Redistributions in binary form must reproduce the above copyright notice, this 00014 * list of conditions and the following disclaimer in the documentation and/or 00015 * other materials provided with the distribution. 00016 * 00017 * o Neither the name of Freescale Semiconductor, Inc. nor the names of its 00018 * contributors may be used to endorse or promote products derived from this 00019 * software without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00022 * ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00023 * WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR 00025 * ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES 00026 * (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00027 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON 00028 * ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00029 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 */ 00032 00033 00034 /***************************************************************************** 00035 * INCLUDED HEADERS * 00036 *---------------------------------------------------------------------------* 00037 * Add to this section all the headers that this module needs to include. * 00038 *---------------------------------------------------------------------------* 00039 *****************************************************************************/ 00040 00041 #include "platform/arm_hal_interrupt.h" 00042 #include "MCR20Drv.h " 00043 #include "MCR20Reg.h" 00044 #include "XcvrSpi.h " 00045 00046 00047 /***************************************************************************** 00048 * PRIVATE VARIABLES * 00049 *---------------------------------------------------------------------------* 00050 * Add to this section all the variables and constants that have local * 00051 * (file) scope. * 00052 * Each of this declarations shall be preceded by the 'static' keyword. * 00053 * These variables / constants cannot be accessed outside this module. * 00054 *---------------------------------------------------------------------------* 00055 *****************************************************************************/ 00056 uint32_t mPhyIrqDisableCnt = 1; 00057 00058 /***************************************************************************** 00059 * PUBLIC VARIABLES * 00060 *---------------------------------------------------------------------------* 00061 * Add to this section all the variables and constants that have global * 00062 * (project) scope. * 00063 * These variables / constants can be accessed outside this module. * 00064 * These variables / constants shall be preceded by the 'extern' keyword in * 00065 * the interface header. * 00066 *---------------------------------------------------------------------------* 00067 *****************************************************************************/ 00068 00069 /***************************************************************************** 00070 * PRIVATE FUNCTIONS PROTOTYPES * 00071 *---------------------------------------------------------------------------* 00072 * Add to this section all the functions prototypes that have local (file) * 00073 * scope. * 00074 * These functions cannot be accessed outside this module. * 00075 * These declarations shall be preceded by the 'static' keyword. * 00076 *---------------------------------------------------------------------------* 00077 *****************************************************************************/ 00078 00079 /***************************************************************************** 00080 * PRIVATE FUNCTIONS * 00081 *---------------------------------------------------------------------------* 00082 * Add to this section all the functions that have local (file) scope. * 00083 * These functions cannot be accessed outside this module. * 00084 * These definitions shall be preceded by the 'static' keyword. * 00085 *---------------------------------------------------------------------------* 00086 *****************************************************************************/ 00087 00088 00089 /***************************************************************************** 00090 * PUBLIC FUNCTIONS * 00091 *---------------------------------------------------------------------------* 00092 * Add to this section all the functions that have global (project) scope. * 00093 * These functions can be accessed outside this module. * 00094 * These functions shall have their declarations (prototypes) within the * 00095 * interface header file and shall be preceded by the 'extern' keyword. * 00096 *---------------------------------------------------------------------------* 00097 *****************************************************************************/ 00098 00099 /*--------------------------------------------------------------------------- 00100 * Name: MCR20Drv_Init 00101 * Description: - 00102 * Parameters: - 00103 * Return: - 00104 *---------------------------------------------------------------------------*/ 00105 void MCR20Drv_Init 00106 ( 00107 void 00108 ) 00109 { 00110 xcvr_spi_init(gXcvrSpiInstance_c); 00111 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00112 00113 gXcvrDeassertCS_d(); 00114 #if !defined(TARGET_KW24D) 00115 MCR20Drv_RST_B_Deassert(); 00116 #endif 00117 RF_IRQ_Init(); 00118 RF_IRQ_Disable(); 00119 mPhyIrqDisableCnt = 1; 00120 } 00121 00122 /*--------------------------------------------------------------------------- 00123 * Name: MCR20Drv_DirectAccessSPIWrite 00124 * Description: - 00125 * Parameters: - 00126 * Return: - 00127 *---------------------------------------------------------------------------*/ 00128 void MCR20Drv_DirectAccessSPIWrite 00129 ( 00130 uint8_t address, 00131 uint8_t value 00132 ) 00133 { 00134 uint16_t txData; 00135 00136 ProtectFromMCR20Interrupt(); 00137 00138 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00139 00140 gXcvrAssertCS_d(); 00141 00142 txData = (address & TransceiverSPI_DirectRegisterAddressMask); 00143 txData |= value << 8; 00144 00145 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t *)&txData, 0, sizeof(txData)); 00146 00147 gXcvrDeassertCS_d(); 00148 UnprotectFromMCR20Interrupt(); 00149 } 00150 00151 /*--------------------------------------------------------------------------- 00152 * Name: MCR20Drv_DirectAccessSPIMultiByteWrite 00153 * Description: - 00154 * Parameters: - 00155 * Return: - 00156 *---------------------------------------------------------------------------*/ 00157 void MCR20Drv_DirectAccessSPIMultiByteWrite 00158 ( 00159 uint8_t startAddress, 00160 uint8_t * byteArray, 00161 uint8_t numOfBytes 00162 ) 00163 { 00164 uint8_t txData; 00165 00166 if( (numOfBytes == 0) || (byteArray == 0) ) 00167 { 00168 return; 00169 } 00170 00171 ProtectFromMCR20Interrupt(); 00172 00173 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00174 00175 gXcvrAssertCS_d(); 00176 00177 txData = (startAddress & TransceiverSPI_DirectRegisterAddressMask); 00178 00179 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, sizeof(txData)); 00180 xcvr_spi_transfer(gXcvrSpiInstance_c, byteArray, 0, numOfBytes); 00181 00182 gXcvrDeassertCS_d(); 00183 UnprotectFromMCR20Interrupt(); 00184 } 00185 00186 /*--------------------------------------------------------------------------- 00187 * Name: MCR20Drv_PB_SPIByteWrite 00188 * Description: - 00189 * Parameters: - 00190 * Return: - 00191 *---------------------------------------------------------------------------*/ 00192 void MCR20Drv_PB_SPIByteWrite 00193 ( 00194 uint8_t address, 00195 uint8_t value 00196 ) 00197 { 00198 uint32_t txData; 00199 00200 ProtectFromMCR20Interrupt(); 00201 00202 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00203 00204 gXcvrAssertCS_d(); 00205 00206 txData = TransceiverSPI_WriteSelect | 00207 TransceiverSPI_PacketBuffAccessSelect | 00208 TransceiverSPI_PacketBuffByteModeSelect; 00209 txData |= (address) << 8; 00210 txData |= (value) << 16; 00211 00212 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, 3); 00213 00214 gXcvrDeassertCS_d(); 00215 UnprotectFromMCR20Interrupt(); 00216 } 00217 00218 /*--------------------------------------------------------------------------- 00219 * Name: MCR20Drv_PB_SPIBurstWrite 00220 * Description: - 00221 * Parameters: - 00222 * Return: - 00223 *---------------------------------------------------------------------------*/ 00224 void MCR20Drv_PB_SPIBurstWrite 