mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
cipher_wrap.c
Go to the documentation of this file.
00001 /** 00002 * \file cipher_wrap.c 00003 * 00004 * \brief Generic cipher wrapper for mbed TLS 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "mbedtls/config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #if defined(MBEDTLS_CIPHER_C) 00033 00034 #include "mbedtls/cipher_internal.h" 00035 00036 #if defined(MBEDTLS_AES_C) 00037 #include "mbedtls/aes.h" 00038 #endif 00039 00040 #if defined(MBEDTLS_ARC4_C) 00041 #include "mbedtls/arc4.h" 00042 #endif 00043 00044 #if defined(MBEDTLS_CAMELLIA_C) 00045 #include "mbedtls/camellia.h" 00046 #endif 00047 00048 #if defined(MBEDTLS_DES_C) 00049 #include "mbedtls/des.h" 00050 #endif 00051 00052 #if defined(MBEDTLS_BLOWFISH_C) 00053 #include "mbedtls/blowfish.h" 00054 #endif 00055 00056 #if defined(MBEDTLS_GCM_C) 00057 #include "mbedtls/gcm.h" 00058 #endif 00059 00060 #if defined(MBEDTLS_CCM_C) 00061 #include "mbedtls/ccm.h" 00062 #endif 00063 00064 #if defined(MBEDTLS_CIPHER_NULL_CIPHER) 00065 #include <string.h> 00066 #endif 00067 00068 #if defined(MBEDTLS_PLATFORM_C) 00069 #include "mbedtls/platform.h" 00070 #else 00071 #include <stdlib.h> 00072 #define mbedtls_calloc calloc 00073 #define mbedtls_free free 00074 #endif 00075 00076 #if defined(MBEDTLS_GCM_C) 00077 /* shared by all GCM ciphers */ 00078 static void *gcm_ctx_alloc( void ) 00079 { 00080 void *ctx = mbedtls_calloc( 1, sizeof( mbedtls_gcm_context ) ); 00081 00082 if( ctx != NULL ) 00083 mbedtls_gcm_init( (mbedtls_gcm_context *) ctx ); 00084 00085 return( ctx ); 00086 } 00087 00088 static void gcm_ctx_free( void *ctx ) 00089 { 00090 mbedtls_gcm_free( ctx ); 00091 mbedtls_free( ctx ); 00092 } 00093 #endif /* MBEDTLS_GCM_C */ 00094 00095 #if defined(MBEDTLS_CCM_C) 00096 /* shared by all CCM ciphers */ 00097 static void *ccm_ctx_alloc( void ) 00098 { 00099 void *ctx = mbedtls_calloc( 1, sizeof( mbedtls_ccm_context ) ); 00100 00101 if( ctx != NULL ) 00102 mbedtls_ccm_init( (mbedtls_ccm_context *) ctx ); 00103 00104 return( ctx ); 00105 } 00106 00107 static void ccm_ctx_free( void *ctx ) 00108 { 00109 mbedtls_ccm_free( ctx ); 00110 mbedtls_free( ctx ); 00111 } 00112 #endif /* MBEDTLS_CCM_C */ 00113 00114 #if defined(MBEDTLS_AES_C) 00115 00116 static int aes_crypt_ecb_wrap( void *ctx, mbedtls_operation_t operation, 00117 const unsigned char *input, unsigned char *output ) 00118 { 00119 return mbedtls_aes_crypt_ecb( (mbedtls_aes_context *) ctx, operation, input, output ); 00120 } 00121 00122 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00123 static int aes_crypt_cbc_wrap( void *ctx, mbedtls_operation_t operation, size_t length, 00124 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00125 { 00126 return mbedtls_aes_crypt_cbc( (mbedtls_aes_context *) ctx, operation, length, iv, input, 00127 output ); 00128 } 00129 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00130 00131 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00132 static int aes_crypt_cfb128_wrap( void *ctx, mbedtls_operation_t operation, 00133 size_t length, size_t *iv_off, unsigned char *iv, 00134 const unsigned char *input, unsigned char *output ) 00135 { 00136 return mbedtls_aes_crypt_cfb128( (mbedtls_aes_context *) ctx, operation, length, iv_off, iv, 00137 input, output ); 00138 } 00139 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00140 00141 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00142 static int aes_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00143 unsigned char *nonce_counter, unsigned char *stream_block, 00144 const unsigned char *input, unsigned char *output ) 00145 { 00146 return mbedtls_aes_crypt_ctr( (mbedtls_aes_context *) ctx, length, nc_off, nonce_counter, 00147 stream_block, input, output ); 00148 } 00149 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00150 00151 static int aes_setkey_dec_wrap( void *ctx, const unsigned char *key, 00152 unsigned int key_bitlen ) 00153 { 00154 return mbedtls_aes_setkey_dec( (mbedtls_aes_context *) ctx, key, key_bitlen ); 00155 } 00156 00157 static int aes_setkey_enc_wrap( void *ctx, const unsigned char *key, 00158 unsigned int key_bitlen ) 00159 { 00160 return mbedtls_aes_setkey_enc( (mbedtls_aes_context *) ctx, key, key_bitlen ); 00161 } 00162 00163 static void * aes_ctx_alloc( void ) 00164 { 00165 mbedtls_aes_context *aes = mbedtls_calloc( 1, sizeof( mbedtls_aes_context ) ); 00166 00167 if( aes == NULL ) 00168 return( NULL ); 00169 00170 mbedtls_aes_init( aes ); 00171 00172 return( aes ); 00173 } 00174 00175 static void aes_ctx_free( void *ctx ) 00176 { 00177 mbedtls_aes_free( (mbedtls_aes_context *) ctx ); 00178 mbedtls_free( ctx ); 00179 } 00180 00181 static const mbedtls_cipher_base_t aes_info = { 00182 MBEDTLS_CIPHER_ID_AES, 00183 aes_crypt_ecb_wrap, 00184 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00185 aes_crypt_cbc_wrap, 00186 #endif 00187 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00188 aes_crypt_cfb128_wrap, 00189 #endif 00190 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00191 aes_crypt_ctr_wrap, 00192 #endif 00193 