
LPC1768 programm for the led matrix.
Dependencies: BufferedSerial DS3231 mbed-rtos mbed
setMatrix.cpp
00001 #include "initalize.h" //Funktionenprototypen 00002 #include "alphabet.h" //Header mit allen Buchstaben und Zeichen Variationen 00003 #include "mbed.h" // 00004 #include <string> // 00005 #include "rtos.h" //Verwendet für RtosTimer 00006 #include <BufferedSerial.h> //MCU besitzt eine Buffer-Limite von 16 bytes. Diese Library erweitert diese Limite 00007 #include "DS3231.h" //Library für die Echtzeituhr DS3231 00008 #include "project.h" //Definitionen 00009 #include "time_adjustment_dates.h" //Daten für die Winter und Sommerzeit 00010 00011 //============================================================================================ 00012 //VARIABLEN 00013 00014 00015 struct LED_Matrix 00016 { 00017 DigitalOut ds,oe,stcp,shcp,mr; 00018 LED_Matrix(PinName pin1, PinName pin2, PinName pin3, PinName pin4, PinName pin5) : ds(pin1), oe(pin2), stcp(pin3), shcp(pin4), mr(pin5) {}; 00019 }; 00020 //PIN-ASSIGNMENT------------------------------------------------------------------------------ 00021 DS3231 RTC(p28,p27); //RTC über p28(SDA) und p27(SCL) verbunden 00022 00023 BusOut led(LED1,LED2,LED3,LED4); 00024 00025 LED_Matrix matrix(p5, p6, p7, p8, p9); //LED-Matrix Data, Enable, Storage, Shift, Masterreset 00026 00027 RtosTimer customDuration(CustomTextCycleFinished); //Timer für benutzerdefinierter Text 00028 RtosTimer TimerTextSwitch(textSwitcher); //Timer für den Wechsel zwischen Zeit und Datum 00029 00030 BufferedSerial bluetooth(p13,p14); //Bluetooth Modul, tx, rx 00031 00032 //GLOBAL VARIABLE---------------------------------------------------------------------------------- 00033 //Clock 00034 int rtc_hour; //Stunden 00035 int rtc_minute; //Minuten 00036 int rtc_second; //Sekunden 00037 int rtc_date; //Datum 00038 int rtc_dayOfWeek; //Tag der Woche 00039 int rtc_month; //Monat 00040 int rtc_year; //Jahr 00041 // 00042 //Time Values 00043 int CustomDuration = TIME_CUSTOM_DEF; 00044 00045 //bluetooth 00046 string TextBluetooth (""); 00047 char ErrorValue = 0; 00048 00049 //matrix 00050 bool matrix_setScroll = 1; 00051 char textSwitch = matrixTIME; 00052 string TextMatrix (""); 00053 00054 int matrixPos = 0; 00055 int matrix_frames; // Bilder-Counter 00056 int matrix_moveFrame = 0; // Variable zum Verschieben des Textes 00057 00058 00059 //ENUM & STRUCT------------------------------------------------------------------------------- 00060 00061 //============================================================================================ 00062 00063 //============================================================================================ 00064 //▄ ▄ ▄▄▄ ▄▄▄▄▄ ▄▄▄▄ ▄▄▄ ▄ ▄ 00065 //█▀▄ ▄▀█ █ █ █ █ █ █ █ █ 00066 //█ ▀ █ █▄▄▄█ █ █▄▄▄▀ █ ▀▄▀ 00067 //█ █ █ █ █ █ ▀▄ █ ▄▀ ▀▄ 00068 //█ █ █ █ █ █ ▀▄ ▄█▄ █ █ 00069 //MATRIX======================================================================= 00070 //Autor: Joel von Rotz 00071 //Typ: void 00072 //parameter: int m_length, int m_height, char *matrix_value 00073 // Matrixlänge Matrixhöhe Array für Textwerte 00074 //Beschreibung: 00075 //============================================================================= 00076 void Matrix(int m_length, int m_height, char *matrix_value) 00077 { 00078 matrix.oe = 1; //Matrix ausschalten, damit keine unerwünschte LEDs leuchten. 