00225 ( 00226 uint8_t * byteArray, 00227 uint8_t numOfBytes 00228 ) 00229 { 00230 uint8_t txData; 00231 00232 if( (numOfBytes == 0) || (byteArray == 0) ) 00233 { 00234 return; 00235 } 00236 00237 ProtectFromMCR20Interrupt(); 00238 00239 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00240 00241 gXcvrAssertCS_d(); 00242 00243 txData = TransceiverSPI_WriteSelect | 00244 TransceiverSPI_PacketBuffAccessSelect | 00245 TransceiverSPI_PacketBuffBurstModeSelect; 00246 00247 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, 1); 00248 xcvr_spi_transfer(gXcvrSpiInstance_c, byteArray, 0, numOfBytes); 00249 00250 gXcvrDeassertCS_d(); 00251 UnprotectFromMCR20Interrupt(); 00252 } 00253 00254 /*--------------------------------------------------------------------------- 00255 * Name: MCR20Drv_DirectAccessSPIRead 00256 * Description: - 00257 * Parameters: - 00258 * Return: - 00259 *---------------------------------------------------------------------------*/ 00260 00261 uint8_t MCR20Drv_DirectAccessSPIRead 00262 ( 00263 uint8_t address 00264 ) 00265 { 00266 uint8_t txData; 00267 uint8_t rxData; 00268 00269 ProtectFromMCR20Interrupt(); 00270 00271 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00272 00273 gXcvrAssertCS_d(); 00274 00275 txData = (address & TransceiverSPI_DirectRegisterAddressMask) | 00276 TransceiverSPI_ReadSelect; 00277 00278 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, 0, sizeof(txData)); 00279 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, &rxData, sizeof(rxData)); 00280 00281 gXcvrDeassertCS_d(); 00282 UnprotectFromMCR20Interrupt(); 00283 00284 return rxData; 00285 00286 } 00287 00288 /*--------------------------------------------------------------------------- 00289 * Name: MCR20Drv_DirectAccessSPIMultyByteRead 00290 * Description: - 00291 * Parameters: - 00292 * Return: - 00293 *---------------------------------------------------------------------------*/ 00294 uint8_t MCR20Drv_DirectAccessSPIMultiByteRead 00295 ( 00296 uint8_t startAddress, 00297 uint8_t * byteArray, 00298 uint8_t numOfBytes 00299 ) 00300 { 00301 uint8_t txData; 00302 uint8_t phyIRQSTS1; 00303 00304 if( (numOfBytes == 0) || (byteArray == 0) ) 00305 { 00306 return 0; 00307 } 00308 00309 ProtectFromMCR20Interrupt(); 00310 00311 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00312 00313 gXcvrAssertCS_d(); 00314 00315 txData = (startAddress & TransceiverSPI_DirectRegisterAddressMask) | 00316 TransceiverSPI_ReadSelect; 00317 00318 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, &phyIRQSTS1, sizeof(txData)); 00319 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00320 00321 gXcvrDeassertCS_d(); 00322 UnprotectFromMCR20Interrupt(); 00323 00324 return phyIRQSTS1; 00325 } 00326 00327 /*--------------------------------------------------------------------------- 00328 * Name: MCR20Drv_PB_SPIBurstRead 00329 * Description: - 00330 * Parameters: - 00331 * Return: - 00332 *---------------------------------------------------------------------------*/ 00333 uint8_t MCR20Drv_PB_SPIBurstRead 00334 ( 00335 uint8_t * byteArray, 00336 uint8_t numOfBytes 00337 ) 00338 { 00339 uint8_t txData; 00340 uint8_t phyIRQSTS1; 00341 00342 if( (numOfBytes == 0) || (byteArray == 0) ) 00343 { 00344 return 0; 00345 } 00346 00347 ProtectFromMCR20Interrupt(); 00348 00349 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00350 00351 gXcvrAssertCS_d(); 00352 00353 txData = TransceiverSPI_ReadSelect | 00354 TransceiverSPI_PacketBuffAccessSelect | 00355 TransceiverSPI_PacketBuffBurstModeSelect; 00356 00357 xcvr_spi_transfer(gXcvrSpiInstance_c, &txData, &phyIRQSTS1, sizeof(txData)); 00358 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00359 00360 gXcvrDeassertCS_d(); 00361 UnprotectFromMCR20Interrupt(); 00362 00363 return phyIRQSTS1; 00364 } 00365 00366 /*--------------------------------------------------------------------------- 00367 * Name: MCR20Drv_IndirectAccessSPIWrite 00368 * Description: - 00369 * Parameters: - 00370 * Return: - 00371 *---------------------------------------------------------------------------*/ 00372 void MCR20Drv_IndirectAccessSPIWrite 00373 ( 00374 uint8_t address, 00375 uint8_t value 00376 ) 