
Wi-Fi追加
Dependencies: RemoteIR TextLCD
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2019 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 #include "mbed.h" 00007 #include "ReceiverIR.h" 00008 #include "rtos.h" 00009 #include <stdint.h> 00010 #include "platform/mbed_thread.h" 00011 #include "TextLCD.h" 00012 00013 Serial pc(USBTX, USBRX); 00014 00015 /* マクロ定義、列挙型定義 */ 00016 #define MIN_V 2.0 // 電圧の最小値 00017 #define MAX_V 2.67 // 電圧の最大値 00018 #define LOW 0 // モーターOFF 00019 #define HIGH 1 // モーターON 00020 #define NORMAL 0 // 普通 00021 #define FAST 1 // 速い 00022 #define VERYFAST 2 // とても速い 00023 00024 /* 操作モード定義 */ 00025 enum MODE{ 00026 READY = -1, // -1:待ち 00027 ADVANCE = 1, // 1:前進 00028 RIGHT, // 2:右折 00029 LEFT, // 3:左折 00030 BACK, // 4:後退 00031 STOP, // 5:停止 00032 LINE_TRACE, // 6:ライントレース 00033 AVOIDANCE, // 7:障害物回避 00034 SPEED, // 8:スピード制御 00035 }; 00036 00037 /* ピン配置 */ 00038 ReceiverIR ir(p5); // リモコン操作 00039 DigitalOut trig(p6); // 超音波センサtrigger 00040 DigitalIn echo(p7); // 超音波センサecho 00041 DigitalIn ss1(p8); // ライントレースセンサ(左) 00042 DigitalIn ss2(p9); // ライントレースセンサ 00043 DigitalIn ss3(p10); // ライントレースセンサ 00044 DigitalIn ss4(p11); // ライントレースセンサ 00045 DigitalIn ss5(p12); // ライントレースセンサ(右) 00046 RawSerial esp(p13, p14); // Wi-Fiモジュール(tx, rx) 00047 AnalogIn battery(p15); // 電池残量読み取り(Max 3.3V) 00048 PwmOut motorR2(p21); // 右モーター後退 00049 PwmOut motorR1(p22); // 右モーター前進 00050 PwmOut motorL2(p23); // 左モーター後退 00051 PwmOut motorL1(p24); // 左モーター前進 00052 PwmOut servo(p25); // サーボ 00053 I2C i2c_lcd(p28,p27); // LCD(tx, rx) 00054 00055 /* 変数宣言 */ 00056 int mode; // 操作モード 00057 int run; // 走行状態 00058 int beforeMode; // 前回のモード 00059 int flag_sp = 0; // スピード変化フラグ 00060 Timer viewTimer; // スピ―ド変更時に3秒計測タイマー 00061 float motorSpeed[9] = {0.5, 0.8, 0.9, 0.75, 0.85, 0.95, 0.8, 0.9, 1.0}; 00062 // モーター速度設定(後半はライントレース用) 00063 00064 Mutex mutex; // ミューテックス 00065 00066 /* decodeIR用変数 */ 00067 RemoteIR::Format format; 00068 uint8_t buf[32]; 00069 uint32_t bitcount; 00070 uint32_t code; 00071 00072 /* bChange, lcdbacklight用変数 */ 00073 TextLCD_I2C lcd(&i2c_lcd, (0x27 << 1), TextLCD::LCD16x2, TextLCD::HD44780); 00074 int b = 0; // バッテリー残量 00075 int flag_b = 0; // バックライト点滅フラグ 00076 int flag_t = 0; // バックライトタイマーフラグ 00077 00078 /* trace用変数 */ 00079 int sensArray[32] = {8,6,2,4,1,1,2,2, // ライントレースセンサパターン 00080 3,1,1,1,3,1,1,2, 00081 7,1,1,1,1,1,1,1, 00082 5,1,1,1,3,1,3,1}; 00083 00084 /* avoidance用変数 */ 00085 Timer timer; // 距離計測用タイマ 00086 int DT; // 距離 00087 int SC; // 正面 00088 int SL; // 左 00089 int SR; // 右 00090 int SLD; // 左前 00091 int SRD; // 右前 00092 int flag_a = 0; // 障害物有無のフラグ 00093 const int limit = 20; // 障害物の距離のリミット(単位:cm) 00094 int far; // 最も遠い距離 00095 int houkou; // 進行方向(1:前 2:左 3:右) 00096 int i; // ループ変数 00097 int t1,t2=0; 00098 00099 /*WiFi用変数*/ 00100 Timer time1; 00101 Timer time2; 00102 int bufflen, DataRX, ount, getcount, replycount, servreq, timeout; 00103 int bufl, ipdLen, linkID, weberror, webcounter,click_flag; 00104 float temperature, AdcIn, Ht; 00105 float R1=100000, R2=10000; // resistor values to give a 10:1 reduction of measured AnalogIn voltage 00106 char Vcc[10]; 00107 char webcount[8]; 00108 char type[16]; 00109 char type1[16]; 00110 char channel[2]; 00111 char cmdbuff[32]; 00112 char replybuff[1024]; 00113 char webdata[1024]; // This may need to be bigger depending on WEB browser used 00114 char webbuff[4096*4]; // Currently using 1986 characters, Increase this if more web page data added 00115 int port =80; // set server port 00116 int SERVtimeout =5; // set server timeout in seconds in case link breaks. 00117 char ssid[32] = "mbed02"; // enter WiFi router ssid inside the quotes 00118 char pwd [32] = "0123456789a"; // enter WiFi router password inside the quotes 00119 00120 00121 /* プロトタイプ宣言 */ 00122 void decodeIR(void const *argument); 00123 void motor(void const *argument); 00124 void changeSpeed(); 00125 void avoidance(/*void const *argument*/); 00126 void trace(/*void const *argument*/); 00127 void watchsurrounding(); 00128 int watch(); 00129 void bChange(); 00130 void display(); 00131 void lcdBacklight(void const *argument); 00132 void SendCMD(),getreply(),ReadWebData(),startserver(),sendpage(),SendWEB(),sendcheck(),touchuan(); 00133 void wifi(void const *argument); 00134 Thread deco_thread(decodeIR, NULL, osPriorityRealtime); // decodeIRをスレッド化 :+3 00135 Thread wifi_thread(wifi,NULL,osPriorityRealtime); // wifiをスレッド化 00136 Thread motor_thread(motor, NULL, osPriorityHigh); // motorをスレッド化 :+2 00137 //Thread avoi_thread(avoidance, NULL, osPriorityHigh); // avoidanceをスレッド化:+2 00138 //Thread trace_thread(trace, NULL, osPriorityHigh); // traceをスレッド化 :+2 00139 RtosTimer bTimer(lcdBacklight, osTimerPeriodic); // lcdBacklightをタイマー割り込みで設定 00140 Thread *avoi_thread; 00141 Thread *trace_thread; 00142 00143 DigitalOut led1(LED1); 00144 DigitalOut led2(LED2); 00145 DigitalOut led3(LED3); 00146 DigitalOut led4(LED4); 00147 00148 /* リモコン受信スレッド */ 00149 void decodeIR(void const *argument){ 00150 while(1){ 00151 // 受信待ち 00152 if (ir.getState() == ReceiverIR::Received){ // コード受信 00153 bitcount = ir.getData(&format, buf, sizeof(buf) * 8); 00154 if(bitcount > 1){ // 受信成功 00155 