CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_mat_sub_q15.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_sub_q15.c 00009 * 00010 * Description: Q15 Matrix subtraction 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @addtogroup MatrixSub 00049 * @{ 00050 */ 00051 00052 /** 00053 * @brief Q15 matrix subtraction. 00054 * @param[in] *pSrcA points to the first input matrix structure 00055 * @param[in] *pSrcB points to the second input matrix structure 00056 * @param[out] *pDst points to output matrix structure 00057 * @return The function returns either 00058 * <code>ARM_MATH_SIZE_MISMATCH</code> or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00059 * 00060 * <b>Scaling and Overflow Behavior:</b> 00061 * \par 00062 * The function uses saturating arithmetic. 00063 * Results outside of the allowable Q15 range [0x8000 0x7FFF] will be saturated. 00064 */ 00065 00066 arm_status arm_mat_sub_q15( 00067 const arm_matrix_instance_q15 * pSrcA, 00068 const arm_matrix_instance_q15 * pSrcB, 00069 arm_matrix_instance_q15 * pDst) 00070 { 00071 q15_t *pInA = pSrcA->pData; /* input data matrix pointer A */ 00072 q15_t *pInB = pSrcB->pData; /* input data matrix pointer B */ 00073 q15_t *pOut = pDst->pData; /* output data matrix pointer */ 00074 uint32_t numSamples; /* total number of elements in the matrix */ 00075 uint32_t blkCnt; /* loop counters */ 00076 arm_status status; /* status of matrix subtraction */ 00077 00078 00079 #ifdef ARM_MATH_MATRIX_CHECK 00080 00081 00082 /* Check for matrix mismatch condition */ 00083 if((pSrcA->numRows != pSrcB->numRows) || 00084 (pSrcA->numCols != pSrcB->numCols) || 00085 (pSrcA->numRows != pDst->numRows) || (pSrcA->numCols != pDst->numCols)) 00086 { 00087 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00088 status = ARM_MATH_SIZE_MISMATCH; 00089 } 00090 else 00091 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00092 00093 { 00094 /* Total number of samples in the input matrix */ 00095 numSamples = (uint32_t) pSrcA->numRows * pSrcA->numCols; 00096 00097 #ifndef ARM_MATH_CM0_FAMILY 00098 00099 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00100 00101 /* Apply loop unrolling */ 00102 blkCnt = numSamples >> 2u; 00103 00104 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00105 ** a second loop below computes the remaining 1 to 3 samples. */ 00106 while(blkCnt > 0u) 00107 { 00108 /* C(m,n) = A(m,n) - B(m,n) */ 00109 /* Subtract, Saturate and then store the results in the destination buffer. */ 00110 *__SIMD32(pOut)++ = __QSUB16(*__SIMD32(pInA)++, *__SIMD32(pInB)++); 00111 *__SIMD32(pOut)++ = __QSUB16(*__SIMD32(pInA)++, *__SIMD32(pInB)++); 00112 00113 /* Decrement the loop counter */ 00114 blkCnt--; 00115 } 00116 00117 /* If the blockSize is not a multiple of 4, compute any remaining output samples here. 00118 ** No loop unrolling is used. */ 00119 blkCnt = numSamples % 0x4u; 00120 00121 while(blkCnt > 0u) 00122 { 00123 /* C(m,n) = A(m,n) - B(m,n) */ 00124 /* Subtract and then store the results in the destination buffer. */ 00125 *pOut++ = (q15_t) __QSUB16(*pInA++, *pInB++); 00126 00127 /* Decrement the loop counter */ 00128 blkCnt--; 00129 } 00130 00131 #else 00132 00133 /* Run the below code for Cortex-M0 */ 00134 00135 /* Initialize blkCnt with number of samples */ 00136 blkCnt = numSamples; 00137 00138 while(blkCnt > 0u) 00139 { 00140 /* C(m,n) = A(m,n) - B(m,n) */ 00141 /* Subtract and then store the results in the destination buffer. */ 00142 *pOut++ = (q15_t) __SSAT(((q31_t) * pInA++ - *pInB++), 16); 00143 00144 /* Decrement the loop counter */ 00145 blkCnt--; 00146 } 00147 00148 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00149 00150 /* Set status as ARM_MATH_SUCCESS */ 00151 status = ARM_MATH_SUCCESS; 00152 } 00153 00154 /* Return to application */ 00155 return (status); 00156 } 00157 00158 /** 00159 * @} end of MatrixSub group 00160 */
Generated on Tue Jul 12 2022 12:36:56 by
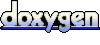