The Cayenne MQTT mbed Library provides functions to easily connect to the Cayenne IoT project builder.
Dependents: Cayenne-ESP8266Interface Cayenne-WIZnet_Library Cayenne-WIZnetInterface Cayenne-X-NUCLEO-IDW01M1 ... more
MQTTSerializePublish.c
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 * Ian Craggs - fix for https://bugs.eclipse.org/bugs/show_bug.cgi?id=453144 00016 *******************************************************************************/ 00017 00018 #include "MQTTPacket.h" 00019 00020 #include <string.h> 00021 00022 00023 /** 00024 * Determines the length of the MQTT publish packet that would be produced using the supplied parameters 00025 * @param qos the MQTT QoS of the publish (packetid is omitted for QoS 0) 00026 * @param topicName the topic name to be used in the publish 00027 * @param payloadlen the length of the payload to be sent 00028 * @return the length of buffer needed to contain the serialized version of the packet 00029 */ 00030 size_t MQTTSerialize_publishLength(int qos, MQTTString topicName, size_t payloadlen) 00031 { 00032 size_t len = 0; 00033 00034 len += 2 + MQTTstrlen(topicName) + payloadlen; 00035 if (qos > 0) 00036 len += 2; /* packetid */ 00037 return len; 00038 } 00039 00040 00041 /** 00042 * Serializes the supplied publish data into the supplied buffer, ready for sending 00043 * @param buf the buffer into which the packet will be serialized 00044 * @param buflen the length in bytes of the supplied buffer 00045 * @param dup integer - the MQTT dup flag 00046 * @param qos integer - the MQTT QoS value 00047 * @param retained integer - the MQTT retained flag 00048 * @param packetid integer - the MQTT packet identifier 00049 * @param topicName MQTTString - the MQTT topic in the publish 00050 * @param payload byte buffer - the MQTT publish payload 00051 * @param payloadlen integer - the length of the MQTT payload 00052 * @return the length of the serialized data. <= 0 indicates error 00053 */ 00054 int MQTTSerialize_publish(unsigned char* buf, size_t buflen, unsigned char dup, int qos, unsigned char retained, unsigned short packetid, 00055 MQTTString topicName, unsigned char* payload, size_t payloadlen) 00056 { 00057 unsigned char *ptr = buf; 00058 MQTTHeader header = {0}; 00059 size_t rem_len = 0; 00060 int rc = 0; 00061 00062 if (MQTTPacket_len(rem_len = MQTTSerialize_publishLength(qos, topicName, payloadlen)) > buflen) 00063 { 00064 rc = MQTTPACKET_BUFFER_TOO_SHORT; 00065 goto exit; 00066 } 00067 00068 header.bits.type = PUBLISH_MSG; 00069 header.bits.dup = dup; 00070 header.bits.qos = qos; 00071 header.bits.retain = retained; 00072 writeChar(&ptr, header.byte); /* write header */ 00073 00074 ptr += MQTTPacket_encode(ptr, rem_len); /* write remaining length */; 00075 00076 writeMQTTString(&ptr, topicName); 00077 00078 if (qos > 0) 00079 writeInt(&ptr, packetid); 00080 00081 memcpy(ptr, payload, payloadlen); 00082 ptr += payloadlen; 00083 00084 rc = (int)(ptr - buf); 00085 00086 exit: 00087 return rc; 00088 } 00089 00090 00091 00092 /** 00093 * Serializes the ack packet into the supplied buffer. 00094 * @param buf the buffer into which the packet will be serialized 00095 * @param buflen the length in bytes of the supplied buffer 00096 * @param type the MQTT packet type 00097 * @param dup the MQTT dup flag 00098 * @param packetid the MQTT packet identifier 00099 * @return serialized length, or error if 0 00100 */ 00101 int MQTTSerialize_ack(unsigned char* buf, size_t buflen, unsigned char packettype, unsigned char dup, unsigned short packetid) 00102 { 00103 MQTTHeader header = {0}; 00104 int rc = 0; 00105 unsigned char *ptr = buf; 00106 00107 if (buflen < 4) 00108 { 00109 rc = MQTTPACKET_BUFFER_TOO_SHORT; 00110 goto exit; 00111 } 00112 header.bits.type = packettype; 00113 header.bits.dup = dup; 00114 header.bits.qos = (packettype == PUBREL_MSG) ? 1 : 0; 00115 writeChar(&ptr, header.byte); /* write header */ 00116 00117 ptr += MQTTPacket_encode(ptr, 2); /* write remaining length */ 00118 writeInt(&ptr, packetid); 00119 rc = (int)(ptr - buf); 00120 exit: 00121 return rc; 00122 } 00123 00124 00125 /** 00126 * Serializes a puback packet into the supplied buffer. 00127 * @param buf the buffer into which the packet will be serialized 00128 * @param buflen the length in bytes of the supplied buffer 00129 * @param packetid integer - the MQTT packet identifier 00130 * @return serialized length, or error if 0 00131 */ 00132 int MQTTSerialize_puback(unsigned char* buf, size_t buflen, unsigned short packetid) 00133 { 00134 return MQTTSerialize_ack(buf, buflen, PUBACK_MSG, 0, packetid); 00135 } 00136 00137 00138 /** 00139 * Serializes a pubrel packet into the supplied buffer. 00140 * @param buf the buffer into which the packet will be serialized 00141 * @param buflen the length in bytes of the supplied buffer 00142 * @param dup integer - the MQTT dup flag 00143 * @param packetid integer - the MQTT packet identifier 00144 * @return serialized length, or error if 0 00145 */ 00146 int MQTTSerialize_pubrel(unsigned char* buf, size_t buflen, unsigned char dup, unsigned short packetid) 00147 { 00148 return MQTTSerialize_ack(buf, buflen, PUBREL_MSG, dup, packetid); 00149 } 00150 00151 00152 /** 00153 * Serializes a pubrel packet into the supplied buffer. 00154 * @param buf the buffer into which the packet will be serialized 00155 * @param buflen the length in bytes of the supplied buffer 00156 * @param packetid integer - the MQTT packet identifier 00157 * @return serialized length, or error if 0 00158 */ 00159 int MQTTSerialize_pubcomp(unsigned char* buf, size_t buflen, unsigned short packetid) 00160 { 00161 return MQTTSerialize_ack(buf, buflen, PUBCOMP_MSG, 0, packetid); 00162 } 00163 00164
Generated on Wed Jul 13 2022 15:49:28 by
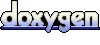