mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
x509.h
Go to the documentation of this file.
00001 /** 00002 * \file x509.h 00003 * 00004 * \brief X.509 generic defines and structures 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_X509_H 00024 #define MBEDTLS_X509_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include "asn1.h" 00033 #include "pk.h" 00034 00035 #if defined(MBEDTLS_RSA_C) 00036 #include "rsa.h" 00037 #endif 00038 00039 /** 00040 * \addtogroup x509_module 00041 * \{ 00042 */ 00043 00044 #if !defined(MBEDTLS_X509_MAX_INTERMEDIATE_CA) 00045 /** 00046 * Maximum number of intermediate CAs in a verification chain. 00047 * That is, maximum length of the chain, excluding the end-entity certificate 00048 * and the trusted root certificate. 00049 * 00050 * Set this to a low value to prevent an adversary from making you waste 00051 * resources verifying an overlong certificate chain. 00052 */ 00053 #define MBEDTLS_X509_MAX_INTERMEDIATE_CA 8 00054 #endif 00055 00056 /** 00057 * \name X509 Error codes 00058 * \{ 00059 */ 00060 #define MBEDTLS_ERR_X509_FEATURE_UNAVAILABLE -0x2080 /**< Unavailable feature, e.g. RSA hashing/encryption combination. */ 00061 #define MBEDTLS_ERR_X509_UNKNOWN_OID -0x2100 /**< Requested OID is unknown. */ 00062 #define MBEDTLS_ERR_X509_INVALID_FORMAT -0x2180 /**< The CRT/CRL/CSR format is invalid, e.g. different type expected. */ 00063 #define MBEDTLS_ERR_X509_INVALID_VERSION -0x2200 /**< The CRT/CRL/CSR version element is invalid. */ 00064 #define MBEDTLS_ERR_X509_INVALID_SERIAL -0x2280 /**< The serial tag or value is invalid. */ 00065 #define MBEDTLS_ERR_X509_INVALID_ALG -0x2300 /**< The algorithm tag or value is invalid. */ 00066 #define MBEDTLS_ERR_X509_INVALID_NAME -0x2380 /**< The name tag or value is invalid. */ 00067 #define MBEDTLS_ERR_X509_INVALID_DATE -0x2400 /**< The date tag or value is invalid. */ 00068 #define MBEDTLS_ERR_X509_INVALID_SIGNATURE -0x2480 /**< The signature tag or value invalid. */ 00069 #define MBEDTLS_ERR_X509_INVALID_EXTENSIONS -0x2500 /**< The extension tag or value is invalid. */ 00070 #define MBEDTLS_ERR_X509_UNKNOWN_VERSION -0x2580 /**< CRT/CRL/CSR has an unsupported version number. */ 00071 #define MBEDTLS_ERR_X509_UNKNOWN_SIG_ALG -0x2600 /**< Signature algorithm (oid) is unsupported. */ 00072 #define MBEDTLS_ERR_X509_SIG_MISMATCH -0x2680 /**< Signature algorithms do not match. (see \c ::mbedtls_x509_crt sig_oid) */ 00073 #define MBEDTLS_ERR_X509_CERT_VERIFY_FAILED -0x2700 /**< Certificate verification failed, e.g. CRL, CA or signature check failed. */ 00074 #define MBEDTLS_ERR_X509_CERT_UNKNOWN_FORMAT -0x2780 /**< Format not recognized as DER or PEM. */ 00075 #define MBEDTLS_ERR_X509_BAD_INPUT_DATA -0x2800 /**< Input invalid. */ 00076 #define MBEDTLS_ERR_X509_ALLOC_FAILED -0x2880 /**< Allocation of memory failed. */ 00077 #define MBEDTLS_ERR_X509_FILE_IO_ERROR -0x2900 /**< Read/write of file failed. */ 00078 #define MBEDTLS_ERR_X509_BUFFER_TOO_SMALL -0x2980 /**< Destination buffer is too small. */ 00079 /* \} name */ 00080 00081 /** 00082 * \name X509 Verify codes 00083 * \{ 00084 */ 00085 /* Reminder: update x509_crt_verify_strings[] in library/x509_crt.c */ 00086 #define MBEDTLS_X509_BADCERT_EXPIRED 0x01 /**< The certificate validity has expired. */ 00087 #define MBEDTLS_X509_BADCERT_REVOKED 0x02 /**< The certificate has been revoked (is on a CRL). */ 00088 #define MBEDTLS_X509_BADCERT_CN_MISMATCH 0x04 /**< The certificate Common Name (CN) does not match with the expected CN. */ 00089 #define MBEDTLS_X509_BADCERT_NOT_TRUSTED 0x08 /**< The certificate