
mainまでいけました カメラのとこからコンパイルできません
Dependencies: mbed CameraUS015sb612-3
base64.cpp
00001 #include "base64.h" 00002 00003 const char *base64::szB64 = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/="; 00004 00005 base64::base64() : lpszOutputString(NULL) 00006 { 00007 } 00008 00009 base64::~base64() 00010 { 00011 if(lpszOutputString) 00012 delete[] lpszOutputString; 00013 } 00014 00015 const char *base64::Encode(const char *szStr, int iLens) 00016 { 00017 int i,j; 00018 00019 if(lpszOutputString) 00020 delete[] lpszOutputString; 00021 00022 if(iLens == -1) 00023 iLen = strlen(szStr); 00024 else 00025 iLen = iLens; 00026 00027 lpszOutputString = 00028 new char[(int)((double)(iLen)*1.5)+10]; 00029 for(i = 0,j = 0; i < (iLen - (iLen % 3)); i+=3) 00030 { 00031 lpszOutputString[j] = szB64[(szStr[i] & 0xfc) >> 2]; 00032 lpszOutputString[j+1] = szB64[((szStr[i] &0x03) << 4) | 00033 ((szStr[i+1] & 0xf0) >> 4)]; 00034 lpszOutputString[j+2] = szB64[((szStr[i+1] & 0x0f) <<2 ) | 00035 ((szStr[i+2] & 0xc0) >> 6)]; 00036 lpszOutputString[j+3] = szB64[(szStr[i+2] & 0x3f)]; 00037 j += 4; 00038 } 00039 i = iLen-(iLen % 3); // 残りのサイズを計算 00040 switch(iLen % 3) 00041 { 00042 case 2: // 1文字分パディングが必要 00043 { 00044 lpszOutputString[j] = szB64[(szStr[i] & 0xfc) >> 2]; 00045 lpszOutputString[j+1] = szB64[((szStr[i] &0x03) << 4) | 00046 ((szStr[i+1] & 0xf0) >> 4)]; 00047 lpszOutputString[j+2] = szB64[((szStr[i+1] & 0x0f) <<2 )]; 00048 lpszOutputString[j+3] = szB64[64]; // Pad 00049 lpszOutputString[j+4] = '\0'; 00050 } 00051 break; 00052 case 1: // 2文字分パディングが必要 00053 { 00054 lpszOutputString[j] = szB64[(szStr[i] & 0xfc) >> 2]; 00055 lpszOutputString[j+1] = szB64[((szStr[i] &0x03) << 4)]; 00056 lpszOutputString[j+2] = szB64[64]; // Pad 00057 lpszOutputString[j+3] = szB64[64]; // Pad 00058 lpszOutputString[j+4] = '\0'; 00059 } 00060 break; 00061 } 00062 lpszOutputString[j+4] = '\0'; 00063 00064 return lpszOutputString; 00065 } 00066 00067 void base64::Encode(istream& istr, ostream& ostr, int iRet) 00068 { 00069 int i; 00070 char c[3]; 00071 00072 i = 0; 00073 while(!istr.eof()) 00074 { 00075 c[0] = c[1] = c[2] = '\0'; 00076 istr.read(c,3); 00077 00078 ostr << szB64[(c[0] & 0xfc) >> 2]; 00079 i++; if(i >= iRet && iRet != -1){ ostr << endl; i = 0; } 00080 ostr << szB64[((c[0] &0x03) << 4) | ((c[1] & 0xf0) >> 4)]; 00081 i++; if(i >= iRet && iRet != -1){ ostr << endl; i = 0; } 00082 if(istr.gcount() == 1) 00083 ostr << szB64[64]; 00084 else 00085 ostr << szB64[((c[1] & 0x0f) <<2 ) | ((c[2] & 0xc0) >> 6)]; 00086 i++; if(i >= iRet && iRet != -1){ ostr << endl; i = 0; } 00087 if(istr.gcount() == 3) 00088 ostr << szB64[(c[2] & 0x3f)]; 00089 else 00090 ostr << szB64[64]; 00091 i++; if(i >= iRet && iRet != -1){ ostr << endl; i = 0; } 00092 } 00093 ostr.flush(); 00094 } 00095 00096 00097 int base64::FindIndexInB64(char c) 00098 { 00099 int index = 0; 00100 while(szB64[index] != '\0' && szB64[index] != c) 00101 index++; 00102 00103 if(szB64[index] == '\0') 00104 return -1; // 見つからず 00105 00106 return index; // 見つかった。 00107 } 00108 00109 const char *base64::Decode(const char *szStr) 00110 { 00111 //lpszOutputString 00112 int i, j, l,iWriteCount,len; 00113 char c; 00114 char buf[4]; 00115 00116 len = iLen = strlen(szStr); 00117 if(lpszOutputString) 00118 delete[] lpszOutputString; 00119 00120 iLen = (int)(((double)(iLen)/4.0)*3.0) + 4; 00121 00122 lpszOutputString = new char[iLen]; 00123 00124 for(i = 0, j = 0; i < len; i+=4) 00125 { 00126 iWriteCount = 3; 00127 for(l = 0; l < 4 && i+l<len; l++) 00128 { 00129 c = szStr[i+l]; 00130 if(c == szB64[64]) // Pad 00131 iWriteCount--; 00132 else { 00133 buf[l] = FindIndexInB64(c); 00134 //if(buf[l] == -1) error!; 00135 } 00136 } 00137 lpszOutputString[j] = ((buf[0] << 2) & 0xfc) | ((buf[1] >> 4) & 0x03); 00138 if(iWriteCount >= 2) 00139 lpszOutputString[j+1] = ((buf[1] << 4) & 0xf0) | ((buf[2] >> 2) & 0x0f); 00140 if(iWriteCount == 3) 00141 lpszOutputString[j+2] = ((buf[2] << 6) & 0xc0) | (buf[3] & 0x3f); 00142 j+=iWriteCount; 00143 } 00144 iLen = j; 00145 lpszOutputString[j] = '\0'; 00146 00147 return lpszOutputString; 00148 } 00149 00150 void base64::Decode(istream& istr, ostream& ostr) 00151 { 00152 int i,iWriteCount; 00153 char c; 00154 char buf[4]; 00155 char out[3]; 00156 00157 while(1) 00158 { 00159 iWriteCount = 3; 00160 for(i = 0; i < 4; i++) 00161 { 00162 istr >> c; 00163 if(istr.eof()) 00164 { 00165 ostr.flush(); 00166 return; 00167 } 00168 if(c == szB64[64]) // Pad 00169 iWriteCount--; 00170 else { 00171 buf[i] = FindIndexInB64(c); 00172 //if(buf[i] == -1) error!; 00173 } 00174 } 00175 out[0] = ((buf[0] << 2) & 0xfc) | ((buf[1] >> 4) & 0x03); 00176 out[1] = ((buf[1] << 4) & 0xf0) | ((buf[2] >> 2) & 0x0f); 00177 out[2] = ((buf[2] << 6) & 0xc0) | (buf[3] & 0x3f); 00178 ostr.write(out, iWriteCount); 00179 } 00180 }
Generated on Wed Jul 13 2022 22:28:08 by
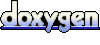