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00194 NULL, 00195 #endif 00196 aes_setkey_enc_wrap, 00197 aes_setkey_dec_wrap, 00198 aes_ctx_alloc, 00199 aes_ctx_free 00200 }; 00201 00202 static const mbedtls_cipher_info_t aes_128_ecb_info = { 00203 MBEDTLS_CIPHER_AES_128_ECB, 00204 MBEDTLS_MODE_ECB, 00205 128, 00206 "AES-128-ECB", 00207 16, 00208 0, 00209 16, 00210 &aes_info 00211 }; 00212 00213 static const mbedtls_cipher_info_t aes_192_ecb_info = { 00214 MBEDTLS_CIPHER_AES_192_ECB, 00215 MBEDTLS_MODE_ECB, 00216 192, 00217 "AES-192-ECB", 00218 16, 00219 0, 00220 16, 00221 &aes_info 00222 }; 00223 00224 static const mbedtls_cipher_info_t aes_256_ecb_info = { 00225 MBEDTLS_CIPHER_AES_256_ECB, 00226 MBEDTLS_MODE_ECB, 00227 256, 00228 "AES-256-ECB", 00229 16, 00230 0, 00231 16, 00232 &aes_info 00233 }; 00234 00235 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00236 static const mbedtls_cipher_info_t aes_128_cbc_info = { 00237 MBEDTLS_CIPHER_AES_128_CBC, 00238 MBEDTLS_MODE_CBC, 00239 128, 00240 "AES-128-CBC", 00241 16, 00242 0, 00243 16, 00244 &aes_info 00245 }; 00246 00247 static const mbedtls_cipher_info_t aes_192_cbc_info = { 00248 MBEDTLS_CIPHER_AES_192_CBC, 00249 MBEDTLS_MODE_CBC, 00250 192, 00251 "AES-192-CBC", 00252 16, 00253 0, 00254 16, 00255 &aes_info 00256 }; 00257 00258 static const mbedtls_cipher_info_t aes_256_cbc_info = { 00259 MBEDTLS_CIPHER_AES_256_CBC, 00260 MBEDTLS_MODE_CBC, 00261 256, 00262 "AES-256-CBC", 00263 16, 00264 0, 00265 16, 00266 &aes_info 00267 }; 00268 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00269 00270 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00271 static const mbedtls_cipher_info_t aes_128_cfb128_info = { 00272 MBEDTLS_CIPHER_AES_128_CFB128, 00273 MBEDTLS_MODE_CFB, 00274 128, 00275 "AES-128-CFB128", 00276 16, 00277 0, 00278 16, 00279 &aes_info 00280 }; 00281 00282 static const mbedtls_cipher_info_t aes_192_cfb128_info = { 00283 MBEDTLS_CIPHER_AES_192_CFB128, 00284 MBEDTLS_MODE_CFB, 00285 192, 00286 "AES-192-CFB128", 00287 16, 00288 0, 00289 16, 00290 &aes_info 00291 }; 00292 00293 static const mbedtls_cipher_info_t aes_256_cfb128_info = { 00294 MBEDTLS_CIPHER_AES_256_CFB128, 00295 MBEDTLS_MODE_CFB, 00296 256, 00297 "AES-256-CFB128", 00298 16, 00299 0, 00300 16, 00301 &aes_info 00302 }; 00303 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00304 00305 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00306 static const mbedtls_cipher_info_t aes_128_ctr_info = { 00307 MBEDTLS_CIPHER_AES_128_CTR, 00308 MBEDTLS_MODE_CTR, 00309 128, 00310 "AES-128-CTR", 00311 16, 00312 0, 00313 16, 00314 &aes_info 00315 }; 00316 00317 static const mbedtls_cipher_info_t aes_192_ctr_info = { 00318 MBEDTLS_CIPHER_AES_192_CTR, 00319 MBEDTLS_MODE_CTR, 00320 192, 00321 "AES-192-CTR", 00322 16, 00323 0, 00324 16, 00325 &aes_info 00326 }; 00327 00328 static const mbedtls_cipher_info_t aes_256_ctr_info = { 00329 MBEDTLS_CIPHER_AES_256_CTR, 00330 MBEDTLS_MODE_CTR, 00331 256, 00332 "AES-256-CTR", 00333 16, 00334 0, 00335 16, 00336 &aes_info 00337 }; 00338 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00339 00340 #if defined(MBEDTLS_GCM_C) 00341 static int gcm_aes_setkey_wrap( void *ctx, const unsigned char *key, 00342 unsigned int key_bitlen ) 00343 { 00344 return mbedtls_gcm_setkey( (mbedtls_gcm_context *) ctx, MBEDTLS_CIPHER_ID_AES, 00345 key, key_bitlen ); 00346 } 00347 00348 static const mbedtls_cipher_base_t gcm_aes_info = { 00349 MBEDTLS_CIPHER_ID_AES, 00350 NULL, 00351 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00352 NULL, 00353 #endif 00354 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00355 NULL, 00356 #endif 00357 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00358 NULL, 00359 #endif 00360 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00361 NULL, 00362 #endif 00363 gcm_aes_setkey_wrap, 00364 gcm_aes_setkey_wrap, 00365 gcm_ctx_alloc, 00366 gcm_ctx_free, 00367 }; 00368 00369 static const mbedtls_cipher_info_t aes_128_gcm_info = { 00370 MBEDTLS_CIPHER_AES_128_GCM, 00371 MBEDTLS_MODE_GCM, 00372 128, 00373 "AES-128-GCM", 00374 12, 00375 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00376 16, 00377 &gcm_aes_info 00378 }; 00379 00380 static const mbedtls_cipher_info_t aes_192_gcm_info = { 00381 MBEDTLS_CIPHER_AES_192_GCM, 00382 MBEDTLS_MODE_GCM, 00383 192, 00384 "AES-192-GCM", 00385 12, 00386 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00387 16, 00388 &gcm_aes_info 00389 }; 00390 00391 static const mbedtls_cipher_info_t aes_256_gcm_info = { 00392 MBEDTLS_CIPHER_AES_256_GCM, 00393 MBEDTLS_MODE_GCM, 00394 256, 00395 "AES-256-GCM", 00396 12, 00397 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00398 16, 00399 &gcm_aes_info 00400 }; 00401 #endif /* MBEDTLS_GCM_C */ 00402 00403 #if defined(MBEDTLS_CCM_C) 00404 static int ccm_aes_setkey_wrap( void *ctx, const unsigned char *key, 00405 unsigned int key_bitlen ) 00406 { 00407 return mbedtls_ccm_setkey( (mbedtls_ccm_context *) ctx, MBEDTLS_CIPHER_ID_AES, 00408 key, key_bitlen ); 00409 } 00410 00411 static const mbedtls_cipher_base_t ccm_aes_info = { 00412 MBEDTLS_CIPHER_ID_AES, 00413 NULL, 00414 