CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_lms_f32.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_lms_f32.c 00009 * 00010 * Description: Processing function for the floating-point LMS filter. 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupFilters 00045 */ 00046 00047 /** 00048 * @defgroup LMS Least Mean Square (LMS) Filters 00049 * 00050 * LMS filters are a class of adaptive filters that are able to "learn" an unknown transfer functions. 00051 * LMS filters use a gradient descent method in which the filter coefficients are updated based on the instantaneous error signal. 00052 * Adaptive filters are often used in communication systems, equalizers, and noise removal. 00053 * The CMSIS DSP Library contains LMS filter functions that operate on Q15, Q31, and floating-point data types. 00054 * The library also contains normalized LMS filters in which the filter coefficient adaptation is indepedent of the level of the input signal. 00055 * 00056 * An LMS filter consists of two components as shown below. 00057 * The first component is a standard transversal or FIR filter. 00058 * The second component is a coefficient update mechanism. 00059 * The LMS filter has two input signals. 00060 * The "input" feeds the FIR filter while the "reference input" corresponds to the desired output of the FIR filter. 00061 * That is, the FIR filter coefficients are updated so that the output of the FIR filter matches the reference input. 00062 * The filter coefficient update mechanism is based on the difference between the FIR filter output and the reference input. 00063 * This "error signal" tends towards zero as the filter adapts. 00064 * The LMS processing functions accept the input and reference input signals and generate the filter output and error signal. 00065 * \image html LMS.gif "Internal structure of the Least Mean Square filter" 00066 * 00067 * The functions operate on blocks of data and each call to the function processes 00068 * <code>blockSize</code> samples through the filter. 00069 * <code>pSrc</code> points to input signal, <code>pRef</code> points to reference signal, 00070 * <code>pOut</code> points to output signal and <code>pErr</code> points to error signal. 00071 * All arrays contain <code>blockSize</code> values. 00072 * 00073 * The functions operate on a block-by-block basis. 00074 * Internally, the filter coefficients <code>b[n]</code> are updated on a sample-by-sample basis. 00075 * The convergence of the LMS filter is slower compared to the normalized LMS algorithm. 00076 * 00077 * \par Algorithm: 00078 * The output signal <code>y[n]</code> is computed by a standard FIR filter: 00079 * <pre> 00080 * y[n] = b[0] * x[n] + b[1] * x[n-1] + b[2] * x[n-2] + ...+ b[numTaps-1] * x[n-numTaps+1] 00081 * </pre> 00082 * 00083 * \par 00084 * The error signal equals the difference between the reference signal <code>d[n]</code> and the filter output: 00085 * <pre> 00086 * e[n] = d[n] - y[n]. 00087 * </pre> 00088 * 00089 * \par 00090 * After each sample of the error signal is computed, the filter coefficients <code>b[k]</code> are updated on a sample-by-sample basis: 00091 * <pre> 00092 * b[k] = b[k] + e[n] * mu * x[n-k], for k=0, 1, ..., numTaps-1 00093 * </pre> 00094 * where <code>mu</code> is the step size and controls the rate of coefficient convergence. 00095 *\par 00096 * In the APIs, <code>pCoeffs</code> points to a coefficient array of size <code>numTaps</code>. 00097 * Coefficients are stored in time reversed order. 00098 * \par 00099 * <pre> 00100 * {b[numTaps-1], b[numTaps-2], b[N-2], ..., b[1], b[0]} 00101 * </pre> 00102 * \par 00103 * <code>pState</code> points to a state array of size <code>numTaps + blockSize - 1</code>. 