mbedtls ported to mbed-classic
Fork of mbedtls by
Embed:
(wiki syntax)
Show/hide line numbers
asn1.h
Go to the documentation of this file.
00001 /** 00002 * \file asn1.h 00003 * 00004 * \brief Generic ASN.1 parsing 00005 * 00006 * Copyright (C) 2006-2015, ARM Limited, All Rights Reserved 00007 * SPDX-License-Identifier: Apache-2.0 00008 * 00009 * Licensed under the Apache License, Version 2.0 (the "License"); you may 00010 * not use this file except in compliance with the License. 00011 * You may obtain a copy of the License at 00012 * 00013 * http://www.apache.org/licenses/LICENSE-2.0 00014 * 00015 * Unless required by applicable law or agreed to in writing, software 00016 * distributed under the License is distributed on an "AS IS" BASIS, WITHOUT 00017 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00018 * See the License for the specific language governing permissions and 00019 * limitations under the License. 00020 * 00021 * This file is part of mbed TLS (https://tls.mbed.org) 00022 */ 00023 #ifndef MBEDTLS_ASN1_H 00024 #define MBEDTLS_ASN1_H 00025 00026 #if !defined(MBEDTLS_CONFIG_FILE) 00027 #include "config.h" 00028 #else 00029 #include MBEDTLS_CONFIG_FILE 00030 #endif 00031 00032 #include <stddef.h> 00033 00034 #if defined(MBEDTLS_BIGNUM_C) 00035 #include "bignum.h" 00036 #endif 00037 00038 /** 00039 * \addtogroup asn1_module 00040 * \{ 00041 */ 00042 00043 /** 00044 * \name ASN1 Error codes 00045 * These error codes are OR'ed to X509 error codes for 00046 * higher error granularity. 00047 * ASN1 is a standard to specify data structures. 00048 * \{ 00049 */ 00050 #define MBEDTLS_ERR_ASN1_OUT_OF_DATA -0x0060 /**< Out of data when parsing an ASN1 data structure. */ 00051 #define MBEDTLS_ERR_ASN1_UNEXPECTED_TAG -0x0062 /**< ASN1 tag was of an unexpected value. */ 00052 #define MBEDTLS_ERR_ASN1_INVALID_LENGTH -0x0064 /**< Error when trying to determine the length or invalid length. */ 00053 #define MBEDTLS_ERR_ASN1_LENGTH_MISMATCH -0x0066 /**< Actual length differs from expected length. */ 00054 #define MBEDTLS_ERR_ASN1_INVALID_DATA -0x0068 /**< Data is invalid. (not used) */ 00055 #define MBEDTLS_ERR_ASN1_ALLOC_FAILED -0x006A /**< Memory allocation failed */ 00056 #define MBEDTLS_ERR_ASN1_BUF_TOO_SMALL -0x006C /**< Buffer too small when writing ASN.1 data structure. */ 00057 00058 /* \} name */ 00059 00060 /** 00061 * \name DER constants 00062 * These constants comply with DER encoded the ANS1 type tags. 00063 * DER encoding uses hexadecimal representation. 00064 * An example DER sequence is:\n 00065 * - 0x02 -- tag indicating INTEGER 00066 * - 0x01 -- length in octets 00067 * - 0x05 -- value 00068 * Such sequences are typically read into \c ::mbedtls_x509_buf. 00069 * \{ 00070 */ 00071 #define MBEDTLS_ASN1_BOOLEAN 0x01 00072 #define MBEDTLS_ASN1_INTEGER 0x02 00073 #define MBEDTLS_ASN1_BIT_STRING 0x03 00074 #define MBEDTLS_ASN1_OCTET_STRING 0x04 00075 #define MBEDTLS_ASN1_NULL 0x05 00076 #define MBEDTLS_ASN1_OID 0x06 00077 #define MBEDTLS_ASN1_UTF8_STRING 0x0C 00078 #define MBEDTLS_ASN1_SEQUENCE 0x10 00079 #define MBEDTLS_ASN1_SET 0x11 00080 #define MBEDTLS_ASN1_PRINTABLE_STRING 0x13 00081 #define MBEDTLS_ASN1_T61_STRING 0x14 00082 #define MBEDTLS_ASN1_IA5_STRING 0x16 00083 #define MBEDTLS_ASN1_UTC_TIME 0x17 00084 #define MBEDTLS_ASN1_GENERALIZED_TIME 0x18 00085 #define MBEDTLS_ASN1_UNIVERSAL_STRING 0x1C 00086 #define MBEDTLS_ASN1_BMP_STRING 0x1E 00087 #define MBEDTLS_ASN1_PRIMITIVE 0x00 00088 #define MBEDTLS_ASN1_CONSTRUCTED 0x20 00089 #define MBEDTLS_ASN1_CONTEXT_SPECIFIC 0x80 00090 /* \} name */ 00091 /* \} addtogroup asn1_module */ 00092 00093 /** Returns the size of the binary string, without the trailing \\0 */ 00094 #define MBEDTLS_OID_SIZE(x) (sizeof(x) - 1) 00095 00096 /** 00097 * Compares an mbedtls_asn1_buf structure to a reference OID. 00098 * 00099 * Only works for 'defined' oid_str values (MBEDTLS_OID_HMAC_SHA1), you cannot use a 00100 * 'unsigned char *oid' here! 00101 */ 00102 #define MBEDTLS_OID_CMP(oid_str, oid_buf) \ 00103 ( ( MBEDTLS_OID_SIZE(oid_str) != (oid_buf)->len ) || \ 00104 memcmp( (oid_str), (oid_buf)->p, (oid_buf)->len) != 0 ) 00105 00106 #ifdef __cplusplus 00107 extern "C" { 00108 #endif 00109 00110 /** 00111 * \name Functions to parse ASN.1 data structures 00112 * \{ 00113 */ 00114 00115 /** 00116 * Type-length-value structure that allows for ASN1 using DER. 00117 */ 00118 typedef struct mbedtls_asn1_buf 00119 { 00120 int tag; /**< ASN1 type, e.g. MBEDTLS_ASN1_UTF8_STRING. */ 00121 size_t len; /**< ASN1 length, in octets. */ 00122 unsigned char *p; /**< ASN1 data, e.g. in ASCII. */ 00123 } 00124 mbedtls_asn1_buf; 00125 00126 /** 00127 * Container for ASN1 bit strings. 