CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_mat_scale_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_mat_scale_f32.c 00009 * 00010 * Description: Multiplies a floating-point matrix by a scalar. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupMatrix 00045 */ 00046 00047 /** 00048 * @defgroup MatrixScale Matrix Scale 00049 * 00050 * Multiplies a matrix by a scalar. This is accomplished by multiplying each element in the 00051 * matrix by the scalar. For example: 00052 * \image html MatrixScale.gif "Matrix Scaling of a 3 x 3 matrix" 00053 * 00054 * The function checks to make sure that the input and output matrices are of the same size. 00055 * 00056 * In the fixed-point Q15 and Q31 functions, <code>scale</code> is represented by 00057 * a fractional multiplication <code>scaleFract</code> and an arithmetic shift <code>shift</code>. 00058 * The shift allows the gain of the scaling operation to exceed 1.0. 00059 * The overall scale factor applied to the fixed-point data is 00060 * <pre> 00061 * scale = scaleFract * 2^shift. 00062 * </pre> 00063 */ 00064 00065 /** 00066 * @addtogroup MatrixScale 00067 * @{ 00068 */ 00069 00070 /** 00071 * @brief Floating-point matrix scaling. 00072 * @param[in] *pSrc points to input matrix structure 00073 * @param[in] scale scale factor to be applied 00074 * @param[out] *pDst points to output matrix structure 00075 * @return The function returns either <code>ARM_MATH_SIZE_MISMATCH</code> 00076 * or <code>ARM_MATH_SUCCESS</code> based on the outcome of size checking. 00077 * 00078 */ 00079 00080 arm_status arm_mat_scale_f32( 00081 const arm_matrix_instance_f32 * pSrc, 00082 float32_t scale, 00083 arm_matrix_instance_f32 * pDst) 00084 { 00085 float32_t *pIn = pSrc->pData; /* input data matrix pointer */ 00086 float32_t *pOut = pDst->pData; /* output data matrix pointer */ 00087 uint32_t numSamples; /* total number of elements in the matrix */ 00088 uint32_t blkCnt; /* loop counters */ 00089 arm_status status; /* status of matrix scaling */ 00090 00091 #ifndef ARM_MATH_CM0_FAMILY 00092 00093 float32_t in1, in2, in3, in4; /* temporary variables */ 00094 float32_t out1, out2, out3, out4; /* temporary variables */ 00095 00096 #endif // #ifndef ARM_MATH_CM0_FAMILY 00097 00098 #ifdef ARM_MATH_MATRIX_CHECK 00099 /* Check for matrix mismatch condition */ 00100 if((pSrc->numRows != pDst->numRows) || (pSrc->numCols != pDst->numCols)) 00101 { 00102 /* Set status as ARM_MATH_SIZE_MISMATCH */ 00103 status = ARM_MATH_SIZE_MISMATCH; 00104 } 00105 else 00106 #endif /* #ifdef ARM_MATH_MATRIX_CHECK */ 00107 { 00108 /* Total number of samples in the input matrix */ 00109 numSamples = (uint32_t) pSrc->numRows * pSrc->numCols; 00110 00111 #ifndef ARM_MATH_CM0_FAMILY 00112 00113 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00114 00115 /* Loop Unrolling */ 00116 blkCnt = numSamples >> 2; 00117 00118 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00119 ** a second loop below computes the remaining 1 to 3 samples. */ 00120 while(blkCnt > 0u) 00121 { 00122 /* C(m,n) = A(m,n) * scale */ 00123 /* Scaling and results are stored in the destination buffer. */ 00124 in1 = pIn[0]; 00125 in2 = pIn[1]; 00126 in3 = pIn[2]; 00127 in4 = pIn[3]; 00128 00129 out1 = in1 * scale; 00130 out2 = in2 * scale; 00131 out3 = in3 * scale; 00132 out4 = in4 * scale; 00133 00134 00135 pOut[0] = out1; 00136 pOut[1] = out2; 00137 pOut[2] = out3; 00138 pOut[3] = out4; 00139 00140 /* update pointers to process next sampels */ 00141 pIn += 4u; 00142 pOut += 4u; 00143 00144 /* Decrement the numSamples loop counter */ 00145 blkCnt--; 00146 } 00147 00148 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00149 ** No loop unrolling is used. */ 00150 blkCnt = numSamples % 0x4u; 00151 00152 #else 00153 00154 /* Run the below code for Cortex-M0 */ 00155 00156 /* Initialize blkCnt with number of samples */ 00157 blkCnt = numSamples; 00158 00159 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00160 00161 while(blkCnt > 0u) 00162 { 00163 /* C(m,n) = A(m,n) * scale */ 00164 /* The results are stored in the destination buffer. */ 00165 *pOut++ = (*pIn++) * scale; 00166 00167 /* Decrement the loop counter */ 00168 blkCnt--; 00169 } 00170 00171 /* Set status as ARM_MATH_SUCCESS */ 00172 status = ARM_MATH_SUCCESS; 00173 } 00174 00175 /* Return to application */ 00176 return (status); 00177 } 00178 00179 /** 00180 * @} end of MatrixScale group 00181 */
Generated on Tue Jul 12 2022 12:36:56 by
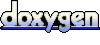