00104 * Samples in the state buffer are stored in the order: 00105 * \par 00106 * <pre> 00107 * {x[n-numTaps+1], x[n-numTaps], x[n-numTaps-1], x[n-numTaps-2]....x[0], x[1], ..., x[blockSize-1]} 00108 * </pre> 00109 * \par 00110 * Note that the length of the state buffer exceeds the length of the coefficient array by <code>blockSize-1</code> samples. 00111 * The increased state buffer length allows circular addressing, which is traditionally used in FIR filters, 00112 * to be avoided and yields a significant speed improvement. 00113 * The state variables are updated after each block of data is processed. 00114 * \par Instance Structure 00115 * The coefficients and state variables for a filter are stored together in an instance data structure. 00116 * A separate instance structure must be defined for each filter and 00117 * coefficient and state arrays cannot be shared among instances. 00118 * There are separate instance structure declarations for each of the 3 supported data types. 00119 * 00120 * \par Initialization Functions 00121 * There is also an associated initialization function for each data type. 00122 * The initialization function performs the following operations: 00123 * - Sets the values of the internal structure fields. 00124 * - Zeros out the values in the state buffer. 00125 * To do this manually without calling the init function, assign the follow subfields of the instance structure: 00126 * numTaps, pCoeffs, mu, postShift (not for f32), pState. Also set all of the values in pState to zero. 00127 * 00128 * \par 00129 * Use of the initialization function is optional. 00130 * However, if the initialization function is used, then the instance structure cannot be placed into a const data section. 00131 * To place an instance structure into a const data section, the instance structure must be manually initialized. 00132 * Set the values in the state buffer to zeros before static initialization. 00133 * The code below statically initializes each of the 3 different data type filter instance structures 00134 * <pre> 00135 * arm_lms_instance_f32 S = {numTaps, pState, pCoeffs, mu}; 00136 * arm_lms_instance_q31 S = {numTaps, pState, pCoeffs, mu, postShift}; 00137 * arm_lms_instance_q15 S = {numTaps, pState, pCoeffs, mu, postShift}; 00138 * </pre> 00139 * where <code>numTaps</code> is the number of filter coefficients in the filter; <code>pState</code> is the address of the state buffer; 00140 * <code>pCoeffs</code> is the address of the coefficient buffer; <code>mu</code> is the step size parameter; and <code>postShift</code> is the shift applied to coefficients. 00141 * 00142 * \par Fixed-Point Behavior: 00143 * Care must be taken when using the Q15 and Q31 versions of the LMS filter. 00144 * The following issues must be considered: 00145 * - Scaling of coefficients 00146 * - Overflow and saturation 00147 * 00148 * \par Scaling of Coefficients: 00149 * Filter coefficients are represented as fractional values and 00150 * coefficients are restricted to lie in the range <code>[-1 +1)</code>. 00151 * The fixed-point functions have an additional scaling parameter <code>postShift</code>. 00152 * At the output of the filter's accumulator is a shift register which shifts the result by <code>postShift</code> bits. 00153 * This essentially scales the filter coefficients by <code>2^postShift</code> and 00154 * allows the filter coefficients to exceed the range <code>[+1 -1)</code>. 00155 * The value of <code>postShift</code> is set by the user based on the expected gain through the system being modeled. 00156 * 00157 * \par Overflow and Saturation: 00158 * Overflow and saturation behavior of the fixed-point Q15 and Q31 versions are 00159 * described separately as part of the function specific documentation below. 