
Websocket client cellular hello world example
Dependencies: C027_Support WebSocketClient mbed
Fork of Websocket_Ethernet_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "Websocket.h" 00003 00004 //------------------------------------------------------------------------------------ 00005 // You need to configure these cellular modem / SIM parameters. 00006 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00007 //------------------------------------------------------------------------------------ 00008 #include "MDM.h" 00009 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00010 #define SIMPIN NULL 00011 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00012 contract with the network operator. You can also try to look-up your settings in 00013 google: https://www.google.de/search?q=APN+list */ 00014 #define APN NULL 00015 //! Set the user name for your APN, or NULL if not needed 00016 #define USERNAME NULL 00017 //! Set the password for your APN, or NULL if not needed 00018 #define PASSWORD NULL 00019 //------------------------------------------------------------------------------------ 00020 00021 Ticker flash; 00022 DigitalOut led(LED1); 00023 void flashLED(void){led = !led;} 00024 00025 int main() 00026 { 00027 flash.attach(&flashLED, 1.0f); 00028 MDMSerial mdm; 00029 //mdm.setDebug(4); // enable this for debugging issues 00030 if (!mdm.connect(SIMPIN, APN,USERNAME,PASSWORD)) 00031 return -1; 00032 00033 // view @ http://sockets.mbed.org/demo/viewer 00034 printf("Websocket View at http://sockets.mbed.org/demo/viewer\n"); 00035 Websocket ws("ws://sockets.mbed.org:443/ws/demo/rw"); 00036 00037 printf("Websocket\n"); 00038 ws.connect(); 00039 char str[100]; 00040 printf("Websocket connected\n"); 00041 00042 for(int i=0; i<0x7fffffff; ++i) { 00043 // string with a message 00044 sprintf(str, "%d WebSocket Hello World over Cellular", i); 00045 ws.send(str); 00046 00047 // clear the buffer and wait a sec... 00048 memset(str, 0, 100); 00049 wait(0.5f); 00050 00051 // websocket server should echo whatever we sent it 00052 if (ws.read(str)) { 00053 printf("rcv'd: %s\n", str); 00054 } 00055 } 00056 ws.close(); 00057 00058 mdm.disconnect(); 00059 mdm.powerOff(); 00060 00061 while(true); 00062 }
Generated on Wed Jul 13 2022 04:38:17 by
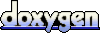