00079 TextMatrix = TextStandard; //Standard-Text zuweisen 00080 wait(0.5); 00081 matrix.oe = 0; //Matrix wieder einschalten 00082 00083 RTC.setI2Cfrequency(400000); 00084 bluetooth.baud(115200); //Bluetooth Module Communication 00085 00086 TimerTextSwitch.start(TIME_CHANGE); 00087 00088 while(1) 00089 { 00090 initVal(); //Variabeln initialisieren 00091 00092 for(int x = 0; x < 512; x++) //matrix_value "leeren" 00093 matrix_value[x] = 0x00; 00094 00095 00096 ReadTimeToString(); //Lese Zeit und konvertiere zu einem String 00097 00098 00099 TextMatrixConvert(TextMatrix,matrix_value, m_length); 00100 00101 /*Überprüft ob es sich um Winterzeit oder Sommerzeit handelt*/ 00102 WinterSummerTime(rtc_year,rtc_month,rtc_date,rtc_hour,rtc_second); 00103 //----------------------------// 00104 if((matrix_frames-64) > m_length) 00105 { 00106 matrix_setScroll = 1; 00107 } 00108 else 00109 { 00110 matrix_setScroll = 0; 00111 } 00112 if(!matrix_setScroll) 00113 { 00114 matrix_moveFrame = 64; 00115 } 00116 //----------------------------// 00117 setMatrix(m_height, m_length, matrix_value); 00118 //----------------------------// 00119 if(bluetooth.readable()) 00120 { 00121 bluetooth_matrix(m_length,m_height,matrix_value); 00122 } 00123 //----------------------------// 00124 } 00125 } 00126 00127 //TEXTSWITCHER================================================================= 00128 //Autor: Joel von Rotz 00129 //Typ: void 00130 //parameter: void 00131 //Beschreibung: Wird für das Wechseln des Textes benötigt 00132 //============================================================================= 00133 void textSwitcher(void) 00134 { 00135 matrixPos = 0; 00136 matrix_moveFrame = 0; 00137 textSwitch = !textSwitch; 00138 } 00139 00140 void CustomTextCycleFinished(void) 00141 { 00142 TimerTextSwitch.start(TIME_CHANGE); 00143 customDuration.stop(); 00144 textSwitch = matrixTIME; 00145 TextMatrix = TextStandard; 00146 00147 } 00148 00149 //INITVAL====================================================================== 00150 //Autor: Joel von Rotz 00151 //Typ: void 00152 //parameter: void 00153 //Beschreibung: Initialisierung der Variabeln und Ein-Ausgängen 00154 //============================================================================= 00155 void initVal(void) 00156 { 00157 //Überprüfung, ob Text verschoben werden muss auf dem Display 00158 00159 //===================== 00160 //Output 00161 matrix.mr = 1; 00162 matrix.oe = 1; 00163 matrix.ds = 0; 00164 matrix.stcp = 0; 00165 matrix.shcp = 0; 00166 //===================== 00167 //Variabeln 00168 matrix_frames = 0; 00169 TextBluetooth = ""; 00170 reset_srg(); 00171 } 00172 00173 //MATRIX======================================================================= 00174 //Autor: Joel von Rotz 00175 //Typ: void 00176 //parameter: int m_height, int m_length, char *matrix_value 00177 //Beschreibung: Lässt Text oder anderes auf einer LED-Matrix anzeigen 00178 //============================================================================= 00179 // MATRIX 00180 //Lässt einen Text auf dem Display anzeigen 00181 void setMatrix(int m_height,int m_length, char *matrix_value) 00182 { 00183 //clock wird als Zählvariable verwendet, darunter als Bitshifter und Multiplexer 00184 for(int frequency = 0; frequency < 4; frequency++) 00185 { 00186 for(int clock = 0; clock < 8; clock++) 00187 { 00188 //Der Text muss von hinten nach vorne abgearbeitet werden, darum wird eine Variable 00189 //names matrixPos verwendet, die die Position des Textes bestimmt. 