CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_std_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_std_f32.c 00009 * 00010 * Description: Standard deviation of the elements of a floating-point vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupStats 00045 */ 00046 00047 /** 00048 * @defgroup STD Standard deviation 00049 * 00050 * Calculates the standard deviation of the elements in the input vector. 00051 * The underlying algorithm is used: 00052 * 00053 * <pre> 00054 * Result = sqrt((sumOfSquares - sum<sup>2</sup> / blockSize) / (blockSize - 1)) 00055 * 00056 * where, sumOfSquares = pSrc[0] * pSrc[0] + pSrc[1] * pSrc[1] + ... + pSrc[blockSize-1] * pSrc[blockSize-1] 00057 * 00058 * sum = pSrc[0] + pSrc[1] + pSrc[2] + ... + pSrc[blockSize-1] 00059 * </pre> 00060 * 00061 * There are separate functions for floating point, Q31, and Q15 data types. 00062 */ 00063 00064 /** 00065 * @addtogroup STD 00066 * @{ 00067 */ 00068 00069 00070 /** 00071 * @brief Standard deviation of the elements of a floating-point vector. 00072 * @param[in] *pSrc points to the input vector 00073 * @param[in] blockSize length of the input vector 00074 * @param[out] *pResult standard deviation value returned here 00075 * @return none. 00076 * 00077 */ 00078 00079 00080 void arm_std_f32( 00081 float32_t * pSrc, 00082 uint32_t blockSize, 00083 float32_t * pResult) 00084 { 00085 float32_t sum = 0.0f; /* Temporary result storage */ 00086 float32_t sumOfSquares = 0.0f; /* Sum of squares */ 00087 float32_t in; /* input value */ 00088 uint32_t blkCnt; /* loop counter */ 00089 00090 #ifndef ARM_MATH_CM0_FAMILY 00091 00092 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00093 00094 float32_t meanOfSquares, mean, squareOfMean; 00095 00096 /*loop Unrolling */ 00097 blkCnt = blockSize >> 2u; 00098 00099 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00100 ** a second loop below computes the remaining 1 to 3 samples. */ 00101 while(blkCnt > 0u) 00102 { 00103 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00104 /* Compute Sum of squares of the input samples 00105 * and then store the result in a temporary variable, sum. */ 00106 in = *pSrc++; 00107 sum += in; 00108 sumOfSquares += in * in; 00109 in = *pSrc++; 00110 sum += in; 00111 sumOfSquares += in * in; 00112 in = *pSrc++; 00113 sum += in; 00114 sumOfSquares += in * in; 00115 in = *pSrc++; 00116 sum += in; 00117 sumOfSquares += in * in; 00118 00119 /* Decrement the loop counter */ 00120 blkCnt--; 00121 } 00122 00123 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00124 ** No loop unrolling is used. */ 00125 blkCnt = blockSize % 0x4u; 00126 00127 while(blkCnt > 0u) 00128 { 00129 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00130 /* Compute Sum of squares of the input samples 00131 * and then store the result in a temporary variable, sum. */ 00132 in = *pSrc++; 00133 sum += in; 00134 sumOfSquares += in * in; 00135 00136 /* Decrement the loop counter */ 00137 blkCnt--; 00138 } 00139 00140 /* Compute Mean of squares of the input samples 00141 * and then store the result in a temporary variable, meanOfSquares. */ 00142 meanOfSquares = sumOfSquares / ((float32_t) blockSize - 1.0f); 00143 00144 /* Compute mean of all input values */ 00145 mean = sum / (float32_t) blockSize; 00146 00147 /* Compute square of mean */ 00148 squareOfMean = (mean * mean) * (((float32_t) blockSize) / 00149 ((float32_t) blockSize - 1.0f)); 00150 00151 /* Compute standard deviation and then store the result to the destination */ 00152 arm_sqrt_f32((meanOfSquares - squareOfMean), pResult); 00153 00154 #else 00155 00156 /* Run the below code for Cortex-M0 */ 00157 00158 float32_t squareOfSum; /* Square of Sum */ 00159 float32_t var; /* Temporary varaince storage */ 00160 00161 /* Loop over blockSize number of values */ 00162 blkCnt = blockSize; 00163 00164 while(blkCnt > 0u) 00165 { 00166 /* C = (A[0] * A[0] + A[1] * A[1] + ... + A[blockSize-1] * A[blockSize-1]) */ 00167 /* Compute Sum of squares of the input samples 00168 * and then store the result in a temporary variable, sumOfSquares. */ 00169 in = *pSrc++; 00170 sumOfSquares += in * in; 00171 00172 /* C = (A[0] + A[1] + ... + A[blockSize-1]) */ 00173 /* Compute Sum of the input samples 00174 * and then store the result in a temporary variable, sum. */ 00175 sum += in; 00176 00177 /* Decrement the loop counter */ 00178 blkCnt--; 00179 } 00180 00181 /* Compute the square of sum */ 00182 squareOfSum = ((sum * sum) / (float32_t) blockSize); 00183 00184 /* Compute the variance */ 00185 var = ((sumOfSquares - squareOfSum) / (float32_t) (blockSize - 1.0f)); 00186 00187 /* Compute standard deviation and then store the result to the destination */ 00188 arm_sqrt_f32(var, pResult); 00189 00190 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00191 00192 } 00193 00194 /** 00195 * @} end of STD group 00196 */
Generated on Tue Jul 12 2022 12:36:57 by
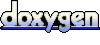