00377 { 00378 uint32_t txData; 00379 00380 ProtectFromMCR20Interrupt(); 00381 00382 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00383 00384 gXcvrAssertCS_d(); 00385 00386 txData = TransceiverSPI_IARIndexReg; 00387 txData |= (address) << 8; 00388 txData |= (value) << 16; 00389 00390 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, 3); 00391 00392 gXcvrDeassertCS_d(); 00393 UnprotectFromMCR20Interrupt(); 00394 } 00395 00396 /*--------------------------------------------------------------------------- 00397 * Name: MCR20Drv_IndirectAccessSPIMultiByteWrite 00398 * Description: - 00399 * Parameters: - 00400 * Return: - 00401 *---------------------------------------------------------------------------*/ 00402 void MCR20Drv_IndirectAccessSPIMultiByteWrite 00403 ( 00404 uint8_t startAddress, 00405 uint8_t * byteArray, 00406 uint8_t numOfBytes 00407 ) 00408 { 00409 uint16_t txData; 00410 00411 if( (numOfBytes == 0) || (byteArray == 0) ) 00412 { 00413 return; 00414 } 00415 00416 ProtectFromMCR20Interrupt(); 00417 00418 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 16000000); 00419 00420 gXcvrAssertCS_d(); 00421 00422 txData = TransceiverSPI_IARIndexReg; 00423 txData |= (startAddress) << 8; 00424 00425 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00426 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)byteArray, 0, numOfBytes); 00427 00428 gXcvrDeassertCS_d(); 00429 UnprotectFromMCR20Interrupt(); 00430 } 00431 00432 /*--------------------------------------------------------------------------- 00433 * Name: MCR20Drv_IndirectAccessSPIRead 00434 * Description: - 00435 * Parameters: - 00436 * Return: - 00437 *---------------------------------------------------------------------------*/ 00438 uint8_t MCR20Drv_IndirectAccessSPIRead 00439 ( 00440 uint8_t address 00441 ) 00442 { 00443 uint16_t txData; 00444 uint8_t rxData; 00445 00446 ProtectFromMCR20Interrupt(); 00447 00448 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00449 00450 gXcvrAssertCS_d(); 00451 00452 txData = TransceiverSPI_IARIndexReg | TransceiverSPI_ReadSelect; 00453 txData |= (address) << 8; 00454 00455 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00456 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, &rxData, sizeof(rxData)); 00457 00458 gXcvrDeassertCS_d(); 00459 UnprotectFromMCR20Interrupt(); 00460 00461 return rxData; 00462 } 00463 00464 /*--------------------------------------------------------------------------- 00465 * Name: MCR20Drv_IndirectAccessSPIMultiByteRead 00466 * Description: - 00467 * Parameters: - 00468 * Return: - 00469 *---------------------------------------------------------------------------*/ 00470 void MCR20Drv_IndirectAccessSPIMultiByteRead 00471 ( 00472 uint8_t startAddress, 00473 uint8_t * byteArray, 00474 uint8_t numOfBytes 00475 ) 00476 { 00477 uint16_t txData; 00478 00479 if( (numOfBytes == 0) || (byteArray == 0) ) 00480 { 00481 return; 00482 } 00483 00484 ProtectFromMCR20Interrupt(); 00485 00486 xcvr_spi_configure_speed(gXcvrSpiInstance_c, 8000000); 00487 00488 gXcvrAssertCS_d(); 00489 00490 txData = (TransceiverSPI_IARIndexReg | TransceiverSPI_ReadSelect); 00491 txData |= (startAddress) << 8; 00492 00493 xcvr_spi_transfer(gXcvrSpiInstance_c, (uint8_t*)&txData, 0, sizeof(txData)); 00494 xcvr_spi_transfer(gXcvrSpiInstance_c, 0, byteArray, numOfBytes); 00495 00496 gXcvrDeassertCS_d(); 00497 UnprotectFromMCR20Interrupt(); 00498 } 00499 00500 /*--------------------------------------------------------------------------- 00501 * Name: MCR20Drv_IsIrqPending 00502 * Description: - 00503 * Parameters: - 00504 * Return: - 00505 *---------------------------------------------------------------------------*/ 00506 uint32_t MCR20Drv_IsIrqPending 00507 ( 00508 void 00509 ) 00510 { 00511 return RF_isIRQ_Pending(); 00512 } 00513 00514 /*--------------------------------------------------------------------------- 00515 * Name: MCR20Drv_IRQ_Disable 00516 * Description: - 00517 * Parameters: - 00518 * Return: - 00519 *---------------------------------------------------------------------------*/ 00520 void MCR20Drv_IRQ_Disable 00521 ( 00522 void 00523 ) 00524 { 00525 platform_enter_critical(); 00526 