00190 if(matrixPos <= 0) 00191 matrixPos = m_length; //setze matrixPos auf die letzte Position des Textes zurück 00192 00193 00194 for(int fill = 0; fill < (m_length/8); fill++) 00195 { 00196 for(int column = 8; column > 0; column--) 00197 { 00198 matrix.ds = (matrix_value[matrixPos-column+matrix_moveFrame] >> clock) & 1; 00199 shift_srg(); 00200 } 00201 matrixPos -= 8; 00202 } 00203 //Multiplexer 00204 //mit dieser For-Schleife wird der Multiplex-Bit gesetzt 00205 for(int cell = 0; cell < 8; cell++) 00206 { 00207 if(clock == cell) //ist clock gleich cell? 00208 matrix.ds = 1; //setze Data-Pin auf 1 00209 else //Sonst 00210 matrix.ds = 0; //setze auf 0 00211 shift_srg(); //Schiebe Data in die Register hinein 00212 } 00213 00214 store_srg(); 00215 matrix.oe = 0; 00216 wait_us(1000); 00217 matrix.oe = 1; 00218 } 00219 00220 } 00221 //=================================================================== 00222 if(matrix_setScroll) 00223 { 00224 matrix_moveFrame++; 00225 } 00226 if(matrix_moveFrame >= matrix_frames) 00227 { 00228 matrix_moveFrame = 0; 00229 } 00230 //=================================================================== 00231 } 00232 00233 00234 //Schieberegister mit einem Bit befüllen 00235 void shift_srg() 00236 { 00237 matrix.shcp = 1; 00238 matrix.shcp = 0; 00239 } 00240 00241 //Speicher von den Schieberegister herausgeben 00242 void store_srg() 00243 { 00244 matrix.stcp = 1; 00245 matrix.stcp = 0; 00246 } 00247 00248 //Schieberegister zurücksetzen 00249 void reset_srg() 00250 { 00251 matrix.mr = 0; 00252 matrix.mr = 1; 00253 } 00254 00255 /*Steuerung für die LED-Matrix*/ 00256 void bluetooth_matrix(int m_length, int m_height, char *matrix_value) 00257 { 00258 TextBluetooth = ""; 00259 ErrorValue = NO_ERROR; 00260 string buff; 00261 00262 while(bluetooth.readable()) 00263 { 00264 TextBluetooth += bluetooth.getc(); 00265 } 00266 //------------------------------------------ 00267 //Bluetooth Nachrichten 00268 if(TextBluetooth.size()==28 && TextBluetooth.find("matrixCONNECT") != string::npos) 00269 { 00270 TextMatrix = "Connected!"; 00271 bluetooth.printf("Hallo! Schreibe etwas..."); 00272 } 00273 else if(TextBluetooth.size()==17 && TextBluetooth.find("matrixNEW_PAIRING") != string::npos || 00274 TextBluetooth.size()==16 && TextBluetooth.find("matrixDISCONNECT") != string::npos ) 00275 { 00276 TextBluetooth = ""; 00277 } 00278 //------------------------------------------ 00279 //Zeit einstellen 00280 else if(TextBluetooth.at(0) == '?') //ist das erste Zeichen ein Befehlszeichen 00281 { 00282 TextBluetooth.erase(0,1); 00283 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00284 if(TextBluetooth.find("ST ")== 0) //Konfiguration Zeit 00285 { TextBluetooth.erase(0,3); 00286 if(TextBluetooth.size() == 8) 00287 { 00288 00289 buff.assign(TextBluetooth,0,2); 00290 rtc_hour = atoi(buff.c_str()); 00291 buff.assign(TextBluetooth,3,2); 00292 rtc_minute = atoi(buff.c_str()); 00293 buff.assign(TextBluetooth,6,2); 00294 rtc_second = atoi(buff.c_str()); 00295 if(rtc_hour <= 23 && rtc_minute <= 59 && rtc_second <= 59) 00296 { 00297 RTC.setTime(rtc_hour,rtc_minute,rtc_second); 00298 } 00299 else 00300 { 00301 ErrorValue = CMD_NOT_IN_RANGE; 00302 } 00303 } 00304 else 00305 { 00306 ErrorValue = CMD_NOT_COMPLETE; 00307 } 00308 } 00309 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00310 else if(TextBluetooth.find("SD ")== 0) //Konfiguration Datum 00311 { TextBluetooth.erase(0,3); 00312 if(TextBluetooth.size() == 10) 00313 { 00314 /*Konvertiere String zu Int*/ 00315 buff.assign(TextBluetooth,0,2); 00316 rtc_date = atoi(buff.c_str()); 00317 buff.assign(TextBluetooth,3,2); 00318 rtc_month = atoi(buff.c_str()); 00319 buff.assign(TextBluetooth,6,4); 00320 rtc_year = atoi(buff.c_str()); 00321 if(rtc_year <= 2100 && rtc_year >= 1900 && checkDate(rtc_year,rtc_month,rtc_date)) 00322 { 00323 RTC.setDate(0,rtc_date,rtc_month,rtc_year); 