
mainまでいけました カメラのとこからコンパイルできません
Dependencies: mbed CameraUS015sb612-3
JPEGCamera.cpp
00001 /* Arduino JPEGCamera Library 00002 * Copyright 2010 SparkFun Electronic 00003 * Written by Ryan Owens 00004 * Modified by arms22 00005 * Ported to mbed by yamaguch 00006 */ 00007 00008 #include "JPEGCamera.h" 00009 00010 #define min(x, y) ((x) < (y)) ? (x) : (y) 00011 00012 const int RESPONSE_TIMEOUT = 500; 00013 const int DATA_TIMEOUT = 1000; 00014 00015 JPEGCamera::JPEGCamera(PinName tx, PinName rx) : Serial(tx, rx) { 00016 printf("AA\r\n"); 00017 baud(38400); 00018 state = READY; 00019 } 00020 00021 bool JPEGCamera::setPictureSize(JPEGCamera::PictureSize size, bool doReset) { 00022 char buf[9] = {0x56, 0x00, 0x31, 0x05, 0x04, 0x01, 0x00, 0x19, (char) size}; 00023 int ret = sendReceive(buf, sizeof buf, 5); 00024 00025 if (ret == 5 && buf[0] == 0x76) { 00026 if (doReset) 00027 reset(); 00028 return true; 00029 } else 00030 return false; 00031 } 00032 00033 bool JPEGCamera::isReady() { 00034 return state == READY; 00035 } 00036 00037 bool JPEGCamera::isProcessing() { 00038 return state == PROCESSING; 00039 } 00040 00041 bool JPEGCamera::takePicture(char *filename) { 00042 if (state == READY) { 00043 fp = fopen(filename, "wb"); 00044 if (fp != 0) { 00045 if (takePicture()) { 00046 imageSize = getImageSize(); 00047 address = 0; 00048 state = PROCESSING; 00049 } else { 00050 fclose(fp); 00051 printf("takePicture(%s) failed", filename); 00052 state = ERROR; 00053 } 00054 } else { 00055 printf("fopen() failed"); 00056 state = ERROR; 00057 } 00058 } 00059 return state != ERROR; 00060 } 00061 00062 bool JPEGCamera::processPicture() { 00063 if (state == PROCESSING) { 00064 if (address < imageSize) { 00065 char data[1024]; 00066 int size = readData(data, min(sizeof(data), imageSize - address), address); 00067 int ret = fwrite(data, size, 1, fp); 00068 if (ret > 0) 00069 address += size; 00070 if (ret == 0 || address >= imageSize) { 00071 stopPictures(); 00072 fclose(fp); 00073 wait(0.1); // ???? 00074 state = ret > 0 ? READY : ERROR; 00075 } 00076 } 00077 } 00078 00079 return state == PROCESSING || state == READY; 00080 } 00081 00082 bool JPEGCamera::reset() { 00083 char buf[4] = {0x56, 0x00, 0x26, 0x00}; 00084 int ret = sendReceive(buf, sizeof buf, 4); 00085 if (ret == 4 && buf[0] == 0x76) { 00086 wait(4.0); 00087 state = READY; 00088 } else { 00089 state = ERROR; 00090 } 00091 return state == READY; 00092 } 00093 00094 bool JPEGCamera::takePicture() { 00095 char buf[5] = {0x56, 0x00, 0x36, 0x01, 0x00}; 00096 int ret = sendReceive(buf, sizeof buf, 5); 00097 00098 return ret == 5 && buf[0] == 0x76; 00099 } 00100 00101 bool JPEGCamera::stopPictures() { 00102 char buf[5] = {0x56, 0x00, 0x36, 0x01, 0x03}; 00103 int ret = sendReceive(buf, sizeof buf, 5); 00104 00105 return ret == 4 && buf[0] == 0x76; 00106 } 00107 00108 int JPEGCamera::getImageSize() { 00109 char buf[9] = {0x56, 0x00, 0x34, 0x01, 0x00}; 00110 int ret = sendReceive(buf, sizeof buf, 9); 00111 00112 //The size is in the last 2 characters of the response. 00113 return (ret == 9 && buf[0] == 0x76) ? (buf[7] << 8 | buf[8]) : 0; 00114 } 00115 00116 int JPEGCamera::readData(char *dataBuf, int size, int address) { 00117 char buf[16] = {0x56, 0x00, 0x32, 0x0C, 0x00, 0x0A, 0x00, 0x00, 00118 address >> 8, address & 255, 0x00, 0x00, size >> 8, size & 255, 0x00, 0x0A 00119 }; 00120 int ret = sendReceive(buf, sizeof buf, 5); 00121 00122 return (ret == 5 && buf[0] == 0x76) ? receive(dataBuf, size, DATA_TIMEOUT) : 0; 00123 } 00124 00125 int JPEGCamera::sendReceive(char *buf, int sendSize, int receiveSize) { 00126 while (readable()) getc(); 00127 00128 for (int i = 0; i < sendSize; i++) putc(buf[i]); 00129 00130 return receive(buf, receiveSize, RESPONSE_TIMEOUT); 00131 } 00132 00133 int JPEGCamera::receive(char *buf, int size, int timeout) { 00134 timer.start(); 00135 timer.reset(); 00136 00137 int i = 0; 00138 while (i < size && timer.read_ms() < timeout) { 00139 if (readable()) 00140 buf[i++] = getc(); 00141 } 00142 00143 return i; 00144 }
Generated on Wed Jul 13 2022 22:28:08 by
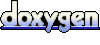