CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_mat_trans_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_trans_q31.c 00009 * 00010 * Description: Q31 matrix transpose. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @addtogroup MatrixTrans 00049 * @{ 00050 */ 00051 00052 /* 00053 * @brief Q31 matrix transpose. 00054 * @param[in] *pSrc points to the input matrix 00055 * @param[out] *pDst points to the output matrix 00056 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00057 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00058 */ 00059 00060 arm_status arm_mat_trans_q31( 00061 const arm_matrix_instance_q31 * pSrc, 00062 arm_matrix_instance_q31 * pDst) 00063 { 00064 q31_t *pIn = pSrc->pData; /* input data matrix pointer */ 00065 q31_t *pOut = pDst->pData; /* output data matrix pointer */ 00066 q31_t *px; /* Temporary output data matrix pointer */ 00067 uint16_t nRows = pSrc->numRows; /* number of nRows */ 00068 uint16_t nColumns = pSrc->numCols; /* number of nColumns */ 00069 00070 #ifndef ARM_MATH_CM0_FAMILY 00071 00072 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00073 00074 uint16_t blkCnt, i = 0u, row = nRows; /* loop counters */ 00075 arm_status status; /* status of matrix transpose */ 00076 00077 00078 #ifdef ARM_MATH_MATRIX_CHECK 00079 00080 00081 /* Check for matrix mismatch condition */ 00082 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00083 { 00084 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00085 status = ARM_MATH_SIZE_MISMATCH; 00086 } 00087 else 00088 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00089 00090 { 00091 /* Matrix transpose by exchanging the rows with columns */ 00092 /* row loop */ 00093 do 00094 { 00095 /* Apply loop unrolling and exchange the columns with row elements */ 00096 blkCnt = nColumns >> 2u; 00097 00098 /* The pointer px is set to starting address of the column being processed */ 00099 px = pOut + i; 00100 00101 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00102 ** a second loop below computes the remaining 1 to 3 samples. */ 00103 while(blkCnt > 0u) 00104 { 00105 /* Read and store the input element in the destination */ 00106 *px = *pIn++; 00107 00108 /* Update the pointer px to point to the next row of the transposed matrix */ 00109 px += nRows; 00110 00111 /* Read and store the input element in the destination */ 00112 *px = *pIn++; 00113 00114 /* Update the pointer px to point to the next row of the transposed matrix */ 00115 px += nRows; 00116 00117 /* Read and store the input element in the destination */ 00118 *px = *pIn++; 00119 00120 /* Update the pointer px to point to the next row of the transposed matrix */ 00121 px += nRows; 00122 00123 /* Read and store the input element in the destination */ 00124 *px = *pIn++; 00125 00126 /* Update the pointer px to point to the next row of the transposed matrix */ 00127 px += nRows; 00128 00129 /* Decrement the column loop counter */ 00130 blkCnt--; 00131 } 00132 00133 /* Perform matrix transpose for last 3 samples here. */ 00134 blkCnt = nColumns % 0x4u; 00135 00136 while(blkCnt > 0u) 00137 { 00138 /* Read and store the input element in the destination */ 00139 *px = *pIn++; 00140 00141 /* Update the pointer px to point to the next row of the transposed matrix */ 00142 px += nRows; 00143 00144 /* Decrement the column loop counter */ 00145 blkCnt--; 00146 } 00147 00148 #else 00149 00150 /* Run the below code for Cortex-M0 */ 00151 00152 uint16_t col, i = 0u, row = nRows; /* loop counters */ 00153 arm_status status; /* status of matrix transpose */ 00154 00155 00156 #ifdef ARM_MATH_MATRIX_CHECK 00157 00158 /* Check for matrix mismatch condition */ 00159 if((pSrc->numRows != pDst->numCols) || (pSrc->numCols != pDst->numRows)) 00160 { 00161 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00162 status = ARM_MATH_SIZE_MISMATCH; 00163 } 00164 else 00165 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00166 00167 { 00168 /* Matrix transpose by exchanging the rows with columns */ 00169 /* row loop */ 00170 do 00171 { 00172 /* The pointer px is set to starting address of the column being processed */ 00173 px = pOut + i; 00174 00175 /* Initialize column loop counter */ 00176 col = nColumns; 00177 00178 while(col > 0u) 00179 { 00180 /* Read and store the input element in the destination */ 00181 *px = *pIn++; 00182 00183 /* Update the pointer px to point to the next row of the transposed matrix */ 00184 px += nRows; 00185 00186 /* Decrement the column loop counter */ 00187 col--; 00188 } 00189 00190 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00191 00192 i++; 00193 00194 /* Decrement the row loop counter */ 00195 row--; 00196 00197 } 00198 while(row > 0u); /* row loop end */ 00199 00200 /* set status as ARM_MATH_SUCCESS */ 00201 status = ARM_MATH_SUCCESS; 00202 } 00203 00204 /* Return to application */ 00205 return (status); 00206 } 00207 00208 /** 00209 * @} end of MatrixTrans group 00210 */
Generated on Tue Jul 12 2022 12:36:56 by
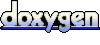