
Demo having the purpose to provide some examples about HTTP and HTTPS procedures.
Dependencies: C027_Support mbed
main.cpp
00001 #include "mbed.h" 00002 00003 //------------------------------------------------------------------------------------ 00004 /* This demo has the purpose to provide some examples about HTTP procedures. 00005 In details are implemented the following actions referring freely available online services: 00006 00007 - HTTP and HTTPS HEAD command -> www.developer.mbed.org 00008 - HTTP and HTTPS GET command -> www.developer.mbed.org 00009 - HTTP and HTTPS DELETE command -> www.httpbin.org 00010 - HTTP and HTTPS PUT command -> www.httpbin.org 00011 - HTTP and HTTPS POST file command -> www.posttestserver.com 00012 - HTTP and HTTPS POST data command -> www.posttestserver.com 00013 00014 P.S.: Please don't use confidential data when trying out the reported examples. 00015 As default, the httpInData variable is set to "Hello World" (see below). 00016 */ 00017 #include "MDM.h" 00018 //------------------------------------------------------------------------------------ 00019 // You need to configure these cellular modem / SIM parameters. 00020 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00021 //------------------------------------------------------------------------------------ 00022 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00023 #define SIMPIN NULL 00024 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00025 contract with the network operator. You can also try to look-up your settings in 00026 google: https://www.google.de/search?q=APN+list */ 00027 #define APN NULL 00028 //! Set the user name for your APN, or NULL if not needed 00029 #define USERNAME NULL 00030 //! Set the password for your APN, or NULL if not needed 00031 #define PASSWORD NULL 00032 //------------------------------------------------------------------------------------ 00033 // You need to configure the buffer data size 00034 //------------------------------------------------------------------------------------ 00035 //! Set the buffer size to 2048 bytes 00036 //#define LARGE_DATA 00037 //! Set the buffer size to 1024 bytes 00038 #define MEDIUM_DATA 00039 //------------------------------------------------------------------------------------ 00040 00041 int main(void) 00042 { 00043 int ret; 00044 #if defined(LARGE_DATA) 00045 printf("Set the buffer size to 2048 bytes\r\n"); 00046 char buf[2048] = ""; 00047 #elif defined(MEDIUM_DATA) 00048 printf("Set the buffer size to 1024 bytes\r\n"); 00049 char buf[1024] = ""; 00050 #else 00051 printf("Set the buffer size to 512 bytes\r\n"); 00052 char buf[512] = ""; 00053 #endif 00054 //give time to module for powering up (ms) 00055 wait_ms(1000); 00056 // Create the modem object 00057 MDMSerial mdm; 00058 //mdm.setDebug(4); // enable this for debugging issues 00059 // initialize the modem 00060 MDMParser::DevStatus devStatus = {}; 00061 MDMParser::NetStatus netStatus = {}; 00062 bool mdmOk = mdm.init(SIMPIN, &devStatus); 00063 mdm.dumpDevStatus(&devStatus); 00064 if (mdmOk) { 00065 #if 0 00066 // file system API 00067 const char* filename = "File"; 00068 char buf[] = "Hello World"; 00069 printf("writeFile \"%s\"\r\n", buf); 00070 if (mdm.writeFile(filename, buf, sizeof(buf))) 00071 { 00072 memset(buf, 0, sizeof(buf)); 00073 int len = mdm.readFile(filename, buf, sizeof(buf)); 00074 if (len >= 0) 00075 printf("readFile %d \"%.*s\"\r\n", len, len, buf); 00076 mdm.delFile(filename); 00077 } 00078 #endif 00079 00080 // wait until we are connected 00081 mdmOk = mdm.registerNet(&netStatus); 00082 mdm.dumpNetStatus(&netStatus); 00083 } 00084 if (mdmOk) 00085 { 00086 // http://www.geckobeach.com/cellular/secrets/gsmcodes.php 00087 // http://de.wikipedia.org/wiki/USSD-Codes 00088 const char* ussd = "*130#"; // You may get answer "UNKNOWN APPLICATION" 00089 printf("Ussd Send Command %s\r\n", ussd); 00090 ret = mdm.ussdCommand(ussd, buf); 00091 if (ret > 0) 00092 printf("Ussd Got Answer: \"%*s\"\r\n", ret, buf); 00093 00094 // join the internet connection 00095 MDMParser::IP ip = mdm.join(APN,USERNAME,PASSWORD); 00096 if (ip != NOIP) 00097 { 00098 mdm.dumpIp(ip); 00099 00100 printf("HTTP and HTTPS management\r\n"); 00101 char httpInData[] = "Hello World"; //input data for some HTTP commands 00102 char httpInFile[48] = "http_in.txt"; //file where saving HTTP commands data 00103 char httpOutFile[48] = "http_out.txt"; //file where saving HTTP commands results 00104 00105 