code=0; 00156 for(int j = 0; j < 4; j++){ 00157 code+=(buf[j]<<(8*(3-j))); 00158 } 00159 if(mode != SPEED){ // スピードモード以外なら 00160 beforeMode=mode; // 前回のモードに現在のモードを設定 00161 } 00162 switch(code){ 00163 case 0x40bf27d8: // クイック 00164 //pc.printf("mode = SPEED\r\n"); 00165 mode = SPEED; // スピードモード 00166 changeSpeed(); // 速度変更 00167 display(); // ディスプレイ表示 00168 mode = beforeMode; // 現在のモードに前回のモードを設定 00169 break; 00170 case 0x40be34cb: // レグザリンク 00171 //pc.printf("mode = LINE_TRACE\r\n"); 00172 if(trace_thread->get_state() == Thread::Deleted){ 00173 delete trace_thread; 00174 trace_thread = new Thread(trace); 00175 trace_thread -> set_priority(osPriorityHigh); 00176 } 00177 mode=LINE_TRACE; // ライントレースモード 00178 display(); // ディスプレイ表示 00179 break; 00180 case 0x40bf6e91: // 番組表 00181 //pc.printf("mode = AVOIDANCE\r\n"); 00182 if(avoi_thread->get_state() == Thread::Deleted){ 00183 delete avoi_thread; 00184 avoi_thread = new Thread(avoidance); 00185 avoi_thread -> set_priority(osPriorityHigh); 00186 } 00187 flag_a = 0; 00188 mode=AVOIDANCE; // 障害物回避モード 00189 run = ADVANCE; // 前進 00190 display(); // ディスプレイ表示 00191 break; 00192 case 0x40bf3ec1: // ↑ 00193 //pc.printf("mode = ADVANCE\r\n"); 00194 mode = ADVANCE; // 前進モード 00195 run = ADVANCE; // 前進 00196 display(); // ディスプレイ表示 00197 break; 00198 case 0x40bf3fc0: // ↓ 00199 //pc.printf("mode = BACK\r\n"); 00200 mode = BACK; // 後退モード 00201 run = BACK; // 後退 00202 display(); // ディスプレイ表示 00203 break; 00204 case 0x40bf5fa0: // ← 00205 //pc.printf("mode = LEFT\r\n"); 00206 mode = LEFT; // 左折モード 00207 run = LEFT; // 左折 00208 display(); // ディスプレイ表示 00209 break; 00210 case 0x40bf5ba4: // → 00211 //pc.printf("mode = RIGHT\r\n"); 00212 mode = RIGHT; // 右折モード 00213 run = RIGHT; // 右折 00214 display(); // ディスプレイ表示 00215 break; 00216 case 0x40bf3dc2: // 決定 00217 //pc.printf("mode = STOP\r\n"); 00218 mode = STOP; // 停止モード 00219 run = STOP; // 停止 00220 display(); // ディスプレイ表示 00221 break; 00222 default: 00223 ; 00224 } 00225 if(mode != LINE_TRACE && trace_thread->get_state() != Thread::Deleted){ 00226 trace_thread->terminate(); 00227 } 00228 if(mode != AVOIDANCE && avoi_thread->get_state() != Thread::Deleted){ 00229 avoi_thread->terminate(); 00230 } 00231 } 00232 } 00233 if(viewTimer.read_ms()>=3000){ // スピードモードのまま3秒経過 00234 viewTimer.stop(); // タイマーストップ 00235 viewTimer.reset(); // タイマーリセット 00236 display(); // ディスプレイ表示 00237 } 00238 ThisThread::sleep_for(90); // 90ms待つ 00239 } 00240 } 00241 00242 /* モーター制御スレッド */ 00243 void motor(void const *argument){ 00244 while(1){ 00245 /* 走行状態の場合分け */ 00246 switch(run){ 00247 /* 前進 */ 00248 case ADVANCE: 00249 motorR1 = motorSpeed[flag_sp]; // 右前進モーターON 00250 motorR2 = LOW; // 右後退モーターOFF 00251 motorL1 = motorSpeed[flag_sp]; // 左前進モーターON 00252 motorL2 = LOW; // 左後退モーターOFF 00253 break; 00254 /* 右折 */ 00255 case RIGHT: 00256 motorR1 = LOW; // 右前進モーターOFF 00257 motorR2 = motorSpeed[flag_sp]; // 右後退モーターON 00258 motorL1 = motorSpeed[flag_sp]; // 左前進モーターON 00259 motorL2 = LOW; // 左後退モーターOFF 00260 break; 00261 /* 左折 */ 00262 case LEFT: 00263 motorR1 = motorSpeed[flag_sp]; // 右前進モーターON 00264 motorR2 = LOW; // 右後退モーターOFF 00265 motorL1 = LOW; // 左前進モーターOFF 00266 motorL2 = motorSpeed[flag_sp]; // 左後退モーターON 00267 break; 00268 /* 後退 */ 00269 case BACK: 00270 motorR1 = LOW; // 右前進モーターOFF 00271 motorR2 = motorSpeed[flag_sp]; // 右後退モーターON 00272 motorL1 = LOW; // 左前進モーターOFF 00273 motorL2 = motorSpeed[flag_sp]; // 左後退モーターON 00274 break; 00275 /* 停止 */ 00276 case STOP: 00277 motorR1 = LOW; // 右前進モーターOFF 00278 motorR2 = LOW; // 右後退モーターOFF 00279 motorL1 = LOW; // 左前進モーターOFF 00280 motorL2 = LOW; // 左後退モーターOFF 00281 break; 00282 } 00283 if(flag_sp > VERYFAST){ // スピード変更フラグが2より大きいなら 00284 flag_sp -= 3 * (flag_sp / 3); // スピード変更フラグ調整 00285 } 00286 ThisThread::sleep_for(30); // 30ms待つ 00287 } 00288 } 00289 00290 /* スピード変更関数 */ 00291 void changeSpeed(){ 00292 if(flag_sp%3 == 2){ // スピード変更フラグを3で割った余りが2なら 00293 flag_sp -= 2; // スピード変更フラグを-2 00294 00295 }else{ // それ以外 00296 flag_sp = flag_sp + 1; // スピード変更フラグを+1 00297 } 00298 } 00299 00300 /* ライントレーススレッド */ 00301 void trace(){ 00302 while(1){ 00303 /* 各センサー値読み取り */ 00304 int sensor1 = ss1; 00305 int sensor2 = ss2; 00306 int sensor3 = ss3; 00307 int sensor4 = ss4; 00308 int sensor5 = ss5; 00309 pc.printf("%d %d %d %d %d \r\n",sensor1,sensor2,sensor3,sensor4,sensor5); 00310 int sensD = 0; 00311 int sensorNum; 00312 00313 /* センサー値の決定 */ 00314 if(sensor1 > 0) sensD |= 0x10; 00315 if(sensor2 > 0) sensD |= 0x08; 00316 if(sensor3 > 0) sensD |= 0x04; 00317 if(sensor4 > 0) sensD |= 0x02; 00318 if(sensor5 > 0) sensD |= 0x01; 00319 00320 sensorNum = sensArray[sensD]; 00321 00322 /* センサー値によって場合分け */ 00323 switch(sensorNum){ 00324 case 1: 00325 run = ADVANCE; // 低速で前進 00326 break; 00327 case 2: 00328 flag_sp = flag_sp % 3 + 6; 00329 run = RIGHT; // 低速で右折 00330 break; 00331 case 3: 00332 flag_sp = flag_sp % 3 + 6; 00333 run = LEFT; // 低速で左折 00334 break; 00335 case 4: 00336 flag_sp = flag_sp % 3 + 6; 00337 run = RIGHT; // 中速で右折 00338 break; 00339 case 5: 00340 flag_sp = flag_sp % 3 + 6; 00341 run = LEFT; // 中速で左折 00342 break; 00343 case 6: 00344 flag_sp = flag_sp % 3 + 6; 00345 run = RIGHT; // 高速で右折 00346 break; 