CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_rms_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_rms_q31.c 00009 * 00010 * Description: Root Mean Square of the elements of a Q31 vector. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * ---------------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @addtogroup RMS 00045 * @{ 00046 */ 00047 00048 00049 /** 00050 * @brief Root Mean Square of the elements of a Q31 vector. 00051 * @param[in] *pSrc points to the input vector 00052 * @param[in] blockSize length of the input vector 00053 * @param[out] *pResult rms value returned here 00054 * @return none. 00055 * 00056 * @details 00057 * <b>Scaling and Overflow Behavior:</b> 00058 * 00059 *\par 00060 * The function is implemented using an internal 64-bit accumulator. 00061 * The input is represented in 1.31 format, and intermediate multiplication 00062 * yields a 2.62 format. 00063 * The accumulator maintains full precision of the intermediate multiplication results, 00064 * but provides only a single guard bit. 00065 * There is no saturation on intermediate additions. 00066 * If the accumulator overflows, it wraps around and distorts the result. 00067 * In order to avoid overflows completely, the input signal must be scaled down by 00068 * log2(blockSize) bits, as a total of blockSize additions are performed internally. 00069 * Finally, the 2.62 accumulator is right shifted by 31 bits to yield a 1.31 format value. 00070 * 00071 */ 00072 00073 void arm_rms_q31( 00074 q31_t * pSrc, 00075 uint32_t blockSize, 00076 q31_t * pResult) 00077 { 00078 q63_t sum = 0; /* accumulator */ 00079 q31_t in; /* Temporary variable to store the input */ 00080 uint32_t blkCnt; /* loop counter */ 00081 00082 #ifndef ARM_MATH_CM0_FAMILY 00083 00084 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00085 00086 q31_t in1, in2, in3, in4; /* Temporary input variables */ 00087 00088 /*loop Unrolling */ 00089 blkCnt = blockSize >> 2u; 00090 00091 /* First part of the processing with loop unrolling. Compute 8 outputs at a time. 00092 ** a second loop below computes the remaining 1 to 7 samples. */ 00093 while(blkCnt > 0u) 00094 { 00095 /* C = A[0] * A[0] + A[1] * A[1] + A[2] * A[2] + ... + A[blockSize-1] * A[blockSize-1] */ 00096 /* Compute sum of the squares and then store the result in a temporary variable, sum */ 00097 /* read two samples from source buffer */ 00098 in1 = pSrc[0]; 00099 in2 = pSrc[1]; 00100 00101 /* calculate power and accumulate to accumulator */ 00102 sum += (q63_t) in1 *in1; 00103 sum += (q63_t) in2 *in2; 00104 00105 /* read two samples from source buffer */ 00106 in3 = pSrc[2]; 00107 in4 = pSrc[3]; 00108 00109 /* calculate power and accumulate to accumulator */ 00110 sum += (q63_t) in3 *in3; 00111 sum += (q63_t) in4 *in4; 00112 00113 00114 /* update source buffer to process next samples */ 00115 pSrc += 4u; 00116 00117 /* Decrement the loop counter */ 00118 blkCnt--; 00119 } 00120 00121 /* If the blockSize is not a multiple of 8, compute any remaining output samples here. 00122 ** No loop unrolling is used. */ 00123 blkCnt = blockSize % 0x4u; 00124 00125 #else 00126 00127 /* Run the below code for Cortex-M0 */ 00128 blkCnt = blockSize; 00129 00130 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00131 00132 while(blkCnt > 0u) 00133 { 00134 /* C = A[0] * A[0] + A[1] * A[1] + A[2] * A[2] + ... + A[blockSize-1] * A[blockSize-1] */ 00135 /* Compute sum of the squares and then store the results in a temporary variable, sum */ 00136 in = *pSrc++; 00137 sum += (q63_t) in *in; 00138 00139 /* Decrement the loop counter */ 00140 blkCnt--; 00141 } 00142 00143 /* Convert data in 2.62 to 1.31 by 31 right shifts and saturate */ 00144 00145 sum = __SSAT(sum >> 31, 31); 00146 00147 00148 /* Compute Rms and store the result in the destination vector */ 00149 arm_sqrt_q31((q31_t) ((q31_t) sum / (int32_t) blockSize), pResult); 00150 } 00151 00152 /** 00153 * @} end of RMS group 00154 */
Generated on Tue Jul 12 2022 12:36:57 by
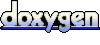