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00415 NULL, 00416 #endif 00417 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00418 NULL, 00419 #endif 00420 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00421 NULL, 00422 #endif 00423 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00424 NULL, 00425 #endif 00426 ccm_aes_setkey_wrap, 00427 ccm_aes_setkey_wrap, 00428 ccm_ctx_alloc, 00429 ccm_ctx_free, 00430 }; 00431 00432 static const mbedtls_cipher_info_t aes_128_ccm_info = { 00433 MBEDTLS_CIPHER_AES_128_CCM, 00434 MBEDTLS_MODE_CCM, 00435 128, 00436 "AES-128-CCM", 00437 12, 00438 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00439 16, 00440 &ccm_aes_info 00441 }; 00442 00443 static const mbedtls_cipher_info_t aes_192_ccm_info = { 00444 MBEDTLS_CIPHER_AES_192_CCM, 00445 MBEDTLS_MODE_CCM, 00446 192, 00447 "AES-192-CCM", 00448 12, 00449 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00450 16, 00451 &ccm_aes_info 00452 }; 00453 00454 static const mbedtls_cipher_info_t aes_256_ccm_info = { 00455 MBEDTLS_CIPHER_AES_256_CCM, 00456 MBEDTLS_MODE_CCM, 00457 256, 00458 "AES-256-CCM", 00459 12, 00460 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00461 16, 00462 &ccm_aes_info 00463 }; 00464 #endif /* MBEDTLS_CCM_C */ 00465 00466 #endif /* MBEDTLS_AES_C */ 00467 00468 #if defined(MBEDTLS_CAMELLIA_C) 00469 00470 static int camellia_crypt_ecb_wrap( void *ctx, mbedtls_operation_t operation, 00471 const unsigned char *input, unsigned char *output ) 00472 { 00473 return mbedtls_camellia_crypt_ecb( (mbedtls_camellia_context *) ctx, operation, input, 00474 output ); 00475 } 00476 00477 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00478 static int camellia_crypt_cbc_wrap( void *ctx, mbedtls_operation_t operation, 00479 size_t length, unsigned char *iv, 00480 const unsigned char *input, unsigned char *output ) 00481 { 00482 return mbedtls_camellia_crypt_cbc( (mbedtls_camellia_context *) ctx, operation, length, iv, 00483 input, output ); 00484 } 00485 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00486 00487 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00488 static int camellia_crypt_cfb128_wrap( void *ctx, mbedtls_operation_t operation, 00489 size_t length, size_t *iv_off, unsigned char *iv, 00490 const unsigned char *input, unsigned char *output ) 00491 { 00492 return mbedtls_camellia_crypt_cfb128( (mbedtls_camellia_context *) ctx, operation, length, 00493 iv_off, iv, input, output ); 00494 } 00495 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00496 00497 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00498 static int camellia_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 00499 unsigned char *nonce_counter, unsigned char *stream_block, 00500 const unsigned char *input, unsigned char *output ) 00501 { 00502 return mbedtls_camellia_crypt_ctr( (mbedtls_camellia_context *) ctx, length, nc_off, 00503 nonce_counter, stream_block, input, output ); 00504 } 00505 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00506 00507 static int camellia_setkey_dec_wrap( void *ctx, const unsigned char *key, 00508 unsigned int key_bitlen ) 00509 { 00510 return mbedtls_camellia_setkey_dec( (mbedtls_camellia_context *) ctx, key, key_bitlen ); 00511 } 00512 00513 static int camellia_setkey_enc_wrap( void *ctx, const unsigned char *key, 00514 unsigned int key_bitlen ) 00515 { 00516 return mbedtls_camellia_setkey_enc( (mbedtls_camellia_context *) ctx, key, key_bitlen ); 00517 } 00518 00519 static void * camellia_ctx_alloc( void ) 00520 { 00521 mbedtls_camellia_context *ctx; 00522 ctx = mbedtls_calloc( 1, sizeof( mbedtls_camellia_context ) ); 00523 00524 if( ctx == NULL ) 00525 return( NULL ); 00526 00527 mbedtls_camellia_init( ctx ); 00528 00529 return( ctx ); 00530 } 00531 00532 static void camellia_ctx_free( void *ctx ) 00533 { 00534 mbedtls_camellia_free( (mbedtls_camellia_context *) ctx ); 00535 mbedtls_free( ctx ); 00536 } 00537 00538 static const mbedtls_cipher_base_t camellia_info = { 00539 MBEDTLS_CIPHER_ID_CAMELLIA, 00540 camellia_crypt_ecb_wrap, 00541 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00542 camellia_crypt_cbc_wrap, 00543 #endif 00544 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00545 camellia_crypt_cfb128_wrap, 00546 #endif 00547 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00548 camellia_crypt_ctr_wrap, 00549 #endif 00550 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00551 NULL, 00552 #endif 00553 camellia_setkey_enc_wrap, 00554 camellia_setkey_dec_wrap, 00555 camellia_ctx_alloc, 00556 camellia_ctx_free 00557 }; 00558 00559 static const mbedtls_cipher_info_t camellia_128_ecb_info = { 00560 MBEDTLS_CIPHER_CAMELLIA_128_ECB, 00561 MBEDTLS_MODE_ECB, 00562 128, 00563 "CAMELLIA-128-ECB", 00564 16, 00565 0, 00566 16, 00567 &camellia_info 00568 }; 00569 00570 static const mbedtls_cipher_info_t camellia_192_ecb_info = { 00571 MBEDTLS_CIPHER_CAMELLIA_192_ECB, 00572 MBEDTLS_MODE_ECB, 00573 192, 00574 "CAMELLIA-192-ECB", 00575 16, 00576 0, 00577 16, 00578 &camellia_info 00579 }; 00580 00581 static const mbedtls_cipher_info_t camellia_256_ecb_info = { 00582 MBEDTLS_CIPHER_CAMELLIA_256_ECB, 