int httpProfile = mdm.httpFindProfile(); //get the HTTP profile identifier 00106 if (httpProfile >= 0) 00107 { 00108 printf("Make an HTTP and HTTPS HEAD Request\r\n"); 00109 if(mdm.httpResetProfile(httpProfile)) 00110 { 00111 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"developer.mbed.org")) 00112 { 00113 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00114 00115 //HTTP HEAD command 00116 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_HEAD, 00117 "/media/uploads/mbed_official/hello.txt", 00118 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00119 if (ret > 0) 00120 printf("HTTP HEAD result \"%*s\"\r\n", ret, buf); 00121 00122 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00123 { 00124 //HTTPS HEAD command 00125 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_HEAD, 00126 "/media/uploads/mbed_official/hello.txt", 00127 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00128 if (ret > 0) 00129 printf("HTTPS HEAD result \"%*s\"\r\n", ret, buf); 00130 } else { 00131 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00132 } 00133 00134 //delete the HTTP output file 00135 mdm.delFile(httpOutFile); 00136 } else { 00137 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00138 } 00139 } else { 00140 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00141 } 00142 00143 printf("Make an HTTP and HTTPS GET Request\r\n"); 00144 if(mdm.httpResetProfile(httpProfile)) 00145 { 00146 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"developer.mbed.org")) 00147 { 00148 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00149 00150 //HTTP GET command 00151 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_GET, 00152 "/media/uploads/mbed_official/hello.txt", 00153 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00154 if (ret > 0) 00155 printf("HTTP GET result \"%*s\"\r\n", ret, buf); 00156 00157 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00158 { 00159 //HTTPS GET command 00160 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_GET, 00161 "/media/uploads/mbed_official/hello.txt", 00162 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00163 if (ret > 0) 00164 printf("HTTPS GET result \"%*s\"\r\n", ret, buf); 00165 } else { 00166 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00167 } 00168 00169 //delete the HTTP output file 00170 mdm.delFile(httpOutFile); 00171 } else { 00172 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00173 } 00174 } else { 00175 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00176 } 00177 00178 printf("Make an HTTP and HTTPS DELETE Request\r\n"); 00179 if(mdm.httpResetProfile(httpProfile)) 00180 { 00181 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"httpbin.org")) 00182 { 00183 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00184 00185 //HTTP DELETE command 00186 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_DELETE,"/delete", \ 00187 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00188 if (ret > 0) 00189 printf("HTTP DELETE result \"%*s\"\r\n", ret, buf); 00190 00191 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00192 { 00193 //HTTPS DELETE command 00194 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_DELETE,"/delete", \ 00195 httpOutFile,NULL,NULL,NULL,buf,sizeof(buf)); 00196 if (ret > 0) 00197 printf("HTTPS DELETE result \"%*s\"\r\n", ret, buf); 00198 } else { 00199 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00200 } 00201 00202 //delete the HTTP output file 00203 mdm.delFile(httpOutFile); 00204 00205 } else { 00206 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00207 } 00208 } else { 00209 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00210 } 00211 00212 printf("Make an HTTP and HTTPS PUT Request\r\n"); 00213 if(mdm.httpResetProfile(httpProfile)) 00214 { 00215 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"httpbin.org")) 00216 { 00217 if(mdm.writeFile(httpInFile,httpInData,sizeof(httpInData))) 00218 { 00219 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00220 00221 //HTTP PUT command 00222 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_PUT,"/put", \ 00223 httpOutFile,httpInFile,NULL,NULL,buf,sizeof(buf)); 00224 if (ret > 0) 00225 printf("HTTP PUT result \"%*s\"\r\n", ret, buf); 00226 00227 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00228 { 00229 //HTTPS PUT command 00230 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_PUT,"/put", \ 00231 httpOutFile,httpInFile,NULL,NULL,buf,sizeof(buf)); 00232 if (ret > 0) 00233 printf("HTTPS PUT result \"%*s\"\r\n", ret, buf); 00234 } else { 00235 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00236 } 00237 00238 //delete the HTTP input file 00239 mdm.delFile(httpInFile); 00240 00241 //delete the HTTP output file 00242 mdm.delFile(httpOutFile); 00243 } else { 00244 printf("Abnormal condition during the writing of the HTTP input file\r\n"); 00245 } 00246 } else { 00247 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00248 } 00249 } else { 00250 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00251 } 00252 00253 printf("Make an HTTP and HTTPS POST file command Request\r\n"); 00254 if(mdm.httpResetProfile(httpProfile)) 00255 { 00256 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"posttestserver.com")) 00257 { 00258 if(mdm.writeFile(httpInFile,httpInData,sizeof(httpInData))) 00259 { 00260 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00261 00262 //HTTP POST file command 00263 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_POST_FILE,"/post.php", \ 00264 httpOutFile,httpInFile,1,NULL,buf,sizeof(buf)); 00265 if (ret > 0) 00266 printf("HTTP POST file command result \"%*s\"\r\n", ret, buf); 00267 00268 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00269 { 00270 //HTTPS POST file command 00271 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_POST_FILE,"/post.php", \ 00272 httpOutFile,httpInFile,1,NULL,buf,sizeof(buf)); 00273 if (ret > 0) 00274 printf("HTTPS POST file command result \"%*s\"\r\n", ret, buf); 00275 } else { 00276 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00277 } 00278 //delete the HTTP input file 00279 mdm.delFile(httpInFile); 00280 00281 //delete the HTTP output file 00282 mdm.delFile(httpOutFile); 00283 } else { 00284 printf("Abnormal condition during the writing of the HTTP input file\r\n"); 00285 } 00286 } else { 00287 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00288 } 00289 } else { 00290 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00291 } 00292 00293 printf("Make an HTTP and HTTPS POST data command Request\r\n"); 00294 if(mdm.httpResetProfile(httpProfile)) 00295 { 00296 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SERVER_NAME,"posttestserver.com")) 00297 { 00298 if(mdm.writeFile(httpInFile,httpInData,sizeof(httpInData))) 00299 { 00300 mdm.httpSetBlocking(httpProfile, 10000); //timeout is 10000 ms: not blocking procedure 00301 00302 //HTTP POST data command 00303 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_POST_DATA,"/post.php", \ 00304 httpOutFile,httpInData,1,NULL,buf,sizeof(buf)); 00305 if (ret > 0) 00306 printf("HTTP POST data command result \"%*s\"\r\n", ret, buf); 00307 00308 if (mdm.httpSetPar(httpProfile,MDMParser::HTTP_SECURE,"1")) //HTTP Secure option enabled 00309 { 00310 //HTTPS POST data command 00311 ret = mdm.httpCommand(httpProfile,MDMParser::HTTP_POST_DATA,"/post.php", \ 00312 httpOutFile,httpInData,1,NULL,buf,sizeof(buf)); 00313 if (ret > 0) 00314 printf("HTTPS POST data command result \"%*s\"\r\n", ret, buf); 00315 } else { 00316 printf("Abnormal condition during the set of HTTP secure option\r\n"); 00317 } 00318 00319 //delete the HTTP input file 00320 mdm.delFile(httpInFile); 00321 00322 //delete the HTTP output file 00323 mdm.delFile(httpOutFile); 00324 } else { 00325 printf("Abnormal condition during the writing of the HTTP input file\r\n"); 00326 } 00327 } else { 00328 printf("Abnormal condition during the set of the HTTP server name\r\n"); 00329 } 00330 } else { 00331 printf("Abnormal condition during the reset of HTTP profile %d\r\n", httpProfile); 00332 } 00333 00334 //free current HTTP profile 00335 mdm.httpFreeProfile(httpProfile); 00336 } else { 00337 printf("Abnormal condition: no available HTTP profiles\r\n"); 00338 } 00339 00340 // disconnect 00341 mdm.disconnect(); 00342 } 00343 } 00344 else 00345 { 00346 //in this case the mdmOk is set to false 00347 printf("Please verify the SIM status and the network coverage\r\n"); 00348 } 00349 printf("Final loop: start\r\n"); 00350 int maxLoops = 3; 00351 const int wait = 1000; 00352 for (int i=0; i < maxLoops; i++) 00353 { 00354 if (mdmOk) 00355 { 00356 // check the network status 00357 if (mdm.checkNetStatus(&netStatus)) { 00358 mdm.dumpNetStatus(&netStatus, fprintf, stdout); 00359 } 00360 } 00361 wait_ms(wait); 00362 } 00363 printf("Final loop: end\r\n"); 00364 mdm.powerOff(); 00365 return 0; 00366 }
Generated on Wed Jul 13 2022 17:43:23 by
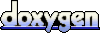