00347 case 7: 00348 flag_sp = flag_sp % 3 + 6; 00349 run = LEFT; // 高速で左折 00350 break; 00351 case 8: 00352 break; 00353 } 00354 ThisThread::sleep_for(30); // 30ms待つ 00355 } 00356 } 00357 00358 /* 障害物回避走行スレッド */ 00359 void avoidance(){ 00360 while(1){ 00361 watchsurrounding(); 00362 pc.printf("%d %d %d %d %d \r\n",SL,SLD,SC,SRD,SR); 00363 if(flag_a == 0){ // 障害物がない場合 00364 run = ADVANCE; // 前進 00365 } 00366 else{ // 障害物がある場合 00367 i = 0; 00368 if(SC < 15){ // 正面15cm以内に障害物が現れた場合 00369 run = BACK; // 後退 00370 while(watch() < limit){ // 正面20cm以内に障害物がある間 00371 } 00372 run = STOP; // 停止 00373 } 00374 if(SC < limit && SLD < limit && SL < limit && SRD < limit && SR < limit){ // 全方向に障害物がある場合 00375 run = LEFT; // 左折 00376 while(i < 3){ // 進行方向確認 00377 if(watch() > limit){ 00378 i++; 00379 } 00380 else{ 00381 i = 0; 00382 } 00383 } 00384 run = STOP; // 停止 00385 } 00386 else { // 全方向以外 00387 far = SC; // 正面を最も遠い距離に設定 00388 houkou = 1; // 進行方向を前に設定 00389 if(far < SLD || far < SL){ // 左または左前がより遠い場合 00390 if(SL < SLD){ // 左前が左より遠い場合 00391 far = SLD; // 左前を最も遠い距離に設定 00392 } 00393 else{ // 左が左前より遠い場合 00394 far = SL; // 左を最も遠い距離に設定 00395 } 00396 houkou = 2; // 進行方向を左に設定 00397 } 00398 if(far < SRD || far < SR){ // 右または右前がより遠い場合 00399 if(SR < SRD){ // 右前が右より遠い場合 00400 far = SRD; // 右前を最も遠い距離に設定 00401 } 00402 else{ // 右が右前よりも遠い場合 00403 far = SR; // 右を最も遠い距離に設定 00404 } 00405 houkou = 3; // 進行方向を右に設定 00406 } 00407 switch(houkou){ // 進行方向の場合分け 00408 case 1: // 前の場合 00409 run = ADVANCE; // 前進 00410 ThisThread::sleep_for(1000); // 1秒待つ 00411 break; 00412 case 2: // 左の場合 00413 run = LEFT; // 左折 00414 while(i < 3){ // 進行方向確認 00415 if(watch() > (far - 2)){ // 正面の計測距離と最も遠い距離が一致したら(誤差-2cm) 00416 i++; // ループ+ 00417 } 00418 else{ 00419 i = 0; 00420 } 00421 } 00422 run = STOP; // 停止 00423 break; 00424 case 3: // 右の場合 00425 run = RIGHT; // 右折 00426 while(i < 3){ // 進行方向確認 00427 if(watch() > (far - 2)){ // 正面の計測距離と最も遠い距離が一致したら(誤差-2cm) 00428 i++; // ループ+ 00429 } 00430 else{ 00431 i = 0; 00432 } 00433 } 00434 run = STOP; // 停止 00435 break; 00436 } 00437 } 00438 } 00439 flag_a = 0; // 障害物有無フラグを0にセット 00440 } 00441 } 00442 00443 /* 距離計測関数 */ 00444 int watch(){ 00445 do{ 00446 trig = 0; 00447 ThisThread::sleep_for(5); // 5ms待つ 00448 trig = 1; 00449 ThisThread::sleep_for(15); // 15ms待つ 00450 trig = 0; 00451 timer.start(); 00452 t1=timer.read_ms(); 00453 t2=timer.read_ms(); 00454 while(echo.read() == 0 &&(t1-t2)<10){ 00455 t1=timer.read_ms(); 00456 led1 = 1; 00457 } 00458 timer.stop(); 00459 timer.reset(); 00460 if((t1-t2) >= 10){ 00461 run = STOP; 00462 } 00463 }while((t1-t2) >= 10); 00464 timer.start(); // 距離計測タイマースタート 00465 while(echo.read() == 1){ 00466 } 00467 timer.stop(); // 距離計測タイマーストップ 00468 DT = (int)(timer.read_us()*0.01657); // 距離計算 00469 if(DT > 150){ // 検知範囲外なら100cmに設定 00470 DT = 150; 00471 } 00472 timer.reset(); // 距離計測タイマーリセット 00473 led1 = 0; 00474 return DT; 00475 } 00476 00477 /* 障害物検知関数 */ 00478 void watchsurrounding(){ 00479 servo.pulsewidth_us(1450); // サーボを中央位置に戻す 00480 ThisThread::sleep_for(90); // 100ms待つ 00481 SC = watch(); 00482 if(SC < limit){ // 正面20cm以内に障害物がある場合 00483 run = STOP; // 停止 00484 } 00485 servo.pulsewidth_us(1925); // サーボを左に40度回転 00486 ThisThread::sleep_for(200); // 250ms待つ 00487 SLD = watch(); 00488 if(SLD < limit){ // 左前20cm以内に障害物がある場合 00489 run = STOP; // 停止 00490 } 00491 servo.pulsewidth_us(2400); // サーボを左に90度回転 00492 ThisThread::sleep_for(200); // 250ms待つ 00493 SL = watch(); 00494 if(SL < limit){ // 左20cm以内に障害物がある場合 00495 run = STOP; // 停止 00496 } 00497 servo.pulsewidth_us(1450); 00498 ThisThread::sleep_for(90); 00499 SC = watch(); 00500 if(SC < limit){ 00501 run = STOP; 00502 } 00503 servo.pulsewidth_us(925); // サーボを右に40度回転 00504 ThisThread::sleep_for(200); // 250ms待つ 00505 SRD = watch(); 00506 if(SRD < limit){ // 右前20cm以内に障害物がある場合 00507 run = STOP; // 停止 00508 } 00509 servo.pulsewidth_us(500); // サーボを右に90度回転 00510 ThisThread::sleep_for(200); // 250ms待つ 00511 SR = watch(); 00512 if(SR < limit){ // 右20cm以内に障害物がある場合 00513 run = STOP; // 停止 00514 } 00515 servo.pulsewidth_us(1450); // サーボを中央位置に戻す 00516 ThisThread::sleep_for(80); // 100ms待つ 00517 if(SC < limit || SLD < limit || SL < limit || SRD < limit || SR < limit){ // 20cm以内に障害物を検知した場合 00518 flag_a = 1; // 障害物有無フラグに1をセット 00519 } 00520 } 00521 00522 /* ディスプレイ表示関数 */ 00523 void display(){ 00524 mutex.lock(); // ミューテックスロック 00525 lcd.setAddress(0,1); 00526 00527 /* 操作モードによる場合分け */ 00528 switch(mode){ 00529 /* 前進 */ 00530 case ADVANCE: 00531 lcd.printf("Mode:Advance "); 00532 break; 00533 /* 右折 */ 00534 case RIGHT: 00535 lcd.printf("Mode:TurnRight "); 00536 break; 00537 /* 左折 */ 00538 case LEFT: 00539 lcd.printf("Mode:TurnLeft "); 00540 break; 00541 /* 後退 */ 00542 case BACK: 00543 lcd.printf("Mode:Back "); 00544 break; 00545 /* 停止 */ 00546 case STOP: 00547 lcd.printf("Mode:Stop "); 00548 break; 00549 /* 待ち */ 00550 case READY: 00551 lcd.printf("Mode:Ready "); 00552 break; 00553 /* ライントレース */ 00554 case LINE_TRACE: 00555 lcd.printf("Mode:LineTrace "); 00556 break; 00557 /* 障害物回避 */ 00558 case AVOIDANCE: 00559 lcd.printf("Mode:Avoidance "); 00560 break; 00561 /* スピード制御 */ 00562 case SPEED: 00563 /* スピードの状態で場合分け */ 00564 switch(flag_sp){ 