
Test for MS5803 and MS5837 pressure sensors (pressure and temp readings) and QEI testing for encoder.
Embed:
(wiki syntax)
Show/hide line numbers
MS5837.cpp
00001 #include <stdlib.h> 00002 #include "MS5837.h" 00003 00004 00005 /* 00006 * Sensor operating function according data sheet 00007 */ 00008 00009 void MS5837::MS5837Init(void) 00010 { 00011 MS5837Reset(); 00012 MS5837ReadProm(); 00013 return; 00014 } 00015 00016 /* Send soft reset to the sensor */ 00017 void MS5837::MS5837Reset(void) 00018 { 00019 /* transmit out 1 byte reset command */ 00020 ms5837_tx_data[0] = ms5837_reset; 00021 if ( i2c.write( device_address, ms5837_tx_data, 1 ) ); 00022 //printf("send soft reset"); 00023 wait_ms(20); 00024 } 00025 00026 /* read the sensor calibration data from rom */ 00027 void MS5837::MS5837ReadProm(void) 00028 { 00029 uint8_t i,j; 00030 for (i=0; i<8; i++) { 00031 j = i; 00032 ms5837_tx_data[0] = ms5837_PROMread + (j<<1); 00033 if ( i2c.write( device_address, ms5837_tx_data, 1 ) ); 00034 if ( i2c.read( device_address, ms5837_rx_data, 2 ) ); 00035 C[i] = ms5837_rx_data[1] + (ms5837_rx_data[0]<<8); 00036 } 00037 } 00038 00039 /* Start the sensor pressure conversion */ 00040 void MS5837::MS5837ConvertD1(void) 00041 { 00042 ms5837_tx_data[0] = ms5837_convD1; 00043 if ( i2c.write( device_address, ms5837_tx_data, 1 ) ); 00044 } 00045 00046 /* Start the sensor temperature conversion */ 00047 void MS5837:: MS5837ConvertD2(void) 00048 { 00049 ms5837_tx_data[0] = ms5837_convD2; 00050 if ( i2c.write( device_address, ms5837_tx_data, 1 ) ); 00051 } 00052 00053 /* Read the previous started conversion results */ 00054 int32_t MS5837::MS5837ReadADC(void) 00055 { 00056 int32_t adc; 00057 wait_ms(150); 00058 ms5837_tx_data[0] = ms5837_ADCread; 00059 if ( i2c.write( device_address, ms5837_tx_data, 1 ) ); 00060 if ( i2c.read( device_address, ms5837_rx_data, 3 ) ); 00061 adc = ms5837_rx_data[2] + (ms5837_rx_data[1]<<8) + (ms5837_rx_data[0]<<16); 00062 return (adc); 00063 } 00064 00065 /* return the results */ 00066 float MS5837::MS5837_Pressure (void) 00067 { 00068 return P_MS5837; 00069 } 00070 float MS5837::MS5837_Temperature (void) 00071 { 00072 return T_MS5837; 00073 } 00074 00075 /* Sensor reading and calculation procedure */ 00076 void MS5837::Barometer_MS5837(void) 00077 { 00078 int32_t dT, temp; 00079 int64_t OFF, SENS, press; 00080 00081 //no need to do this everytime! 00082 //MS5837Reset(); // reset the sensor 00083 //MS5837ReadProm(); // read the calibration values 00084 00085 00086 MS5837ConvertD1(); // start pressure conversion 00087 D1 = MS5837ReadADC(); // read the pressure value 00088 MS5837ConvertD2(); // start temperature conversion 00089 D2 = MS5837ReadADC(); // read the temperature value 00090 00091 /* calculation according MS5837-01BA data sheet DA5837-01BA_006 */ 00092 dT = D2 - (C[5]* 256); 00093 OFF = (int64_t)C[2] * (1<<16) + ((int64_t)dT * (int64_t)C[4]) / (1<<7); 00094 SENS = (int64_t)C[1] * (1<<15) + ((int64_t)dT * (int64_t)C[3]) / (1<<8); 00095 00096 temp = 2000 + (dT * C[6]) / (1<<23); 00097 T_MS5837 = (float) temp / 100.0f; // result of temperature in deg C in this var 00098 press = (((int64_t)D1 * SENS) / (1<<21) - OFF) / (1<<13); 00099 P_MS5837 = (float) press / 10.0f; // result of pressure in mBar in this var 00100 }
Generated on Fri Jul 15 2022 08:03:19 by
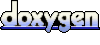