CMSIS DSP library
Dependents: KL25Z_FFT_Demo Hat_Board_v5_1 KL25Z_FFT_Demo_tony KL25Z_FFT_Demo_tony ... more
Fork of mbed-dsp by
arm_cmplx_mult_real_q31.c
00001 /* ---------------------------------------------------------------------- 00002 * Copyright (C) 2010-2013 ARM Limited. All rights reserved. 00003 * 00004 * $Date: 17. January 2013 00005 * $Revision: V1.4.1 00006 * 00007 * Project: CMSIS DSP Library 00008 * Title: arm_cmplx_mult_real_q31.c 00009 * 00010 * Description: Q31 complex by real multiplication 00011 * 00012 * Target Processor: Cortex-M4/Cortex-M3/Cortex-M0 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted provided that the following conditions 00016 * are met: 00017 * - Redistributions of source code must retain the above copyright 00018 * notice, this list of conditions and the following disclaimer. 00019 * - Redistributions in binary form must reproduce the above copyright 00020 * notice, this list of conditions and the following disclaimer in 00021 * the documentation and/or other materials provided with the 00022 * distribution. 00023 * - Neither the name of ARM LIMITED nor the names of its contributors 00024 * may be used to endorse or promote products derived from this 00025 * software without specific prior written permission. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00028 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00029 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00030 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE 00031 * COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00032 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00033 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; 00034 * LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00036 * LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN 00037 * ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00038 * POSSIBILITY OF SUCH DAMAGE. 00039 * -------------------------------------------------------------------- */ 00040 00041 #include "arm_math.h" 00042 00043 /** 00044 * @ingroup groupCmplxMath 00045 */ 00046 00047 /** 00048 * @addtogroup CmplxByRealMult 00049 * @{ 00050 */ 00051 00052 00053 /** 00054 * @brief Q31 complex-by-real multiplication 00055 * @param[in] *pSrcCmplx points to the complex input vector 00056 * @param[in] *pSrcReal points to the real input vector 00057 * @param[out] *pCmplxDst points to the complex output vector 00058 * @param[in] numSamples number of samples in each vector 00059 * @return none. 00060 * 00061 * <b>Scaling and Overflow Behavior:</b> 00062 * \par 00063 * The function uses saturating arithmetic. 00064 * Results outside of the allowable Q31 range[0x80000000 0x7FFFFFFF] will be saturated. 00065 */ 00066 00067 void arm_cmplx_mult_real_q31( 00068 q31_t * pSrcCmplx, 00069 q31_t * pSrcReal, 00070 q31_t * pCmplxDst, 00071 uint32_t numSamples) 00072 { 00073 q31_t inA1; /* Temporary variable to store input value */ 00074 00075 #ifndef ARM_MATH_CM0_FAMILY 00076 00077 /* Run the below code for Cortex-M4 and Cortex-M3 */ 00078 uint32_t blkCnt; /* loop counters */ 00079 q31_t inA2, inA3, inA4; /* Temporary variables to hold input data */ 00080 q31_t inB1, inB2; /* Temporary variabels to hold input data */ 00081 q31_t out1, out2, out3, out4; /* Temporary variables to hold output data */ 00082 00083 /* loop Unrolling */ 00084 blkCnt = numSamples >> 2u; 00085 00086 /* First part of the processing with loop unrolling. Compute 4 outputs at a time. 00087 ** a second loop below computes the remaining 1 to 3 samples. */ 00088 while(blkCnt > 0u) 00089 { 00090 /* C[2 * i] = A[2 * i] * B[i]. */ 00091 /* C[2 * i + 1] = A[2 * i + 1] * B[i]. */ 00092 /* read real input from complex input buffer */ 00093 inA1 = *pSrcCmplx++; 00094 inA2 = *pSrcCmplx++; 00095 /* read input from real input bufer */ 00096 inB1 = *pSrcReal++; 00097 inB2 = *pSrcReal++; 00098 /* read imaginary input from complex input buffer */ 00099 inA3 = *pSrcCmplx++; 00100 inA4 = *pSrcCmplx++; 00101 00102 /* multiply complex input with real input */ 00103 out1 = ((q63_t) inA1 * inB1) >> 32; 00104 out2 = ((q63_t) inA2 * inB1) >> 32; 00105 out3 = ((q63_t) inA3 * inB2) >> 32; 00106 out4 = ((q63_t) inA4 * inB2) >> 32; 00107 00108 /* sature the result */ 00109 out1 = __SSAT(out1, 31); 00110 out2 = __SSAT(out2, 31); 00111 out3 = __SSAT(out3, 31); 00112 out4 = __SSAT(out4, 31); 00113 00114 /* get result in 1.31 format */ 00115 out1 = out1 << 1; 00116 out2 = out2 << 1; 00117 out3 = out3 << 1; 00118 out4 = out4 << 1; 00119 00120 /* store the result to destination buffer */ 00121 *pCmplxDst++ = out1; 00122 *pCmplxDst++ = out2; 00123 *pCmplxDst++ = out3; 00124 *pCmplxDst++ = out4; 00125 00126 /* read real input from complex input buffer */ 00127 inA1 = *pSrcCmplx++; 00128 inA2 = *pSrcCmplx++; 00129 /* read input from real input bufer */ 00130 inB1 = *pSrcReal++; 00131 inB2 = *pSrcReal++; 00132 /* read imaginary input from complex input buffer */ 00133 inA3 = *pSrcCmplx++; 00134 inA4 = *pSrcCmplx++; 00135 00136 /* multiply complex input with real input */ 00137 out1 = ((q63_t) inA1 * inB1) >> 32; 00138 out2 = ((q63_t) inA2 * inB1) >> 32; 00139 out3 = ((q63_t) inA3 * inB2) >> 32; 00140 out4 = ((q63_t) inA4 * inB2) >> 32; 00141 00142 /* sature the result */ 00143 out1 = __SSAT(out1, 31); 00144 out2 = __SSAT(out2, 31); 00145 out3 = __SSAT(out3, 31); 00146 out4 = __SSAT(out4, 31); 00147 00148 /* get result in 1.31 format */ 00149 out1 = out1 << 1; 00150 out2 = out2 << 1; 00151 out3 = out3 << 1; 00152 out4 = out4 << 1; 00153 00154 /* store the result to destination buffer */ 00155 *pCmplxDst++ = out1; 00156 *pCmplxDst++ = out2; 00157 *pCmplxDst++ = out3; 00158 *pCmplxDst++ = out4; 00159 00160 /* Decrement the numSamples loop counter */ 00161 blkCnt--; 00162 } 00163 00164 /* If the numSamples is not a multiple of 4, compute any remaining output samples here. 00165 ** No loop unrolling is used. */ 00166 blkCnt = numSamples % 0x4u; 00167 00168 while(blkCnt > 0u) 00169 { 00170 /* C[2 * i] = A[2 * i] * B[i]. */ 00171 /* C[2 * i + 1] = A[2 * i + 1] * B[i]. */ 00172 /* read real input from complex input buffer */ 00173 inA1 = *pSrcCmplx++; 00174 inA2 = *pSrcCmplx++; 00175 /* read input from real input bufer */ 00176 inB1 = *pSrcReal++; 00177 00178 /* multiply complex input with real input */ 00179 out1 = ((q63_t) inA1 * inB1) >> 32; 00180 out2 = ((q63_t) inA2 * inB1) >> 32; 00181 00182 /* sature the result */ 00183 out1 = __SSAT(out1, 31); 00184 out2 = __SSAT(out2, 31); 00185 00186 /* get result in 1.31 format */ 00187 out1 = out1 << 1; 00188 out2 = out2 << 1; 00189 00190 /* store the result to destination buffer */ 00191 *pCmplxDst++ = out1; 00192 *pCmplxDst++ = out2; 00193 00194 /* Decrement the numSamples loop counter */ 00195 blkCnt--; 00196 } 00197 00198 #else 00199 00200 /* Run the below code for Cortex-M0 */ 00201 00202 while(numSamples > 0u) 00203 { 00204 /* realOut = realA * realB. */ 00205 /* imagReal = imagA * realB. */ 00206 inA1 = *pSrcReal++; 00207 /* store the result in the destination buffer. */ 00208 *pCmplxDst++ = 00209 (q31_t) clip_q63_to_q31(((q63_t) * pSrcCmplx++ * inA1) >> 31); 00210 *pCmplxDst++ = 00211 (q31_t) clip_q63_to_q31(((q63_t) * pSrcCmplx++ * inA1) >> 31); 00212 00213 /* Decrement the numSamples loop counter */ 00214 numSamples--; 00215 } 00216 00217 #endif /* #ifndef ARM_MATH_CM0_FAMILY */ 00218 00219 } 00220 00221 /** 00222 * @} end of CmplxByRealMult group 00223 */
Generated on Tue Jul 12 2022 12:36:54 by
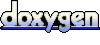