
Import of CSR's demo for SirfV. Has minor cleanup.
Dependencies: CsrLocation mbed GPSProvider
Fork of CsrLocationDemo by
CsrLocationDemo.cpp
00001 /* CSRLocation class for mbed Microcontroller 00002 * Copyright 2014 CSR plc 00003 */ 00004 00005 #include "mbed.h" 00006 #include "GPSProvider.h" 00007 00008 #define PINMAP_GPIO_BTN D5 00009 #define PINMAP_GPIO_TEST D10 00010 #define LOC_LED1 D7 00011 #define LOC_LED2 D6 00012 00013 #define APP_DBG_PORT_BAUD 115200 00014 #define CSR_APP_LOG_INFO(...) sSerialDebug.printf(__VA_ARGS__) 00015 00016 /* appliation commands */ 00017 typedef enum AppCmd { 00018 APP_CMD_IDLE, // No special command 00019 APP_CMD_HELP, // Show the supported commands 00020 APP_CMD_START, // Start location 00021 APP_CMD_STOP, // Stop location 00022 APP_CMD_PM_FULL, // Set full power mode 00023 APP_CMD_PM_PTF, // Set low power PTF mode 00024 APP_CMD_PTF_GETPOS, // Get location immediately in low power PTF mode 00025 APP_CMD_RESET, // Debug command, pull reset pin high level 00026 } eAppCmd; 00027 00028 static void _AppShowCmd(void); 00029 static void _AppBtnPushed(void); 00030 static void _ConsoleRxHandler(void); 00031 static void _AppCmdProcess(char *pCmd); 00032 00033 static int sAppCmd = APP_CMD_IDLE; 00034 00035 static DigitalOut sLedLocOn(LOC_LED1); 00036 static DigitalOut sLedPosReport(LOC_LED2); 00037 static InterruptIn sBtn(PINMAP_GPIO_BTN); 00038 static DigitalOut sPinTest(PINMAP_GPIO_TEST); 00039 00040 Serial sSerialDebug(USBTX, USBRX); 00041 00042 void 00043 locationHandler(const GPSProvider::LocationUpdateParams_t *params) 00044 { 00045 if (params->valid) { 00046 /* application specific handling of location data; */ 00047 } 00048 } 00049 00050 int main(void) 00051 { 00052 sLedLocOn = 0; 00053 sLedPosReport = 0; 00054 sPinTest = 1; 00055 sBtn.mode(PullUp); 00056 sBtn.fall(&_AppBtnPushed); 00057 00058 /* initialize the debug serial port */ 00059 sSerialDebug.baud(APP_DBG_PORT_BAUD); 00060 sSerialDebug.attach(&_ConsoleRxHandler); 00061 00062 GPSProvider gps; 00063 gps.setPowerMode(GPSProvider::POWER_FULL); 00064 gps.reset(); 00065 gps.onLocationUpdate(locationHandler); 00066 CSR_APP_LOG_INFO("Success to new csrLocation.\r\n"); 00067 00068 _AppShowCmd(); 00069 00070 while (true) { 00071 switch (sAppCmd) { 00072 case APP_CMD_HELP: 00073 sAppCmd = APP_CMD_IDLE; 00074 _AppShowCmd(); 00075 break; 00076 case APP_CMD_IDLE: 00077 gps.process(); 00078 sleep(); 00079 break; 00080 case APP_CMD_START: 00081 sAppCmd = APP_CMD_IDLE; 00082 CSR_APP_LOG_INFO("start location.\r\n"); 00083 gps.start(); 00084 sLedLocOn = 1; 00085 break; 00086 case APP_CMD_STOP: 00087 sAppCmd = APP_CMD_IDLE; 00088 CSR_APP_LOG_INFO("stop location.\r\n"); 00089 gps.stop(); 00090 sLedLocOn = 0; 00091 break; 00092 case APP_CMD_RESET: 00093 sAppCmd = APP_CMD_IDLE; 00094 gps.reset(); 00095 CSR_APP_LOG_INFO("reset on.\r\n"); 00096 break; 00097 case APP_CMD_PTF_GETPOS: 00098 CSR_APP_LOG_INFO("lpm get pos.\r\n"); 00099 sAppCmd = APP_CMD_IDLE; 00100 gps.lpmGetImmediateLocation(); 00101 break; 00102 case APP_CMD_PM_FULL: 00103 sAppCmd = APP_CMD_IDLE; 00104 gps.setPowerMode(GPSProvider::POWER_FULL); 00105 CSR_APP_LOG_INFO("fpm set.\r\n"); 00106 break; 00107 case APP_CMD_PM_PTF: 00108 sAppCmd = APP_CMD_IDLE; 00109 gps.setPowerMode(GPSProvider::POWER_LOW); 00110 CSR_APP_LOG_INFO("lpm ptf set.\r\n"); 00111 break; 00112 } 00113 } 00114 } 00115 00116 static void _AppShowCmd(void) 00117 { 00118 CSR_APP_LOG_INFO("Location commands:\r\n"); 00119 CSR_APP_LOG_INFO(" help - help to show supported commands\r\n"); 00120 CSR_APP_LOG_INFO(" start - begin location\r\n"); 00121 CSR_APP_LOG_INFO(" stop - end location\r\n"); 00122 CSR_APP_LOG_INFO(" fpm - full power mode\r\n"); 00123 CSR_APP_LOG_INFO(" ptf - ptf low power mode\r\n"); 00124 CSR_APP_LOG_INFO(" getpos - get location immediately in low power ptf mode\r\n"); 00125 } 00126 00127 static void _AppBtnPushed(void) 00128 { 00129 sAppCmd = APP_CMD_PTF_GETPOS; 00130 // sLedLocOn = !sLedLocOn; 00131 } 00132 00133 static void _ConsoleRxHandler(void) 00134 { 00135 static char cmd[32] = {0}; 00136 char ch; 00137 00138 ch = sSerialDebug.getc(); 00139 sSerialDebug.putc(ch); 00140 if (ch == '\r') { 00141 sSerialDebug.putc('\n'); 00142 if (strlen(cmd) > 0) { 00143 _AppCmdProcess(cmd); 00144 memset(cmd, 0, sizeof(cmd)); 00145 } 00146 } else { 00147 cmd[strlen(cmd)] = ch; 00148 } 00149 } 00150 00151 static void _AppCmdProcess(char *pCmd) 00152 { 00153 if (strcmp(pCmd, "help") == 0) { 00154 sAppCmd = APP_CMD_HELP; 00155 } else if (strcmp(pCmd, "start") == 0) { 00156 sAppCmd = APP_CMD_START; 00157 } else if (strcmp(pCmd, "stop") == 0) { 00158 sAppCmd = APP_CMD_STOP; 00159 } else if (strcmp(pCmd, "fpm") == 0) { 00160 sAppCmd = APP_CMD_PM_FULL; 00161 } else if (strcmp(pCmd, "ptf") == 0) { 00162 sAppCmd = APP_CMD_PM_PTF; 00163 } else if (strcmp(pCmd, "getpos") == 0) { 00164 sAppCmd = APP_CMD_PTF_GETPOS; 00165 } else if (strcmp(pCmd, "reset") == 0) { 00166 sAppCmd = APP_CMD_RESET; 00167 } else { 00168 CSR_APP_LOG_INFO("\r\nUnknown command %s\r\n", pCmd); 00169 } 00170 00171 CSR_APP_LOG_INFO("\r\n"); 00172 }
Generated on Fri Jul 15 2022 11:27:47 by
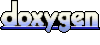