00583 MBEDTLS_MODE_ECB, 00584 256, 00585 "CAMELLIA-256-ECB", 00586 16, 00587 0, 00588 16, 00589 &camellia_info 00590 }; 00591 00592 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00593 static const mbedtls_cipher_info_t camellia_128_cbc_info = { 00594 MBEDTLS_CIPHER_CAMELLIA_128_CBC, 00595 MBEDTLS_MODE_CBC, 00596 128, 00597 "CAMELLIA-128-CBC", 00598 16, 00599 0, 00600 16, 00601 &camellia_info 00602 }; 00603 00604 static const mbedtls_cipher_info_t camellia_192_cbc_info = { 00605 MBEDTLS_CIPHER_CAMELLIA_192_CBC, 00606 MBEDTLS_MODE_CBC, 00607 192, 00608 "CAMELLIA-192-CBC", 00609 16, 00610 0, 00611 16, 00612 &camellia_info 00613 }; 00614 00615 static const mbedtls_cipher_info_t camellia_256_cbc_info = { 00616 MBEDTLS_CIPHER_CAMELLIA_256_CBC, 00617 MBEDTLS_MODE_CBC, 00618 256, 00619 "CAMELLIA-256-CBC", 00620 16, 00621 0, 00622 16, 00623 &camellia_info 00624 }; 00625 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00626 00627 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00628 static const mbedtls_cipher_info_t camellia_128_cfb128_info = { 00629 MBEDTLS_CIPHER_CAMELLIA_128_CFB128, 00630 MBEDTLS_MODE_CFB, 00631 128, 00632 "CAMELLIA-128-CFB128", 00633 16, 00634 0, 00635 16, 00636 &camellia_info 00637 }; 00638 00639 static const mbedtls_cipher_info_t camellia_192_cfb128_info = { 00640 MBEDTLS_CIPHER_CAMELLIA_192_CFB128, 00641 MBEDTLS_MODE_CFB, 00642 192, 00643 "CAMELLIA-192-CFB128", 00644 16, 00645 0, 00646 16, 00647 &camellia_info 00648 }; 00649 00650 static const mbedtls_cipher_info_t camellia_256_cfb128_info = { 00651 MBEDTLS_CIPHER_CAMELLIA_256_CFB128, 00652 MBEDTLS_MODE_CFB, 00653 256, 00654 "CAMELLIA-256-CFB128", 00655 16, 00656 0, 00657 16, 00658 &camellia_info 00659 }; 00660 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 00661 00662 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00663 static const mbedtls_cipher_info_t camellia_128_ctr_info = { 00664 MBEDTLS_CIPHER_CAMELLIA_128_CTR, 00665 MBEDTLS_MODE_CTR, 00666 128, 00667 "CAMELLIA-128-CTR", 00668 16, 00669 0, 00670 16, 00671 &camellia_info 00672 }; 00673 00674 static const mbedtls_cipher_info_t camellia_192_ctr_info = { 00675 MBEDTLS_CIPHER_CAMELLIA_192_CTR, 00676 MBEDTLS_MODE_CTR, 00677 192, 00678 "CAMELLIA-192-CTR", 00679 16, 00680 0, 00681 16, 00682 &camellia_info 00683 }; 00684 00685 static const mbedtls_cipher_info_t camellia_256_ctr_info = { 00686 MBEDTLS_CIPHER_CAMELLIA_256_CTR, 00687 MBEDTLS_MODE_CTR, 00688 256, 00689 "CAMELLIA-256-CTR", 00690 16, 00691 0, 00692 16, 00693 &camellia_info 00694 }; 00695 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 00696 00697 #if defined(MBEDTLS_GCM_C) 00698 static int gcm_camellia_setkey_wrap( void *ctx, const unsigned char *key, 00699 unsigned int key_bitlen ) 00700 { 00701 return mbedtls_gcm_setkey( (mbedtls_gcm_context *) ctx, MBEDTLS_CIPHER_ID_CAMELLIA, 00702 key, key_bitlen ); 00703 } 00704 00705 static const mbedtls_cipher_base_t gcm_camellia_info = { 00706 MBEDTLS_CIPHER_ID_CAMELLIA, 00707 NULL, 00708 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00709 NULL, 00710 #endif 00711 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00712 NULL, 00713 #endif 00714 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00715 NULL, 00716 #endif 00717 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00718 NULL, 00719 #endif 00720 gcm_camellia_setkey_wrap, 00721 gcm_camellia_setkey_wrap, 00722 gcm_ctx_alloc, 00723 gcm_ctx_free, 00724 }; 00725 00726 static const mbedtls_cipher_info_t camellia_128_gcm_info = { 00727 MBEDTLS_CIPHER_CAMELLIA_128_GCM, 00728 MBEDTLS_MODE_GCM, 00729 128, 00730 "CAMELLIA-128-GCM", 00731 12, 00732 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00733 16, 00734 &gcm_camellia_info 00735 }; 00736 00737 static const mbedtls_cipher_info_t camellia_192_gcm_info = { 00738 MBEDTLS_CIPHER_CAMELLIA_192_GCM, 00739 MBEDTLS_MODE_GCM, 00740 192, 00741 "CAMELLIA-192-GCM", 00742 12, 00743 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00744 16, 00745 &gcm_camellia_info 00746 }; 00747 00748 static const mbedtls_cipher_info_t camellia_256_gcm_info = { 00749 MBEDTLS_CIPHER_CAMELLIA_256_GCM, 00750 MBEDTLS_MODE_GCM, 00751 256, 00752 "CAMELLIA-256-GCM", 00753 12, 00754 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00755 16, 00756 &gcm_camellia_info 00757 }; 00758 #endif /* MBEDTLS_GCM_C */ 00759 00760 #if defined(MBEDTLS_CCM_C) 00761 static int ccm_camellia_setkey_wrap( void *ctx, const unsigned char *key, 00762 unsigned int key_bitlen ) 00763 { 00764 return mbedtls_ccm_setkey( (mbedtls_ccm_context *) ctx, MBEDTLS_CIPHER_ID_CAMELLIA, 00765 key, key_bitlen ); 00766 } 00767 00768 static const mbedtls_cipher_base_t ccm_camellia_info = { 00769 MBEDTLS_CIPHER_ID_CAMELLIA, 00770 NULL, 00771 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00772 NULL, 00773 #endif 00774 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00775 NULL, 00776 #endif 00777 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00778 NULL, 00779 #endif 00780 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00781 NULL, 00782 #endif 00783 ccm_camellia_setkey_wrap, 00784 ccm_camellia_setkey_wrap, 00785 