
スマホのアプリで温度を表示するプログラム
Dependencies: BLE_API mbed nRF51822
main.cpp
00001 #include "mbed.h" 00002 #include "BLE.h" 00003 #include "config.h" 00004 00005 #define MY_NAME "@toyowata" 00006 00007 const static char DEVICE_NAME[] = MY_NAME; 00008 static volatile bool triggerSensorPolling = false; 00009 00010 BLEDevice ble; 00011 AnalogIn ain(P0_2); 00012 00013 /* LEDs for indication: */ 00014 DigitalOut oneSecondLed(LED2, LED_OFF); /* LED2 is toggled every second. */ 00015 DigitalOut advertisingStateLed(LED1, LED_OFF); /* LED1 is on when we are advertising, otherwise off. */ 00016 00017 /* Health Thermometer Service */ 00018 uint8_t thermTempPayload[5] = { 0, 0, 0, 0, 0 }; 00019 00020 GattCharacteristic tempChar (GattCharacteristic::UUID_TEMPERATURE_MEASUREMENT_CHAR, 00021 thermTempPayload, 5, 5, 00022 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_INDICATE); 00023 /* Battery Level Service */ 00024 uint8_t batt = 100; /* Battery level */ 00025 uint8_t read_batt = 0; /* Variable to hold battery level reads */ 00026 GattCharacteristic battLevel ( GattCharacteristic::UUID_BATTERY_LEVEL_CHAR, 00027 (uint8_t *)batt, 1, 1, 00028 GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY); 00029 GattCharacteristic *htmChars[] = {&tempChar, }; 00030 GattCharacteristic *battChars[] = {&battLevel, }; 00031 GattService htmService(GattService::UUID_HEALTH_THERMOMETER_SERVICE, htmChars, 00032 sizeof(htmChars) / sizeof(GattCharacteristic *)); 00033 GattService battService(GattService::UUID_BATTERY_SERVICE, battChars, 00034 sizeof(battChars) / sizeof(GattCharacteristic *)); 00035 00036 uint16_t uuid16_list[] = {GattService::UUID_HEALTH_THERMOMETER_SERVICE, 00037 GattService::UUID_BATTERY_SERVICE}; 00038 00039 uint32_t quick_ieee11073_from_float(float temperature); 00040 void updateServiceValues(void); 00041 00042 static Gap::ConnectionParams_t connectionParams; 00043 00044 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) // Mod 00045 { 00046 advertisingStateLed = LED_OFF; 00047 00048 DEBUG("Disconnected handle %u, reason %u\r\n", params->handle, params->reason); 00049 DEBUG("Restarting the advertising process\r\n"); 00050 ble.gap().startAdvertising(); 00051 } 00052 00053 void onConnectionCallback(const Gap::ConnectionCallbackParams_t *params) //Mod 00054 { 00055 advertisingStateLed = LED_ON; 00056 00057 DEBUG("connected. Got handle %u\r\n", params->handle); 00058 00059 connectionParams.slaveLatency = 1; 00060 if (ble.gap().updateConnectionParams(params->handle, &connectionParams) != BLE_ERROR_NONE) { 00061 DEBUG("failed to update connection paramter\r\n"); 00062 } 00063 } 00064 00065 void periodicCallback(void) 00066 { 00067 oneSecondLed = !oneSecondLed; /* Do blinky on LED1 while we're waiting for BLE events */ 00068 00069 /* Note that the periodicCallback() executes in interrupt context, so it is safer to do 00070 * heavy-weight sensor polling from the main thread. */ 00071 triggerSensorPolling = true; 00072 } 00073 00074 /**************************************************************************/ 00075 /*! 00076 @brief Program entry point 00077 */ 00078 /**************************************************************************/ 00079 int main(void) 00080 { 00081 00082 /* Setup blinky led */ 00083 oneSecondLed = 1; 00084 Ticker ticker; 00085 ticker.attach(periodicCallback, 0.5); 00086 00087 DEBUG("Initialising the nRF51822\r\n"); 00088 ble.init(); 00089 DEBUG("Init done\r\n"); 00090 ble.gap().onDisconnection(disconnectionCallback); 00091 ble.gap().onConnection(onConnectionCallback); 00092 00093 ble.gap().getPreferredConnectionParams(&connectionParams); 00094 00095 /* setup advertising */ 00096 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00097 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t*)uuid16_list, sizeof(uuid16_list)); 00098 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::GENERIC_THERMOMETER); 00099 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00100 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::SHORTENED_LOCAL_NAME, (uint8_t *)DEVICE_NAME, sizeof(DEVICE_NAME)); 00101 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00102 ble.gap().setAdvertisingInterval(160); /* 100ms; in multiples of 0.625ms. */ 00103 ble.gap().startAdvertising(); 00104 advertisingStateLed = LED_OFF; 00105 DEBUG("Start Advertising\r\n"); 00106 00107 ble.gattServer().addService(htmService); 00108 ble.gattServer().addService(battService); 00109 DEBUG("Add Service\r\n"); 00110 00111 while (true) { 00112 if (triggerSensorPolling) { 00113 triggerSensorPolling = false; 00114 updateServiceValues(); 00115 } else { 00116 ble.waitForEvent(); 00117 } 00118 } 00119 } 00120 00121 /**************************************************************************/ 00122 /*! 00123 @brief Ticker callback to switch advertisingStateLed state 00124 */ 00125 /**************************************************************************/ 00126 void updateServiceValues(void) 00127 { 00128 /* Decrement the battery level. */ 00129 batt <=50 ? batt=100 : batt--; 00130 00131 float temperature = (ain.read() * 3.2 - 0.6) / 0.01; 00132 00133 DEBUG("temp:%f\r\n", temperature); 00134 uint32_t temp_ieee11073 = quick_ieee11073_from_float(temperature); 00135 memcpy(thermTempPayload+1, &temp_ieee11073, 4); 00136 ble.gattServer().write(tempChar.getValueAttribute().getHandle(), thermTempPayload, sizeof(thermTempPayload)); //Mod 00137 ble.gattServer().write(battLevel.getValueAttribute().getHandle(), (uint8_t *)&batt, sizeof(batt)); //Mod 00138 } 00139 00140 /** 00141 * @brief A very quick conversion between a float temperature and 11073-20601 FLOAT-Type. 00142 * @param temperature The temperature as a float. 00143 * @return The temperature in 11073-20601 FLOAT-Type format. 00144 */ 00145 uint32_t quick_ieee11073_from_float(float temperature) 00146 { 00147 uint8_t exponent = 0xFF; //exponent is -1 00148 uint32_t mantissa = (uint32_t)(temperature*10); 00149 00150 return ( ((uint32_t)exponent) << 24) | mantissa; 00151 }
Generated on Tue Jul 12 2022 18:31:09 by
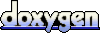