Virtual base class for sensors that measure motion
Dependents: FXOS8700Q MAG3110 MMA8451Q MAG3110 ... more
MotionSensor.h
00001 /* MotionSensor Base Class 00002 * Copyright (c) 2014-2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef MOTIONSENSOR_H 00018 #define MOTIONSENSOR_H 00019 00020 #include "stdint.h" 00021 00022 /** motion_data_counts_t struct 00023 */ 00024 typedef struct { 00025 int16_t x ; /*!< x-axis counts */ 00026 int16_t y ; /*!< y-axis counts */ 00027 int16_t z ; /*!< z-axis counts */ 00028 } motion_data_counts_t; 00029 00030 /** motion_data_units_t struct 00031 */ 00032 typedef struct { 00033 float x ; /*!< x-axis counts */ 00034 float y ; /*!< y-axis counts */ 00035 float z ; /*!< z-axis counts */ 00036 } motion_data_units_t; 00037 00038 00039 /** Motion Sensor Base Class 00040 Useful for accessing data in a common way 00041 */ 00042 class MotionSensor 00043 { 00044 public: 00045 00046 /** Enable the sensor for operation 00047 */ 00048 virtual void enable(void) const = 0; 00049 00050 /** disable the sensors operation 00051 */ 00052 virtual void disable(void) const = 0; 00053 00054 /** Set the sensor sample rate 00055 @param frequency The desires sample frequency 00056 @return The amount of error in Hz between desired and actual frequency 00057 */ 00058 virtual uint32_t sampleRate(uint32_t frequency) const = 0; 00059 00060 /** Tells of new data is ready 00061 @return The amount of data samples ready to be read from a device 00062 */ 00063 virtual uint32_t dataReady(void) const = 0; 00064 00065 /** Get the x data in counts 00066 @param x A referene to the variable to put the data in, 0 denotes not used 00067 @return The x data in counts 00068 */ 00069 virtual int16_t getX(int16_t &x) const = 0; 00070 00071 /** Get the y data in counts 00072 @param y A referene to the variable to put the data in, 0 denotes not used 00073 @return The y data in counts 00074 */ 00075 virtual int16_t getY(int16_t &y) const = 0; 00076 00077 /** Get the z data in counts 00078 @param z A referene to the variable to put the data in, 0 denotes not used 00079 @return The z data in counts 00080 */ 00081 virtual int16_t getZ(int16_t &z) const = 0; 00082 00083 /** Get the x data in units 00084 @param x A referene to the variable to put the data in, 0 denotes not used 00085 @return The x data in units 00086 */ 00087 virtual float getX(float &x) const = 0; 00088 00089 /** Get the y data in units 00090 @param y A referene to the variable to put the data in, 0 denotes not used 00091 @return The y data in units 00092 */ 00093 virtual float getY(float &y) const = 0; 00094 00095 /** Get the z data in units 00096 @param z A referene to the variable to put the data in, 0 denotes not used 00097 @return The z data in units 00098 */ 00099 virtual float getZ(float &z) const = 0; 00100 00101 /** Get the x,y,z data in counts 00102 @param xyz A referene to the variable to put the data in, 0 denotes not used 00103 */ 00104 virtual void getAxis(motion_data_counts_t &xyz) const = 0; 00105 00106 /** Get the x,y,z data in units 00107 @param xyz A referene to the variable to put the data in, 0 denotes not used 00108 */ 00109 virtual void getAxis(motion_data_units_t &xyz) const = 0; 00110 }; 00111 00112 #endif
Generated on Tue Jul 12 2022 15:29:52 by
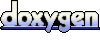