is not correctly signed by the trusted CA. */ 00090 #define MBEDTLS_X509_BADCRL_NOT_TRUSTED 0x10 /**< The CRL is not correctly signed by the trusted CA. */ 00091 #define MBEDTLS_X509_BADCRL_EXPIRED 0x20 /**< The CRL is expired. */ 00092 #define MBEDTLS_X509_BADCERT_MISSING 0x40 /**< Certificate was missing. */ 00093 #define MBEDTLS_X509_BADCERT_SKIP_VERIFY 0x80 /**< Certificate verification was skipped. */ 00094 #define MBEDTLS_X509_BADCERT_OTHER 0x0100 /**< Other reason (can be used by verify callback) */ 00095 #define MBEDTLS_X509_BADCERT_FUTURE 0x0200 /**< The certificate validity starts in the future. */ 00096 #define MBEDTLS_X509_BADCRL_FUTURE 0x0400 /**< The CRL is from the future */ 00097 #define MBEDTLS_X509_BADCERT_KEY_USAGE 0x0800 /**< Usage does not match the keyUsage extension. */ 00098 #define MBEDTLS_X509_BADCERT_EXT_KEY_USAGE 0x1000 /**< Usage does not match the extendedKeyUsage extension. */ 00099 #define MBEDTLS_X509_BADCERT_NS_CERT_TYPE 0x2000 /**< Usage does not match the nsCertType extension. */ 00100 #define MBEDTLS_X509_BADCERT_BAD_MD 0x4000 /**< The certificate is signed with an unacceptable hash. */ 00101 #define MBEDTLS_X509_BADCERT_BAD_PK 0x8000 /**< The certificate is signed with an unacceptable PK alg (eg RSA vs ECDSA). */ 00102 #define MBEDTLS_X509_BADCERT_BAD_KEY 0x010000 /**< The certificate is signed with an unacceptable key (eg bad curve, RSA too short). */ 00103 #define MBEDTLS_X509_BADCRL_BAD_MD 0x020000 /**< The CRL is signed with an unacceptable hash. */ 00104 #define MBEDTLS_X509_BADCRL_BAD_PK 0x040000 /**< The CRL is signed with an unacceptable PK alg (eg RSA vs ECDSA). */ 00105 #define MBEDTLS_X509_BADCRL_BAD_KEY 0x080000 /**< The CRL is signed with an unacceptable key (eg bad curve, RSA too short). */ 00106 00107 /* \} name */ 00108 /* \} addtogroup x509_module */ 00109 00110 /* 00111 * X.509 v3 Key Usage Extension flags 00112 * Reminder: update x509_info_key_usage() when adding new flags. 00113 */ 00114 #define MBEDTLS_X509_KU_DIGITAL_SIGNATURE (0x80) /* bit 0 */ 00115 #define MBEDTLS_X509_KU_NON_REPUDIATION (0x40) /* bit 1 */ 00116 #define MBEDTLS_X509_KU_KEY_ENCIPHERMENT (0x20) /* bit 2 */ 00117 #define MBEDTLS_X509_KU_DATA_ENCIPHERMENT (0x10) /* bit 3 */ 00118 #define MBEDTLS_X509_KU_KEY_AGREEMENT (0x08) /* bit 4 */ 00119 #define MBEDTLS_X509_KU_KEY_CERT_SIGN (0x04) /* bit 5 */ 00120 #define MBEDTLS_X509_KU_CRL_SIGN (0x02) /* bit 6 */ 00121 #define MBEDTLS_X509_KU_ENCIPHER_ONLY (0x01) /* bit 7 */ 00122 #define MBEDTLS_X509_KU_DECIPHER_ONLY (0x8000) /* bit 8 */ 00123 00124 /* 00125 * Netscape certificate types 00126 * (http://www.mozilla.org/projects/security/pki/nss/tech-notes/tn3.html) 00127 */ 00128 00129 #define MBEDTLS_X509_NS_CERT_TYPE_SSL_CLIENT (0x80) /* bit 0 */ 00130 #define MBEDTLS_X509_NS_CERT_TYPE_SSL_SERVER (0x40) /* bit 1 */ 00131 #define MBEDTLS_X509_NS_CERT_TYPE_EMAIL (0x20) /* bit 2 */ 00132 #define MBEDTLS_X509_NS_CERT_TYPE_OBJECT_SIGNING (0x10) /* bit 3 */ 00133 #define MBEDTLS_X509_NS_CERT_TYPE_RESERVED (0x08) /* bit 4 */ 00134 #define MBEDTLS_X509_NS_CERT_TYPE_SSL_CA (0x04) /* bit 5 */ 00135 #define MBEDTLS_X509_NS_CERT_TYPE_EMAIL_CA (0x02) /* bit 6 */ 00136 #define MBEDTLS_X509_NS_CERT_TYPE_OBJECT_SIGNING_CA (0x01) /* bit 7 */ 00137 00138 /* 00139 * X.509 extension types 00140 * 00141 * Comments refer to the status for using certificates. Status can be 00142 * different for writing certificates or reading CRLs or CSRs. 00143 */ 00144 #define MBEDTLS_X509_EXT_AUTHORITY_KEY_IDENTIFIER (1 << 0) 00145 #define MBEDTLS_X509_EXT_SUBJECT_KEY_IDENTIFIER (1 << 1) 00146 #define