
mainまでいけました カメラのとこからコンパイルできません
Dependencies: mbed CameraUS015sb612-3
base64.h
00001 #if !defined BASE64_H_INC_ 00002 #define BASE64_H_INC_ 00003 00004 #include <string.h> 00005 #include <iostream> 00006 #include <fstream> 00007 00008 using namespace std; 00009 00010 // Base64 Encodeing 00011 class base64 00012 { 00013 int iLen; 00014 char *lpszOutputString; // 結果出力先 00015 static const char *szB64; // Base64変換テーブル 00016 00017 static int FindIndexInB64(char c);//szB64の Base64変換テーブルのなかの、どれか、探し出す。ない場合、-1 00018 public: 00019 base64(); 00020 ~base64(); 00021 const char *Encode(const char *szStr, int iLens = -1); // エンコード(文字列用)。ただし、データの長さを指定すれば、バイナリデータが可能 00022 const char *Decode(const char *szStr); // デコード(文字列用) 00023 int GetLenght() const { return iLen; } // 出力された長さ 00024 const char *Get() const { return lpszOutputString; } // 文字列用の結果を取得 00025 00026 static void Encode(istream& istr, ostream& ostr, int iRet = 76); // istrはバイナリストリーム推奨 00027 // エンコード(ストリーム用) iRetは、何文字目で改行するか。-1なら、改行しない。 00028 static void Decode(istream& istr, ostream& ostr); // ostrはバイナリストリーム推奨 00029 // デコード(ストリーム用) 00030 00031 static void Encode(const char *lpszImputFileName, const char *lpszOutputFileName, int iRet = 76) 00032 { 00033 ifstream f(lpszImputFileName,ios::in | ios::binary); 00034 ofstream of(lpszOutputFileName); 00035 Encode(f,of); 00036 } 00037 static void Decode(const char *lpszImputFileName, const char *lpszOutputFileName) 00038 { 00039 ifstream f(lpszImputFileName); 00040 ofstream of(lpszOutputFileName,ios::out | ios::trunc | ios::binary); 00041 Decode(f,of); 00042 } 00043 }; 00044 00045 #endif // #if !defined BASE64_H_INC_
Generated on Wed Jul 13 2022 22:28:08 by
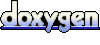