mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
cipher_internal.h
Go to the documentation of this file.
00001 /** 00002 * \file cipher_internal.h 00003 * 00004 * \brief Cipher wrappers. 00005 * 00006 * \author Adriaan de Jong <dejong@fox-it.com> 00007 * 00008 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00009 * SPDX-License-Identifier: Apache-2.0 00010 * 00011 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00012 * not use this file except in compliance with the License. 00013 * You may obtain a copy of the License at 00014 * 00015 * http://www.apache.org/licenses/LICENSE-2.0 00016 * 00017 * Unless required by applicable law or agreed to in writing, software 00018 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00019 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00020 * See the License for the specific language governing permissions and 00021 * limitations under the License. 00022 * 00023 * This file is part of mbed TLS (https://tls.mbed.org) 00024 */ 00025 #ifndef MBEDTLS_CIPHER_WRAP_H 00026 #define MBEDTLS_CIPHER_WRAP_H 00027 00028 #if !defined(MBEDTLS_CONFIG_FILE) 00029 #include "config.h" 00030 #else 00031 #include MBEDTLS_CONFIG_FILE 00032 #endif 00033 00034 #include "cipher.h" 00035 00036 #ifdef __cplusplus 00037 extern "C" { 00038 #endif 00039 00040 /** 00041 * Base cipher information. The non-mode specific functions and values. 00042 */ 00043 struct mbedtls_cipher_base_t 00044 { 00045 /** Base Cipher type (e.g. MBEDTLS_CIPHER_ID_AES) */ 00046 mbedtls_cipher_id_t cipher; 00047 00048 /** Encrypt using ECB */ 00049 int (*ecb_func)( void *ctx, mbedtls_operation_t mode, 00050 const unsigned char *input, unsigned char *output ); 00051 00052 #if defined(MBEDTLS_CIPHER_MODE_CBC) 00053 /** Encrypt using CBC */ 00054 int (*cbc_func)( void *ctx, mbedtls_operation_t mode, size_t length, 00055 unsigned char *iv, const unsigned char *input, 00056 unsigned char *output ); 00057 #endif 00058 00059 #if defined(MBEDTLS_CIPHER_MODE_CFB) 00060 /** Encrypt using CFB (Full length) */ 00061 int (*cfb_func)( void *ctx, mbedtls_operation_t mode, size_t length, size_t *iv_off, 00062 unsigned char *iv, const unsigned char *input, 00063 unsigned char *output ); 00064 #endif 00065 00066 #if defined(MBEDTLS_CIPHER_MODE_CTR) 00067 /** Encrypt using CTR */ 00068 int (*ctr_func)( void *ctx, size_t length, size_t *nc_off, 00069 unsigned char *nonce_counter, unsigned char *stream_block, 00070 const unsigned char *input, unsigned char *output ); 00071 #endif 00072 00073 #if defined(MBEDTLS_CIPHER_MODE_STREAM) 00074 /** Encrypt using STREAM */ 00075 int (*stream_func)( void *ctx, size_t length, 00076 const unsigned char *input, unsigned char *output ); 00077 #endif 00078 00079 /** Set key for encryption purposes */ 00080 int (*setkey_enc_func)( void *ctx, const unsigned char *key, 00081 unsigned int key_bitlen ); 00082 00083 /** Set key for decryption purposes */ 00084 int (*setkey_dec_func)( void *ctx, const unsigned char *key, 00085 unsigned int key_bitlen); 00086 00087 /** Allocate a new context */ 00088 void * (*ctx_alloc_func)( void ); 00089 00090 /** Free the given context */ 00091 void (*ctx_free_func)( void *ctx ); 00092 00093 }; 00094 00095 typedef struct 00096 { 00097 mbedtls_cipher_type_t type; 00098 const mbedtls_cipher_info_t *info; 00099 } mbedtls_cipher_definition_t; 00100 00101 extern const mbedtls_cipher_definition_t mbedtls_cipher_definitions[]; 00102 00103 extern int mbedtls_cipher_supported[]; 00104 00105 #ifdef __cplusplus 00106 } 00107 #endif 00108 00109 #endif /* MBEDTLS_CIPHER_WRAP_H */
Generated on Tue Jul 12 2022 12:52:42 by
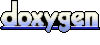