
display&defaultView
Dependencies: RemoteIR TextLCD
main.cpp
00001 #include "mbed.h" 00002 #include "ReceiverIR.h" 00003 #include "rtos.h" 00004 #include "TextLCD.h" 00005 #include <string.h> 00006 //#include <stdint.h> 00007 //3.30 00008 //2.23 00009 ReceiverIR ir_rx(p16); 00010 Serial pc(USBTX, USBRX); 00011 I2C i2c_lcd(p28, p27); 00012 TextLCD_I2C lcd(&i2c_lcd, (0x27 << 1), TextLCD::LCD16x2, TextLCD::HD44780); 00013 enum Mode{ 00014 ADVANCE, 00015 RIGHT, 00016 LEFT, 00017 BACK, 00018 STOP, 00019 LINE_TRACE, 00020 AVOIDANCE, 00021 READY 00022 }; 00023 00024 Timer viewTimer; 00025 Mode mode = READY; 00026 int remind = 100; 00027 00028 uint32_t remoter_code; 00029 void remoter_thread(void const *argument){ 00030 RemoteIR::Format format; 00031 //uint8_t buf[32]; 00032 uint8_t buf[32]; 00033 //uint32_t bitcount; 00034 int bitcount; 00035 00036 while(1){ 00037 // 受信待ち 00038 if (ir_rx.getState() == ReceiverIR::Received) { 00039 // pc.printf("get ir data\r\n"); 00040 // コード受信 00041 //bitcount = ir_rx.getData(&format, buf, sizeof(buf) * 16); 00042 remoter_code=0; 00043 bitcount = ir_rx.getData(&format, buf, sizeof(buf) * 8); 00044 pc.printf("bitcount %d\r\n",bitcount); 00045 00046 for(int i=0;i<(bitcount>>3);i++){ 00047 pc.printf("16 : %02X \r\n",buf[i]); 00048 pc.printf("10 : %d\r\n",buf[i]); 00049 remoter_code=remoter_code<<(i > 0 ? 8 : 0); 00050 //remoter_code << 2; 00051 remoter_code += buf[i]; 00052 } 00053 pc.printf("remote_code %08X \r\n",remoter_code); 00054 pc.printf("\r\n"); 00055 } 00056 } 00057 } 00058 00059 void defaultView(){ 00060 lcd.setAddress(0,0); 00061 lcd.printf("Battery:"); 00062 lcd.setAddress(0,1); 00063 lcd.printf("Mode:"); 00064 } 00065 00066 void display(){ 00067 /*lcd.cls(); 00068 //i2c_lcd.frequency(500); 00069 lcd.setBacklight(TextLCD::LightOn); 00070 lcd.locate(0,0); 00071 lcd.printf("Battery:%3d%%",remind); 00072 lcd.locate(0,1); 00073 lcd.printf("Mode:Ready");*/ 00074 //char DispMode[16]; 00075 while (true) { 00076 lcd.locate(0,1); 00077 switch(mode){ 00078 case ADVANCE: 00079 //strcpy(DispMode,"Mode:Advance"); 00080 lcd.printf("Mode:Advance "); 00081 break; 00082 case RIGHT: 00083 //strcpy(DispMode,"Mode:Right"); 00084 lcd.printf("Mode:TurnRight "); 00085 break; 00086 case LEFT: 00087 //strcpy(DispMode,"Mode:Left"); 00088 lcd.printf("Mode:TurnLeft "); 00089 break; 00090 case BACK: 00091 //strcpy(DispMode,"Mode:Back"); 00092 lcd.printf("Mode:Back "); 00093 break; 00094 case STOP: 00095 //strcpy(DispMode,"Mode:Stop"); 00096 lcd.printf("Mode:Stop "); 00097 break; 00098 case LINE_TRACE: 00099 //strcpy(DispMode,"Mode:LineTrace"); 00100 lcd.printf("Mode:LineTrace "); 00101 break; 00102 case AVOIDANCE: 00103 //strcpy(DispMode,"Mode:Avoidance"); 00104 lcd.printf("Mode:Avoidance "); 00105 break; 00106 case READY: 00107 //strcpy(DispMode,"Mode:Ready"); 00108 lcd.printf("Mode:Ready "); 00109 break; 00110 case SPEED: 00111 switch(flag_sp){ 00112 case(NORMAL): 00113 lcd.printf("Speed:Normal "); 00114 break; 00115 case(FAST): 00116 lcd.printf("Speed:Fast "); 00117 break; 00118 case(VERYFAST): 00119 lcd.printf("Speed:VeryFast "); 00120 break; 00121 } 00122 viewTimer.reset(); 00123 viewTimer.start(); 00124 } 00125 //lcd.printf("%16d",DispMode); 00126 00127 } 00128 } 00129 00130 int main() { 00131 // Thread thread_Re(remoter_thread , NULL , osPriorityHigh); 00132 // ポートD6を赤外線受信モジュールの接続先に指定 00133 int i = 100; 00134 int printC = 0; 00135 lcd.cls(); 00136 //i2c_lcd.frequency(500); 00137 lcd.setBacklight(TextLCD::LightOn); 00138 lcd.locate(0,0); 00139 lcd.printf("Battery:%3d%%",remind); 00140 lcd.locate(0,1); 00141 lcd.printf("Mode:Ready"); 00142 while (true) { 00143 lcd.locate(0,0); 00144 lcd.locate(8,0); 00145 lcd.printf("%3d",i--); 00146 if(i == -1){ 00147 i = 100; 00148 lcd.locate(0,1); 00149 /*switch(mode){ 00150 case ADVANCE: 00151 lcd.printf("Mode:ADVENCE"); 00152 break; 00153 case 0: 00154 lcd.printf("Mode:Line.trace "); 00155 break; 00156 }*/ 00157 //lcd.printf("%-11s",); 00158 00159 } 00160 thread_sleep_for(10); 00161 } 00162 00163 }
Generated on Sat Aug 20 2022 08:25:10 by
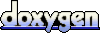