00527 if( mPhyIrqDisableCnt == 0 ) 00528 { 00529 RF_IRQ_Disable(); 00530 } 00531 00532 mPhyIrqDisableCnt++; 00533 00534 platform_exit_critical(); 00535 } 00536 00537 /*--------------------------------------------------------------------------- 00538 * Name: MCR20Drv_IRQ_Enable 00539 * Description: - 00540 * Parameters: - 00541 * Return: - 00542 *---------------------------------------------------------------------------*/ 00543 void MCR20Drv_IRQ_Enable 00544 ( 00545 void 00546 ) 00547 { 00548 platform_enter_critical(); 00549 00550 if( mPhyIrqDisableCnt ) 00551 { 00552 mPhyIrqDisableCnt--; 00553 00554 if( mPhyIrqDisableCnt == 0 ) 00555 { 00556 RF_IRQ_Enable(); 00557 } 00558 } 00559 00560 platform_exit_critical(); 00561 } 00562 00563 /*--------------------------------------------------------------------------- 00564 * Name: MCR20Drv_RST_Assert 00565 * Description: - 00566 * Parameters: - 00567 * Return: - 00568 *---------------------------------------------------------------------------*/ 00569 void MCR20Drv_RST_B_Assert 00570 ( 00571 void 00572 ) 00573 { 00574 RF_RST_Set(0); 00575 } 00576 00577 /*--------------------------------------------------------------------------- 00578 * Name: MCR20Drv_RST_Deassert 00579 * Description: - 00580 * Parameters: - 00581 * Return: - 00582 *---------------------------------------------------------------------------*/ 00583 void MCR20Drv_RST_B_Deassert 00584 ( 00585 void 00586 ) 00587 { 00588 RF_RST_Set(1); 00589 } 00590 00591 /*--------------------------------------------------------------------------- 00592 * Name: MCR20Drv_SoftRST_Assert 00593 * Description: - 00594 * Parameters: - 00595 * Return: - 00596 *---------------------------------------------------------------------------*/ 00597 void MCR20Drv_SoftRST_Assert 00598 ( 00599 void 00600 ) 00601 { 00602 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x80)); 00603 } 00604 00605 /*--------------------------------------------------------------------------- 00606 * Name: MCR20Drv_SoftRST_Deassert 00607 * Description: - 00608 * Parameters: - 00609 * Return: - 00610 *---------------------------------------------------------------------------*/ 00611 void MCR20Drv_SoftRST_Deassert 00612 ( 00613 void 00614 ) 00615 { 00616 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x00)); 00617 } 00618 00619 /*--------------------------------------------------------------------------- 00620 * Name: MCR20Drv_Soft_RESET 00621 * Description: - 00622 * Parameters: - 00623 * Return: - 00624 *---------------------------------------------------------------------------*/ 00625 void MCR20Drv_Soft_RESET 00626 ( 00627 void 00628 ) 00629 { 00630 //assert SOG_RST 00631 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x80)); 00632 00633 //deassert SOG_RST 00634 MCR20Drv_IndirectAccessSPIWrite(SOFT_RESET, (0x00)); 00635 } 00636 00637 /*--------------------------------------------------------------------------- 00638 * Name: MCR20Drv_RESET 00639 * Description: - 00640 * Parameters: - 00641 * Return: - 00642 *---------------------------------------------------------------------------*/ 00643 void MCR20Drv_RESET 00644 ( 00645 void 00646 ) 00647 { 00648 #if !defined(TARGET_KW24D) 00649 volatile uint32_t delay = 1000; 00650 //assert RST_B 00651 MCR20Drv_RST_B_Assert(); 00652 00653 while(delay--); 00654 00655 //deassert RST_B 00656 MCR20Drv_RST_B_Deassert(); 00657 #endif 00658 } 00659 00660 /*--------------------------------------------------------------------------- 00661 * Name: MCR20Drv_Set_CLK_OUT_Freq 00662 * Description: - 00663 * Parameters: - 00664 * Return: - 00665 *---------------------------------------------------------------------------*/ 00666 void MCR20Drv_Set_CLK_OUT_Freq 00667 ( 00668 uint8_t freqDiv 00669 ) 00670 { 00671 uint8_t clkOutCtrlReg = (freqDiv & cCLK_OUT_DIV_Mask) | cCLK_OUT_EN | cCLK_OUT_EXTEND; 00672 00673 if(freqDiv == gCLK_OUT_FREQ_DISABLE) 00674 { 00675 clkOutCtrlReg = (cCLK_OUT_EXTEND | gCLK_OUT_FREQ_4_MHz); //reset value with clock out disabled 00676 } 00677 00678 MCR20Drv_DirectAccessSPIWrite((uint8_t) CLK_OUT_CTRL, clkOutCtrlReg); 00679 }
Generated on Tue Jul 12 2022 15:14:29 by
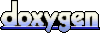