mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
sha512.h
00001 /** 00002 * \file mbedtls_sha512.h 00003 * 00004 * \brief SHA-384 and SHA-512 cryptographic hash function 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_SHA512_H 00024 #define MBEDTLS_SHA512_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #if !defined(MBEDTLS_SHA512_ALT) 00036 // Regular implementation 00037 // 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /** 00044 * \brief SHA-512 context structure 00045 */ 00046 typedef struct 00047 { 00048 uint64_t total[2]; /*!< number of bytes processed */ 00049 uint64_t state[8]; /*!< intermediate digest state */ 00050 unsigned char buffer[128]; /*!< data block being processed */ 00051 int is384 ; /*!< 0 => SHA-512, else SHA-384 */ 00052 } 00053 mbedtls_sha512_context; 00054 00055 /** 00056 * \brief Initialize SHA-512 context 00057 * 00058 * \param ctx SHA-512 context to be initialized 00059 */ 00060 void mbedtls_sha512_init( mbedtls_sha512_context *ctx ); 00061 00062 /** 00063 * \brief Clear SHA-512 context 00064 * 00065 * \param ctx SHA-512 context to be cleared 00066 */ 00067 void mbedtls_sha512_free( mbedtls_sha512_context *ctx ); 00068 00069 /** 00070 * \brief Clone (the state of) a SHA-512 context 00071 * 00072 * \param dst The destination context 00073 * \param src The context to be cloned 00074 */ 00075 void mbedtls_sha512_clone( mbedtls_sha512_context *dst, 00076 const mbedtls_sha512_context *src ); 00077 00078 /** 00079 * \brief SHA-512 context setup 00080 * 00081 * \param ctx context to be initialized 00082 * \param is384 0 = use SHA512, 1 = use SHA384 00083 */ 00084 void mbedtls_sha512_starts( mbedtls_sha512_context *ctx, int is384 ); 00085 00086 /** 00087 * \brief SHA-512 process buffer 00088 * 00089 * \param ctx SHA-512 context 00090 * \param input buffer holding the data 00091 * \param ilen length of the input data 00092 */ 00093 void mbedtls_sha512_update( mbedtls_sha512_context *ctx, const unsigned char *input, 00094 size_t ilen ); 00095 00096 /** 00097 * \brief SHA-512 final digest 00098 * 00099 * \param ctx SHA-512 context 00100 * \param output SHA-384/512 checksum result 00101 */ 00102 void mbedtls_sha512_finish( mbedtls_sha512_context *ctx, unsigned char output[64] ); 00103 00104 #ifdef __cplusplus 00105 } 00106 #endif 00107 00108 #else /* MBEDTLS_SHA512_ALT */ 00109 #include "sha512_alt.h" 00110 #endif /* MBEDTLS_SHA512_ALT */ 00111 00112 #ifdef __cplusplus 00113 extern "C" { 00114 #endif 00115 00116 /** 00117 * \brief Output = SHA-512( input buffer ) 00118 * 00119 * \param input buffer holding the data 00120 * \param ilen length of the input data 00121 * \param output SHA-384/512 checksum result 00122 * \param is384 0 = use SHA512, 1 = use SHA384 00123 */ 00124 void mbedtls_sha512( const unsigned char *input, size_t ilen, 00125 unsigned char output[64], int is384 ); 00126 00127 /** 00128 * \brief Checkup routine 00129 * 00130 * \return 0 if successful, or 1 if the test failed 00131 */ 00132 int mbedtls_sha512_self_test( int verbose ); 00133 00134 /* Internal use */ 00135 void mbedtls_sha512_process( mbedtls_sha512_context *ctx, const unsigned char data[128] ); 00136 00137 #ifdef __cplusplus 00138 } 00139 #endif 00140 00141 #endif /* mbedtls_sha512.h */
Generated on Tue Jul 12 2022 12:52:46 by
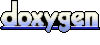