
HTTP and HTTPS example application for Mbed OS 5
Dependencies: mbed-http
network-helper.h
00001 #ifndef _MBED_HTTP_EXAMPLE_H_ 00002 #define _MBED_HTTP_EXAMPLE_H_ 00003 00004 #include "mbed.h" 00005 #include "NetworkInterface.h" 00006 00007 /** 00008 * Connect to the network using the default networking interface, 00009 * you can also swap this out with a driver for a different networking interface 00010 * if you use WiFi: see mbed_app.json for the credentials 00011 */ 00012 NetworkInterface *connect_to_default_network_interface() { 00013 printf("[NWKH] Connecting to network...\n"); 00014 00015 NetworkInterface* network = NetworkInterface::get_default_instance(); 00016 00017 if (!network) { 00018 printf("[NWKH] No network interface found, select an interface in 'network-helper.h'\n"); 00019 return NULL; 00020 } 00021 00022 nsapi_error_t connect_status = network->connect(); 00023 00024 if (connect_status != NSAPI_ERROR_OK) { 00025 printf("[NWKH] Failed to connect to network (%d)\n", connect_status); 00026 return NULL; 00027 } 00028 00029 printf("[NWKH] Connected to the network\n"); 00030 printf("[NWKH] IP address: %s\n", network->get_ip_address()); 00031 return network; 00032 } 00033 00034 #endif // _MBED_HTTP_EXAMPLE_H_
Generated on Thu Jul 14 2022 06:47:14 by
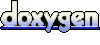