00565 /* 普通 */ 00566 case(NORMAL): 00567 lcd.printf("Speed:Normal "); 00568 break; 00569 /* 速い */ 00570 case(FAST): 00571 lcd.printf("Speed:Fast "); 00572 break; 00573 /* とても速い */ 00574 case(VERYFAST): 00575 lcd.printf("Speed:VeryFast "); 00576 break; 00577 } 00578 viewTimer.reset(); // タイマーリセット 00579 viewTimer.start(); // タイマースタート 00580 break; 00581 } 00582 mutex.unlock(); // ミューテックスアンロック 00583 } 00584 00585 /* バックライト点滅 */ 00586 void lcdBacklight(void const *argument){ 00587 if(flag_b == 1){ // バックライト点滅フラグが1なら 00588 lcd.setBacklight(TextLCD::LightOn); // バックライトON 00589 }else{ // バックライト点滅フラグが0なら 00590 lcd.setBacklight(TextLCD::LightOff); // バックライトOFF 00591 } 00592 flag_b = !flag_b; // バックライト点滅フラグ切り替え 00593 } 00594 00595 /* バッテリー残量更新関数 */ 00596 void bChange(){ 00597 //pc.printf(" bChange1\r\n"); 00598 b = (int)(((battery.read() * 3.3 - MIN_V)/0.67)*10+0.5)*10; 00599 if(b <= 0){ // バッテリー残量0%なら全ての機能停止 00600 b = 0; 00601 //lcd.setBacklight(TextLCD::LightOff); 00602 //run = STOP; 00603 //exit(1); // 電池残量が5%未満の時、LCDを消灯し、モーターを停止し、プログラムを終了する。 00604 } 00605 mutex.lock(); // ミューテックスロック 00606 lcd.setAddress(0,0); 00607 lcd.printf("Battery:%3d%%",b); // バッテリー残量表示 00608 mutex.unlock(); // ミューテックスアンロック 00609 if(b <= 30){ // バッテリー残量30%以下なら 00610 if(flag_t == 0){ // バックライトタイマーフラグが0なら 00611 bTimer.start(500); // 0.5秒周期でバックライトタイマー割り込み 00612 flag_t = 1; // バックライトタイマーフラグを1に切り替え 00613 } 00614 }else{ 00615 if(flag_t == 1){ // バックライトタイマーフラグが1なら 00616 bTimer.stop(); // バックライトタイマーストップ 00617 lcd.setBacklight(TextLCD::LightOn); // バックライトON 00618 flag_t = 0; // バックライトタイマーフラグを0に切り替え 00619 } 00620 } 00621 } 00622 00623 // Serial Interrupt read ESP data 00624 void callback() 00625 { 00626 //pc.printf("\n\r------------ callback is being called --------------\n\r"); 00627 led3=1; 00628 while (esp.readable()) { 00629 webbuff[ount] = esp.getc(); 00630 ount++; 00631 } 00632 if(strlen(webbuff)>bufflen) { 00633 pc.printf("\f\n\r------------ webbuff over bufflen --------------\n\r"); 00634 DataRX=1; 00635 led3=0; 00636 } 00637 } 00638 00639 void wifi(void const *argument) 00640 { 00641 pc.printf("\f\n\r------------ ESP8266 Hardware Reset psq --------------\n\r"); 00642 ThisThread::sleep_for(500); 00643 led1=1,led2=0,led3=0; 00644 timeout=6000; 00645 getcount=500; 00646 getreply(); 00647 esp.baud(115200); // ESP8266 baudrate. Maximum on KLxx' is 115200, 230400 works on K20 and K22F 00648 startserver(); 00649 00650 while(1) { 00651 if(DataRX==1) { 00652 pc.printf("\f\n\r------------ main while > if --------------\n\r"); 00653 click_flag = 1; 00654 ReadWebData(); 00655 pc.printf("\f\n\r------------ click_flag=%d --------------\n\r",click_flag); 00656 //if ((servreq == 1 && weberror == 0) && click_flag == 1) { 00657 if (servreq == 1 && weberror == 0) { 00658 pc.printf("\f\n\r------------ befor send page --------------\n\r"); 00659 sendpage(); 00660 } 00661 pc.printf("\f\n\r------------ send_check begin --------------\n\r"); 00662 00663 //sendcheck(); 00664 pc.printf("\f\n\r------------ ssend_check end--------------\n\r"); 00665 00666 esp.attach(&callback); 00667 pc.printf(" IPD Data:\r\n\n Link ID = %d,\r\n IPD Header Length = %d \r\n IPD Type = %s\r\n", linkID, ipdLen, type); 00668 pc.printf("\n\n HTTP Packet: \n\n%s\n", webdata); 00669 pc.printf(" Web Characters sent : %d\n\n", bufl); 00670 pc.printf(" -------------------------------------\n\n"); 00671 servreq=0; 00672 } 00673 ThisThread::sleep_for(100); 00674 } 00675 } 00676 // Static WEB page 00677 void sendpage() 00678 { 00679 // WEB page data 00680 00681 strcpy(webbuff, "<!DOCTYPE html>"); 00682 strcat(webbuff, "<html><head><title>RobotCar</title><meta name='viewport' content='width=device-width'/>"); 00683 strcat(webbuff, "<style type=\"text/css\">.noselect{ width:100px;height:60px;}.light{ width:100px;height:60px;background-color:#00ff66;}</style>"); 00684 strcat(webbuff, "</head><body><center><p><strong>Robot Car Remote Controller"); 00685 strcat(webbuff, "</strong></p><td style='vertical-align:top;'><strong>Battery level "); 00686 strcat(webbuff, "<input type=\"text\" id=\"leftms\" size=4 value=250>%</strong>"); 00687 strcat(webbuff, "</td></p>"); 00688 strcat(webbuff, "<br>"); 00689 strcat(webbuff, "<table><tr><td></td><td>"); 00690 00691 switch(mode) { 00692 case ADVANCE: 00693 strcat(webbuff, "<button id='gobtn' type='button' class=\"light\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00694 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00695 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00696 strcat(webbuff, "</button></td><td>"); 00697 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00698 strcat(webbuff, "</button></td><td>"); 00699 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00700 strcat(webbuff, "</button></td></tr><td></td><td>"); 00701 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00702 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00703 strcat(webbuff, "<strong>Mode</strong>"); 00704 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00705 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00706 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00707 break; 00708 case LEFT: 00709 