Library for the Shield Bot by SeeedStudio
Dependents: Seeed_Bot_Shield Seeed_BlueBot_demo Seeed_BlueBot_demo_test1 LAB03_Oppgav1 ... more
Fork of SeeedShieldBot by
SeeedStudioShieldBot.h
00001 #include "mbed.h" 00002 00003 /** Seeed Studio Shield Bot Control Class 00004 * In order to use this properly, you need to connect a jumper between pins eight and three on the shield bot, and you can't use either pins 8 or 3, which correspond to PTA13 and PTA12, respectively. 00005 * Also, in order to provide power to the freedom board, when running just of a lipo battery, you need to connect between 5V and VIN on the shield bot. 00006 * Code/notes above only tested with version 0.9b, may not be needed/may not work in other cases... 00007 */ 00008 class SeeedStudioShieldBot { 00009 public: 00010 00011 /** Create a shield bot object 00012 * 00013 * @param mot1A Left Motor A pin, default D5 00014 * @param mot1B Left Motor B pin, default D6 00015 * @param mot1En Left Motor enable pin, default D7 00016 * @param mot2A Right Motor A pin, default D8 00017 * @param mot2B Right Motor B pin, default D9 00018 * @param mot2En Right Motor enable pin, default D10 00019 * @param sensor_right Sensor right pin, default A0 00020 * @param sensor_inright Sensor in-right pin, default A1 00021 * @param sensor_center Sensor right pin, default A2 00022 * @param sensor_inleft Sensor in-left pin, default A3 00023 * @param sensor_left Sensor left pin, default D4 00024 */ 00025 SeeedStudioShieldBot(PinName mot1A, PinName mot1En, PinName mot1B, PinName mot2A, PinName mot2En, PinName mot2B, PinName sensor_right, PinName sensor_inright, PinName sensor_center, PinName sensor_inleft, PinName sensor_left); 00026 00027 /** Switch on the left motor at the given speed. 00028 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00029 */ 00030 void left_motor(float speed); 00031 00032 /** Switch on the right motor at the given speed. 00033 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00034 */ 00035 void right_motor(float speed); 00036 00037 /** Switch on both motors, forwards at the given speed. 00038 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00039 */ 00040 void forward(float speed); 00041 00042 /** Switch on both motors, backwards at the given speed. 00043 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00044 */ 00045 void backward(float speed); 00046 00047 /** Switch on both motors at the given speed, in opposite directions so as to turn left. 00048 * @param speed The speed, from 0.0 to 1.0 at which to spin the motors. 00049 */ 00050 void left(float speed); 00051 00052 /** Switch on both motors at the given speed, in opposite directions so as to turn right. 00053 * @param speed The speed, from 0.0 to 1.0 at which to spin the motors. 00054 */ 00055 void right(float speed); 00056 00057 /** Turns left. 00058 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00059 */ 00060 void turn_left(float speed); 00061 00062 /** Turns right. 00063 * @param speed The speed, from 0.0 to 1.0 at which to spin the motor. 00064 */ 00065 void turn_right(float speed); 00066 00067 /** Disable the left motor, by driving enable pin for the second motor low... 00068 */ 00069 void disable_left_motor(); 00070 00071 /** Disable the left motor, by driving enable pin for the first motor low... 00072 */ 00073 void disable_right_motor(); 00074 00075 /** Enable the left motor, by driving enable pin for the second motor high... 00076 */ 00077 void enable_left_motor(); 00078 00079 /** Enable the left motor, by driving enable pin for the first motor high... 00080 */ 00081 void enable_right_motor(); 00082 00083 /** Stop a chosen motor. 00084 * @param motor Number, either 1 or 2 choosing the motor. 00085 */ 00086 void stop(int motor); 00087 00088 /** Stop left motor. 00089 */ 00090 void stopLeft(); 00091 00092 /** Stop right motor. 00093 */ 00094 void stopRight(); 00095 00096 /** Stop both motors at the same time. Different to disable. 00097 */ 00098 void stopAll(); 00099 00100 // Need to do something to do with detected line... 00101 00102 /** Gives an indication of the data given by the reflectivity sensors. 00103 */ 00104 float line_position(); 00105 00106 DigitalIn rightSensor; 00107 DigitalIn inRightSensor; 00108 DigitalIn centreSensor; 00109 DigitalIn inLeftSensor; 00110 DigitalIn leftSensor; 00111 00112 private: 00113 PwmOut motor1A; 00114 DigitalOut motor1B; 00115 DigitalOut motor1En; 00116 00117 PwmOut motor2A; 00118 DigitalOut motor2B; 00119 DigitalOut motor2En; 00120 };
Generated on Tue Jul 12 2022 15:19:06 by
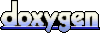