mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
md5.h
00001 /** 00002 * \file mbedtls_md5.h 00003 * 00004 * \brief MD5 message digest algorithm (hash function) 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_MD5_H 00024 #define MBEDTLS_MD5_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 #include <stdint.h> 00034 00035 #if !defined(MBEDTLS_MD5_ALT) 00036 // Regular implementation 00037 // 00038 00039 #ifdef __cplusplus 00040 extern "C" { 00041 #endif 00042 00043 /** 00044 * \brief MD5 context structure 00045 */ 00046 typedef struct 00047 { 00048 uint32_t total[2]; /*!< number of bytes processed */ 00049 uint32_t state[4]; /*!< intermediate digest state */ 00050 unsigned char buffer[64]; /*!< data block being processed */ 00051 } 00052 mbedtls_md5_context; 00053 00054 /** 00055 * \brief Initialize MD5 context 00056 * 00057 * \param ctx MD5 context to be initialized 00058 */ 00059 void mbedtls_md5_init( mbedtls_md5_context *ctx ); 00060 00061 /** 00062 * \brief Clear MD5 context 00063 * 00064 * \param ctx MD5 context to be cleared 00065 */ 00066 void mbedtls_md5_free( mbedtls_md5_context *ctx ); 00067 00068 /** 00069 * \brief Clone (the state of) an MD5 context 00070 * 00071 * \param dst The destination context 00072 * \param src The context to be cloned 00073 */ 00074 void mbedtls_md5_clone( mbedtls_md5_context *dst, 00075 const mbedtls_md5_context *src ); 00076 00077 /** 00078 * \brief MD5 context setup 00079 * 00080 * \param ctx context to be initialized 00081 */ 00082 void mbedtls_md5_starts( mbedtls_md5_context *ctx ); 00083 00084 /** 00085 * \brief MD5 process buffer 00086 * 00087 * \param ctx MD5 context 00088 * \param input buffer holding the data 00089 * \param ilen length of the input data 00090 */ 00091 void mbedtls_md5_update( mbedtls_md5_context *ctx, const unsigned char *input, size_t ilen ); 00092 00093 /** 00094 * \brief MD5 final digest 00095 * 00096 * \param ctx MD5 context 00097 * \param output MD5 checksum result 00098 */ 00099 void mbedtls_md5_finish( mbedtls_md5_context *ctx, unsigned char output[16] ); 00100 00101 /* Internal use */ 00102 void mbedtls_md5_process( mbedtls_md5_context *ctx, const unsigned char data[64] ); 00103 00104 #ifdef __cplusplus 00105 } 00106 #endif 00107 00108 #else /* MBEDTLS_MD5_ALT */ 00109 #include "md5_alt.h" 00110 #endif /* MBEDTLS_MD5_ALT */ 00111 00112 #ifdef __cplusplus 00113 extern "C" { 00114 #endif 00115 00116 /** 00117 * \brief Output = MD5( input buffer ) 00118 * 00119 * \param input buffer holding the data 00120 * \param ilen length of the input data 00121 * \param output MD5 checksum result 00122 */ 00123 void mbedtls_md5( const unsigned char *input, size_t ilen, unsigned char output[16] ); 00124 00125 /** 00126 * \brief Checkup routine 00127 * 00128 * \return 0 if successful, or 1 if the test failed 00129 */ 00130 int mbedtls_md5_self_test( int verbose ); 00131 00132 #ifdef __cplusplus 00133 } 00134 #endif 00135 00136 #endif /* mbedtls_md5.h */
Generated on Tue Jul 12 2022 12:52:44 by
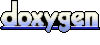