
C027_Support library test
Dependencies: C027_Support
Dependents: C027_SupportTest_xively_location software_test_v1
Fork of Seeed_GPRS_Library_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 00003 //------------------------------------------------------------------------------------ 00004 /* This example was tested on C027-U20 and C027-G35 with the on board modem. 00005 00006 Additionally it was tested with a shield where the SARA-G350/U260/U270 RX/TX/PWRON 00007 is connected to D0/D1/D4 and the GPS SCL/SDA is connected D15/D15. In this 00008 configuration the following platforms were tested (it is likely that others 00009 will work as well) 00010 - U-BLOX: C027-G35, C027-U20, C027-C20 (for shield set define C027_FORCE_SHIELD) 00011 - NXP: LPC1549v2, LPC4088qsb 00012 - Freescale: FRDM-KL05Z, FRDM-KL25Z, FRDM-KL46Z, FRDM-K64F 00013 - STM: NUCLEO-F401RE, NUCLEO-F030R8 00014 mount resistors SB13/14 1k, SB62/63 0R 00015 */ 00016 #include "GPS.h" 00017 #include "MDM.h" 00018 //------------------------------------------------------------------------------------ 00019 // You need to configure these cellular modem / SIM parameters. 00020 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00021 //------------------------------------------------------------------------------------ 00022 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00023 #define SIMPIN "1922" 00024 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00025 contract with the network operator. You can also try to look-up your settings in 00026 google: https://www.google.de/search?q=APN+list */ 00027 #define APN NULL 00028 //! Set the user name for your APN, or NULL if not needed 00029 #define USERNAME NULL 00030 //! Set the password for your APN, or NULL if not needed 00031 #define PASSWORD NULL 00032 //------------------------------------------------------------------------------------ 00033 00034 //#define CELLOCATE 00035 00036 int main(void) 00037 { 00038 int ret; 00039 #ifdef LARGE_DATA 00040 char buf[2048] = ""; 00041 #else 00042 char buf[512] = ""; 00043 #endif 00044 00045 // Create the GPS object 00046 #if 1 // use GPSI2C class 00047 GPSI2C gps; 00048 #else // or GPSSerial class 00049 GPSSerial gps; 00050 #endif 00051 // Create the modem object 00052 MDMSerial mdm; // use mdm(D1,D0) if you connect the cellular shield to a C027 00053 //mdm.setDebug(4); // enable this for debugging issues 00054 // initialize the modem 00055 MDMParser::DevStatus devStatus = {}; 00056 MDMParser::NetStatus netStatus = {}; 00057 bool mdmOk = mdm.init(SIMPIN, &devStatus); 00058 mdm.dumpDevStatus(&devStatus); 00059 if (mdmOk) { 00060 #if 0 00061 // file system API 00062 const char* filename = "File"; 00063 char buf[] = "Hello World"; 00064 printf("writeFile \"%s\"\r\n", buf); 00065 if (mdm.writeFile(filename, buf, sizeof(buf))) 00066 { 00067 memset(buf, 0, sizeof(buf)); 00068 int len = mdm.readFile(filename, buf, sizeof(buf)); 00069 if (len >= 0) 00070 printf("readFile %d \"%.*s\"\r\n", len, len, buf); 00071 mdm.delFile(filename); 00072 } 00073 #endif 00074 00075 // wait until we are connected 00076 mdmOk = mdm.registerNet(&netStatus); 00077 mdm.dumpNetStatus(&netStatus); 00078 } 00079 if (mdmOk) 00080 { 00081 // join the internet connection 00082 MDMParser::IP ip = mdm.join(APN,USERNAME,PASSWORD); 00083 if (ip == NOIP) 00084 printf("Not able to join network"); 00085 else 00086 { 00087 mdm.dumpIp(ip); 00088 printf("Make a Http Post Request\r\n"); 00089 int socket = mdm.socketSocket(MDMParser::IPPROTO_TCP); 00090 if (socket >= 0) 00091 { 00092 mdm.socketSetBlocking(socket, 10000); 00093 if (mdm.socketConnect(socket, "mbed.org", 80)) 00094 { 00095 const char http[] = "GET /media/uploads/mbed_official/hello.txt HTTP/1.0\r\n\r\n"; 00096 mdm.socketSend(socket, http, sizeof(http)-1); 00097 00098 ret = mdm.socketRecv(socket, buf, sizeof(buf)-1); 