CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
Sine
[Fast Math Functions]
Computes the trigonometric sine function using a combination of table lookup and cubic interpolation. More...
Functions | |
float32_t | arm_sin_f32 (float32_t x) |
Fast approximation to the trigonometric sine function for floating-point data. | |
q15_t | arm_sin_q15 (q15_t x) |
Fast approximation to the trigonometric sine function for Q15 data. | |
q31_t | arm_sin_q31 (q31_t x) |
Fast approximation to the trigonometric sine function for Q31 data. | |
Variables | |
static const float32_t | sinTable [259] |
static const q15_t | sinTableQ15 [259] |
static const q31_t | sinTableQ31 [259] |
Detailed Description
Computes the trigonometric sine function using a combination of table lookup and cubic interpolation.
There are separate functions for Q15, Q31, and floating-point data types. The input to the floating-point version is in radians while the fixed-point Q15 and Q31 have a scaled input with the range [0 +0.9999] mapping to [0 2*pi). The fixed-point range is chosen so that a value of 2*pi wraps around to 0.
The implementation is based on table lookup using 256 values together with cubic interpolation. The steps used are:
- Calculation of the nearest integer table index
- Fetch the four table values a, b, c, and d
- Compute the fractional portion (fract) of the table index.
- Calculation of wa, wb, wc, wd
- The final result equals
a*wa + b*wb + c*wc + d*wd
where
a=Table[index-1]; b=Table[index+0]; c=Table[index+1]; d=Table[index+2];
and
wa=-(1/6)*fract.^3 + (1/2)*fract.^2 - (1/3)*fract; wb=(1/2)*fract.^3 - fract.^2 - (1/2)*fract + 1; wc=-(1/2)*fract.^3+(1/2)*fract.^2+fract; wd=(1/6)*fract.^3 - (1/6)*fract;
Function Documentation
float32_t arm_sin_f32 | ( | float32_t | x ) |
Fast approximation to the trigonometric sine function for floating-point data.
end of LinearInterpolate group
- Parameters:
-
[in] x input value in radians.
- Returns:
- sin(x).
Definition at line 208 of file arm_sin_f32.c.
q15_t arm_sin_q15 | ( | q15_t | x ) |
Fast approximation to the trigonometric sine function for Q15 data.
- Parameters:
-
[in] x Scaled input value in radians.
- Returns:
- sin(x).
The Q15 input value is in the range [0 +0.9999] and is mapped to a radian value in the range [0 2*pi).
Definition at line 117 of file arm_sin_q15.c.
q31_t arm_sin_q31 | ( | q31_t | x ) |
Fast approximation to the trigonometric sine function for Q31 data.
- Parameters:
-
[in] x Scaled input value in radians.
- Returns:
- sin(x).
The Q31 input value is in the range [0 +0.9999] and is mapped to a radian value in the range [0 2*pi).
Definition at line 147 of file arm_sin_q31.c.
Variable Documentation
const float32_t sinTable[259] [static] |
- Example code for the generation of the floating-point sine table:
tableSize = 256; for(n = -1; n < (tableSize + 1); n++) { sinTable[n+1]=sin(2*pi*n/tableSize); }
- where pi value is 3.14159265358979
Definition at line 101 of file arm_sin_f32.c.
const q15_t sinTableQ15[259] [static] |
- Table values are in Q15 (1.15 fixed-point format) and generation is done in three steps. First, generate sin values in floating point:
tableSize = 256; for(n = -1; n < (tableSize + 1); n++) { sinTable[n+1]= sin(2*pi*n/tableSize); }
where pi value is 3.14159265358979
- Second, convert floating-point to Q15 (fixed-point): (sinTable[i] * pow(2, 15))
- Finally, round to the nearest integer value: sinTable[i] += (sinTable[i] > 0 ? 0.5 :-0.5);
Definition at line 72 of file arm_sin_q15.c.
const q31_t sinTableQ31[259] [static] |
- Table values are in Q31 (1.31 fixed-point format) and generation is done in three steps. First, generate sin values in floating point:
tableSize = 256; for(n = -1; n < (tableSize + 1); n++) { sinTable[n+1]= sin(2*pi*n/tableSize); }
where pi value is 3.14159265358979
- Second, convert floating-point to Q31 (Fixed point): (sinTable[i] * pow(2, 31))
- Finally, round to the nearest integer value: sinTable[i] += (sinTable[i] > 0 ? 0.5 :-0.5);
Definition at line 71 of file arm_sin_q31.c.
Generated on Tue Jul 12 2022 12:36:58 by
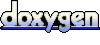