ccm_ctx_alloc, 00786 ccm_ctx_free, 00787 }; 00788 00789 static const mbedtls_cipher_info_t camellia_128_ccm_info = { 00790 MBEDTLS_CIPHER_CAMELLIA_128_CCM, 00791 MBEDTLS_MODE_CCM, 00792 128, 00793 "CAMELLIA-128-CCM", 00794 12, 00795 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00796 16, 00797 &ccm_camellia_info 00798 }; 00799 00800 static const mbedtls_cipher_info_t camellia_192_ccm_info = { 00801 MBEDTLS_CIPHER_CAMELLIA_192_CCM, 00802 MBEDTLS_MODE_CCM, 00803 192, 00804 "CAMELLIA-192-CCM", 00805 12, 00806 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00807 16, 00808 &ccm_camellia_info 00809 }; 00810 00811 static const mbedtls_cipher_info_t camellia_256_ccm_info = { 00812 MBEDTLS_CIPHER_CAMELLIA_256_CCM, 00813 MBEDTLS_MODE_CCM, 00814 256, 00815 "CAMELLIA-256-CCM", 00816 12, 00817 MBEDTLS_CIPHER_VARIABLE_IV_LEN, 00818 16, 00819 &ccm_camellia_info 00820 }; 00821 #endif /* MBEDTLS_CCM_C */ 00822 00823 #endif /* MBEDTLS_CAMELLIA_C */ 00824 00825 #if defined(MBEDTLS_DES_C) 00826 00827 static int des_crypt_ecb_wrap( void *ctx, mbedtls_operation_t operation, 00828 const unsigned char *input, unsigned char *output ) 00829 { 00830 ((void) operation); 00831 return mbedtls_des_crypt_ecb( (mbedtls_des_context *) ctx, input, output ); 00832 } 00833 00834 static int des3_crypt_ecb_wrap( void *ctx, mbedtls_operation_t operation, 00835 const unsigned char *input, unsigned char *output ) 00836 { 00837 ((void) operation); 00838 return mbedtls_des3_crypt_ecb( (mbedtls_des3_context *) ctx, input, output ); 00839 } 00840 00841 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00842 static int des_crypt_cbc_wrap( void *ctx, mbedtls_operation_t operation, size_t length, 00843 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00844 { 00845 return mbedtls_des_crypt_cbc( (mbedtls_des_context *) ctx, operation, length, iv, input, 00846 output ); 00847 } 00848 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00849 00850 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00851 static int des3_crypt_cbc_wrap( void *ctx, mbedtls_operation_t operation, size_t length, 00852 unsigned char *iv, const unsigned char *input, unsigned char *output ) 00853 { 00854 return mbedtls_des3_crypt_cbc( (mbedtls_des3_context *) ctx, operation, length, iv, input, 00855 output ); 00856 } 00857 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00858 00859 static int des_setkey_dec_wrap( void *ctx, const unsigned char *key, 00860 unsigned int key_bitlen ) 00861 { 00862 ((void) key_bitlen); 00863 00864 return mbedtls_des_setkey_dec( (mbedtls_des_context *) ctx, key ); 00865 } 00866 00867 static int des_setkey_enc_wrap( void *ctx, const unsigned char *key, 00868 unsigned int key_bitlen ) 00869 { 00870 ((void) key_bitlen); 00871 00872 return mbedtls_des_setkey_enc( (mbedtls_des_context *) ctx, key ); 00873 } 00874 00875 static int des3_set2key_dec_wrap( void *ctx, const unsigned char *key, 00876 unsigned int key_bitlen ) 00877 { 00878 ((void) key_bitlen); 00879 00880 return mbedtls_des3_set2key_dec( (mbedtls_des3_context *) ctx, key ); 00881 } 00882 00883 static int des3_set2key_enc_wrap( void *ctx, const unsigned char *key, 00884 unsigned int key_bitlen ) 00885 { 00886 ((void) key_bitlen); 00887 00888 return mbedtls_des3_set2key_enc( (mbedtls_des3_context *) ctx, key ); 00889 } 00890 00891 static int des3_set3key_dec_wrap( void *ctx, const unsigned char *key, 00892 unsigned int key_bitlen ) 00893 { 00894 ((void) key_bitlen); 00895 00896 return mbedtls_des3_set3key_dec( (mbedtls_des3_context *) ctx, key ); 00897 } 00898 00899 static int des3_set3key_enc_wrap( void *ctx, const unsigned char *key, 00900 unsigned int key_bitlen ) 00901 { 00902 ((void) key_bitlen); 00903 00904 return mbedtls_des3_set3key_enc( (mbedtls_des3_context *) ctx, key ); 00905 } 00906 00907 static void * des_ctx_alloc( void ) 00908 { 00909 mbedtls_des_context *des = mbedtls_calloc( 1, sizeof( mbedtls_des_context ) ); 00910 00911 if( des == NULL ) 00912 return( NULL ); 00913 00914 mbedtls_des_init( des ); 00915 00916 return( des ); 00917 } 00918 00919 static void des_ctx_free( void *ctx ) 00920 { 00921 mbedtls_des_free( (mbedtls_des_context *) ctx ); 00922 mbedtls_free( ctx ); 00923 } 00924 00925 static void * des3_ctx_alloc( void ) 00926 { 00927 mbedtls_des3_context *des3; 00928 des3 = mbedtls_calloc( 1, sizeof( mbedtls_des3_context ) ); 00929 00930 if( des3 == NULL ) 00931 return( NULL ); 00932 00933 mbedtls_des3_init( des3 ); 00934 00935 return( des3 ); 00936 } 00937 00938 static void des3_ctx_free( void *ctx ) 00939 { 00940 mbedtls_des3_free( (mbedtls_des3_context *) ctx ); 00941 mbedtls_free( ctx ); 00942 } 00943 00944 static const mbedtls_cipher_base_t des_info = { 00945 MBEDTLS_CIPHER_ID_DES, 00946 des_crypt_ecb_wrap, 00947 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00948 des_crypt_cbc_wrap, 00949 #endif 00950 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00951 NULL, 00952 #endif 00953 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00954 NULL, 00955 #endif 00956 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00957 NULL, 00958 #endif 00959 des_setkey_enc_wrap, 00960 