00324 } 00325 else if( ErrorValue == FEB_NO_LEAP) {} 00326 else 00327 { 00328 ErrorValue = CMD_NOT_IN_RANGE; 00329 } 00330 } 00331 else 00332 { 00333 ErrorValue = CMD_NOT_COMPLETE; 00334 } 00335 } 00336 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00337 else if(TextBluetooth.find("SW ")== 0) //Konfiguration Winterzeit 00338 { TextBluetooth.erase(0,3); 00339 TextBluetooth = "Winterzeit"; 00340 } 00341 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00342 else if(TextBluetooth.find("SS ")== 0) //Konfiguration Sommerzeit 00343 { TextBluetooth.erase(0,3); 00344 TextBluetooth = "Sommerzeit"; 00345 } 00346 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00347 else if(TextBluetooth.find("CD ")== 0) //Konfiguration Benutzerdefinierte Textdauer 00348 { TextBluetooth.erase(0,3); 00349 if(TextBluetooth.size() == 3) 00350 { 00351 CustomDuration = atoi(TextBluetooth.c_str()); 00352 if(CustomDuration <= 600 && CustomDuration >= 1){} 00353 else 00354 { 00355 ErrorValue = CMD_NOT_IN_RANGE; 00356 CustomDuration = TIME_CUSTOM_DEF; 00357 } 00358 } 00359 else 00360 { 00361 ErrorValue = CMD_NOT_COMPLETE; 00362 } 00363 } 00364 /*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/ 00365 else 00366 { 00367 ErrorValue = INVALID_CMD; 00368 } 00369 BLE_ErrorHandler(ErrorValue); 00370 } 00371 //----------------------------------------------------- 00372 //Benutzerdefinierter Text 00373 else 00374 { 00375 TextMatrix = TextBluetooth; 00376 textSwitch = matrixCUSTOM; 00377 TimerTextSwitch.stop(); //Stoppe den Timer für den Zeit-Datum-Wechsel 00378 customDuration.start(CustomDuration*1000); //2 Minuten eigener Text 00379 } 00380 } 00381 00382 void BLE_ErrorHandler(char value) 00383 { 00384 switch(value) 00385 { 00386 case NO_ERROR: 00387 TimerTextSwitch.start(TIME_CHANGE); 00388 customDuration.stop(); 00389 textSwitch = matrixTIME; 00390 break; 00391 case INVALID_CMD: 00392 TextMatrix = "ERROR: Invalid Command"; 00393 break; 00394 case CMD_NOT_COMPLETE: 00395 TextMatrix = "ERROR: Missing Command Parameters"; 00396 break; 00397 case CMD_NOT_IN_RANGE: 00398 TextMatrix = "ERROR: Values Not In Range"; 00399 break; 00400 case FEB_NO_LEAP: 00401 TextMatrix = "ERROR: February-Date Too Big (Not a Leapyear)"; 00402 break; 00403 } 00404 if(value != NO_ERROR) 00405 { 00406 textSwitch = matrixCUSTOM; 00407 TimerTextSwitch.stop(); //Stoppe den Timer für den Zeit-Datum-Wechsel 00408 customDuration.start(10000); //2 Minuten eigener Text 00409 } 00410 } 00411 00412 void WinterSummerTime(int yearVal,int monthVal,int dateVal,int hourVal,int secondVal) 00413 { 00414 /*timeLock wird als Wiederholungsstop verwendet. Da bei der Winterzeit eine Stunden * 00415 *zurückgestellt wird, wird die Zeit immer wieder um eine Stunde zurückgestellt. */ 00416 static bool timeLock = 0; 00417 00418 //Summertime 00419 if( dateVal == summerdates[(yearVal % 2000)][0] && 00420 monthVal == summerdates[(yearVal % 2000)][1] && 00421 (yearVal % 2000)== summerdates[(yearVal % 2000)][2] && 00422 hourVal == summerdates[(yearVal % 2000)][3] && 00423 secondVal== summerdates[(yearVal % 2000)][4] && 00424 timeLock == 1) 00425 { 00426 timeLock = 0; 00427 hourVal += 1; 00428 RTC.setTime(hourVal,rtc_minute,rtc_second); 00429 } 00430 //WINTERTIME 00431 if( dateVal == winterdates[(yearVal % 2000)][0] && 00432 monthVal == winterdates[(yearVal % 2000)][1] && 00433 (yearVal % 2000)== winterdates[(yearVal % 2000)][2] && 00434 hourVal == winterdates[(yearVal % 2000)][3] && 00435 secondVal== winterdates[(yearVal % 2000)][4] && 00436 timeLock == 0) 00437 { 00438 timeLock = 1; 00439 hourVal -= 1; 00440 RTC.setTime(hourVal,rtc_minute,rtc_second); 00441 } 00442 } 00443 00444 //============================================================================= 00445 00446 00447 //TimeStringConvert============================================================ 00448 //Autor: Joel von Rotz 00449 //Typ: void 00450 //parameter: void 00451 //Beschreibung: 00452 // Das Datum und die Uhrzeit wird am Terminal angezeigt. 