00160 */ 00161 00162 /** 00163 * @addtogroup LMS 00164 * @{ 00165 */ 00166 00167 /** 00168 * @details 00169 * This function operates on floating-point data types. 00170 * 00171 * @brief Processing function for floating-point LMS filter. 00172 * @param[in] *S points to an instance of the floating-point LMS filter structure. 00173 * @param[in] *pSrc points to the block of input data. 00174 * @param[in] *pRef points to the block of reference data. 00175 * @param[out] *pOut points to the block of output data. 00176 * @param[out] *pErr points to the block of error data. 00177 * @param[in] blockSize number of samples to process. 00178 * @return none. 00179 */ 00180 00181 void arm_lms_f32( 00182 const arm_lms_instance_f32 * S, 00183 float32_t * pSrc, 00184 float32_t * pRef, 00185 float32_t * pOut, 00186 float32_t * pErr, 00187 uint32_t blockSize) 00188 { 00189 float32_t *pState = S->pState; /* State pointer */ 00190 float32_t *pCoeffs = S->pCoeffs; /* Coefficient pointer */ 00191 float32_t *pStateCurnt; /* Points to the current sample of the state */ 00192 float32_t *px, *pb; /* Temporary pointers for state and coefficient buffers */ 00193 float32_t mu = S->mu; /* Adaptive factor */ 00194 uint32_t numTaps = S->numTaps; /* Number of filter coefficients in the filter */ 00195 uint32_t tapCnt, blkCnt; /* Loop counters */ 00196 float32_t sum, e, d; /* accumulator, error, reference data sample */ 00197 float32_t w = 0.0f; /* weight factor */ 00198 00199 e = 0.0f; 00200 d = 0.0f; 00201 00202 /* S->pState points to state array which contains previous frame (numTaps - 1) samples */ 00203 /* pStateCurnt points to the location where the new input data should be written */ 00204 pStateCurnt = &(S->pState[(numTaps - 1u)]); 00205 00206 blkCnt = blockSize; 00207 00208 00209 #ifndef ARM_MATH_CM0_FAMILY 00210 00211 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00212 00213 while(blkCnt > 0u) 00214 { 00215 /* Copy the new input sample into the state buffer */ 00216 *pStateCurnt++ = *pSrc++; 00217 00218 /* Initialize pState pointer */ 00219 px = pState; 00220 00221 /* Initialize coeff pointer */ 00222 pb = (pCoeffs); 00223 00224 /* Set the accumulator to zero */ 00225 sum = 0.0f; 00226 00227 /* Loop unrolling. Process 4 taps at a time. */ 00228 tapCnt = numTaps >> 2; 00229 00230 while(tapCnt > 0u) 00231 { 00232 /* Perform the multiply-accumulate */ 00233 sum += (*px++) * (*pb++); 00234 sum += (*px++) * (*pb++); 00235 sum += (*px++) * (*pb++); 00236 sum += (*px++) * (*pb++); 00237 00238 /* Decrement the loop counter */ 00239 tapCnt--; 00240 } 00241 00242 /* If the filter length is not a multiple of 4, compute the remaining filter taps */ 00243 tapCnt = numTaps % 0x4u; 00244 00245 while(tapCnt > 0u) 00246 { 00247 /* Perform the multiply-accumulate */ 00248 sum += (*px++) * (*pb++); 00249 00250 /* Decrement the loop counter */ 00251 tapCnt--; 00252 } 00253 00254 /* The result in the accumulator, store in the destination buffer. */ 00255 *pOut++ = sum; 00256 00257 /* Compute and store error */ 00258 d = (float32_t) (*pRef++); 00259 e = d - sum; 00260 *pErr++ = e; 00261 00262 /* Calculation of Weighting factor for the updating filter coefficients */ 00263 w = e * mu; 00264 00265 /* Initialize pState pointer */ 00266 px = pState; 00267 00268 /* Initialize coeff pointer */ 00269 pb = (pCoeffs); 00270 00271 /* Loop unrolling. Process 4 taps at a time. */ 00272 tapCnt = numTaps >> 2; 00273 00274 /* Update filter coefficients */ 00275 while(tapCnt > 0u) 00276 { 00277 /* Perform the multiply-accumulate */ 00278 *pb = *pb + (w * (*px++)); 00279 pb++; 00280 00281 *pb = *pb + (w * (*px++)); 00282 pb++; 00283 00284 *pb = *pb + (w * (*px++)); 00285 pb++; 00286 00287 *pb = *pb + (w * (*px++)); 00288 pb++; 00289 00290 /* Decrement the loop counter */ 00291 tapCnt--; 00292 } 00293 00294 /* If the filter length is not a multiple of 4, compute the remaining filter taps */ 00295 tapCnt = numTaps % 0x4u; 00296 00297 while(tapCnt > 0u) 00298 { 00299 /* Perform the multiply-accumulate */ 00300 *pb = *pb + (w * (*px++)); 00301 pb++; 00302 00303 /* Decrement the loop counter */ 00304 tapCnt--; 00305 } 00306 00307 /* Advance state pointer by 1 for the next sample */ 00308 pState = pState + 1; 00309 00310 /* Decrement the loop counter */ 00311 blkCnt--; 00312 } 00313 00314 00315 /* Processing is complete. Now copy the last numTaps - 1 samples to the 00316 satrt of the state buffer. This prepares the state buffer for the 00317 next function call. */ 00318 00319 /* Points to the start of the pState buffer */ 00320 pStateCurnt = S->pState; 00321 00322 /* Loop unrolling for (numTaps - 1u) samples copy */ 00323 tapCnt = (numTaps - 1u) >> 2u; 00324 00325 /* copy data */ 00326 while(tapCnt > 0u) 00327 { 00328 *pStateCurnt++ = *pState++; 00329 *pStateCurnt++ = *pState++; 00330 *pStateCurnt++ = *pState++; 00331 *pStateCurnt++ = *pState++; 00332 00333 /* Decrement the loop counter */ 00334 tapCnt--; 00335 } 00336 00337 /* Calculate remaining number of copies */ 00338 tapCnt = (numTaps - 1u) % 0x4u; 00339 00340 /* Copy the remaining q31_t data */ 00341 while(tapCnt > 0u) 00342 { 00343 *pStateCurnt++ = *pState++; 00344 00345 /* Decrement the loop counter */ 00346 tapCnt--; 00347 } 00348 00349 #else 00350 00351 /* Run the below code for Cortex-M0 */ 00352 00353 while(blkCnt > 0u) 00354 { 00355 /* Copy the new input sample into the state buffer */ 00356 *pStateCurnt++ = *pSrc++; 00357 00358 /* Initialize pState pointer */ 00359 px = pState; 00360 00361 /* Initialize pCoeffs pointer */ 00362 pb = pCoeffs; 00363 00364 /* Set the accumulator to zero */ 00365 sum = 0.0f; 00366 00367 /* Loop over numTaps number of values */ 00368 tapCnt = numTaps; 00369 00370 while(tapCnt > 0u) 00371 { 00372 /* Perform the multiply-accumulate */ 00373 sum += (*px++) * (*pb++); 00374 00375 /* Decrement the loop counter */ 00376 tapCnt--; 00377 } 00378 00379 /* The result is stored in the destination buffer. */ 00380 *pOut++ = sum; 00381 00382 /* Compute and store error */ 00383 d = (float32_t) (*pRef++); 00384 e = d - sum; 00385 *pErr++ = e; 00386 00387 /* Weighting factor for the LMS version */ 00388 w = e * mu; 00389 00390 /* Initialize pState pointer */ 00391 px = pState; 00392 00393 /* Initialize pCoeffs pointer */ 00394 pb = pCoeffs; 00395 00396 /* Loop over numTaps number of values */ 00397 tapCnt = numTaps; 00398 00399 while(tapCnt > 0u) 00400 { 00401 /* Perform the multiply-accumulate */ 00402 *pb = *pb + (w * (*px++)); 00403 pb++; 00404 00405 /* Decrement the loop counter */ 00406 tapCnt--; 00407 } 00408 00409 /* Advance state pointer by 1 for the next sample */ 00410 pState = pState + 1; 00411 00412 /* Decrement the loop counter */ 00413 blkCnt--; 00414 } 00415 00416 00417 /* Processing is complete. Now copy the last numTaps - 1 samples to the 00418 * start of the state buffer. This prepares the state buffer for the 00419 * next function call. */ 00420 00421 /* Points to the start of the pState buffer */ 00422 pStateCurnt = S->pState; 00423 00424 /* Copy (numTaps - 1u) samples */ 00425 tapCnt = (numTaps - 1u); 00426 00427 /* Copy the data */ 00428 while(tapCnt > 0u) 00429 { 00430 *pStateCurnt++ = *pState++; 00431 00432 /* Decrement the loop counter */ 00433 tapCnt--; 00434 } 00435 00436 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00437 00438 } 00439 00440 /** 00441 * @} end of LMS group 00442 */
Generated on Tue Jul 12 2022 12:36:55 by
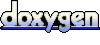