des_setkey_dec_wrap, 00961 des_ctx_alloc, 00962 des_ctx_free 00963 }; 00964 00965 static const mbedtls_cipher_info_t des_ecb_info = { 00966 MBEDTLS_CIPHER_DES_ECB, 00967 MBEDTLS_MODE_ECB, 00968 MBEDTLS_KEY_LENGTH_DES, 00969 "DES-ECB", 00970 8, 00971 0, 00972 8, 00973 &des_info 00974 }; 00975 00976 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00977 static const mbedtls_cipher_info_t des_cbc_info = { 00978 MBEDTLS_CIPHER_DES_CBC, 00979 MBEDTLS_MODE_CBC, 00980 MBEDTLS_KEY_LENGTH_DES, 00981 "DES-CBC", 00982 8, 00983 0, 00984 8, 00985 &des_info 00986 }; 00987 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 00988 00989 static const mbedtls_cipher_base_t des_ede_info = { 00990 MBEDTLS_CIPHER_ID_DES, 00991 des3_crypt_ecb_wrap, 00992 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00993 des3_crypt_cbc_wrap, 00994 #endif 00995 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00996 NULL, 00997 #endif 00998 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00999 NULL, 01000 #endif 01001 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 01002 NULL, 01003 #endif 01004 des3_set2key_enc_wrap, 01005 des3_set2key_dec_wrap, 01006 des3_ctx_alloc, 01007 des3_ctx_free 01008 }; 01009 01010 static const mbedtls_cipher_info_t des_ede_ecb_info = { 01011 MBEDTLS_CIPHER_DES_EDE_ECB, 01012 MBEDTLS_MODE_ECB, 01013 MBEDTLS_KEY_LENGTH_DES_EDE, 01014 "DES-EDE-ECB", 01015 8, 01016 0, 01017 8, 01018 &des_ede_info 01019 }; 01020 01021 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01022 static const mbedtls_cipher_info_t des_ede_cbc_info = { 01023 MBEDTLS_CIPHER_DES_EDE_CBC, 01024 MBEDTLS_MODE_CBC, 01025 MBEDTLS_KEY_LENGTH_DES_EDE, 01026 "DES-EDE-CBC", 01027 8, 01028 0, 01029 8, 01030 &des_ede_info 01031 }; 01032 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01033 01034 static const mbedtls_cipher_base_t des_ede3_info = { 01035 MBEDTLS_CIPHER_ID_3DES, 01036 des3_crypt_ecb_wrap, 01037 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01038 des3_crypt_cbc_wrap, 01039 #endif 01040 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01041 NULL, 01042 #endif 01043 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01044 NULL, 01045 #endif 01046 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 01047 NULL, 01048 #endif 01049 des3_set3key_enc_wrap, 01050 des3_set3key_dec_wrap, 01051 des3_ctx_alloc, 01052 des3_ctx_free 01053 }; 01054 01055 static const mbedtls_cipher_info_t des_ede3_ecb_info = { 01056 MBEDTLS_CIPHER_DES_EDE3_ECB, 01057 MBEDTLS_MODE_ECB, 01058 MBEDTLS_KEY_LENGTH_DES_EDE3, 01059 "DES-EDE3-ECB", 01060 8, 01061 0, 01062 8, 01063 &des_ede3_info 01064 }; 01065 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01066 static const mbedtls_cipher_info_t des_ede3_cbc_info = { 01067 MBEDTLS_CIPHER_DES_EDE3_CBC, 01068 MBEDTLS_MODE_CBC, 01069 MBEDTLS_KEY_LENGTH_DES_EDE3, 01070 "DES-EDE3-CBC", 01071 8, 01072 0, 01073 8, 01074 &des_ede3_info 01075 }; 01076 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01077 #endif /* MBEDTLS_DES_C */ 01078 01079 #if defined(MBEDTLS_BLOWFISH_C) 01080 01081 static int blowfish_crypt_ecb_wrap( void *ctx, mbedtls_operation_t operation, 01082 const unsigned char *input, unsigned char *output ) 01083 { 01084 return mbedtls_blowfish_crypt_ecb( (mbedtls_blowfish_context *) ctx, operation, input, 01085 output ); 01086 } 01087 01088 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01089 static int blowfish_crypt_cbc_wrap( void *ctx, mbedtls_operation_t operation, 01090 size_t length, unsigned char *iv, const unsigned char *input, 01091 unsigned char *output ) 01092 { 01093 return mbedtls_blowfish_crypt_cbc( (mbedtls_blowfish_context *) ctx, operation, length, iv, 01094 input, output ); 01095 } 01096 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01097 01098 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01099 static int blowfish_crypt_cfb64_wrap( void *ctx, mbedtls_operation_t operation, 01100 size_t length, size_t *iv_off, unsigned char *iv, 01101 const unsigned char *input, unsigned char *output ) 01102 { 01103 return mbedtls_blowfish_crypt_cfb64( (mbedtls_blowfish_context *) ctx, operation, length, 01104 iv_off, iv, input, output ); 01105 } 01106 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 01107 01108 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01109 static int blowfish_crypt_ctr_wrap( void *ctx, size_t length, size_t *nc_off, 01110 unsigned char *nonce_counter, unsigned char *stream_block, 01111 const unsigned char *input, unsigned char *output ) 01112 { 01113 return mbedtls_blowfish_crypt_ctr( (mbedtls_blowfish_context *) ctx, length, nc_off, 01114 nonce_counter, stream_block, input, output ); 01115 } 01116 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01117 01118 static int blowfish_setkey_wrap( void *ctx, const unsigned char *key, 01119 unsigned int key_bitlen ) 01120 { 01121 return mbedtls_blowfish_setkey( (mbedtls_blowfish_context *) ctx, key, key_bitlen ); 01122 } 01123 01124 static void * blowfish_ctx_alloc( void ) 01125 { 01126 mbedtls_blowfish_context *ctx; 01127 ctx = mbedtls_calloc( 1, sizeof( mbedtls_blowfish_context ) ); 01128 01129 if( ctx == NULL ) 01130 return( NULL ); 01131 01132 mbedtls_blowfish_init( ctx ); 01133 01134 return( ctx ); 01135 } 01136 01137 static void blowfish_ctx_free( void *ctx ) 01138 { 01139 mbedtls_blowfish_free( (mbedtls_blowfish_context *) ctx ); 01140 mbedtls_free( ctx ); 01141 } 01142 01143 static const mbedtls_cipher_base_t blowfish_info = { 01144 MBEDTLS_CIPHER_ID_BLOWFISH, 01145 blowfish_crypt_ecb_wrap, 01146 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01147 blowfish_crypt_cbc_wrap, 01148 #endif 01149 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01150 blowfish_crypt_cfb64_wrap, 01151 #endif 01152 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01153 blowfish_crypt_ctr_wrap, 01154 #endif 01155 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 01156 NULL, 01157 #endif 01158 blowfish_setkey_wrap, 01159 blowfish_setkey_wrap, 01160 blowfish_ctx_alloc, 01161 blowfish_ctx_free 01162 }; 01163 01164 static const mbedtls_cipher_info_t blowfish_ecb_info = { 01165 MBEDTLS_CIPHER_BLOWFISH_ECB, 01166 MBEDTLS_MODE_ECB, 01167 128, 01168 "BLOWFISH-ECB", 01169 8, 01170 MBEDTLS_CIPHER_VARIABLE_KEY_LEN, 01171 8, 01172 &blowfish_info 01173 }; 01174 01175 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01176 static const mbedtls_cipher_info_t blowfish_cbc_info = { 01177 MBEDTLS_CIPHER_BLOWFISH_CBC, 01178 MBEDTLS_MODE_CBC, 01179 128, 01180 "BLOWFISH-CBC", 01181 8, 01182 MBEDTLS_CIPHER_VARIABLE_KEY_LEN, 01183 8, 01184 &blowfish_info 01185 }; 01186 #endif /* MBEDTLS_CIPHER_MODE_CBC */ 01187 01188 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01189 static const mbedtls_cipher_info_t blowfish_cfb64_info = { 01190 MBEDTLS_CIPHER_BLOWFISH_CFB64, 01191 MBEDTLS_MODE_CFB, 01192 128, 01193 "BLOWFISH-CFB64", 01194 8, 01195 MBEDTLS_CIPHER_VARIABLE_KEY_LEN, 01196 8, 01197 &blowfish_info 01198 }; 01199 #endif /* MBEDTLS_CIPHER_MODE_CFB */ 01200 01201 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01202 static const mbedtls_cipher_info_t blowfish_ctr_info = { 01203 MBEDTLS_CIPHER_BLOWFISH_CTR, 01204 MBEDTLS_MODE_CTR, 01205 128, 01206 "BLOWFISH-CTR", 01207 8, 01208 MBEDTLS_CIPHER_VARIABLE_KEY_LEN, 01209 8, 01210 &blowfish_info 01211 }; 01212 #endif /* MBEDTLS_CIPHER_MODE_CTR */ 01213 #endif /* MBEDTLS_BLOWFISH_C */ 01214 01215 #if defined(MBEDTLS_ARC4_C) 01216 static int arc4_crypt_stream_wrap( void *ctx, size_t length, 01217 const unsigned char *input, 01218 unsigned char *output ) 01219 { 01220 return( mbedtls_arc4_crypt( (mbedtls_arc4_context *) ctx, length, input, output ) ); 01221 } 01222 01223 static int arc4_setkey_wrap( void *ctx, const unsigned char *key, 01224 unsigned int key_bitlen ) 01225 { 01226 /* we get key_bitlen in bits, arc4 expects it in bytes */ 01227 if( key_bitlen % 8 != 0 ) 01228 return( MBEDTLS_ERR_CIPHER_BAD_INPUT_DATA ); 01229 01230 mbedtls_arc4_setup( (mbedtls_arc4_context *) ctx, key, key_bitlen / 8 ); 01231 return( 0 ); 01232 } 01233 01234 static void * arc4_ctx_alloc( void ) 01235 { 01236 mbedtls_arc4_context *ctx; 01237 ctx = mbedtls_calloc( 1, sizeof( mbedtls_arc4_context ) ); 01238 01239 if( ctx == NULL ) 01240 return( NULL ); 01241 01242 mbedtls_arc4_init( ctx ); 01243 01244 return( ctx ); 01245 } 01246 01247 static void arc4_ctx_free( void *ctx ) 01248 { 01249 mbedtls_arc4_free( (mbedtls_arc4_context *) ctx ); 01250 mbedtls_free( ctx ); 01251 } 01252 01253 static const mbedtls_cipher_base_t arc4_base_info = { 01254 MBEDTLS_CIPHER_ID_ARC4, 01255 NULL, 01256 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01257 NULL, 01258 #endif 01259 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01260 NULL, 01261 #endif 01262 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01263 NULL, 01264 #endif 01265 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 01266 arc4_crypt_stream_wrap, 01267 #endif 01268 arc4_setkey_wrap, 01269 arc4_setkey_wrap, 01270 arc4_ctx_alloc, 01271 arc4_ctx_free 01272 }; 01273 01274 static const mbedtls_cipher_info_t arc4_128_info = { 01275 MBEDTLS_CIPHER_ARC4_128, 01276 MBEDTLS_MODE_STREAM, 01277 128, 01278 "ARC4-128", 01279 0, 01280 0, 01281 1, 01282 &arc4_base_info 01283 }; 01284 #endif /* MBEDTLS_ARC4_C */ 01285 01286 #if defined(MBEDTLS_CIPHER_NULL_CIPHER) 01287 static int null_crypt_stream( void *ctx, size_t length, 01288 const unsigned char *input, 01289 unsigned char *output ) 01290 { 01291 ((void) ctx); 01292 memmove( output, input, length ); 01293 return( 0 ); 01294 } 01295 01296 static int null_setkey( void *ctx, const unsigned char *key, 01297 unsigned int key_bitlen ) 01298 { 01299 ((void) ctx); 01300 ((void) key); 01301 ((void) key_bitlen); 01302 01303 return( 0 ); 01304 } 01305 01306 static void * null_ctx_alloc( void ) 01307 { 01308 return( (void *) 1 ); 01309 } 01310 01311 static void null_ctx_free( void *ctx ) 01312 { 01313 ((void) ctx); 01314 } 01315 01316 static const mbedtls_cipher_base_t null_base_info = { 01317 MBEDTLS_CIPHER_ID_NULL, 01318 NULL, 01319 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01320 NULL, 01321 #endif 01322 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01323 NULL, 01324 #endif 01325 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01326 