MBEDTLS_X509_EXT_KEY_USAGE (1 << 2) 00147 #define MBEDTLS_X509_EXT_CERTIFICATE_POLICIES (1 << 3) 00148 #define MBEDTLS_X509_EXT_POLICY_MAPPINGS (1 << 4) 00149 #define MBEDTLS_X509_EXT_SUBJECT_ALT_NAME (1 << 5) /* Supported (DNS) */ 00150 #define MBEDTLS_X509_EXT_ISSUER_ALT_NAME (1 << 6) 00151 #define MBEDTLS_X509_EXT_SUBJECT_DIRECTORY_ATTRS (1 << 7) 00152 #define MBEDTLS_X509_EXT_BASIC_CONSTRAINTS (1 << 8) /* Supported */ 00153 #define MBEDTLS_X509_EXT_NAME_CONSTRAINTS (1 << 9) 00154 #define MBEDTLS_X509_EXT_POLICY_CONSTRAINTS (1 << 10) 00155 #define MBEDTLS_X509_EXT_EXTENDED_KEY_USAGE (1 << 11) 00156 #define MBEDTLS_X509_EXT_CRL_DISTRIBUTION_POINTS (1 << 12) 00157 #define MBEDTLS_X509_EXT_INIHIBIT_ANYPOLICY (1 << 13) 00158 #define MBEDTLS_X509_EXT_FRESHEST_CRL (1 << 14) 00159 00160 #define MBEDTLS_X509_EXT_NS_CERT_TYPE (1 << 16) /* Parsed (and then ?) */ 00161 00162 /* 00163 * Storage format identifiers 00164 * Recognized formats: PEM and DER 00165 */ 00166 #define MBEDTLS_X509_FORMAT_DER 1 00167 #define MBEDTLS_X509_FORMAT_PEM 2 00168 00169 #define MBEDTLS_X509_MAX_DN_NAME_SIZE 256 /**< Maximum value size of a DN entry */ 00170 00171 #ifdef __cplusplus 00172 extern "C" { 00173 #endif 00174 00175 /** 00176 * \addtogroup x509_module 00177 * \{ */ 00178 00179 /** 00180 * \name Structures for parsing X.509 certificates, CRLs and CSRs 00181 * \{ 00182 */ 00183 00184 /** 00185 * Type-length-value structure that allows for ASN1 using DER. 00186 */ 00187 typedef mbedtls_asn1_buf mbedtls_x509_buf; 00188 00189 /** 00190 * Container for ASN1 bit strings. 00191 */ 00192 typedef mbedtls_asn1_bitstring mbedtls_x509_bitstring; 00193 00194 /** 00195 * Container for ASN1 named information objects. 00196 * It allows for Relative Distinguished Names (e.g. cn=localhost,ou=code,etc.). 00197 */ 00198 typedef mbedtls_asn1_named_data mbedtls_x509_name; 00199 00200 /** 00201 * Container for a sequence of ASN.1 items 00202 */ 00203 typedef mbedtls_asn1_sequence mbedtls_x509_sequence; 00204 00205 /** Container for date and time (precision in seconds). */ 00206 typedef struct mbedtls_x509_time 00207 { 00208 int year, mon, day; /**< Date. */ 00209 int hour, min, sec; /**< Time. */ 00210 } 00211 mbedtls_x509_time; 00212 00213 /** \} name Structures for parsing X.509 certificates, CRLs and CSRs */ 00214 /** \} addtogroup x509_module */ 00215 00216 /** 00217 * \brief Store the certificate DN in printable form into buf; 00218 * no more than size characters will be written. 00219 * 00220 * \param buf Buffer to write to 00221 * \param size Maximum size of buffer 00222 * \param dn The X509 name to represent 00223 * 00224 * \return The length of the string written (not including the 00225 * terminated nul byte), or a negative error code. 00226 */ 00227 int mbedtls_x509_dn_gets( char *buf, size_t size, const mbedtls_x509_name *dn ); 00228 00229 /** 00230 * \brief Store the certificate serial in printable form into buf; 00231 * no more than size characters will be written. 00232 * 00233 * \param buf Buffer to write to 00234 * \param size Maximum size of buffer 00235 * \param serial The X509 serial to represent 00236 * 00237 * \return The length of the string written (not including the 00238 * terminated nul byte), or a negative error code. 00239 */ 00240 int mbedtls_x509_serial_gets( char *buf, size_t size, const mbedtls_x509_buf *serial ); 00241 00242 /** 00243 * \brief Check a given mbedtls_x509_time against the system time 00244 * and tell if it's in the past. 00245 * 00246 * \note Intended usage is "if( is_past( valid_to ) ) ERROR". 00247 * Hence the return value of 1 if on internal errors. 