
Hello world program for mbed SDK AnalogOut API
Dependencies: mbed
main.cpp
00001 /* mbed Example Program 00002 * Copyright (c) 2006-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "mbed.h" 00018 00019 // Initialize a pins to perform analog and digital output fucntions 00020 AnalogOut aout(p18); 00021 DigitalOut dout(LED1); 00022 00023 int main(void) 00024 { 00025 while (1) { 00026 // change the voltage on the digital output pin by 0.1 * VCC 00027 // and print what the measured voltage should be (assuming VCC = 3.3v) 00028 for (float i = 0.0f; i < 1.0f; i += 0.1f) { 00029 aout = i; 00030 printf("aout = %1.2f volts\n", aout.read() * 3.3f); 00031 // turn on the led if the voltage is greater than 0.5f * VCC 00032 dout = (aout > 0.5f) ? 1 : 0; 00033 wait(1.0f); 00034 } 00035 } 00036 }
Generated on Tue Jul 12 2022 11:29:33 by
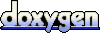