mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ecdsa.h
Go to the documentation of this file.
00001 /** 00002 * \file ecdsa.h 00003 * 00004 * \brief Elliptic curve DSA 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ECDSA_H 00024 #define MBEDTLS_ECDSA_H 00025 00026 #include "ecp.h" 00027 #include "md.h" 00028 00029 /* 00030 * RFC 4492 page 20: 00031 * 00032 * Ecdsa-Sig-Value ::= SEQUENCE { 00033 * r INTEGER, 00034 * s INTEGER 00035 * } 00036 * 00037 * Size is at most 00038 * 1 (tag) + 1 (len) + 1 (initial 0) + ECP_MAX_BYTES for each of r and s, 00039 * twice that + 1 (tag) + 2 (len) for the sequence 00040 * (assuming ECP_MAX_BYTES is less than 126 for r and s, 00041 * and less than 124 (total len <= 255) for the sequence) 00042 */ 00043 #if MBEDTLS_ECP_MAX_BYTES > 124 00044 #error "MBEDTLS_ECP_MAX_BYTES bigger than expected, please fix MBEDTLS_ECDSA_MAX_LEN" 00045 #endif 00046 /** Maximum size of an ECDSA signature in bytes */ 00047 #define MBEDTLS_ECDSA_MAX_LEN ( 3 + 2 * ( 3 + MBEDTLS_ECP_MAX_BYTES ) ) 00048 00049 /** 00050 * \brief ECDSA context structure 00051 */ 00052 typedef mbedtls_ecp_keypair mbedtls_ecdsa_context; 00053 00054 #ifdef __cplusplus 00055 extern "C" { 00056 #endif 00057 00058 /** 00059 * \brief Compute ECDSA signature of a previously hashed message 00060 * 00061 * \note The deterministic version is usually prefered. 00062 * 00063 * \param grp ECP group 00064 * \param r First output integer 00065 * \param s Second output integer 00066 * \param d Private signing key 00067 * \param buf Message hash 00068 * \param blen Length of buf 00069 * \param f_rng RNG function 00070 * \param p_rng RNG parameter 00071 * 00072 * \return 0 if successful, 00073 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00074 */ 00075 int mbedtls_ecdsa_sign( mbedtls_ecp_group *grp, mbedtls_mpi *r, mbedtls_mpi *s, 00076 const mbedtls_mpi *d, const unsigned char *buf, size_t blen, 00077 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00078 00079 #if defined(MBEDTLS_ECDSA_DETERMINISTIC) 00080 /** 00081 * \brief Compute ECDSA signature of a previously hashed message, 00082 * deterministic version (RFC 6979). 00083 * 00084 * \param grp ECP group 00085 * \param r First output integer 00086 * \param s Second output integer 00087 * \param d Private signing key 00088 * \param buf Message hash 00089 * \param blen Length of buf 00090 * \param md_alg MD algorithm used to hash the message 00091 * 00092 * \return 0 if successful, 00093 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00094 */ 00095 int mbedtls_ecdsa_sign_det( mbedtls_ecp_group *grp, mbedtls_mpi *r, mbedtls_mpi *s, 00096 const mbedtls_mpi *d, const unsigned char *buf, size_t blen, 00097 mbedtls_md_type_t md_alg ); 00098 #endif /* MBEDTLS_ECDSA_DETERMINISTIC */ 00099 00100 /** 00101 * \brief Verify ECDSA signature of a previously hashed message 00102 * 00103 * \param grp ECP group 00104 * \param buf Message hash 00105 * \param blen Length of buf 00106 * \param Q Public key to use for verification 00107 * \param r First integer of the signature 00108 * \param s Second integer of the signature 00109 * 00110 * \return 0 if successful, 00111 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if signature is invalid 00112 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00113 */ 00114 int mbedtls_ecdsa_verify( mbedtls_ecp_group *grp, 00115 const unsigned char *buf, size_t blen, 00116 const mbedtls_ecp_point *Q, const mbedtls_mpi *r, const mbedtls_mpi *s); 00117 00118 /** 00119 * \brief Compute ECDSA signature and write it to buffer, 00120 * serialized as defined in RFC 4492 page 20. 00121 * (Not thread-safe to use same context in multiple threads) 00122 * 00123 * \note The deterministice version (RFC 6979) is used if 00124 * MBEDTLS_ECDSA_DETERMINISTIC is defined. 