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00710 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00711 strcat(webbuff, "<button id='leftbtn' type='button' class=\"light\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00712 strcat(webbuff, "</button></td><td>"); 00713 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00714 strcat(webbuff, "</button></td><td>"); 00715 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00716 strcat(webbuff, "</button></td></tr><td></td><td>"); 00717 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00718 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00719 strcat(webbuff, "<strong>Mode</strong>"); 00720 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00721 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00722 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00723 break; 00724 case STOP: 00725 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00726 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00727 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00728 strcat(webbuff, "</button></td><td>"); 00729 strcat(webbuff, "<button id='stopbtn' type='button' class=\"light\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00730 strcat(webbuff, "</button></td><td>"); 00731 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00732 strcat(webbuff, "</button></td></tr><td></td><td>"); 00733 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00734 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00735 strcat(webbuff, "<strong>Mode</strong>"); 00736 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00737 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00738 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00739 break; 00740 case RIGHT: 00741 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00742 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00743 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00744 strcat(webbuff, "</button></td><td>"); 00745 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00746 strcat(webbuff, "</button></td><td>"); 00747 strcat(webbuff, "<button id='rightbtn' type='button' class=\"light\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00748 strcat(webbuff, "</button></td></tr><td></td><td>"); 00749 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00750 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00751 strcat(webbuff, "<strong>Mode</strong>"); 00752 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00753 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00754 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00755 break; 00756 case BACK: 00757 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00758 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00759 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00760 strcat(webbuff, "</button></td><td>"); 00761 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00762 strcat(webbuff, "</button></td><td>"); 00763 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00764 strcat(webbuff, "</button></td></tr><td></td><td>"); 00765 strcat(webbuff, "<button id='backbtn' type='button' class=\"light\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00766 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr><td>"); 00767 strcat(webbuff, "<strong>Mode</strong>"); 00768 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00769 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00770 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00771 break; 00772 case AVOIDANCE: 00773 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00774 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00775 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00776 strcat(webbuff, "</button></td><td>"); 00777 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00778 strcat(webbuff, "</button></td><td>"); 00779 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00780 strcat(webbuff, "</button></td></tr><td></td><td>"); 00781 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00782 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00783 strcat(webbuff, "<strong>Mode</strong>"); 00784 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"light\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00785 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00786 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00787 break; 00788 case LINE_TRACE: 00789 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00790 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00791 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00792 strcat(webbuff, "</button></td><td>"); 00793 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00794 strcat(webbuff, "</button></td><td>"); 00795 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00796 strcat(webbuff, "</button></td></tr><td></td><td>"); 00797 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00798 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00799 strcat(webbuff, "<strong>Mode</strong>"); 00800 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00801 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00802 strcat(webbuff, "<button