Driver for the ESP8266 WiFi module using ATParser library. Espressif Firmware.
Dependencies: ATParser
Fork of ESP8266 by
ESP8266.cpp
00001 /* ESP8266 Example 00002 * Copyright (c) 2015 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "ESP8266.h" 00018 00019 ESP8266::ESP8266(PinName tx, PinName rx, bool debug) 00020 : _serial(tx, rx, 1024), _parser(_serial) 00021 { 00022 _serial.baud(115200); 00023 _parser.debugOn(debug); 00024 } 00025 00026 bool ESP8266::startup(int mode) 00027 { 00028 //only 3 valid modes 00029 if(mode < 1 || mode > 3) { 00030 return false; 00031 } 00032 00033 return reset() 00034 && _parser.send("AT+CWMODE=%d", mode) 00035 && _parser.recv("OK") 00036 && _parser.send("AT+CIPMUX=1") 00037 && _parser.recv("OK"); 00038 } 00039 00040 bool ESP8266::reset(void) 00041 { 00042 for (int i = 0; i < 2; i++) { 00043 if (_parser.send("AT+RST") 00044 && _parser.recv("OK\r\nready")) { 00045 return true; 00046 } 00047 } 00048 00049 return false; 00050 } 00051 00052 bool ESP8266::dhcp(bool enabled, int mode) 00053 { 00054 //only 3 valid modes 00055 if(mode < 0 || mode > 2) { 00056 return false; 00057 } 00058 00059 return _parser.send("AT+CWDHCP=%d,%d", enabled?1:0, mode) 00060 && _parser.recv("OK"); 00061 } 00062 00063 bool ESP8266::connect(const char *ap, const char *passPhrase) 00064 { 00065 return _parser.send("AT+CWJAP=\"%s\",\"%s\"", ap, passPhrase) 00066 && _parser.recv("OK"); 00067 } 00068 00069 bool ESP8266::disconnect(void) 00070 { 00071 return _parser.send("AT+CWQAP") && _parser.recv("OK"); 00072 } 00073 00074 const char *ESP8266::getIPAddress(void) 00075 { 00076 if (!(_parser.send("AT+CIFSR") 00077 && _parser.recv("+CIFSR:STAIP,\"%[^\"]\"", _ip_buffer) 00078 && _parser.recv("OK"))) { 00079 return 0; 00080 } 00081 00082 return _ip_buffer; 00083 } 00084 00085 const char *ESP8266::getMACAddress(void) 00086 { 00087 if (!(_parser.send("AT+CIFSR") 00088 && _parser.recv("+CIFSR:STAMAC,\"%[^\"]\"", _mac_buffer) 00089 && _parser.recv("OK"))) { 00090 return 0; 00091 } 00092 00093 return _mac_buffer; 00094 } 00095 00096 bool ESP8266::isConnected(void) 00097 { 00098 return getIPAddress() != 0; 00099 } 00100 00101 bool ESP8266::open(const char *type, int id, const char* addr, int port) 00102 { 00103 //IDs only 0-4 00104 if(id > 4) { 00105 return false; 00106 } 00107 00108 return _parser.send("AT+CIPSTART=%d,\"%s\",\"%s\",%d", id, type, addr, port) 00109 && _parser.recv("OK"); 00110 } 00111 00112 bool ESP8266::send(int id, const void *data, uint32_t amount) 00113 { 00114 //May take a second try if device is busy 00115 for (unsigned i = 0; i < 2; i++) { 00116 if (_parser.send("AT+CIPSEND=%d,%d", id, amount) 00117 && _parser.recv(">") 00118 && _parser.write((char*)data, (int)amount) >= 0) { 00119 return true; 00120 } 00121 } 00122 00123 return false; 00124 } 00125 00126 int32_t ESP8266::recv(int id, void *data, uint32_t amount) 00127 { 00128 uint32_t recv_amount; 00129 int recv_id; 00130 00131 if (!(_parser.recv("+IPD,%d,%d:", &recv_id, &recv_amount) 00132 && recv_id == id 00133 && recv_amount <= amount 00134 && _parser.read((char*)data, recv_amount) 00135 && _parser.recv("OK"))) { 00136 return -1; 00137 } 00138 00139 return recv_amount; 00140 } 00141 00142 bool ESP8266::close(int id) 00143 { 00144 //May take a second try if device is busy 00145 for (unsigned i = 0; i < 2; i++) { 00146 if (_parser.send("AT+CIPCLOSE=%d", id) 00147 && _parser.recv("OK")) { 00148 return true; 00149 } 00150 } 00151 00152 return false; 00153 } 00154 00155 void ESP8266::setTimeout(uint32_t timeout_ms) 00156 { 00157 _parser.setTimeout(timeout_ms); 00158 } 00159 00160 bool ESP8266::readable() 00161 { 00162 return _serial.readable(); 00163 } 00164 00165 bool ESP8266::writeable() 00166 { 00167 return _serial.writeable(); 00168 } 00169
Generated on Wed Jul 20 2022 22:18:34 by
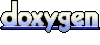