
wifi1
Dependencies: mbed
Fork of ESP8266-configuaration-baudrate by
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); 00004 Serial esp(p9,p10); // tx, rx 00005 00006 Timer t; 00007 00008 int count,ended,timeout; 00009 char buf[1024]; 00010 char snd[255]; 00011 00012 char ssid[32] = "CWMWIFI"; // enter router ssid inside the quotes 00013 char pwd [32] = "CWM2016TT"; // enter router password inside the quotes 00014 00015 void SendCMD(),getreply(),ESPconfig(),ESPsetbaudrate(); 00016 00017 00018 int main() { 00019 00020 pc.baud(115200); // set what you want here depending on your terminal program speed 00021 esp.baud(115200); // change this to the new ESP8266 baudrate if it is changed at any time. 00022 00023 //ESPsetbaudrate(); //****************** include this routine to set a different ESP8266 baudrate ****************** 00024 00025 ESPconfig(); //****************** include Config to set the ESP8266 configuration *********************** 00026 00027 00028 // contiuosly get AP list and IP 00029 while(1){ 00030 pc.printf("\n---------- Listing Acces Points ----------\r\n"); 00031 strcpy(snd, "AT+CWLAP\r\n"); 00032 SendCMD(); 00033 timeout=3; 00034 getreply(); 00035 pc.printf(buf); 00036 00037 pc.printf("\n---------- Get IP's ----------\r\n"); 00038 strcpy(snd, "AT+CIFSR\r\n"); 00039 SendCMD(); 00040 timeout=2; 00041 getreply(); 00042 pc.printf(buf); 00043 } 00044 00045 } 00046 00047 // Sets new ESP8266 baurate, change the esp.baud(xxxxx) to match your new setting once this has been executed 00048 void ESPsetbaudrate() 00049 { 00050 strcpy(snd, "AT+CIOBAUD=115200\r\n"); // change the numeric value to the required baudrate 00051 SendCMD(); 00052 } 00053 00054 // +++++++++++++++++++++++++++++++++ This is for ESP8266 config only, run this once to set up the ESP8266 +++++++++++++++ 00055 void ESPconfig() 00056 { 00057 00058 pc.printf("---------- Starting ESP Config ----------\r\n\n"); 00059 wait(2); 00060 pc.printf("---------- Reset & get Firmware ----------\r\n"); 00061 strcpy(snd,"AT+RST\r\n"); 00062 SendCMD(); 00063 timeout=2; 00064 getreply(); 00065 pc.printf(buf); 00066 00067 wait(1); 00068 00069 pc.printf("\n---------- Get Version ----------\r\n"); 00070 strcpy(snd,"AT+GMR\r\n"); 00071 SendCMD(); 00072 timeout=1; 00073 getreply(); 00074 pc.printf(buf); 00075 00076 wait(1); 00077 00078 // set CWMODE to 1=Station,2=AP,3=BOTH, default mode 1 (Station) 00079 pc.printf("\n---------- Setting Mode ----------\r\n"); 00080 strcpy(snd, "AT+CWMODE=1\r\n"); 00081 SendCMD(); 00082 timeout=1; 00083 getreply(); 00084 pc.printf(buf); 00085 00086 wait(1); 00087 00088 // set CIPMUX to 0=Single,1=Multi 00089 pc.printf("\n---------- Setting Connection Mode ----------\r\n"); 00090 strcpy(snd, "AT+CIPMUX=1\r\n"); 00091 SendCMD(); 00092 timeout=1; 00093 getreply(); 00094 pc.printf(buf); 00095 00096 wait(1); 00097 00098 pc.printf("\n---------- Listing Acces Points ----------\r\n"); 00099 strcpy(snd, "AT+CWLAP\r\n"); 00100 SendCMD(); 00101 timeout=3; 00102 getreply(); 00103 pc.printf(buf); 00104 00105 wait(1); 00106 00107 pc.printf("\n---------- Connecting to AP ----------\r\n"); 00108 pc.printf("ssid = %s pwd = %s\r\n",ssid,pwd); 00109 strcpy(snd, "AT+CWJAP=\""); 00110 strcat(snd, ssid); 00111 strcat(snd, "\",\""); 00112 strcat(snd, pwd); 00113 strcat(snd, "\"\r\n"); 00114 SendCMD(); 00115 timeout=8; 00116 getreply(); 00117 pc.printf(buf); 00118 00119 wait(5); 00120 00121 pc.printf("\n---------- Get IP's ----------\r\n"); 00122 strcpy(snd, "AT+CIFSR\r\n"); 00123 SendCMD(); 00124 timeout=2; 00125 getreply(); 00126 pc.printf(buf); 00127 00128 wait(1); 00129 00130 pc.printf("\n---------- Get Connection Status ----------\r\n"); 00131 strcpy(snd, "AT+CIPSTATUS\r\n"); 00132 SendCMD(); 00133 timeout=2; 00134 getreply(); 00135 pc.printf(buf); 00136 00137 pc.printf("\n\n\n If you get a valid IP, ESP8266 has been set up.\r\n"); 00138 pc.printf(" Run this if you want to reconfig the ESP8266 at any time.\r\n"); 00139 } 00140 00141 void SendCMD() 00142 { 00143 esp.printf("%s", snd); 00144 } 00145 00146 void getreply() 00147 { 00148 memset(buf, '\0', sizeof(buf)); 00149 t.start(); 00150 ended=0;count=0; 00151 while(!ended) { 00152 if(esp.readable()) { 00153 buf[count] = esp.getc();count++; 00154 } 00155 if(t.read() > timeout) { 00156 ended = 1;t.stop();t.reset(); 00157 } 00158 } 00159 } 00160
Generated on Sat Jul 16 2022 14:04:11 by
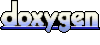