00248 * 00249 * \param time mbedtls_x509_time to check 00250 * 00251 * \return 1 if the given time is in the past or an error occured, 00252 * 0 otherwise. 00253 */ 00254 int mbedtls_x509_time_is_past( const mbedtls_x509_time *time ); 00255 00256 /** 00257 * \brief Check a given mbedtls_x509_time against the system time 00258 * and tell if it's in the future. 00259 * 00260 * \note Intended usage is "if( is_future( valid_from ) ) ERROR". 00261 * Hence the return value of 1 if on internal errors. 00262 * 00263 * \param time mbedtls_x509_time to check 00264 * 00265 * \return 1 if the given time is in the future or an error occured, 00266 * 0 otherwise. 00267 */ 00268 int mbedtls_x509_time_is_future( const mbedtls_x509_time *time ); 00269 00270 /** 00271 * \brief Checkup routine 00272 * 00273 * \return 0 if successful, or 1 if the test failed 00274 */ 00275 int mbedtls_x509_self_test( int verbose ); 00276 00277 /* 00278 * Internal module functions. You probably do not want to use these unless you 00279 * know you do. 00280 */ 00281 int mbedtls_x509_get_name( unsigned char **p, const unsigned char *end, 00282 mbedtls_x509_name *cur ); 00283 int mbedtls_x509_get_alg_null( unsigned char **p, const unsigned char *end, 00284 mbedtls_x509_buf *alg ); 00285 int mbedtls_x509_get_alg( unsigned char **p, const unsigned char *end, 00286 mbedtls_x509_buf *alg, mbedtls_x509_buf *params ); 00287 #if defined(MBEDTLS_X509_RSASSA_PSS_SUPPORT) 00288 int mbedtls_x509_get_rsassa_pss_params( const mbedtls_x509_buf *params, 00289 mbedtls_md_type_t *md_alg, mbedtls_md_type_t *mgf_md, 00290 int *salt_len ); 00291 #endif 00292 int mbedtls_x509_get_sig( unsigned char **p, const unsigned char *end, mbedtls_x509_buf *sig ); 00293 int mbedtls_x509_get_sig_alg( const mbedtls_x509_buf *sig_oid, const mbedtls_x509_buf *sig_params, 00294 mbedtls_md_type_t *md_alg, mbedtls_pk_type_t *pk_alg, 00295 void **sig_opts ); 00296 int mbedtls_x509_get_time( unsigned char **p, const unsigned char *end, 00297 mbedtls_x509_time *time ); 00298 int mbedtls_x509_get_serial( unsigned char **p, const unsigned char *end, 00299 mbedtls_x509_buf *serial ); 00300 int mbedtls_x509_get_ext( unsigned char **p, const unsigned char *end, 00301 mbedtls_x509_buf *ext, int tag ); 00302 int mbedtls_x509_sig_alg_gets( char *buf, size_t size, const mbedtls_x509_buf *sig_oid, 00303 mbedtls_pk_type_t pk_alg, mbedtls_md_type_t md_alg, 00304 const void *sig_opts ); 00305 int mbedtls_x509_key_size_helper( char *buf, size_t buf_size, const char *name ); 00306 int mbedtls_x509_string_to_names( mbedtls_asn1_named_data **head, const char *name ); 00307 int mbedtls_x509_set_extension( mbedtls_asn1_named_data **head, const char *oid, size_t oid_len, 00308 int critical, const unsigned char *val, 00309 size_t val_len ); 00310 int mbedtls_x509_write_extensions( unsigned char **p, unsigned char *start, 00311 mbedtls_asn1_named_data *first ); 00312 int mbedtls_x509_write_names( unsigned char **p, unsigned char *start, 00313 mbedtls_asn1_named_data *first ); 00314 int mbedtls_x509_write_sig( unsigned char **p, unsigned char *start, 00315 const char *oid, size_t oid_len, 00316 unsigned char *sig, size_t size ); 00317 00318 #define MBEDTLS_X509_SAFE_SNPRINTF \ 00319 do { \ 00320 if( ret < 0 || (size_t) ret >= n ) \ 00321 return( MBEDTLS_ERR_X509_BUFFER_TOO_SMALL ); \ 00322 \ 00323 n -= (size_t) ret; \ 00324 p += (size_t) ret; \ 00325 } while( 0 ) 00326 00327 #ifdef __cplusplus 00328 } 00329 #endif 00330 00331 #endif /* x509.h */
Generated on Tue Jul 12 2022 12:52:49 by
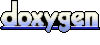