00128 */ 00129 typedef struct mbedtls_asn1_bitstring 00130 { 00131 size_t len; /**< ASN1 length, in octets. */ 00132 unsigned char unused_bits; /**< Number of unused bits at the end of the string */ 00133 unsigned char *p; /**< Raw ASN1 data for the bit string */ 00134 } 00135 mbedtls_asn1_bitstring; 00136 00137 /** 00138 * Container for a sequence of ASN.1 items 00139 */ 00140 typedef struct mbedtls_asn1_sequence 00141 { 00142 mbedtls_asn1_buf buf; /**< Buffer containing the given ASN.1 item. */ 00143 struct mbedtls_asn1_sequence *next; /**< The next entry in the sequence. */ 00144 } 00145 mbedtls_asn1_sequence; 00146 00147 /** 00148 * Container for a sequence or list of 'named' ASN.1 data items 00149 */ 00150 typedef struct mbedtls_asn1_named_data 00151 { 00152 mbedtls_asn1_buf oid; /**< The object identifier. */ 00153 mbedtls_asn1_buf val; /**< The named value. */ 00154 struct mbedtls_asn1_named_data *next; /**< The next entry in the sequence. */ 00155 unsigned char next_merged; /**< Merge next item into the current one? */ 00156 } 00157 mbedtls_asn1_named_data; 00158 00159 /** 00160 * \brief Get the length of an ASN.1 element. 00161 * Updates the pointer to immediately behind the length. 00162 * 00163 * \param p The position in the ASN.1 data 00164 * \param end End of data 00165 * \param len The variable that will receive the value 00166 * 00167 * \return 0 if successful, MBEDTLS_ERR_ASN1_OUT_OF_DATA on reaching 00168 * end of data, MBEDTLS_ERR_ASN1_INVALID_LENGTH if length is 00169 * unparseable. 00170 */ 00171 int mbedtls_asn1_get_len( unsigned char **p, 00172 const unsigned char *end, 00173 size_t *len ); 00174 00175 /** 00176 * \brief Get the tag and length of the tag. Check for the requested tag. 00177 * Updates the pointer to immediately behind the tag and length. 00178 * 00179 * \param p The position in the ASN.1 data 00180 * \param end End of data 00181 * \param len The variable that will receive the length 00182 * \param tag The expected tag 00183 * 00184 * \return 0 if successful, MBEDTLS_ERR_ASN1_UNEXPECTED_TAG if tag did 00185 * not match requested tag, or another specific ASN.1 error code. 00186 */ 00187 int mbedtls_asn1_get_tag( unsigned char **p, 00188 const unsigned char *end, 00189 size_t *len, int tag ); 00190 00191 /** 00192 * \brief Retrieve a boolean ASN.1 tag and its value. 00193 * Updates the pointer to immediately behind the full tag. 00194 * 00195 * \param p The position in the ASN.1 data 00196 * \param end End of data 00197 * \param val The variable that will receive the value 00198 * 00199 * \return 0 if successful or a specific ASN.1 error code. 00200 */ 00201 int mbedtls_asn1_get_bool( unsigned char **p, 00202 const unsigned char *end, 00203 int *val ); 00204 00205 /** 00206 * \brief Retrieve an integer ASN.1 tag and its value. 00207 * Updates the pointer to immediately behind the full tag. 00208 * 00209 * \param p The position in the ASN.1 data 00210 * \param end End of data 00211 * \param val The variable that will receive the value 00212 * 00213 * \return 0 if successful or a specific ASN.1 error code. 00214 */ 00215 int mbedtls_asn1_get_int( unsigned char **p, 00216 const unsigned char *end, 00217 int *val ); 00218 00219 /** 00220 * \brief Retrieve a bitstring ASN.1 tag and its value. 