00099 if (ret > 0) 00100 printf("Socket Recv \"%*s\"\r\n", ret, buf); 00101 mdm.socketClose(socket); 00102 } 00103 mdm.socketFree(socket); 00104 } 00105 00106 int port = 7; 00107 const char* host = "echo.u-blox.com"; 00108 MDMParser::IP ip = mdm.gethostbyname(host); 00109 char data[] = "\r\nxxx Socket Hello World\r\n" 00110 #ifdef LARGE_DATA 00111 "00 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00112 "01 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00113 "02 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00114 "03 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00115 "04 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00116 00117 "05 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00118 "06 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00119 "07 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00120 "08 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00121 "09 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00122 00123 "10 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00124 "11 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00125 "12 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00126 "13 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00127 "14 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00128 00129 "15 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00130 "16 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00131 "17 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00132 "18 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00133 "19 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00134 #endif 00135 "End\r\n"; 00136 00137 printf("Testing TCP sockets with ECHO server\r\n"); 00138 socket = mdm.socketSocket(MDMParser::IPPROTO_TCP); 00139 if (socket >= 0) 00140 { 00141 mdm.socketSetBlocking(socket, 10000); 00142 if (mdm.socketConnect(socket, host, port)) { 00143 memcpy(data, "\r\nTCP", 5); 00144 ret = mdm.socketSend(socket, data, sizeof(data)-1); 00145 if (ret == sizeof(data)-1) { 00146 printf("Socket Send %d \"%s\"\r\n", ret, data); 00147 } 00148 ret = mdm.socketRecv(socket, buf, sizeof(buf)-1); 00149 if (ret >= 0) { 00150 printf("Socket Recv %d \"%.*s\"\r\n", ret, ret, buf); 00151 } 00152 mdm.socketClose(socket); 00153 } 00154 mdm.socketFree(socket); 00155 } 00156 00157 printf("Testing UDP sockets with ECHO server\r\n"); 00158 socket = mdm.socketSocket(MDMParser::IPPROTO_UDP, port); 00159 if (socket >= 0) 00160 { 00161 mdm.socketSetBlocking(socket, 10000); 00162 memcpy(data, "\r\nUDP", 5); 00163 ret = mdm.socketSendTo(socket, ip, port, data, sizeof(data)-1); 00164 if (ret == sizeof(data)-1) { 00165 printf("Socket SendTo %s:%d " IPSTR " %d \"%s\"\r\n", host, port, IPNUM(ip), ret, data); 00166 } 00167 ret = mdm.socketRecvFrom(socket, &ip, &port, buf, sizeof(buf)-1); 00168 if (ret >= 0) { 00169 printf("Socket RecvFrom " IPSTR ":%d %d \"%.*s\" \r\n", IPNUM(ip),port, ret, ret,buf); 00170 } 00171 mdm.socketFree(socket); 00172 } 00173 00174 // disconnect 00175 mdm.disconnect(); 00176 } 00177 00178 // http://www.geckobeach.com/cellular/secrets/gsmcodes.php 00179 // http://de.wikipedia.org/wiki/USSD-Codes 00180 const char* ussd = "*130#"; // You may get answer "UNKNOWN APPLICATION" 00181 printf("Ussd Send Command %s\r\n", ussd); 00182 ret = mdm.ussdCommand(ussd, buf); 00183 if (ret > 0) 00184 printf("Ussd Got Answer: \"%s\"\r\n", buf); 00185 } 00186 00187 printf("SMS and GPS Loop\r\n"); 00188 char link[128] = ""; 00189 unsigned int i = 0xFFFFFFFF; 00190 const int wait = 100; 00191 bool abort = false; 00192 #ifdef CELLOCATE 00193 const int sensorMask = 3; // Hybrid: GNSS + CellLocate 00194 const int timeoutMargin = 5; // seconds 00195 const int submitPeriod = 60; // 1 minutes in seconds 00196 const int targetAccuracy = 1; // meters 00197 unsigned int j = submitPeriod * 1000/wait; 00198 bool cellLocWait = false; 00199 MDMParser::CellLocData loc; 00200 00201 //Token can be released from u-blox site, when you got one replace "TOKEN" below 00202 if (!mdm.cellLocSrvHttp("TOKEN")) 00203 mdm.cellLocSrvUdp(); 00204 mdm.cellLocConfigSensor(1); // Deep scan mode 00205 //mdm.cellUnsolIndication(1); 00206 #endif 00207 //DigitalOut led(LED1); 00208 while (!abort) { 00209 // led = !led; 00210 #ifndef CELLOCATE 00211 while ((ret = gps.getMessage(buf, sizeof(buf))) > 0) 00212 { 00213 int len = LENGTH(ret); 00214 //printf("NMEA: %.