id='tracebtn' type='button' class=\"light\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00803 break; 00804 default: 00805 strcat(webbuff, "<button id='gobtn' type='button' class=\"noselect\" value=\"GO\" onClick='send_mes(this.id,this.value)'>GO"); 00806 strcat(webbuff, "</button></td><td></td></tr><tr><td>"); 00807 strcat(webbuff, "<button id='leftbtn' type='button' class=\"noselect\" value=\"LEFT\" onClick='send_mes(this.id,this.value)' >LEFT"); 00808 strcat(webbuff, "</button></td><td>"); 00809 strcat(webbuff, "<button id='stopbtn' type='button' class=\"noselect\" value=\"STOP\" onClick='send_mes(this.id,this.value)' >STOP"); 00810 strcat(webbuff, "</button></td><td>"); 00811 strcat(webbuff, "<button id='rightbtn' type='button' class=\"noselect\" value=\"RIGHT\" onClick='send_mes(this.id,this.value)' >RIGHT"); 00812 strcat(webbuff, "</button></td></tr><td></td><td>"); 00813 strcat(webbuff, "<button id='backbtn' type='button' class=\"noselect\" value=\"BACK\" onClick='send_mes(this.id,this.value)' >BACK"); 00814 strcat(webbuff, "</button></td><td style='vertical-align:top; text-align:right;'></td></tr></table>"); 00815 strcat(webbuff, "<strong>Mode</strong>"); 00816 strcat(webbuff, "<table><tr><td><button id='avoidbtn' type='button' class=\"noselect\" value=\"AVOIDANCE\" onClick='send_mes(this.id,this.value)' >"); 00817 strcat(webbuff, "AVOIDANCE</button></td><td>"); 00818 strcat(webbuff, "<button id='tracebtn' type='button' class=\"noselect\" value=\"LINE_TRACE\" onClick='send_mes(this.id,this.value)' >LINE_TRACE"); 00819 break; 00820 } 00821 strcat(webbuff, "</button></td></tr></table>"); 00822 strcat(webbuff, "<strong>Speed</strong>"); 00823 strcat(webbuff, "<table><tr><td>"); 00824 //ready示速度だけ点灯 00825 switch (flag_sp) { 00826 case 0: 00827 strcat(webbuff, "<button id='sp1btn' type='button' class=\"light\" value=\"Normal\" onClick='send_mes_spe(this.id,this.value)' >Normal"); 00828 strcat(webbuff, "</button></td><td>"); 00829 strcat(webbuff, "<button id='sp2btn' type='button' class=\"noselect\" value=\"Fast\" onClick='send_mes_spe(this.id,this.value)' >Fast"); 00830 strcat(webbuff, "</button></td><td>"); 00831 strcat(webbuff, "<button id='sp3btn' type='button' class=\"noselect\" value=\"VeryFast\" onClick='send_mes_spe(this.id,this.value)' >VeryFast"); 00832 break; 00833 case 1: 00834 strcat(webbuff, "<button id='sp1btn' type='button' class=\"noselect\" value=\"Normal\" onClick='send_mes_spe(this.id,this.value)' >Normal"); 00835 strcat(webbuff, "</button></td><td>"); 00836 strcat(webbuff, "<button id='sp2btn' type='button' class=\"light\" value=\"Fast\" onClick='send_mes_spe(this.id,this.value)' >Fast"); 00837 strcat(webbuff, "</button></td><td>"); 00838 strcat(webbuff, "<button id='sp3btn' type='button' class=\"noselect\" value=\"VeryFast\" onClick='send_mes_spe(this.id,this.value)' >VeryFast"); 00839 break; 00840 case 2: 00841 strcat(webbuff, "<button id='sp1btn' type='button' class=\"noselect\" value=\"Normal\" onClick='send_mes_spe(this.id,this.value)' >Normal"); 00842 strcat(webbuff, "</button></td><td>"); 00843 strcat(webbuff, "<button id='sp2btn' type='button' class=\"noselect\" value=\"Fast\" onClick='send_mes_spe(this.id,this.value)' >Fast"); 00844 strcat(webbuff, "</button></td><td>"); 00845 strcat(webbuff, "<button id='sp3btn' type='button' class=\"light\" value=\"VeryFast\" onClick='send_mes_spe(this.id,this.value)' >VeryFast"); 00846 break; 00847 default: 00848 strcat(webbuff, "<button id='sp1btn' type='button' class=\"noselect\" value=\"Normal\" onClick='send_mes_spe(this.id,this.value)' >Normal"); 00849 strcat(webbuff, "</button></td><td>"); 00850 strcat(webbuff, "<button id='sp2btn' type='button' class=\"noselect\" value=\"Fast\" onClick='send_mes_spe(this.id,this.value)' >Fast"); 00851 strcat(webbuff, "</button></td><td>"); 00852 strcat(webbuff, "<button id='sp3btn' type='button' class=\"noselect\" value=\"VeryFast\" onClick='send_mes_spe(this.id,this.value)' >VeryFast"); 00853 break; 00854 } 00855 strcat(webbuff, "</button></td></tr></table>"); 00856 00857 strcat(webbuff, "</center>"); 00858 strcat(webbuff, "</body>"); 00859 strcat(webbuff, "</html>"); 00860 strcat(webbuff, "<script language=\"javascript\" type=\"text/javascript\">"); 00861 00862 strcat(webbuff, "function htmlacs(url) {"); 00863 strcat(webbuff, "var xhr = new XMLHttpRequest();"); 00864 strcat(webbuff, "xhr.open(\"GET\", url);"); 00865 strcat(webbuff, "xhr.send(\"\");"); 00866 strcat(webbuff, "}"); 00867 00868 strcat(webbuff, "function send_mes(btnmes,btnval){"); 00869 strcat(webbuff, "console.log(btnval);"); 00870 00871 strcat(webbuff, "var url = \"http://\" + window.location.hostname + \"/cargo?a=\" + btnval;"); 00872 strcat(webbuff, "htmlacs(url);"); 00873 strcat(webbuff, "console.log(url);"); 00874 strcat(webbuff, "var buttons = document.getElementsByTagName(\"button\");"); 00875 strcat(webbuff, "for(var i=0;i<7;i++){"); 00876 strcat(webbuff, "if(buttons[i].value == btnval){"); 00877 strcat(webbuff, "buttons[i].className=\"light\";"); 00878 strcat(webbuff, "}else{"); 00879 strcat(webbuff, "buttons[i].className=\"noselect\";"); 00880 strcat(webbuff, "}"); 00881 strcat(webbuff, "}"); 