NULL, 01327 #endif 01328 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 01329 null_crypt_stream, 01330 #endif 01331 null_setkey, 01332 null_setkey, 01333 null_ctx_alloc, 01334 null_ctx_free 01335 }; 01336 01337 static const mbedtls_cipher_info_t null_cipher_info = { 01338 MBEDTLS_CIPHER_NULL, 01339 MBEDTLS_MODE_STREAM, 01340 0, 01341 "NULL", 01342 0, 01343 0, 01344 1, 01345 &null_base_info 01346 }; 01347 #endif /* defined(MBEDTLS_CIPHER_NULL_CIPHER) */ 01348 01349 const mbedtls_cipher_definition_t mbedtls_cipher_definitions[] = 01350 { 01351 #if defined(MBEDTLS_AES_C) 01352 { MBEDTLS_CIPHER_AES_128_ECB, &aes_128_ecb_info }, 01353 { MBEDTLS_CIPHER_AES_192_ECB, &aes_192_ecb_info }, 01354 { MBEDTLS_CIPHER_AES_256_ECB, &aes_256_ecb_info }, 01355 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01356 { MBEDTLS_CIPHER_AES_128_CBC, &aes_128_cbc_info }, 01357 { MBEDTLS_CIPHER_AES_192_CBC, &aes_192_cbc_info }, 01358 { MBEDTLS_CIPHER_AES_256_CBC, &aes_256_cbc_info }, 01359 #endif 01360 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01361 { MBEDTLS_CIPHER_AES_128_CFB128, &aes_128_cfb128_info }, 01362 { MBEDTLS_CIPHER_AES_192_CFB128, &aes_192_cfb128_info }, 01363 { MBEDTLS_CIPHER_AES_256_CFB128, &aes_256_cfb128_info }, 01364 #endif 01365 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01366 { MBEDTLS_CIPHER_AES_128_CTR, &aes_128_ctr_info }, 01367 { MBEDTLS_CIPHER_AES_192_CTR, &aes_192_ctr_info }, 01368 { MBEDTLS_CIPHER_AES_256_CTR, &aes_256_ctr_info }, 01369 #endif 01370 #if defined(MBEDTLS_GCM_C) 01371 { MBEDTLS_CIPHER_AES_128_GCM, &aes_128_gcm_info }, 01372 { MBEDTLS_CIPHER_AES_192_GCM, &aes_192_gcm_info }, 01373 { MBEDTLS_CIPHER_AES_256_GCM, &aes_256_gcm_info }, 01374 #endif 01375 #if defined(MBEDTLS_CCM_C) 01376 { MBEDTLS_CIPHER_AES_128_CCM, &aes_128_ccm_info }, 01377 { MBEDTLS_CIPHER_AES_192_CCM, &aes_192_ccm_info }, 01378 { MBEDTLS_CIPHER_AES_256_CCM, &aes_256_ccm_info }, 01379 #endif 01380 #endif /* MBEDTLS_AES_C */ 01381 01382 #if defined(MBEDTLS_ARC4_C) 01383 { MBEDTLS_CIPHER_ARC4_128, &arc4_128_info }, 01384 #endif 01385 01386 #if defined(MBEDTLS_BLOWFISH_C) 01387 { MBEDTLS_CIPHER_BLOWFISH_ECB, &blowfish_ecb_info }, 01388 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01389 { MBEDTLS_CIPHER_BLOWFISH_CBC, &blowfish_cbc_info }, 01390 #endif 01391 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01392 { MBEDTLS_CIPHER_BLOWFISH_CFB64, &blowfish_cfb64_info }, 01393 #endif 01394 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01395 { MBEDTLS_CIPHER_BLOWFISH_CTR, &blowfish_ctr_info }, 01396 #endif 01397 #endif /* MBEDTLS_BLOWFISH_C */ 01398 01399 #if defined(MBEDTLS_CAMELLIA_C) 01400 { MBEDTLS_CIPHER_CAMELLIA_128_ECB, &camellia_128_ecb_info }, 01401 { MBEDTLS_CIPHER_CAMELLIA_192_ECB, &camellia_192_ecb_info }, 01402 { MBEDTLS_CIPHER_CAMELLIA_256_ECB, &camellia_256_ecb_info }, 01403 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01404 { MBEDTLS_CIPHER_CAMELLIA_128_CBC, &camellia_128_cbc_info }, 01405 { MBEDTLS_CIPHER_CAMELLIA_192_CBC, &camellia_192_cbc_info }, 01406 { MBEDTLS_CIPHER_CAMELLIA_256_CBC, &camellia_256_cbc_info }, 01407 #endif 01408 #if defined(MBEDTLS_CIPHER_MODE_CFB) 01409 { MBEDTLS_CIPHER_CAMELLIA_128_CFB128, &camellia_128_cfb128_info }, 01410 { MBEDTLS_CIPHER_CAMELLIA_192_CFB128, &camellia_192_cfb128_info }, 01411 { MBEDTLS_CIPHER_CAMELLIA_256_CFB128, &camellia_256_cfb128_info }, 01412 #endif 01413 #if defined(MBEDTLS_CIPHER_MODE_CTR) 01414 { MBEDTLS_CIPHER_CAMELLIA_128_CTR, &camellia_128_ctr_info }, 01415 { MBEDTLS_CIPHER_CAMELLIA_192_CTR, &camellia_192_ctr_info }, 01416 { MBEDTLS_CIPHER_CAMELLIA_256_CTR, &camellia_256_ctr_info }, 01417 #endif 01418 #if defined(MBEDTLS_GCM_C) 01419 { MBEDTLS_CIPHER_CAMELLIA_128_GCM, &camellia_128_gcm_info }, 01420 { MBEDTLS_CIPHER_CAMELLIA_192_GCM, &camellia_192_gcm_info }, 01421 { MBEDTLS_CIPHER_CAMELLIA_256_GCM, &camellia_256_gcm_info }, 01422 #endif 01423 #if defined(MBEDTLS_CCM_C) 01424 { MBEDTLS_CIPHER_CAMELLIA_128_CCM, &camellia_128_ccm_info }, 01425 { MBEDTLS_CIPHER_CAMELLIA_192_CCM, &camellia_192_ccm_info }, 01426 { MBEDTLS_CIPHER_CAMELLIA_256_CCM, &camellia_256_ccm_info }, 01427 #endif 01428 #endif /* MBEDTLS_CAMELLIA_C */ 01429 01430 #if defined(MBEDTLS_DES_C) 01431 { MBEDTLS_CIPHER_DES_ECB, &des_ecb_info }, 01432 { MBEDTLS_CIPHER_DES_EDE_ECB, &des_ede_ecb_info }, 01433 { MBEDTLS_CIPHER_DES_EDE3_ECB, &des_ede3_ecb_info }, 01434 #if defined(MBEDTLS_CIPHER_MODE_CBC) 01435 { MBEDTLS_CIPHER_DES_CBC, &des_cbc_info }, 01436 { MBEDTLS_CIPHER_DES_EDE_CBC, &des_ede_cbc_info }, 01437 { MBEDTLS_CIPHER_DES_EDE3_CBC, &des_ede3_cbc_info }, 01438 #endif 01439 #endif /* MBEDTLS_DES_C */ 01440 01441 #if defined(MBEDTLS_CIPHER_NULL_CIPHER) 01442 { MBEDTLS_CIPHER_NULL, &null_cipher_info }, 01443 #endif /* MBEDTLS_CIPHER_NULL_CIPHER */ 01444 01445 { MBEDTLS_CIPHER_NONE, NULL } 01446 }; 01447 01448 #define NUM_CIPHERS sizeof mbedtls_cipher_definitions / sizeof mbedtls_cipher_definitions[0] 01449 int mbedtls_cipher_supported[NUM_CIPHERS]; 01450 01451 #endif /* MBEDTLS_CIPHER_C */
Generated on Tue Jul 12 2022 12:52:42 by
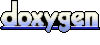