
port to cellular
Dependencies: C027_Support M2XStreamClient jsonlite mbed-rtos mbed
Fork of Cellular_m2x-demo-all by
main.cpp
00001 #include <jsonlite.h> 00002 #include "M2XStreamClient.h" 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #define RTOS_H 00007 #include "GPS.h" //GPS 00008 00009 //------------------------------------------------------------------------------------ 00010 // You need to configure these cellular modem / SIM parameters. 00011 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00012 //------------------------------------------------------------------------------------ 00013 #include "MDM.h" 00014 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00015 #define SIMPIN NULL 00016 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00017 contract with the network operator. You can also try to look-up your settings in 00018 google: https://www.google.de/search?q=APN+list */ 00019 #define APN NULL 00020 //! Set the user name for your APN, or NULL if not needed 00021 #define USERNAME NULL 00022 //! Set the password for your APN, or NULL if not needed 00023 #define PASSWORD NULL 00024 //------------------------------------------------------------------------------------ 00025 00026 char feedId[] = "81b9fcc5a8585c55ae622488f50d8de0"; // Feed you want to post to 00027 char m2xKey[] = "1e1133cd475954868602c0f7503d4f22"; // Your M2X access key 00028 00029 char name[] = "<location name>"; // Name of current location of datasource 00030 double latitude = 33.007872; 00031 double longitude = -96.751614; // You can also read those values from a GPS 00032 double elevation = 697.00; 00033 bool location_valid = false; 00034 00035 Client client; 00036 M2XStreamClient m2xClient(&client, m2xKey); 00037 00038 void on_data_point_found(const char* at, const char* value, int index, void* context, int type) { 00039 printf("Found a data point, index: %d\r\n", index); 00040 printf("At: %s Value: %s\r\n", at, value); 00041 } 00042 00043 void on_location_found(const char* name, 00044 double latitude, 00045 double longitude, 00046 double elevation, 00047 const char* timestamp, 00048 int index, 00049 void* context) { 00050 printf("Found a location, index: %d\r\n", index); 00051 printf("Name: %s Latitude: %lf Longitude: %lf\r\n", name, latitude, longitude); 00052 printf("Elevation: %lf Timestamp: %s\r\n", elevation, timestamp); 00053 } 00054 00055 void gpsTask(void const* argument) 00056 { 00057 GPSI2C gps; 00058 char buf[256]; 00059 while (1) { 00060 int ret = gps.getMessage(buf, sizeof(buf)); 00061 if (ret > 0) { 00062 int len = LENGTH(ret); 00063 if ((PROTOCOL(ret) == GPSParser::NMEA) && (len > 6)) { 00064 // talker is $GA=Galileo $GB=Beidou $GL=Glonass $GN=Combined $GP=GPS 00065 if ((buf[0] == '$') || buf[1] == 'G') { 00066 #define _CHECK_TALKER(s) ((buf[3] == s[0]) && (buf[4] == s[1]) && (buf[5] == s[2])) 00067 if (_CHECK_TALKER("GGA")) { 00068 //printf("%.*sr\n", len, buf); 00069 char ch; 00070 if (gps.getNmeaAngle(2,buf,len,latitude) && 00071 gps.getNmeaAngle(4,buf,len,longitude) && 00072 gps.getNmeaItem(6,buf,len,ch) && 00073 gps.getNmeaItem(9,buf,len,elevation)) { 00074 printf("GPS Location: %.5f %.5f %.1f %c\r\n", latitude, longitude, elevation, ch); 00075 location_valid = ch == '1' || ch == '2' || ch == '6'; 00076 } 00077 } 00078 } 00079 } 00080 } else 00081 Thread::wait(100); 00082 } 00083 } 00084 00085 int main() { 00086 #if defined(TARGET_STM) 00087 MDMSerial mdm(D8,D2); // use the serrial port on D2 / D8 00088 #else 00089 MDMSerial mdm; 00090 #endif 00091 GPSI2C gps; 00092 Thread task2(gpsTask, NULL, osPriorityNormal, 2048); 00093 00094 //mdm.setDebug(4); // enable this for debugging issues 00095 if (!mdm.connect(SIMPIN, APN,USERNAME,PASSWORD)) 00096 return -1; 00097 00098 char buf[256]; 00099 00100 Timer tmr; 00101 tmr.reset(); 00102 tmr.start(); 00103 while (1) { 00104 if (tmr.read_ms() > 1000) { 00105 tmr.reset(); 00106 tmr.start(); 00107 int response; 00108 00109 MDMParser::NetStatus status; 00110 if (mdm.checkNetStatus(&status)) { 00111 sprintf(buf, "%d", status.rssi); 00112 response = m2xClient.updateStreamValue(feedId, "rssi", buf); 00113 printf("Put response code: %d\r\n", response); 00114 if (response == -1) while (true) ; 00115 } 00116 #define READING 00117 #ifdef READING 00118 // read signal strength 00119 response = m2xClient.listStreamValues(feedId, "rssi", on_data_point_found, NULL); 00120 printf("Fetch response code: %d\r\n", response); 00121 if (response == -1) while (true) ; 00122 #endif 00123 // update location 00124 if (location_valid) { 00125 response = m2xClient.updateLocation(feedId, name, latitude, longitude, elevation); 00126 printf("updateLocation response code: %d\r\n", response); 00127 if (response == -1) while (true) ; 00128 } 00129 #ifdef READING 00130 // read location 00131 response = m2xClient.readLocation(feedId, on_location_found, NULL); 00132 printf("readLocation response code: %d\r\n", response); 00133 if (response == -1) while (true) ; 00134 #endif 00135 } 00136 else { 00137 Thread::wait(100); 00138 } 00139 } 00140 00141 mdm.disconnect(); 00142 mdm.powerOff(); 00143 }
Generated on Fri Jul 15 2022 02:46:15 by
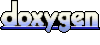