00221 * Updates the pointer to immediately behind the full tag. 00222 * 00223 * \param p The position in the ASN.1 data 00224 * \param end End of data 00225 * \param bs The variable that will receive the value 00226 * 00227 * \return 0 if successful or a specific ASN.1 error code. 00228 */ 00229 int mbedtls_asn1_get_bitstring( unsigned char **p, const unsigned char *end, 00230 mbedtls_asn1_bitstring *bs); 00231 00232 /** 00233 * \brief Retrieve a bitstring ASN.1 tag without unused bits and its 00234 * value. 00235 * Updates the pointer to the beginning of the bit/octet string. 00236 * 00237 * \param p The position in the ASN.1 data 00238 * \param end End of data 00239 * \param len Length of the actual bit/octect string in bytes 00240 * 00241 * \return 0 if successful or a specific ASN.1 error code. 00242 */ 00243 int mbedtls_asn1_get_bitstring_null( unsigned char **p, const unsigned char *end, 00244 size_t *len ); 00245 00246 /** 00247 * \brief Parses and splits an ASN.1 "SEQUENCE OF <tag>" 00248 * Updated the pointer to immediately behind the full sequence tag. 00249 * 00250 * \param p The position in the ASN.1 data 00251 * \param end End of data 00252 * \param cur First variable in the chain to fill 00253 * \param tag Type of sequence 00254 * 00255 * \return 0 if successful or a specific ASN.1 error code. 00256 */ 00257 int mbedtls_asn1_get_sequence_of( unsigned char **p, 00258 const unsigned char *end, 00259 mbedtls_asn1_sequence *cur, 00260 int tag); 00261 00262 #if defined(MBEDTLS_BIGNUM_C) 00263 /** 00264 * \brief Retrieve a MPI value from an integer ASN.1 tag. 00265 * Updates the pointer to immediately behind the full tag. 00266 * 00267 * \param p The position in the ASN.1 data 00268 * \param end End of data 00269 * \param X The MPI that will receive the value 00270 * 00271 * \return 0 if successful or a specific ASN.1 or MPI error code. 00272 */ 00273 int mbedtls_asn1_get_mpi( unsigned char **p, 00274 const unsigned char *end, 00275 mbedtls_mpi *X ); 00276 #endif /* MBEDTLS_BIGNUM_C */ 00277 00278 /** 00279 * \brief Retrieve an AlgorithmIdentifier ASN.1 sequence. 00280 * Updates the pointer to immediately behind the full 00281 * AlgorithmIdentifier. 00282 * 00283 * \param p The position in the ASN.1 data 00284 * \param end End of data 00285 * \param alg The buffer to receive the OID 00286 * \param params The buffer to receive the params (if any) 00287 * 00288 * \return 0 if successful or a specific ASN.1 or MPI error code. 00289 */ 00290 int mbedtls_asn1_get_alg( unsigned char **p, 00291 const unsigned char *end, 00292 mbedtls_asn1_buf *alg, mbedtls_asn1_buf *params ); 00293 00294 /** 00295 * \brief Retrieve an AlgorithmIdentifier ASN.1 sequence with NULL or no 00296 * params. 00297 * Updates the pointer to immediately behind the full 00298 * AlgorithmIdentifier. 00299 * 00300 * \param p The position in the ASN.1 data 00301 * \param end End of data 00302 * \param alg The buffer to receive the OID 00303 * 00304 * \return 0 if successful or a specific ASN.1 or MPI error code. 00305 */ 00306 int mbedtls_asn1_get_alg_null( unsigned char **p, 00307 const unsigned char *end, 00308 mbedtls_asn1_buf *alg ); 00309 00310 /** 00311 * \brief Find a specific named_data entry in a sequence or list based on 00312 * the OID. 00313 * 00314 * \param list The list to seek through 00315 * \param oid The OID to look for 00316 * \param len Size of the OID 00317 * 00318 * \return NULL if not found, or a pointer to the existing entry. 00319 */ 00320 mbedtls_asn1_named_data *mbedtls_asn1_find_named_data( mbedtls_asn1_named_data *list, 00321 const char *oid, size_t len ); 00322 00323 /** 00324 * \brief Free a mbedtls_asn1_named_data entry 00325 * 00326 * \param entry The named data entry to free 00327 */ 00328 void mbedtls_asn1_free_named_data( mbedtls_asn1_named_data *entry ); 00329 00330 /** 00331 * \brief Free all entries in a mbedtls_asn1_named_data list 00332 * Head will be set to NULL 00333 * 00334 * \param head Pointer to the head of the list of named data entries to free 00335 */ 00336 void mbedtls_asn1_free_named_data_list( mbedtls_asn1_named_data **head ); 00337 00338 #ifdef __cplusplus 00339 } 00340 #endif 00341 00342 #endif /* asn1.h */
Generated on Tue Jul 12 2022 12:52:41 by
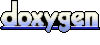