00125 * 00126 * \param ctx ECDSA context 00127 * \param md_alg Algorithm that was used to hash the message 00128 * \param hash Message hash 00129 * \param hlen Length of hash 00130 * \param sig Buffer that will hold the signature 00131 * \param slen Length of the signature written 00132 * \param f_rng RNG function 00133 * \param p_rng RNG parameter 00134 * 00135 * \note The "sig" buffer must be at least as large as twice the 00136 * size of the curve used, plus 9 (eg. 73 bytes if a 256-bit 00137 * curve is used). MBEDTLS_ECDSA_MAX_LEN is always safe. 00138 * 00139 * \return 0 if successful, 00140 * or a MBEDTLS_ERR_ECP_XXX, MBEDTLS_ERR_MPI_XXX or 00141 * MBEDTLS_ERR_ASN1_XXX error code 00142 */ 00143 int mbedtls_ecdsa_write_signature( mbedtls_ecdsa_context *ctx, mbedtls_md_type_t md_alg, 00144 const unsigned char *hash, size_t hlen, 00145 unsigned char *sig, size_t *slen, 00146 int (*f_rng)(void *, unsigned char *, size_t), 00147 void *p_rng ); 00148 00149 #if defined(MBEDTLS_ECDSA_DETERMINISTIC) 00150 #if ! defined(MBEDTLS_DEPRECATED_REMOVED) 00151 #if defined(MBEDTLS_DEPRECATED_WARNING) 00152 #define MBEDTLS_DEPRECATED __attribute__((deprecated)) 00153 #else 00154 #define MBEDTLS_DEPRECATED 00155 #endif 00156 /** 00157 * \brief Compute ECDSA signature and write it to buffer, 00158 * serialized as defined in RFC 4492 page 20. 00159 * Deterministic version, RFC 6979. 00160 * (Not thread-safe to use same context in multiple threads) 00161 * 00162 * \deprecated Superseded by mbedtls_ecdsa_write_signature() in 2.0.0 00163 * 00164 * \param ctx ECDSA context 00165 * \param hash Message hash 00166 * \param hlen Length of hash 00167 * \param sig Buffer that will hold the signature 00168 * \param slen Length of the signature written 00169 * \param md_alg MD algorithm used to hash the message 00170 * 00171 * \note The "sig" buffer must be at least as large as twice the 00172 * size of the curve used, plus 9 (eg. 73 bytes if a 256-bit 00173 * curve is used). MBEDTLS_ECDSA_MAX_LEN is always safe. 00174 * 00175 * \return 0 if successful, 00176 * or a MBEDTLS_ERR_ECP_XXX, MBEDTLS_ERR_MPI_XXX or 00177 * MBEDTLS_ERR_ASN1_XXX error code 00178 */ 00179 int mbedtls_ecdsa_write_signature_det( mbedtls_ecdsa_context *ctx, 00180 const unsigned char *hash, size_t hlen, 00181 unsigned char *sig, size_t *slen, 00182 mbedtls_md_type_t md_alg ) MBEDTLS_DEPRECATED; 00183 #undef MBEDTLS_DEPRECATED 00184 #endif /* MBEDTLS_DEPRECATED_REMOVED */ 00185 #endif /* MBEDTLS_ECDSA_DETERMINISTIC */ 00186 00187 /** 00188 * \brief Read and verify an ECDSA signature 00189 * 00190 * \param ctx ECDSA context 00191 * \param hash Message hash 00192 * \param hlen Size of hash 00193 * \param sig Signature to read and verify 00194 * \param slen Size of sig 00195 * 00196 * \return 0 if successful, 00197 * MBEDTLS_ERR_ECP_BAD_INPUT_DATA if signature is invalid, 00198 * MBEDTLS_ERR_ECP_SIG_LEN_MISMATCH if the signature is 00199 * valid but its actual length is less than siglen, 00200 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_ERR_MPI_XXX error code 00201 */ 00202 int mbedtls_ecdsa_read_signature( mbedtls_ecdsa_context *ctx, 00203 const unsigned char *hash, size_t hlen, 00204 const unsigned char *sig, size_t slen ); 00205 00206 /** 00207 * \brief Generate an ECDSA keypair on the given curve 00208 * 00209 * \param ctx ECDSA context in which the keypair should be stored 00210 * \param gid Group (elliptic curve) to use. One of the various 00211 * MBEDTLS_ECP_DP_XXX macros depending on configuration. 00212 * \param f_rng RNG function 00213 * \param p_rng RNG parameter 00214 * 00215 * \return 0 on success, or a MBEDTLS_ERR_ECP_XXX code. 00216 */ 00217 int mbedtls_ecdsa_genkey( mbedtls_ecdsa_context *ctx, mbedtls_ecp_group_id gid, 00218 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00219 00220 /** 00221 * \brief Set an ECDSA context from an EC key pair 00222 * 00223 * \param ctx ECDSA context to set 00224 * \param key EC key to use 00225 * 00226 * \return 0 on success, or a MBEDTLS_ERR_ECP_XXX code. 00227 */ 00228 int mbedtls_ecdsa_from_keypair( mbedtls_ecdsa_context *ctx, const mbedtls_ecp_keypair *key ); 00229 00230 /** 00231 * \brief Initialize context 00232 * 00233 * \param ctx Context to initialize 00234 */ 00235 void mbedtls_ecdsa_init( mbedtls_ecdsa_context *ctx ); 00236 00237 /** 00238 * \brief Free context 00239 * 00240 * \param ctx Context to free 00241 */ 00242 void mbedtls_ecdsa_free( mbedtls_ecdsa_context *ctx ); 00243 00244 #ifdef __cplusplus 00245 } 00246 #endif 00247 00248 #endif /* ecdsa.h */
Generated on Tue Jul 12 2022 12:52:43 by
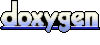