00453 //============================================================================= 00454 void ReadTimeToString(void) 00455 { 00456 RTC.readDateTime(&rtc_dayOfWeek,&rtc_date,&rtc_month,&rtc_year,&rtc_hour,&rtc_minute,&rtc_second); 00457 char buff[100]; 00458 if(textSwitch == matrixTIME) 00459 { 00460 sprintf(buff," %02i:%02i:%02i",rtc_hour,rtc_minute,rtc_second); 00461 00462 } 00463 else if(textSwitch == matrixDATE) 00464 { 00465 sprintf(buff," %02i.%02i.%04i",rtc_date,rtc_month,rtc_year); 00466 } 00467 if(textSwitch != matrixCUSTOM) 00468 { 00469 TextMatrix = buff; 00470 } 00471 } 00472 //============================================================================= 00473 00474 //SETDATEMAX=================================================================== 00475 //Autor: Joel von Rotz 00476 //Typ: char 00477 //parameter: int monthVal (Monat wird übergeben) 00478 //Beschreibung: 00479 // Es wird anhand dem Monat die dazugehörigen maximalen Tagen zurück- 00480 // gegeben. 00481 //============================================================================= 00482 bool checkDate(int yearVal, int monthVal, int dateVal) 00483 { 00484 const char month_limit[12]={31,28,31,30,31,30,31,31,30,31,30,31}; 00485 if(monthVal == februar && checkLeapyear(yearVal)) 00486 { return true; } 00487 else if(dateVal > 28 && monthVal == februar) 00488 { 00489 ErrorValue = FEB_NO_LEAP; 00490 return false; 00491 } 00492 if(dateVal <= month_limit[monthVal-1] && dateVal >= 1) 00493 { return true; } 00494 else 00495 { return false; } 00496 } 00497 //============================================================================= 00498 00499 00500 //CHECKLEAPYEAR================================================================ 00501 //Autor: Joel von Rotz 00502 //Typ: bool 00503 //parameter: int yearVal 00504 //Beschreibung: 00505 // Es wird überprüft, ob das Jahr ein Schaltjahr ist oder nicht. 00506 // Dabei ist das Jahr NUR ein Schaltjahr, wenn das Jahr durch 4 oder durch 400 00507 // teilbar, aber sobald es durch 100 teilbar ist, ist es kein Schaltjahr. 00508 // Source -> Gregorianischer Kalender 00509 //============================================================================= 00510 #define ISLEAPYEAR 1 00511 #define ISNOTLEAPYEAR 0 00512 bool checkLeapyear(int yearVal) 00513 { 00514 if(yearVal % 4 == 0) 00515 { 00516 if(yearVal % 100 == 0) 00517 { 00518 if(yearVal % 400 == 0) 00519 { 00520 return ISLEAPYEAR; //ist Schaltjahr 00521 } 00522 return ISNOTLEAPYEAR; //ist nicht Schaltjahr 00523 } 00524 return ISLEAPYEAR; //ist Schaltjahr 00525 } 00526 return ISNOTLEAPYEAR; //ist nicht Schaltjahr 00527 } 00528 //============================================================================= 00529 00530 void initArray(int const *letter, int size, char *matrix_value) 00531 { 00532 matrix_frames = matrix_frames + 1; /*A small space, about 1 led column, is being added. */ 00533 00534 for(int position = 0; position < size; position++) 00535 { 00536 /* Hex-values are added to the matrix-text and matrix_frames is incremented */ 00537 matrix_value[matrix_frames] = letter[position]; 00538 matrix_frames++; 00539 } 00540 