
projet en 1 main.cpp
Embed:
(wiki syntax)
Show/hide line numbers
gps.h
00001 #include "mbed.h" 00002 00003 char lati[20], longi[20]; 00004 int Ilati, Ilongi; 00005 00006 Serial pc(PA_0,PA_1); // tx, rx 00007 00008 char gpsString[1024]; 00009 char tmp[20] = {}; 00010 char tmp2[20] = {}; 00011 char gga1[1024]; 00012 char * gga2; 00013 char * fix; 00014 uint8_t sep; 00015 uint8_t mode = 2; //mode=1 fix information; mode=2 normal 00016 00017 typedef struct { 00018 uint8_t heure; 00019 uint8_t minute; 00020 uint8_t seconde; 00021 } tim_t; 00022 00023 typedef struct { 00024 uint8_t deg; 00025 uint8_t min; 00026 double sec; 00027 char azimute; 00028 } pos_t; 00029 00030 typedef struct { 00031 tim_t tim; 00032 pos_t lat; 00033 pos_t lon; 00034 uint8_t sat; // Nombre de satellites utilisés pour calculer les coordonnées 00035 uint8_t fix; // Fix qualification : (0 = non valide, 1 = Fix GPS, 2 = Fix DGPS), Type de positionnement (le 1 est un positionnement GPS) 00036 float prs; // Précision horizontale ou HDOP (Horizontal dilution of precision) 00037 float alt; // Altitude, en Metres, au dessus du MSL (mean see level) niveau moyen des Océans. 00038 char unitAlt; // Unité de l'altitude (en mètre dans la majorité des trames) 00039 } gps_t; 00040 00041 // structure contenant les données de la trame GGA à envoyer 00042 gps_t GPGGA; 00043 00044 char * convert(char* ch, pos_t* pos) { 00045 00046 double f = atof(ch); 00047 00048 pos->deg = (uint8_t)(f / 100.0); 00049 pos->min = (uint8_t)(f - ((pos->deg) * 100.0)); 00050 pos->sec = 60.0*(f - ((pos->deg)*100.0) - (pos->min)); 00051 00052 char *s = (char*)calloc(14,sizeof(char)); 00053 00054 sprintf(s,"%3d'%2d'%5.3f\"",pos->deg,pos->min,pos->sec); 00055 00056 return s; 00057 } 00058 00059 char * time(char* ch, tim_t* tim) { 00060 00061 char hh[3], mm[3], ss[7]; 00062 //hhmmss.nnn 00063 memcpy(hh, &ch[0], 2); 00064 memcpy(mm, &ch[2], 2); 00065 memcpy(ss, &ch[4], 6); 00066 00067 tim->heure = atoi(hh); 00068 tim->minute = atoi(mm); 00069 tim->seconde = atoi(ss); //cast de double vers intteger, on perd la précision des millisecondes 00070 tim->heure++; //convertion des heures de UTC vers UTC+1 00071 00072 char *s = (char*)calloc(14,sizeof(char)); 00073 00074 sprintf(s,"%02d:%02d:%02d",tim->heure,tim->minute,tim->seconde); 00075 00076 return s; 00077 } 00078 /**************************************/ 00079 00080 00081 uint8_t parseGGA() { 00082 gga2 = strtok(gga1, ","); 00083 while (gga2 != NULL) { 00084 switch (sep) { 00085 case 1: // heure d'envoi de la trame 00086 if (mode == 2) { 00087 strcpy(tmp2,time( gga2,&(GPGGA.tim) )); 00088 pc.printf("\r\n-----Donnees GPS-----\r\nTim: %s\r\n",tmp2); 00089 00090 } 00091 break; 00092 case 2 : // latitude 00093 if (mode == 2) { 00094 strcpy(tmp,convert( gga2,&(GPGGA.lat) )); 00095 sprintf(lati,"\r%s",tmp); 00096 pc.printf("\r%s",lati); 00097 00098 } 00099 break; 00100 case 3 : // N: Nord, S : Sud 00101 if (mode == 2) { 00102 GPGGA.lat.azimute = gga2[0]; 00103 pc.printf("%s\n\r",gga2); 00104 wait(0.25); 00105 } 00106 break; 00107 case 4 : // longitude 00108 if (mode == 2) { 00109 strcpy(tmp,convert( gga2,&(GPGGA.lat) )); 00110 sprintf(longi,"\r%s",tmp); 00111 pc.printf("\r%s",longi); 00112 } 00113 break; 00114 case 5 : // E: Est, W: Ouest 00115 if (mode == 2) { 00116 GPGGA.lat.azimute = gga2[0]; 00117 pc.printf("%s\n\r-----Donnees GPS-----\r\n",gga2); 00118 00119 wait(0.25); 00120 } 00121 break; 00122 case 6: 00123 if (mode == 1) { 00124 if (gga2 == "0") { 00125 fix = "Invalid"; 00126 } 00127 if (gga2 == "1") { 00128 fix = "GPS Fix (SPS)"; 00129 } 00130 if (gga2 == "2") { 00131 fix = "DGPS Fix"; 00132 } 00133 if (gga2 == "3") { 00134 fix = "PPS Fix"; 00135 } 00136 if (gga2 == "4") { 00137 fix = "Real Time Kinematic"; 00138 } 00139 if (gga2 == "5") { 00140 fix = "Float RTK"; 00141 } 00142 if (gga2 == "6") { 00143 fix = "Estimated (Dead Reckoning)"; 00144 } 00145 if (gga2 == "7") { 00146 fix = "Manual Input Mode"; 00147 } 00148 if (gga2 == "8") { 00149 fix = "Simulation Mode"; 00150 } 00151 GPGGA.fix = atoi(gga2); 00152 pc.printf("FIX: %s_%s",gga2,fix); 00153 } 00154 break; 00155 case 7 : // Nombre de satellites 00156 if (mode == 2) { 00157 GPGGA.sat = atoi(gga2); 00158 pc.printf("Inf: Sat:%s",gga2); 00159 } 00160 break; 00161 case 8 : // Precision 00162 if (mode == 2) { 00163 GPGGA.prs = atof(gga2); 00164 pc.printf(" Prs:%s",gga2); 00165 } 00166 break; 00167 case 9 : // Altitude 00168 if (mode == 2) { 00169 GPGGA.alt = atof(gga2); 00170 pc.printf(" Alt:%s",gga2); 00171 } 00172 break; 00173 case 10 : // Unité altitude 00174 if (mode == 2) { 00175 GPGGA.unitAlt = gga2[0]; 00176 pc.printf("%s\n\r",gga2); 00177 } 00178 break; 00179 } 00180 gga2 = strtok(NULL, ","); 00181 sep++; 00182 } 00183 sep = 1; 00184 return *gga2; 00185 00186 } 00187 00188 uint8_t getGPSstring() { // str used to choose between GPS trame type, here we have only GPGGA wich is available 00189 uint8_t str=1; 00190 if (pc.scanf("%s", &gpsString) ==1) { 00191 if(str==1) { 00192 if (sscanf(gpsString, "$GPGGA,%s",gga1) >=1) 00193 { 00194 sep = 1; 00195 parseGGA(); 00196 } 00197 return *gga2; 00198 } 00199 } 00200 else 00201 { 00202 pc.printf("NO GPGGA DATA RECEIVED\n\r"); 00203 } 00204 return 0; 00205 } 00206
Generated on Wed Jul 13 2022 20:54:22 by
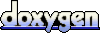