mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
ecdh.h
Go to the documentation of this file.
00001 /** 00002 * \file ecdh.h 00003 * 00004 * \brief Elliptic curve Diffie-Hellman 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ECDH_H 00024 #define MBEDTLS_ECDH_H 00025 00026 #include "ecp.h" 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 /** 00033 * When importing from an EC key, select if it is our key or the peer's key 00034 */ 00035 typedef enum 00036 { 00037 MBEDTLS_ECDH_OURS, 00038 MBEDTLS_ECDH_THEIRS, 00039 } mbedtls_ecdh_side; 00040 00041 /** 00042 * \brief ECDH context structure 00043 */ 00044 typedef struct 00045 { 00046 mbedtls_ecp_group grp ; /*!< elliptic curve used */ 00047 mbedtls_mpi d ; /*!< our secret value (private key) */ 00048 mbedtls_ecp_point Q ; /*!< our public value (public key) */ 00049 mbedtls_ecp_point Qp ; /*!< peer's public value (public key) */ 00050 mbedtls_mpi z ; /*!< shared secret */ 00051 int point_format ; /*!< format for point export in TLS messages */ 00052 mbedtls_ecp_point Vi ; /*!< blinding value (for later) */ 00053 mbedtls_ecp_point Vf ; /*!< un-blinding value (for later) */ 00054 mbedtls_mpi _d ; /*!< previous d (for later) */ 00055 } 00056 mbedtls_ecdh_context; 00057 00058 /** 00059 * \brief Generate a public key. 00060 * Raw function that only does the core computation. 00061 * 00062 * \param grp ECP group 00063 * \param d Destination MPI (secret exponent, aka private key) 00064 * \param Q Destination point (public key) 00065 * \param f_rng RNG function 00066 * \param p_rng RNG parameter 00067 * 00068 * \return 0 if successful, 00069 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00070 */ 00071 int mbedtls_ecdh_gen_public( mbedtls_ecp_group *grp, mbedtls_mpi *d, mbedtls_ecp_point *Q, 00072 int (*f_rng)(void *, unsigned char *, size_t), 00073 void *p_rng ); 00074 00075 /** 00076 * \brief Compute shared secret 00077 * Raw function that only does the core computation. 00078 * 00079 * \param grp ECP group 00080 * \param z Destination MPI (shared secret) 00081 * \param Q Public key from other party 00082 * \param d Our secret exponent (private key) 00083 * \param f_rng RNG function (see notes) 00084 * \param p_rng RNG parameter 00085 * 00086 * \return 0 if successful, 00087 * or a MBEDTLS_ERR_ECP_XXX or MBEDTLS_MPI_XXX error code 00088 * 00089 * \note If f_rng is not NULL, it is used to implement 00090 * countermeasures against potential elaborate timing 00091 * attacks, see \c mbedtls_ecp_mul() for details. 00092 */ 00093 int mbedtls_ecdh_compute_shared( mbedtls_ecp_group *grp, mbedtls_mpi *z, 00094 const mbedtls_ecp_point *Q, const mbedtls_mpi *d, 00095 int (*f_rng)(void *, unsigned char *, size_t), 00096 void *p_rng ); 00097 00098 /** 00099 * \brief Initialize context 00100 * 00101 * \param ctx Context to initialize 00102 */ 00103 void mbedtls_ecdh_init( mbedtls_ecdh_context *ctx ); 00104 00105 /** 00106 * \brief Free context 00107 * 00108 * \param ctx Context to free 00109 */ 00110 void mbedtls_ecdh_free( mbedtls_ecdh_context *ctx ); 00111 00112 /** 00113 * \brief Generate a public key and a TLS ServerKeyExchange payload. 00114 * (First function used by a TLS server for ECDHE.) 00115 * 00116 * \param ctx ECDH context 00117 * \param olen number of chars written 00118 * \param buf destination buffer 00119 * \param blen length of buffer 00120 * \param f_rng RNG function 00121 * \param p_rng RNG parameter 00122 * 00123 * \note This function assumes that ctx->grp has already been 00124 * properly set (for example using mbedtls_ecp_group_load). 00125 * 00126 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00127 */ 00128 int mbedtls_ecdh_make_params( mbedtls_ecdh_context *ctx, size_t *olen, 00129 unsigned char *buf, size_t blen, 00130 int (*f_rng)(void *, unsigned char *, size_t), 00131 void *p_rng ); 00132 00133 /** 00134 * \brief Parse and procress a TLS ServerKeyExhange payload. 00135 * (First function used by a TLS client for ECDHE.) 00136 * 00137 * \param ctx ECDH context 00138 * \param buf pointer to start of input buffer 00139 * \param end one past end of buffer 00140 * 00141 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00142 */ 00143 int mbedtls_ecdh_read_params( mbedtls_ecdh_context *ctx, 00144 const unsigned char **buf, const unsigned char *end ); 00145 00146 /** 00147 * \brief Setup an ECDH context from an EC key. 00148 * (Used by clients and servers in place of the 00149 * ServerKeyEchange for static ECDH: import ECDH parameters 00150 * from a certificate's EC key information.) 00151 * 00152 * \param ctx ECDH constext to set 00153 * \param key EC key to use 00154 * \param side Is it our key (1) or the peer's key (0) ? 00155 * 00156 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00157 */ 00158 int mbedtls_ecdh_get_params( mbedtls_ecdh_context *ctx, const mbedtls_ecp_keypair *key, 00159 mbedtls_ecdh_side side ); 00160 00161 /** 00162 * \brief Generate a public key and a TLS ClientKeyExchange payload. 00163 * (Second function used by a TLS client for ECDH(E).) 00164 * 00165 * \param ctx ECDH context 00166 * \param olen number of bytes actually written 00167 * \param buf destination buffer 00168 * \param blen size of destination buffer 00169 * \param f_rng RNG function 00170 * \param p_rng RNG parameter 00171 * 00172 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00173 */ 00174 int mbedtls_ecdh_make_public( mbedtls_ecdh_context *ctx, size_t *olen, 00175 unsigned char *buf, size_t blen, 00176 int (*f_rng)(void *, unsigned char *, size_t), 00177 void *p_rng ); 00178 00179 /** 00180 * \brief Parse and process a TLS ClientKeyExchange payload. 00181 * (Second function used by a TLS server for ECDH(E).) 00182 * 00183 * \param ctx ECDH context 00184 * \param buf start of input buffer 00185 * \param blen length of input buffer 00186 * 00187 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00188 */ 00189 int mbedtls_ecdh_read_public( mbedtls_ecdh_context *ctx, 00190 const unsigned char *buf, size_t blen ); 00191 00192 /** 00193 * \brief Derive and export the shared secret. 00194 * (Last function used by both TLS client en servers.) 00195 * 00196 * \param ctx ECDH context 00197 * \param olen number of bytes written 00198 * \param buf destination buffer 00199 * \param blen buffer length 00200 * \param f_rng RNG function, see notes for \c mbedtls_ecdh_compute_shared() 00201 * \param p_rng RNG parameter 00202 * 00203 * \return 0 if successful, or an MBEDTLS_ERR_ECP_XXX error code 00204 */ 00205 int mbedtls_ecdh_calc_secret( mbedtls_ecdh_context *ctx, size_t *olen, 00206 unsigned char *buf, size_t blen, 00207 int (*f_rng)(void *, unsigned char *, size_t), 00208 void *p_rng ); 00209 00210 #ifdef __cplusplus 00211 } 00212 #endif 00213 00214 #endif /* ecdh.h */
Generated on Tue Jul 12 2022 12:52:43 by
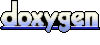