00882 strcat(webbuff, "}"); 00883 00884 strcat(webbuff, "function send_mes_spe(btnmes,btnval){"); 00885 strcat(webbuff, "var url = \"http://\" + window.location.hostname + \"/cargo?a=\" + btnval;"); 00886 strcat(webbuff, "htmlacs(url);"); 00887 strcat(webbuff, "console.log(url);"); 00888 strcat(webbuff, "var buttons = document.getElementsByTagName(\"button\");"); 00889 strcat(webbuff, "for(var i=7;i<10;i++){"); 00890 strcat(webbuff, "if(buttons[i].value == btnval){"); 00891 strcat(webbuff, "buttons[i].className=\"light\";"); 00892 strcat(webbuff, "}else{"); 00893 strcat(webbuff, "buttons[i].className=\"noselect\";"); 00894 strcat(webbuff, "}"); 00895 strcat(webbuff, "}"); 00896 strcat(webbuff, "}"); 00897 strcat(webbuff, "</script>"); 00898 // end of WEB page data 00899 bufl = strlen(webbuff); // get total page buffer length 00900 //sprintf(cmdbuff,"AT+CIPSEND=%d,%d\r\n", linkID, bufl); // send IPD link channel and buffer character length. 00901 00902 sprintf(cmdbuff,"AT+CIPSENDBUF=%d,%d\r\n", linkID, (bufl>2048?2048:bufl)); // send IPD link channel and buffer character length. 00903 timeout=500; 00904 getcount=40; 00905 SendCMD(); 00906 getreply(); 00907 pc.printf(replybuff); 00908 pc.printf("\n++++++++++ AT+CIPSENDBUF=%d,%d+++++++++\r\n", linkID, (bufl>2048?2048:bufl)); 00909 00910 pc.printf("\n++++++++++ bufl is %d ++++++++++\r\n",bufl); 00911 00912 //pastthrough mode 00913 SendWEB(); // send web page 00914 pc.printf("\n++++++++++ webbuff clear ++++++++++\r\n"); 00915 00916 memset(webbuff, '\0', sizeof(webbuff)); 00917 sendcheck(); 00918 } 00919 00920 // Large WEB buffer data send 00921 void SendWEB() 00922 { 00923 int i=0; 00924 if(esp.writeable()) { 00925 while(webbuff[i]!='\0') { 00926 esp.putc(webbuff[i]); 00927 00928 //**** 00929 //output at command when 2000 00930 if(((i%2047)==0) && (i>0)) { 00931 //wait_ms(10); 00932 sprintf(cmdbuff,"AT+CIPSENDBUF=%d,%d\r\n", linkID, (bufl-2048)>2048?2048:(bufl-2048)); // send IPD link channel and buffer character length. 00933 pc.printf("\r\n++++++++++ AT+CIPSENDBUF=%d,%d ++++++++++\r\n", linkID, (bufl-2048)>2048?2048:(bufl-2048)); 00934 timeout=600; 00935 getcount=50; 00936 SendCMD(); 00937 getreply(); 00938 pc.printf(replybuff); 00939 pc.printf("\r\n+++++++++++++++++++\r\n"); 00940 } 00941 //**** 00942 i++; 00943 pc.printf("%c",webbuff[i]); 00944 } 00945 } 00946 pc.printf("\n++++++++++ send web i= %dinfo ++++++++++\r\n",i); 00947 } 00948 00949 00950 00951 void sendcheck() 00952 { 00953 weberror=1; 00954 timeout=500; 00955 getcount=24; 00956 time2.reset(); 00957 time2.start(); 00958 00959 /* 00960 while(weberror==1 && time2.read() <5) { 00961 getreply(); 00962 if (strstr(replybuff, "SEND OK") != NULL) { 00963 weberror=0; // wait for valid SEND OK 00964 } 00965 } 00966 */ 00967 if(weberror==1) { // restart connection 00968 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); 00969 timeout=500; 00970 getcount=10; 00971 SendCMD(); 00972 getreply(); 00973 pc.printf(replybuff); 00974 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 00975 timeout=500; 00976 getcount=10; 00977 SendCMD(); 00978 getreply(); 00979 pc.printf(replybuff); 00980 } else { 00981 sprintf(cmdbuff, "AT+CIPCLOSE=%s\r\n",channel); // close current connection 00982 SendCMD(); 00983 getreply(); 00984 pc.printf(replybuff); 00985 } 00986 time2.reset(); 00987 } 00988 00989 // Reads and processes GET and POST web data 00990 void ReadWebData() 00991 { 00992 pc.printf("+++++++++++++++++Read Web Data+++++++++++++++++++++\r\n"); 00993 wait_ms(200); 00994 esp.attach(NULL); 00995 ount=0; 00996 DataRX=0; 00997 weberror=0; 00998 memset(webdata, '\0', sizeof(webdata)); 00999 int x = strcspn (webbuff,"+"); 01000 if(x) { 01001 strcpy(webdata, webbuff + x); 01002 weberror=0; 01003 int numMatched = sscanf(webdata,"+IPD,%d,%d:%s", &linkID, &ipdLen, type); 01004 //int i=0; 01005 pc.printf("+++++++++++++++++succed rec begin+++++++++++++++++++++\r\n"); 01006 pc.printf("%s",webdata); 01007 pc.printf("+++++++++++++++++succed rec end+++++++++++++++++++++\r\n"); 01008 if( strstr(webdata, "GO") != NULL ) { 01009 pc.printf("+++++++++++++++++前進+++++++++++++++++++++\r\n"); 01010 run = ADVANCE; // 前進 01011 mode = READY; // モードs変更 01012 display(); // ディスプレイ表示 01013 } 01014 01015 if( strstr(webdata, "LEFT") != NULL ) { 01016 pc.printf("+++++++++++++++++左折+++++++++++++++++++++\r\n"); 01017 run = LEFT; 01018 mode = READY; 01019 display(); // ディスプレイ表示 01020 } 01021 01022 if( strstr(webdata, "STOP") != NULL ) { 01023 pc.printf("+++++++++++++++++停止+++++++++++++++++++++\r\n"); 01024 run = STOP; 01025 mode = READY; 01026 display(); // ディスプレイ表示 01027 } 01028 01029 if( strstr(webdata, "RIGHT") != NULL ) { 01030 pc.printf("+++++++++++++++++右折+++++++++++++++++++++\r\n"); 01031 run = RIGHT; 01032 mode = READY; 01033 display(); // ディスプレイ表示 01034 } 01035 01036 if( strstr(webdata, "BACK") != NULL ) { 01037 pc.printf("+++++++++++++++++後進+++++++++++++++++++++\r\n"); 01038 run = BACK; 01039 mode = READY; 01040 display(); // ディスプレイ表示 01041 } 01042 pc.printf("+++++++++++++++++succed+++++++++++++++++++++"); 01043 01044 if( strstr(webdata, "AVOIDANCE") != NULL ) { 01045 pc.printf("+++++++++++++++++AVOIDANCE+++++++++++++++++++++"); 