matrix_value[matrix_frames] = 0x00; 00541 } 00542 00543 //=============================================================================// 00544 //=============================================================================// 00545 void TextMatrixConvert(const string& text_convertion, char *matrix_value, int m_length) 00546 { 00547 matrix_frames = matrix_frames + 64; 00548 for(int LetterPos = 0; LetterPos < text_convertion.size(); LetterPos++) 00549 { 00550 //Letters ------------------------ 00551 if (text_convertion.at(LetterPos) == 'A') 00552 { 00553 initArray(A,4,matrix_value); 00554 } 00555 else if(text_convertion.at(LetterPos) == 'a') 00556 { 00557 initArray(a,4,matrix_value); 00558 } 00559 else if(text_convertion.at(LetterPos) == 'B') 00560 { 00561 initArray(B,4,matrix_value); 00562 } 00563 else if(text_convertion.at(LetterPos) == 'b') 00564 { 00565 initArray(b,4,matrix_value); 00566 } 00567 else if(text_convertion.at(LetterPos) == 'C') 00568 { 00569 initArray(C,4,matrix_value); 00570 } 00571 else if(text_convertion.at(LetterPos) == 'c') 00572 { 00573 initArray(c,4,matrix_value); 00574 } 00575 else if(text_convertion.at(LetterPos) == 'D') 00576 { 00577 initArray(D,4,matrix_value); 00578 } 00579 else if(text_convertion.at(LetterPos) == 'd') 00580 { 00581 initArray(d,5,matrix_value); 00582 } 00583 else if(text_convertion.at(LetterPos) == 'E') 00584 { 00585 initArray(E,4,matrix_value); 00586 } 00587 else if(text_convertion.at(LetterPos) == 'e') 00588 { 00589 initArray(e,4,matrix_value); 00590 } 00591 else if(text_convertion.at(LetterPos) == 'F') 00592 { 00593 initArray(F,4,matrix_value); 00594 } 00595 else if(text_convertion.at(LetterPos) == 'f') 00596 { 00597 initArray(f,3,matrix_value); 00598 } 00599 else if(text_convertion.at(LetterPos) == 'G') 00600 { 00601 initArray(G,4,matrix_value); 00602 } 00603 else if(text_convertion.at(LetterPos) == 'g') 00604 { 00605 initArray(g,4,matrix_value); 00606 } 00607 else if(text_convertion.at(LetterPos) == 'H') 00608 { 00609 initArray(H,4,matrix_value); 00610 } 00611 else if(text_convertion.at(LetterPos) == 'h') 00612 { 00613 initArray(h,5,matrix_value); 00614 } 00615 else if(text_convertion.at(LetterPos) == 'I') 00616 { 00617 initArray(I,3,matrix_value); 00618 } 00619 else if(text_convertion.at(LetterPos) == 'i') 00620 { 00621 initArray(i,3,matrix_value); 00622 } 00623 else if(text_convertion.at(LetterPos) == 'J') 00624 { 00625 initArray(J,4,matrix_value); 00626 } 00627 else if(text_convertion.at(LetterPos) == 'j') 00628 { 00629 initArray(j,3,matrix_value); 00630 } 00631 else if(text_convertion.at(LetterPos) == 'K') 00632 { 00633 initArray(K,5,matrix_value); 00634 } 00635 else if(text_convertion.at(LetterPos) == 'k') 00636 { 00637 initArray(k,4,matrix_value); 00638 } 00639 else if(text_convertion.at(LetterPos) == 'L') 00640 { 00641 initArray(L,4,matrix_value); 00642 } 00643 else if(text_convertion.at(LetterPos) == 'l') 00644 { 00645 initArray(l,3,matrix_value); 00646 } 00647 else if(text_convertion.at(LetterPos) == 'M') 00648 { 00649 initArray(M,5,matrix_value); 00650 } 00651 else if(text_convertion.at(LetterPos) == 'm') 00652 { 00653 initArray(m,5,matrix_value); 00654 } 00655 else if(text_convertion.at(LetterPos) == 'N') 00656 { 00657 initArray(N,5,matrix_value); 00658 } 00659 else if(text_convertion.at(LetterPos) == 'n') 00660 { 00661 initArray(n,4,matrix_value); 00662 } 00663 else if(text_convertion.at(LetterPos) == 'O') 00664 { 00665 initArray(O,4,matrix_value); 00666 } 00667 else if(text_convertion.at(LetterPos) == 'o') 00668 { 00669 initArray(o,5,matrix_value); 00670 } 00671 else