mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
debug.h
Go to the documentation of this file.
00001 /** 00002 * \file debug.h 00003 * 00004 * \brief Debug functions 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_DEBUG_H 00024 #define MBEDTLS_DEBUG_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include "ssl.h" 00033 00034 #if defined(MBEDTLS_ECP_C) 00035 #include "ecp.h" 00036 #endif 00037 00038 #if defined(MBEDTLS_DEBUG_C) 00039 00040 #define MBEDTLS_DEBUG_STRIP_PARENS( ... ) __VA_ARGS__ 00041 00042 #define MBEDTLS_SSL_DEBUG_MSG( level, args ) \ 00043 mbedtls_debug_print_msg( ssl, level, __FILE__, __LINE__, \ 00044 MBEDTLS_DEBUG_STRIP_PARENS args ) 00045 00046 #define MBEDTLS_SSL_DEBUG_RET( level, text, ret ) \ 00047 mbedtls_debug_print_ret( ssl, level, __FILE__, __LINE__, text, ret ) 00048 00049 #define MBEDTLS_SSL_DEBUG_BUF( level, text, buf, len ) \ 00050 mbedtls_debug_print_buf( ssl, level, __FILE__, __LINE__, text, buf, len ) 00051 00052 #if defined(MBEDTLS_BIGNUM_C) 00053 #define MBEDTLS_SSL_DEBUG_MPI( level, text, X ) \ 00054 mbedtls_debug_print_mpi( ssl, level, __FILE__, __LINE__, text, X ) 00055 #endif 00056 00057 #if defined(MBEDTLS_ECP_C) 00058 #define MBEDTLS_SSL_DEBUG_ECP( level, text, X ) \ 00059 mbedtls_debug_print_ecp( ssl, level, __FILE__, __LINE__, text, X ) 00060 #endif 00061 00062 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00063 #define MBEDTLS_SSL_DEBUG_CRT( level, text, crt ) \ 00064 mbedtls_debug_print_crt( ssl, level, __FILE__, __LINE__, text, crt ) 00065 #endif 00066 00067 #else /* MBEDTLS_DEBUG_C */ 00068 00069 #define MBEDTLS_SSL_DEBUG_MSG( level, args ) do { } while( 0 ) 00070 #define MBEDTLS_SSL_DEBUG_RET( level, text, ret ) do { } while( 0 ) 00071 #define MBEDTLS_SSL_DEBUG_BUF( level, text, buf, len ) do { } while( 0 ) 00072 #define MBEDTLS_SSL_DEBUG_MPI( level, text, X ) do { } while( 0 ) 00073 #define MBEDTLS_SSL_DEBUG_ECP( level, text, X ) do { } while( 0 ) 00074 #define MBEDTLS_SSL_DEBUG_CRT( level, text, crt ) do { } while( 0 ) 00075 00076 #endif /* MBEDTLS_DEBUG_C */ 00077 00078 #ifdef __cplusplus 00079 extern "C" { 00080 #endif 00081 00082 /** 00083 * \brief Set the level threshold to handle globally. Messages that have a 00084 * level over the threshold value are ignored. 00085 * (Default value: 0 (No debug)) 00086 * 00087 * \param threshold maximum level of messages to pass on 00088 */ 00089 void mbedtls_debug_set_threshold( int threshold ); 00090 00091 void mbedtls_debug_print_msg( const mbedtls_ssl_context *ssl, int level, 00092 const char *file, int line, 00093 const char *format, ... ); 00094 00095 void mbedtls_debug_print_ret( const mbedtls_ssl_context *ssl, int level, 00096 const char *file, int line, 00097 const char *text, int ret ); 00098 00099 void mbedtls_debug_print_buf( const mbedtls_ssl_context *ssl, int level, 00100 const char *file, int line, const char *text, 00101 const unsigned char *buf, size_t len ); 00102 00103 #if defined(MBEDTLS_BIGNUM_C) 00104 void mbedtls_debug_print_mpi( const mbedtls_ssl_context *ssl, int level, 00105 const char *file, int line, 00106 const char *text, const mbedtls_mpi *X ); 00107 #endif 00108 00109 #if defined(MBEDTLS_ECP_C) 00110 void mbedtls_debug_print_ecp( const mbedtls_ssl_context *ssl, int level, 00111 const char *file, int line, 00112 const char *text, const mbedtls_ecp_point *X ); 00113 #endif 00114 00115 #if defined(MBEDTLS_X509_CRT_PARSE_C) 00116 void mbedtls_debug_print_crt( const mbedtls_ssl_context *ssl, int level, 00117 const char *file, int line, 00118 const char *text, const mbedtls_x509_crt *crt ); 00119 #endif 00120 00121 #ifdef __cplusplus 00122 } 00123 #endif 00124 00125 #endif /* debug.h */
Generated on Tue Jul 12 2022 12:52:42 by
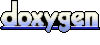