01046 if(avoi_thread->get_state() == Thread::Deleted) { 01047 delete avoi_thread; 01048 avoi_thread = new Thread(avoidance); 01049 avoi_thread -> set_priority(osPriorityHigh); 01050 } 01051 mode=AVOIDANCE; 01052 run = ADVANCE; 01053 display(); // ディスプレイ表示 01054 } 01055 if( strstr(webdata, "LINE_TRACE") != NULL ) { 01056 pc.printf("+++++++++++++++++LINET RACE+++++++++++++++++++++"); 01057 pc.printf("mode = LINE_TRACE\r\n"); 01058 if(trace_thread->get_state() == Thread::Deleted) { 01059 delete trace_thread; 01060 trace_thread = new Thread(trace); 01061 trace_thread -> set_priority(osPriorityHigh); 01062 } 01063 mode=LINE_TRACE; 01064 display(); // ディスプレイ表示 01065 } 01066 if( strstr(webdata, "Normal") != NULL ) { 01067 pc.printf("++++++++++++++++++Normal++++++++++++++++++++"); 01068 mode = SPEED; // スピードモード 01069 flag_sp = 0; 01070 display(); // ディスプレイ表示 01071 mode = beforeMode; // 現在のモードに前回のモードを設定 01072 } 01073 if( strstr(webdata, "Fast") != NULL ) { 01074 pc.printf("++++++++++++++++++++Fast++++++++++++++++++"); 01075 mode = SPEED; // スピードモード 01076 flag_sp = 1; 01077 display(); // ディスプレイ表示 01078 mode = beforeMode; // 現在のモードに前回のモードを設定 01079 } 01080 if( strstr(webdata, "VeryFast") != NULL ) { 01081 pc.printf("+++++++++++++++++++VeryFast+++++++++++++++++++"); 01082 mode = SPEED; // スピードモード 01083 flag_sp = 2; 01084 display(); // ディスプレイ表示 01085 mode = beforeMode; // 現在のモードに前回のモードを設定 01086 } 01087 if(mode != LINE_TRACE && trace_thread->get_state() != Thread::Deleted){ 01088 trace_thread->terminate(); 01089 } 01090 if(mode != AVOIDANCE && avoi_thread->get_state() != Thread::Deleted){ 01091 avoi_thread->terminate(); 01092 } 01093 sprintf(channel, "%d",linkID); 01094 if (strstr(webdata, "GET") != NULL) { 01095 servreq=1; 01096 } 01097 if (strstr(webdata, "POST") != NULL) { 01098 servreq=1; 01099 } 01100 webcounter++; 01101 sprintf(webcount, "%d",webcounter); 01102 } else { 01103 memset(webbuff, '\0', sizeof(webbuff)); 01104 esp.attach(&callback); 01105 weberror=1; 01106 } 01107 } 01108 // Starts and restarts webserver if errors detected. 01109 void startserver() 01110 { 01111 pc.printf("++++++++++ Resetting ESP ++++++++++\r\n"); 01112 strcpy(cmdbuff,"AT+RST\r\n"); 01113 timeout=8000; 01114 getcount=1000; 01115 SendCMD(); 01116 getreply(); 01117 pc.printf(replybuff); 01118 pc.printf("%d",ount); 01119 if (strstr(replybuff, "OK") != NULL) { 01120 pc.printf("\n++++++++++ Starting Server ++++++++++\r\n"); 01121 strcpy(cmdbuff, "AT+CIPMUX=1\r\n"); // set multiple connections. 01122 timeout=500; 01123 getcount=20; 01124 SendCMD(); 01125 getreply(); 01126 pc.printf(replybuff); 01127 sprintf(cmdbuff,"AT+CIPSERVER=1,%d\r\n", port); 01128 timeout=500; 01129 getcount=20; 01130 SendCMD(); 01131 getreply(); 01132 pc.printf(replybuff); 01133 ThisThread::sleep_for(1000); 01134 sprintf(cmdbuff,"AT+CIPSTO=%d\r\n",SERVtimeout); 01135 timeout=500; 01136 getcount=50; 01137 SendCMD(); 01138 getreply(); 01139 pc.printf(replybuff); 01140 ThisThread::sleep_for(5000); 01141 pc.printf("\n Getting Server IP \r\n"); 01142 strcpy(cmdbuff, "AT+CIFSR\r\n"); 01143 timeout=2500; 01144 getcount=200; 01145 while(weberror==0) { 01146 SendCMD(); 01147 getreply(); 01148 if (strstr(replybuff, "0.0.0.0") == NULL) { 01149 weberror=1; // wait for valid IP 01150 } 01151 } 01152 pc.printf("\n Enter WEB address (IP) found below in your browser \r\n\n"); 01153 pc.printf("\n The MAC address is also shown below,if it is needed \r\n\n"); 01154 replybuff[strlen(replybuff)-1] = '\0'; 01155 //char* IP = replybuff + 5; 01156 sprintf(webdata,"%s", replybuff); 01157 pc.printf(webdata); 01158 led2=1; 01159 bufflen=200; 01160 //bufflen=100; 01161 ount=0; 01162 pc.printf("\n\n++++++++++ Ready ++++++++++\r\n\n"); 01163 esp.attach(&callback); 01164 } else { 01165 pc.printf("\n++++++++++ ESP8266 error, check power/connections ++++++++++\r\n"); 01166 led1=1; 01167 led2=1; 01168 led3=1; 01169 led4=1; 01170 while(1) { 01171 led1=!led1; 01172 led2=!led2; 01173 led3=!led3; 01174 led4=!led4; 01175 ThisThread::sleep_for(1000); 01176 } 01177 } 01178 time2.reset(); 01179 time2.start(); 01180 } 01181 // ESP Command data send 01182 void SendCMD() 01183 { 01184 esp.printf("%s", cmdbuff); 01185 } 01186 // Get Command and ESP status replies 01187 void getreply() 01188 { 01189 memset(replybuff, '\0', sizeof(replybuff)); 01190 time1.reset(); 01191 time1.start(); 01192 replycount=0; 01193 while(time1.read_ms()< timeout && replycount < getcount) { 01194 if(esp.readable()) { 01195 replybuff[replycount] = esp.getc(); 01196 replycount++; 01197 } 01198 } 01199 time1.stop(); 01200 } 01201 01202 /* mainスレッド */ 01203 int main() { 01204 /* 初期設定 */ 01205 avoi_thread = new Thread(avoidance); 01206 avoi_thread->terminate(); 01207 trace_thread = new Thread(trace); 01208 trace_thread->terminate(); 01209 mode = READY; // 現在待ちモード 01210 beforeMode = READY; // 前回待ちモード 01211 run = STOP; // 停止 01212 flag_sp = NORMAL; // スピード(普通) 01213 lcd.setBacklight(TextLCD::LightOn); // バックライトON 01214 display(); // ディスプレイ表示 01215 01216 while(1){ 01217 bChange(); // バッテリー残量更新 01218 } 01219 }
Generated on Thu Aug 11 2022 09:13:17 by
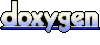