if(text_convertion.at(LetterPos) == 'P') 00672 { 00673 initArray(P,4,matrix_value); 00674 } 00675 else if(text_convertion.at(LetterPos) == 'p') 00676 { 00677 initArray(p,4,matrix_value); 00678 } 00679 else if(text_convertion.at(LetterPos) == 'Q') 00680 { 00681 initArray(Q,5,matrix_value); 00682 } 00683 else if(text_convertion.at(LetterPos) == 'q') 00684 { 00685 initArray(q,4,matrix_value); 00686 } 00687 else if(text_convertion.at(LetterPos) == 'R') 00688 { 00689 initArray(R,4,matrix_value); 00690 } 00691 else if(text_convertion.at(LetterPos) == 'r') 00692 { 00693 initArray(r,3,matrix_value); 00694 } 00695 else if(text_convertion.at(LetterPos) == 'S') 00696 { 00697 initArray(S,4,matrix_value); 00698 } 00699 else if(text_convertion.at(LetterPos) == 's') 00700 { 00701 initArray(s,4,matrix_value); 00702 } 00703 else if(text_convertion.at(LetterPos) == 'T') 00704 { 00705 initArray(T,5,matrix_value); 00706 } 00707 else if(text_convertion.at(LetterPos) == 't') 00708 { 00709 initArray(t,3,matrix_value); 00710 } 00711 else if(text_convertion.at(LetterPos) == 'U') 00712 { 00713 initArray(U,4,matrix_value); 00714 } 00715 else if(text_convertion.at(LetterPos) == 'u') 00716 { 00717 initArray(u,5,matrix_value); 00718 } 00719 else if(text_convertion.at(LetterPos) == 'V') 00720 { 00721 initArray(V,5,matrix_value); 00722 } 00723 else if(text_convertion.at(LetterPos) == 'v') 00724 { 00725 initArray(v,5,matrix_value); 00726 } 00727 else if(text_convertion.at(LetterPos) == 'W') 00728 { 00729 initArray(W,5,matrix_value); 00730 } 00731 else if(text_convertion.at(LetterPos) == 'w') 00732 { 00733 initArray(w,5,matrix_value); 00734 } 00735 else if(text_convertion.at(LetterPos) == 'X') 00736 { 00737 initArray(X,4,matrix_value); 00738 } 00739 else if(text_convertion.at(LetterPos) == 'x') 00740 { 00741 initArray(x,4,matrix_value); 00742 } 00743 else if(text_convertion.at(LetterPos) == 'Y') 00744 { 00745 initArray(Y,5,matrix_value); 00746 } 00747 else if(text_convertion.at(LetterPos) == 'y') 00748 { 00749 initArray(y,5,matrix_value); 00750 } 00751 else if(text_convertion.at(LetterPos) == 'Z') 00752 { 00753 initArray(Z,4,matrix_value); 00754 } 00755 else if(text_convertion.at(LetterPos) == 'z') 00756 { 00757 initArray(z,4,matrix_value); 00758 } 00759 // Numbers ----------------------- 00760 else if(text_convertion.at(LetterPos) == '0') 00761 { 00762 initArray(zero,4,matrix_value); 00763 } 00764 else if(text_convertion.at(LetterPos) == '1') 00765 { 00766 initArray(one,4,matrix_value); 00767 } 00768 else if(text_convertion.at(LetterPos) == '2') 00769 { 00770 initArray(two,4,matrix_value); 00771 } 00772 else if(text_convertion.at(LetterPos) == '3') 00773 { 00774 initArray(three,4,matrix_value); 00775 } 00776 else if(text_convertion.at(LetterPos) == '4') 00777 { 00778 initArray(four,4,matrix_value); 00779 } 00780 else if(text_convertion.at(LetterPos) == '5') 00781 { 00782 initArray(five,4,matrix_value); 00783 } 00784 else if(text_convertion.at(LetterPos) == '6') 00785 { 00786 initArray(six,4,matrix_value); 00787 } 00788 else if(text_convertion.at(LetterPos) == '7') 00789 { 00790 initArray(seven,4,matrix_value); 00791 } 00792 else if(text_convertion.at(LetterPos) == '8') 00793 { 00794 initArray(eight,4,matrix_value); 00795 } 00796 else if(text_convertion.at(LetterPos) == '9') 00797 { 00798 initArray(nine,4,matrix_value); 00799 } 00800 // Symbols ----------------------- 00801 else if(text_convertion.at(LetterPos) == '!') 00802 { 00803 initArray(exclam,1,matrix_value); 00804 } 00805 else if(text_convertion.at(LetterPos) == '?') 