*s\r\n", len-2, msg); 00215 if ((PROTOCOL(ret) == GPSParser::NMEA) && (len > 6)) 00216 { 00217 // talker is $GA=Galileo $GB=Beidou $GL=Glonass $GN=Combined $GP=GPS 00218 if ((buf[0] == '$') || buf[1] == 'G') { 00219 #define _CHECK_TALKER(s) ((buf[3] == s[0]) && (buf[4] == s[1]) && (buf[5] == s[2])) 00220 if (_CHECK_TALKER("GLL")) { 00221 double la = 0, lo = 0; 00222 char ch; 00223 if (gps.getNmeaAngle(1,buf,len,la) && 00224 gps.getNmeaAngle(3,buf,len,lo) && 00225 gps.getNmeaItem(6,buf,len,ch) && ch == 'A') 00226 { 00227 printf("GPS Location: %.5f %.5f\r\n", la, lo); 00228 sprintf(link, "I am here!\n" 00229 "https://maps.google.com/?q=%.5f,%.5f", la, lo); 00230 } 00231 } else if (_CHECK_TALKER("GGA") || _CHECK_TALKER("GNS") ) { 00232 double a = 0; 00233 if (gps.getNmeaItem(9,buf,len,a)) // altitude msl [m] 00234 printf("GPS Altitude: %.1f\r\n", a); 00235 } else if (_CHECK_TALKER("VTG")) { 00236 double s = 0; 00237 if (gps.getNmeaItem(7,buf,len,s)) // speed [km/h] 00238 printf("GPS Speed: %.1f\r\n", s); 00239 } 00240 } 00241 } 00242 } 00243 #endif 00244 #ifdef CELLOCATE 00245 if (mdmOk && (j++ == submitPeriod * 1000/wait)) { 00246 j=0; 00247 printf("CellLocate Request\r\n"); 00248 mdm.cellLocRequest(sensorMask, submitPeriod-timeoutMargin, targetAccuracy); 00249 cellLocWait = true; 00250 } 00251 if (cellLocWait && mdm.cellLocGet(&loc)){ 00252 cellLocWait = false; 00253 printf("CellLocate position received, sensor_used: %d, \r\n", loc.sensorUsed ); 00254 printf(" latitude: %0.5f, longitude: %0.5f, altitute: %d\r\n", loc.latitue, loc.longitude, loc.altitutude); 00255 if (loc.sensorUsed == 1) 00256 printf(" uncertainty: %d, speed: %d, direction: %d, vertical_acc: %d, satellite used: %d \r\n", loc.uncertainty,loc.speed,loc.direction,loc.verticalAcc,loc.svUsed); 00257 if (loc.sensorUsed == 1 || loc.sensorUsed == 2) 00258 sprintf(link, "I am here!\n" 00259 "https://maps.google.com/?q=%.5f,%.5f", loc.latitue, loc.longitude); 00260 } 00261 if (cellLocWait && (j%100 == 0 )) 00262 printf("Waiting for CellLocate...\r\n"); 00263 #endif 00264 if (mdmOk && (i++ == 5000/wait)) { 00265 i = 0; 00266 // check the network status 00267 if (mdm.checkNetStatus(&netStatus)) { 00268 mdm.dumpNetStatus(&netStatus, fprintf, stdout); 00269 } 00270 00271 // checking unread sms 00272 int ix[8]; 00273 int n = mdm.smsList("REC UNREAD", ix, 8); 00274 if (8 < n) n = 8; 00275 while (0 < n--) 00276 { 00277 char num[32]; 00278 printf("Unread SMS at index %d\r\n", ix[n]); 00279 if (mdm.smsRead(ix[n], num, buf, sizeof(buf))) { 00280 printf("Got SMS from \"%s\" with text \"%s\"\r\n", num, buf); 00281 printf("Delete SMS at index %d\r\n", ix[n]); 00282 mdm.smsDelete(ix[n]); 00283 // provide a reply 00284 const char* reply = "Hello my friend"; 00285 if (strstr(buf, /*w*/"here are you")) 00286 reply = *link ? link : "I don't know"; // reply wil location link 00287 else if (strstr(buf, /*s*/"hutdown")) 00288 abort = true, reply = "bye bye"; 00289 printf("Send SMS reply \"%s\" to \"%s\"\r\n", reply, num); 00290 mdm.smsSend(num, reply); 00291 } 00292 } 00293 } 00294 #ifdef RTOS_H 00295 Thread::wait(wait); 00296 #else 00297 ::wait_ms(wait); 00298 #endif 00299 } 00300 gps.powerOff(); 00301 mdm.powerOff(); 00302 return 0; 00303 }
Generated on Wed Jul 20 2022 08:27:44 by
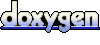