
test avoid bulled game
Dependencies: C12832_lcd LCD_fonts mbed mbed-rtos
Person.cpp
00001 #include "point.h" 00002 #include "models.h" 00003 00004 Person::Person(){ 00005 p.x = DEFAULT_X; 00006 p.y = DEFAULT_Y; 00007 jump_count = 0; 00008 jump_time = 0; 00009 } 00010 00011 point Person::update(int h){ 00012 int height = LCD_Y - h - 1; 00013 if(jump_time > 0){ 00014 //while jumpping 00015 p.y -= JUMP_SIZE; 00016 jump_time--; 00017 }else{ 00018 //while down or go 00019 if(isGround(height)){ 00020 //while go 00021 jump_count = 0; 00022 }else{ 00023 //while down 00024 p.y += JUMP_SIZE; 00025 } 00026 } 00027 return p; 00028 } 00029 00030 point Person::jump(){ 00031 //start jump 00032 if(jump_count < MAX_JUMP_COUNT){ 00033 p.y -= JUMP_SIZE; 00034 jump_time = MAX_JUMP_TIME; 00035 jump_count++; 00036 } 00037 return p; 00038 } 00039 00040 bool Person::isGround(int height){ 00041 return height == (p.y + PERSON_SIZE - 1); 00042 }
Generated on Wed Jul 13 2022 00:30:16 by
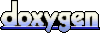