00806 { 00807 initArray(quest,3,matrix_value); 00808 } 00809 else if(text_convertion.at(LetterPos) == 34) 00810 { 00811 initArray(quote,3,matrix_value); 00812 } 00813 else if(text_convertion.at(LetterPos) == '#') 00814 { 00815 initArray(hash,5,matrix_value); 00816 } 00817 else if(text_convertion.at(LetterPos) == '$') 00818 { 00819 initArray(dollar,5,matrix_value); 00820 } 00821 else if(text_convertion.at(LetterPos) == '%') 00822 { 00823 initArray(prcent,7,matrix_value); 00824 } 00825 else if(text_convertion.at(LetterPos) == '&') 00826 { 00827 initArray(_and,5,matrix_value); 00828 } 00829 else if(text_convertion.at(LetterPos) == '(') 00830 { 00831 initArray(round_o,2,matrix_value); 00832 } 00833 else if(text_convertion.at(LetterPos) == ')') 00834 { 00835 initArray(round_c,2,matrix_value); 00836 } 00837 else if(text_convertion.at(LetterPos) == '*') 00838 { 00839 initArray(star,5,matrix_value); 00840 } 00841 else if(text_convertion.at(LetterPos) == '+') 00842 { 00843 initArray(plus,5,matrix_value); 00844 } 00845 else if(text_convertion.at(LetterPos) == '-') 00846 { 00847 initArray(minus,3,matrix_value); 00848 } 00849 else if(text_convertion.at(LetterPos) == '=') 00850 { 00851 initArray(_equal,4,matrix_value); 00852 } 00853 else if(text_convertion.at(LetterPos) == ',') 00854 { 00855 initArray(comma,2,matrix_value); 00856 } 00857 else if(text_convertion.at(LetterPos) == '.') 00858 { 00859 initArray(point,1,matrix_value); 00860 } 00861 else if(text_convertion.at(LetterPos) == '/') 00862 { 00863 initArray(slash,5,matrix_value); 00864 } 00865 else if(text_convertion.at(LetterPos) == 58) 00866 { 00867 initArray(d_point,1,matrix_value); 00868 } 00869 else if(text_convertion.at(LetterPos) == 59) 00870 { 00871 initArray(poicom,2,matrix_value); 00872 } 00873 else if(text_convertion.at(LetterPos) == 95) 00874 { 00875 initArray(undlin,4,matrix_value); 00876 } 00877 else if(text_convertion.at(LetterPos) == 92) 00878 { 00879 initArray(b_slash,5,matrix_value); 00880 } 00881 else if(text_convertion.at(LetterPos) == 64) 00882 { 00883 initArray(at,5,matrix_value); 00884 } 00885 else if(text_convertion.at(LetterPos) == 62) 00886 { 00887 initArray(more,4,matrix_value); 00888 } 00889 else if(text_convertion.at(LetterPos) == 60) 00890 { 00891 initArray(less,4,matrix_value); 00892 } 00893 else if(text_convertion.at(LetterPos) == '[') 00894 { 00895 initArray(brack_o,2,matrix_value); 00896 } 00897 else if(text_convertion.at(LetterPos) == ']') 00898 { 00899 initArray(brack_c,2,matrix_value); 00900 } 00901 else if(text_convertion.at(LetterPos) == 94) 00902 { 00903 initArray(roof,3,matrix_value); 00904 } 00905 else if(text_convertion.at(LetterPos) == '{') 00906 { 00907 initArray(brace_o,3,matrix_value); 00908 } 00909 else if(text_convertion.at(LetterPos) == '}') 00910 { 00911 initArray(brace_c,3,matrix_value); 00912 } 00913 else if(text_convertion.at(LetterPos) == '~') 00914 { 00915 initArray(wave,4,matrix_value); 00916 } 00917 else if(text_convertion.at(LetterPos) == '|') 00918 { 00919 initArray(stick,1,matrix_value); 00920 } 00921 else if(text_convertion.at(LetterPos) == '`') 00922 { 00923 initArray(frapo,2,matrix_value); 00924 } 00925 else if(text_convertion.at(LetterPos) == ' ') 00926 { 00927 initArray(space,3,matrix_value); 00928 } 00929 } 00930 } 00931 00932 //============================================================================================ 00933 //▄▄▄ ▄▄ ▄▄ ▄▄ ▄▄ ▄ 00934 //█ █ █ ▀ ▀ █ ▀ █ ▀ █ ▀█ 00935 //█ █ ▀▄▄ ▄▄▀ ▄▄▀ ▄▄▀ █ 00936 //█ █ █ █ █ █ █ 00937 //█▄▄▀ ▀▄▄▀ ▀▄▄▀ ▀▄▄▀ ▀▄▄▀ ▄█▄
Generated on Sat Jul 23 2022 07:37:33 by
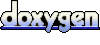